Boost C++: Unleashing the Power of Concurrency with ChatGPT
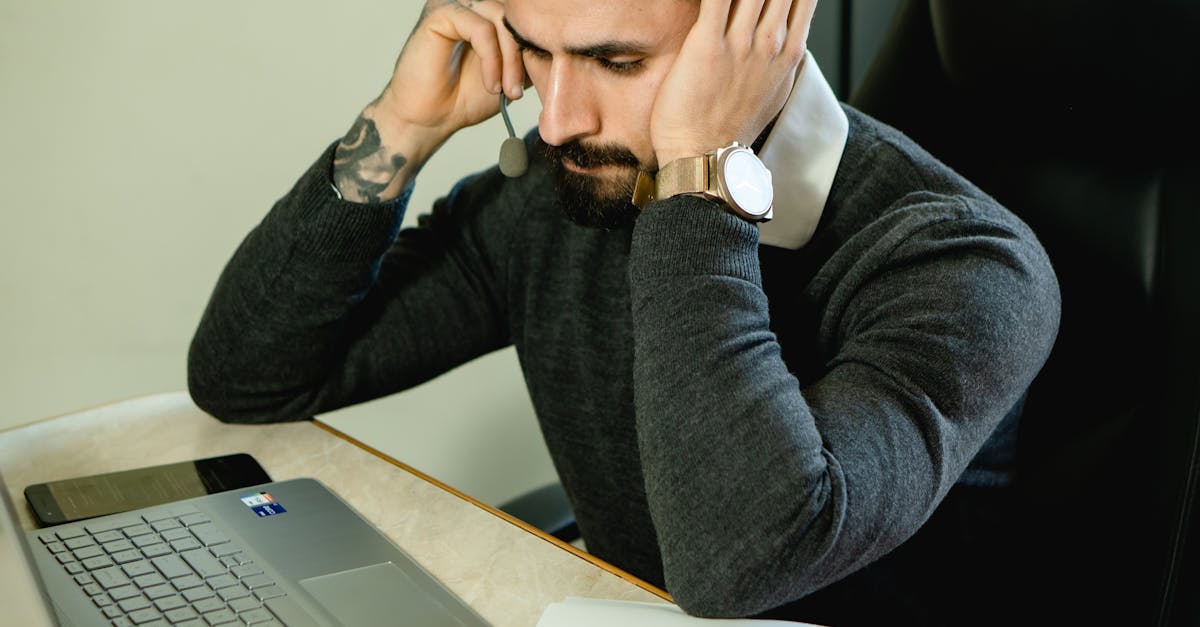
Boost C++ is a widely used set of C++ libraries that provides support for various aspects of the language and its standard library. Boost C++ offers a range of libraries for different purposes, including concurrency and multithreading support.
What is Concurrency and Multithreading?
Concurrency refers to the ability of an application to execute multiple tasks simultaneously. Multithreading, on the other hand, is a specific technique for achieving concurrency by breaking a program into multiple threads of execution. Each thread represents an independent sequence of instructions that can be scheduled and executed independently by the operating system.
Why Boost C++ for Concurrency and Multithreading?
Boost C++ provides a set of libraries that simplify the implementation of concurrency and multithreading in C++. These libraries offer high-level abstractions and tools that can assist developers in efficiently handling multithreaded programs, such as synchronization primitives, thread-safe data structures, and algorithms for parallel computing.
Boost C++ Libraries for Concurrency and Multithreading
Boost.Thread: This library provides a portable C++ multithreading interface. It offers classes and functions for creating and managing threads, synchronization mechanisms, and thread-local storage. Boost.Thread allows developers to write concurrent programs that can take full advantage of the available hardware resources.
Boost.Atomic: This library provides atomic operations that are essential for writing lock-free concurrent code. Atomic operations ensure that memory accesses are performed atomically, without interference from other threads. Boost.Atomic supports a wide range of atomic types and operations, making it easier to write efficient and correct concurrent algorithms.
Boost.Chrono: This library provides timers and clocks that can be used for measuring and controlling time in concurrent programs. It offers high-resolution clocks for accurate time measurements, as well as timers that can be used to trigger actions periodically or after a certain duration.
Boost.Asio: Although primarily known for its networking capabilities, Boost.Asio also provides support for concurrent programming. It offers asynchronous I/O operations, timers, and synchronization mechanisms that can be used to build high-performance, scalable, and concurrent applications.
Example Usage of Boost C++ for Concurrency and Multithreading
Let's consider a simple example that demonstrates the usage of Boost.Thread for concurrency and multithreading. Suppose we have a computationally intensive task that we want to execute concurrently using multiple threads. We can achieve this using Boost.Thread as follows:
#include
#include
// Function that performs the computationally intensive task
void compute(int id)
{
std::cout << "Executing task " << id << std::endl;
// Perform computation
}
int main()
{
const int numThreads = 4;
boost::thread_group threads;
for (int i = 0; i < numThreads; ++i)
{
threads.create_thread(boost::bind(&compute, i));
}
threads.join_all();
return 0;
}
In this example, we first include the necessary Boost.Thread headers. We define a function compute
that represents our computationally intensive task. Inside this function, we assume some computation is performed.
In the main
function, we create a boost::thread_group
to manage multiple threads. We use a loop to create and start numThreads
threads, each executing the compute
function with a different ID. Finally, we call join_all
on the thread group to wait for all threads to complete before ending the program.
With Boost.Thread, we can easily create and manage multiple threads, allowing our computationally intensive task to execute concurrently and maximize the utilization of system resources.
Conclusion
Boost C++ provides a powerful set of libraries for concurrency and multithreading. These libraries offer high-level abstractions and tools that simplify the implementation of concurrent programs, allowing developers to write efficient and correct multithreaded code. Boost C++ is a valuable resource for those seeking to gain insights into concurrency and multithreading in C++.
Comments:
Thank you all for joining the discussion! I'm excited to hear your thoughts on Boost C++ and ChatGPT's Concurrency.
Concurrency is such a crucial aspect of modern programming languages. Looking forward to reading more about how Boost C++ and ChatGPT combine forces.
I've recently started learning C++. Can anyone explain the basics behind concurrency and how it can be beneficial?
Concurrency refers to the ability of a program to execute multiple tasks simultaneously. It allows for better utilization of system resources and can improve performance in certain scenarios.
Thanks, David! Are there any challenges or considerations to keep in mind when working with concurrency in C++?
I'm curious to know more about ChatGPT's role in Boost C++. How does it contribute to concurrency?
@Julia ChatGPT utilizes Boost C++'s concurrency features to enhance its performance in handling multiple chat requests simultaneously, providing efficient and quick responses.
@Maribeth Wansley Thanks for the insight! It's great to see how ChatGPT leverages Boost C++'s concurrency features to provide efficient chat responses.
@Maribeth Wansley I'm amazed at the seamless integration of Boost C++ and ChatGPT's concurrency. It opens up a world of possibilities for creating responsive and powerful applications.
Boost C++ is an amazing library with so many useful features. Excited to see how ChatGPT's concurrency takes it to the next level.
I've been a fan of Boost C++ for a while now. It'll be interesting to learn about the specific use cases of ChatGPT's concurrency.
Concurrency in C++ can be complex, but it's essential for optimizing program execution. Boost C++ seems like a valuable tool to achieve that.
@Nathan I completely agree! Boost C++ is a reliable and efficient library for achieving optimal concurrency in C++ programs.
As a programmer, I'm always excited about new ways to improve concurrency in my applications. Looking forward to exploring Boost C++ and ChatGPT's capabilities.
Boost C++ has been a reliable companion for me. The addition of ChatGPT's concurrency sounds promising. Can't wait to try it out!
I recommend checking out the Boost C++ documentation for detailed information on how to leverage its concurrency features effectively.
Can anyone share some practical examples of how they have used Boost C++ and ChatGPT's concurrency in their projects?
@Adam In one of my projects, I used Boost C++ and ChatGPT's concurrency to implement a real-time chat support system. It allowed us to handle multiple customer queries simultaneously without significantly impacting performance.
@Brandon That's impressive! Implementing real-time chat support with the efficiency of Boost C++ and ChatGPT's concurrency must have improved the user experience significantly.
@Adam In one project, we used Boost C++ and ChatGPT's concurrency to create a recommendation system. It allowed us to process user input concurrently and provide personalized recommendations much faster.
@Julia That sounds fascinating! Boost C++ and ChatGPT's concurrency must have significantly improved the responsiveness of the recommendation system. Great use case!
@Adam Absolutely! The real-time chat support system became much more reliable with Boost C++ and ChatGPT's concurrency. A win-win for both customers and support agents.
@Brandon Real-time chat support with Boost C++ and ChatGPT's concurrency sounds impressive. It must have made a significant impact on customer satisfaction and response times.
I'm also interested in hearing some real-world use cases where Boost C++ and ChatGPT's concurrency have made a significant impact.
@Emily One challenge of working with concurrency in C++ is handling shared resources to avoid race conditions and deadlocks. Proper synchronization mechanisms like mutexes and condition variables become crucial.
@Thomas I completely agree. Boost C++ already empowers developers with its extensive features, and the integration of ChatGPT's concurrency takes it to a whole new level.
@Karen Definitely! It's exciting to witness the continuous evolution of Boost C++ and its ability to address modern concurrency challenges.
@Thomas Thanks for highlighting the challenges. It's essential to handle shared resources correctly to ensure the reliability of concurrent programs.
@Emily You're welcome! Indeed, handling shared resources appropriately and ensuring proper synchronization are crucial aspects of writing reliable concurrent programs.
@Emily You're welcome! Feel free to reach out if you have any more questions about concurrency in C++. Happy to help!
@David Thank you! I appreciate your willingness to help. I'll make sure to explore more resources on concurrency in C++ and Boost C++.
@Thomas Absolutely! Boost C++ has always been a game-changer, and bringing ChatGPT's concurrency capabilities into the mix is truly exciting.
@Karen Boost C++ enhances the scalability and performance of C++ applications, especially when it comes to concurrent execution. It's a great library to leverage.
Boost C++ is always my go-to library for powerful features. Excited to dive into ChatGPT's concurrency integration and its potential benefits.
Concurrency can be a bit challenging, especially when it comes to debugging. I wonder if Boost C++ offers any debugging tools or features specifically for concurrency.
@Laura Boost C++ offers several debugging tools like 'lock_debug' and 'detect_deadlock' to help identify and debug concurrency-related issues. They can be handy when working on concurrent projects.
@Laura Boost C++ does indeed provide debugging tools like 'lock_debug' to assist with identifying and resolving concurrency-related bugs.
It's crucial to ensure thread safety when working with concurrency in C++. Boost C++'s concurrency features provide mechanisms to handle synchronization and avoid data races.
Boost C++ is an incredible library that simplifies complex tasks. Looking forward to exploring its concurrency capabilities with ChatGPT.
Concurrency can lead to performance improvements, but it can also introduce new challenges like thread synchronization and resource management. Boost C++ seems like a reliable solution for tackling such complexities.
@Sarah That's true! Boost C++ provides synchronization objects like mutexes and condition variables, ensuring thread-safe concurrent programming.
Understanding the underlying threading models and types of locks provided by Boost C++ is vital for effective concurrency programming. It pays off in terms of performance and robustness.
@Emily Absolutely! Matching the right threading model and lock type in Boost C++ to the specific use case greatly enhances the potential benefits of concurrency.
@Emily When working with concurrency, you need to handle shared resources carefully to prevent conflicts. Mutexes, semaphores, or other synchronization mechanisms can help ensure data integrity.
@David Thanks for the explanation! I'll keep that in mind while exploring concurrency in C++. Appreciate the insight!
I've been meaning to delve deeper into C++ concurrency. Boost C++ seems like a good starting point. Excited to learn more about ChatGPT's impact on it.
Concurrency can be complex but rewarding. Boost C++ and ChatGPT provide powerful tools to explore and unleash the potential of concurrent programming.
Boost C++ shines in its ability to provide portable and efficient tools for concurrent programming. Pairing it with ChatGPT takes it to new heights.
Concurrency in C++ can be challenging, but with the right libraries like Boost C++ and innovative approaches like ChatGPT's integration, it becomes more accessible to developers.
Thank you all for the engaging discussion! Your experiences and insights on Boost C++ and ChatGPT's concurrency have been enlightening. Keep exploring and harnessing the power of concurrency!