Accelerating Entity Framework with ChatGPT's Lazy Loading: A Powerful Combination for Efficient Data Retrieval
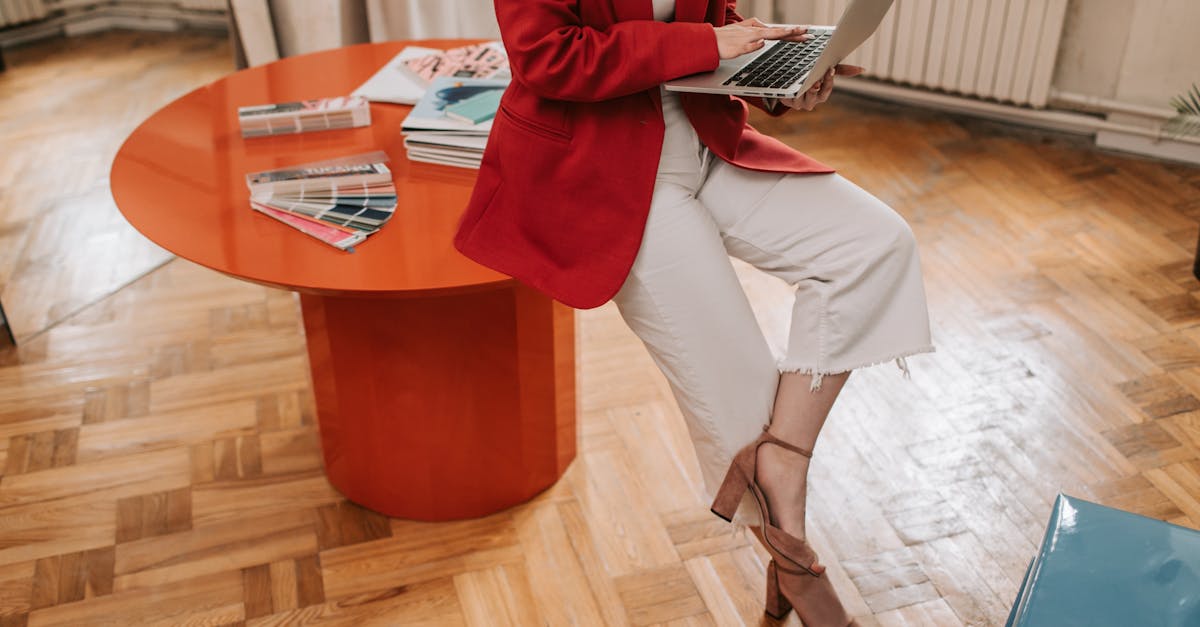
The Entity Framework is a popular Object-Relational Mapping (ORM) framework developed by Microsoft. It provides a convenient way to work with databases using domain-specific objects instead of writing complex SQL queries.
Lazy loading is a technique used in Entity Framework to load related entities only when they are accessed. This allows for better performance as it avoids loading unnecessary data upfront and reduces the overall database query execution time.
What is Lazy Loading?
Lazy loading is a concept where related entities are not loaded with the primary entity immediately, but instead, they are loaded on-demand when accessed by the application. This delayed loading helps reduce the initial data retrieval time and enhances the performance of the application.
How Does Lazy Loading Work in Entity Framework?
In Entity Framework, lazy loading is enabled by default when using the Entity Framework Core or EF6 versions. It works by creating proxy classes at runtime that inherit from the entity classes. These proxy classes override the navigation properties to intercept property access and load the related entities if they have not been loaded yet.
When lazy loading is enabled, accessing a navigation property triggers a database query to load the related entities automatically. For example, if you have an entity called "User" with a navigation property "Orders," accessing the "Orders" property would issue a query to load the associated orders only when it is accessed for the first time.
Enabling Lazy Loading in Entity Framework
To enable lazy loading in Entity Framework, you need to install the required Entity Framework NuGet packages and configure the lazy loading behavior. Here are the steps:
- Install the Entity Framework NuGet package in your project.
- Configure lazy loading in your DbContext class by overriding the OnConfiguring method:
- Mark the navigation properties as virtual in your entity classes to enable lazy loading:
Install-Package Microsoft.EntityFrameworkCore
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder.UseLazyLoadingProxies();
}
public class User
{
public int Id { get; set; }
public string Name { get; set; }
public virtual ICollection<Order> Orders { get; set; }
}
With the above steps, lazy loading is enabled in Entity Framework, and related entities will be loaded on-demand when accessed. It is essential to note that lazy loading is only available for navigation properties, including one-to-many, many-to-many, and one-to-one relationships.
Benefits of Lazy Loading
Lazy loading offers several benefits when working with Entity Framework:
- Improved Performance: By loading related entities only when required, lazy loading reduces the initial data retrieval time and improves the overall performance of the application.
- Reduced Memory Usage: Lazy loading avoids loading all related entities upfront, which helps conserve memory resources, especially when dealing with large datasets.
- Simpler Data Access Code: Lazy loading simplifies the code by automatically handling the loading of related entities, eliminating the need for explicit queries to retrieve them.
Conclusion
Lazy loading is a powerful feature provided by Entity Framework that improves the performance and memory usage of your applications. By implementing lazy loading, you can achieve better data retrieval times, handle large datasets efficiently, and simplify the data access code.
With the steps mentioned above, you can enable lazy loading in Entity Framework and take advantage of its benefits. So, go ahead and leverage this feature in your application development process with Entity Framework.
Comments:
Thank you all for your comments on my article! I'm glad you found it interesting. If you have any questions or further insights, feel free to ask.
Great article, Cantrina! I've always wondered about the combination of Lazy Loading and Entity Framework. Definitely going to try it out now.
David, let me know how it goes when you try it out. I'm curious to hear your experience with Lazy Loading in EF.
Adam, sure! I'll share my experience once I've tried it out. Planning to implement it in our current project.
Looking forward to hearing about your experience, David. It would be helpful to know if you encounter any challenges or best practices to keep in mind.
Lazy Loading has definitely helped optimize our data retrieval in certain scenarios. It's a powerful feature of EF.
Emma, can you share an example of a scenario where Lazy Loading significantly improved data retrieval performance?
Olivia, one scenario where I found Lazy Loading useful is when loading related entities that are not always needed. Lazy Loading defers the loading until the related entities are accessed, reducing unnecessary queries upfront.
Thanks, Emma! That scenario makes sense. Lazy Loading can be a great optimization technique when used appropriately.
I'm impressed with the performance gains achieved by combining Lazy Loading with EF. Thanks for sharing!
The combination of Lazy Loading and EF is indeed powerful. It helps avoid unnecessary database queries and improves overall efficiency.
Lazy Loading can be a double-edged sword. While it improves performance in certain scenarios, it can also lead to potential performance issues if not used carefully. It's important to understand its impact on the overall application.
Nice article, Cantrina! Lazy Loading can be handy, but it's crucial to be aware of the potential pitfalls it may bring if misused.
Great write-up, Cantrina, and an interesting combination indeed. I'll definitely be exploring this further for my projects.
Cantrina, your article provided a fresh perspective on using Lazy Loading with Entity Framework. I appreciate the insights you shared!
I've been skeptical of Lazy Loading in the past, but based on your article, Cantrina, I'm convinced to give it another try.
The combination of Lazy Loading and Entity Framework sounds promising. Definitely considering it for my next project.
Lazy Loading can be a blessing when used properly. It's important to have a good understanding of its behavior to leverage the benefits effectively.
Thank you all for your positive feedback and engagement! It's great to see your interest in Lazy Loading and Entity Framework. Keep exploring and experimenting with this powerful combination!
Definitely, Cantrina. Understanding the trade-offs and being mindful of performance implications are key to utilizing Lazy Loading effectively.
Exactly, Sarah. Lazy Loading can lead to unexpected performance bottlenecks if not used judiciously. Striking a balance is important.
I couldn't agree more, Javier. It's crucial to understand Lazy Loading's implications and use it in the appropriate context to avoid potential issues.
You're welcome, Cantrina. Your article was informative and well-presented. Lazy Loading is now on my list of techniques to explore further.
Thanks, Cantrina! Your article gave me the confidence to embrace Lazy Loading and reap its benefits when working with Entity Framework.
That's wonderful, Alex! I'm glad I could inspire you to explore Lazy Loading further. All the best with your upcoming project!
Thank you, Cantrina! I'm excited to implement Lazy Loading and witness the performance improvements firsthand. Your article was a great starting point.
You're welcome, Cantrina! It was a valuable discussion. I'll definitely keep your insights in mind while working with Lazy Loading and EF.
Thank you, Cantrina, for the informative discussion. I'm looking forward to applying Lazy Loading wisely in my projects.
Absolutely, Cantrina! Leveraging the power of Lazy Loading with EF can lead to significant performance gains. Thanks again for sharing your insights.
Indeed, Olivia. Cantrina's article made me realize I might have overlooked the full potential of Lazy Loading in the past.
I'm glad your article convinced me to give Lazy Loading another shot. It seems like a valuable technique when used properly.
Excellent article, Cantrina! Lazy Loading has been on my radar, and your article provided a comprehensive overview of its benefits.
Lazy Loading combined with Entity Framework sounds like a winning combination. Looking forward to employing it in my upcoming project.
I appreciate all your kind words, Marie and Alex! Lazy Loading can indeed be a powerful addition to your toolbox when working with EF.
Absolutely, Cantrina! Proper usage and understanding of Lazy Loading's impact are key to making the most out of it.
You're welcome, Cantrina! Your article was well-written, and it shed light on the potential benefits of Lazy Loading with EF.
Absolutely! Understanding the nuances of Lazy Loading can help improve the overall performance and efficiency of our applications.
Thank you for your insightful comments, everyone! It's been a pleasure discussing the advantages of Lazy Loading in conjunction with Entity Framework. If you have any more questions or thoughts, feel free to share.
Exactly, Cantrina! The use of Lazy Loading can be a game-changer for optimizing data retrieval. Thanks for sharing your knowledge.
Cantrina, your article provided a comprehensive understanding of Lazy Loading with EF. I appreciate your effort in bringing this topic to light.