Boosting Performance in Angular.js: Leveraging ChatGPT for Optimal Performance Optimization
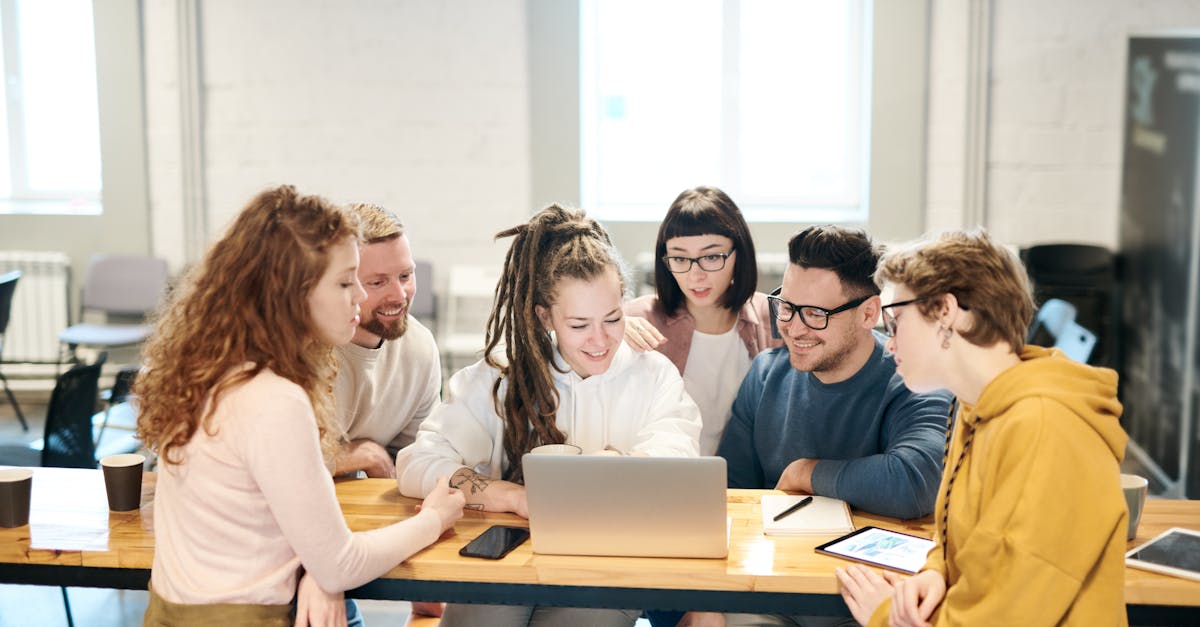
AngularJS is a popular JavaScript framework used for building web applications. While it offers many powerful features, it can also be prone to performance issues if not optimized correctly. This article aims to provide guidance on how to optimize the performance of AngularJS applications.
1. Minify and Concatenate Files
Minification and concatenation of JavaScript and CSS files can significantly improve the performance of your AngularJS application. Minifying your code reduces the file size by removing unnecessary whitespaces, comments, and renaming variables. Concatenation combines multiple files into a single file, reducing the number of network requests required to load your application.
2. Use AngularJS Dependency Injection
AngularJS has a built-in dependency injection system that manages the instantiation of your application's components. By using dependency injection, you can ensure that only the necessary components are loaded at runtime, reducing the initial load time of your application. Avoid manually instantiating services or controllers, and let AngularJS handle the dependencies for you.
3. Limit Two-Way Data Binding
Two-way data binding in AngularJS can be convenient, but it can contribute to performance issues, especially when working with large datasets. Consider using one-way data binding (using the "ng-bind" directive) or one-time binding (using double curly brackets "{{::variable}}") where applicable. This reduces the number of digest cycles and improves the overall performance of your application.
4. Use ng-cloak Directive
The "ng-cloak" directive prevents the display of AngularJS expressions before the application has fully loaded and compiled. This directive can be useful in preventing a "flash of unstyled content" (FOUC) effect, where uncompiled AngularJS expressions are briefly visible on the page. Use the "ng-cloak" directive along with the corresponding CSS to hide the uncompiled expressions until they are ready to be rendered.
5. Enable AngularJS Production Mode
AngularJS provides a production mode that disables debug features and improves the performance of your application. To enable production mode, set the "ng-app" directive to include the "ng-csp" attribute. This will disable features like debug information and assertions, resulting in a faster and more optimized application.
6. Optimize ng-repeat Loops
AngularJS's "ng-repeat" directive can be a performance bottleneck, especially when working with large datasets. To optimize ng-repeat loops, use the "track by" clause to specify a unique identifier for each item. This helps AngularJS to efficiently update the DOM when changes occur. Additionally, consider using pagination or virtual scrolling to limit the number of items rendered at once and improve rendering performance.
7. Use Custom AngularJS Directives
Custom directives allow you to encapsulate reusable functionality in AngularJS. By creating custom directives, you can optimize performance by eliminating unnecessary AngularJS features or by implementing optimizations specific to your application. Break down complex components into smaller, more manageable directives to improve reusability and performance.
8. Optimize AJAX Calls
When making AJAX calls in AngularJS, optimize the requests to minimize response times. Use techniques like server-side pagination, caching, and gzip compression to reduce the size and round-trip time of your AJAX responses. Additionally, consider using AngularJS's built-in HTTP interceptors to intercept and modify HTTP requests and responses, providing further opportunities for optimization.
9. Defer or Asynchronously Load Scripts
To improve the initial load time of your AngularJS application, consider deferring or asynchronously loading non-critical scripts. This allows your main content to load and render quickly, while secondary scripts are loaded in the background. Use techniques like async and defer attributes, or dynamically load scripts using JavaScript to control the loading behavior.
10. Use the AngularJS Profiler
AngularJS provides a built-in profiler tool that helps you identify performance bottlenecks and optimize your application. By enabling the AngularJS profiler, you can monitor the digest cycle, watch expressions, and performance of individual components. Use the profiler to identify areas of your application that can be optimized and improve the overall performance of your AngularJS application.
By following these optimization techniques, you can ensure that your AngularJS application performs at its best. Remember to measure and benchmark the performance of your application before and after implementing optimizations to track the improvements. Happy optimizing!
Comments:
Thank you all for taking the time to read my article on boosting performance in Angular.js. I'm excited to discuss this topic with you!
Great article, Rowena! I found your tips on leveraging ChatGPT for performance optimization really insightful. It's amazing how AI can help us improve Angular.js applications. Keep up the good work!
Hey Steve, I couldn't agree more. Rowena did a fantastic job explaining how ChatGPT can be used as a tool for performance optimization in Angular.js. I'm excited to implement some of these strategies in my next project.
Thank you, Alex! I'm thrilled to hear that you found the strategies useful. If you encounter any challenges during implementation, feel free to ask for help. Good luck with your project!
Thanks for offering your help, Rowena! I'll definitely reach out if I encounter any issues during implementation. Your support is greatly appreciated!
I completely agree, Steve! The potential of AI in performance optimization is fascinating. Rowena, your article was well-written and informative. I look forward to learning more about leveraging ChatGPT in Angular.js projects.
Sophie, I'm glad you agree with me! Rowena's article was a valuable resource, and I'm excited to see how AI technologies like ChatGPT continue to shape the future of web development.
This article was a great read, Rowena. I appreciate the practical examples you provided. It really helped me understand how to leverage ChatGPT in Angular.js applications for optimal performance. Thank you!
Thanks, Rachel! I'm glad you found the practical examples helpful. It's always rewarding to know that my articles make a difference. If you have any specific questions or need further clarification, feel free to ask!
I have to admit, I was initially skeptical about the effectiveness of leveraging AI for performance optimization. But after reading your article, Rowena, I'm convinced that ChatGPT can be a valuable tool in Angular.js development. Thank you for changing my perspective!
I'm glad I could change your perspective, Sarah! AI technologies like ChatGPT are constantly improving, and they can indeed be powerful tools in our development workflows. If you have any specific questions or need further examples, feel free to ask!
Rowena, your article couldn't have come at a better time for me. I've been struggling with optimizing performance in my Angular.js projects, and your insights on using ChatGPT give me new avenues to explore. Thank you!
You're most welcome, David! I'm glad my article came at the right time for you. If you have any specific questions or need further guidance while working on performance optimization, feel free to ask!
Rowena, your article was a breath of fresh air. As someone relatively new to Angular.js, I appreciate the step-by-step explanations you provided. It made it much easier for me to grasp the concepts you discussed. Kudos!
Thank you, Michael! I'm thrilled to hear that my step-by-step explanations helped you grasp the concepts more easily. If you have any further questions while working with Angular.js, feel free to reach out!
Thank you for your kind offer, Rowena! I will definitely reach out if I encounter any challenges while working with Angular.js. Your guidance is much appreciated!
Great job, Rowena! Your article was a comprehensive guide to optimizing Angular.js performance using ChatGPT. I particularly enjoyed the section on minimizing API calls. Keep up the good work!
Rowena, I've been following your articles for a while, and you never disappoint. Your insights on leveraging AI for Angular.js performance optimization were spot on. Looking forward to your future articles!
Thank you for your continued support, Mark! I'm thrilled that you find my insights on Angular.js performance optimization helpful. Stay tuned for more articles!
I'm eagerly waiting for your future articles, Rowena! Your expertise in Angular.js optimization is highly valuable, and your willingness to share it is much appreciated.
Rowena, thank you for sharing your expertise on optimizing Angular.js performance. Your article provided valuable information, and I appreciate the effort you put into explaining complex concepts in an approachable manner.
Rowena, your article was a fantastic resource for developers looking to optimize Angular.js performance. The way you combined the concepts of AI and web development was remarkable. Well done!
I have to say, Rowena, your writing style is engaging and easy to follow. As a developer, I often find technical articles overwhelming, but yours was a pleasure to read. Thank you for making it accessible!
Rowena, you've done a remarkable job with this article. I've been exploring how to optimize Angular.js performance, and your insights on leveraging ChatGPT are invaluable. Thank you for sharing your knowledge!
Rowena, your article provided valuable insights into optimizing Angular.js performance. I particularly liked your tips on lazy loading and code splitting. Thank you for sharing your knowledge with the community!
Great article, Rowena! I enjoyed your explanations on how ChatGPT can be used to improve performance in Angular.js. Keep up the fantastic work!
Thank you, Rowena, for your thorough article on leveraging ChatGPT for performance optimization in Angular.js. It was informative and well-structured. Looking forward to more of your content!
Rowena, your article was an excellent read! I appreciate how you provided real-world examples of using ChatGPT to achieve performance gains in Angular.js. Keep up the great work!
Rowena, your knowledge shines through in this article. I'm always impressed by the depth and clarity of your explanations. Well done!
Rowena, your article was a goldmine of practical advice. The strategies you shared on optimizing performance with ChatGPT will undoubtedly benefit many developers. Thank you for your expertise!
Great job, Rowena! Your article was both informative and accessible. I appreciate the effort you put into ensuring that complex concepts are easily understandable. Looking forward to your future articles!
Rowena, your article was an enlightening read. I've been looking for ways to optimize the performance of my Angular.js projects, and your insights on leveraging ChatGPT were exactly what I needed. Thank you!
Rowena, your article on leveraging ChatGPT for performance optimization in Angular.js was exceptional. You managed to break down complex concepts into simple, actionable steps. Kudos!
Rowena, your article was an absolute gem! As an aspiring Angular.js developer, I found your insights invaluable. Thank you for sharing your knowledge and expertise!
Rowena, your article was a game-changer for me. I've struggled with optimizing performance in Angular.js, but your explanations on using ChatGPT have opened up new possibilities. Thank you!
Thank you for the amazing article, Rowena! You've provided practical tips and real-world examples that are invaluable for anyone looking to optimize Angular.js performance.
You're welcome, Emma! I'm glad you found the tips and examples helpful. If you have any specific questions in the future or need further assistance, feel free to ask. Happy optimizing!
Rowena, your expertise shines through in this article. Your explanations were concise yet comprehensive. Thank you for sharing your knowledge with the community!
Rowena, your article on performance optimization in Angular.js using ChatGPT was excellent. It provided a comprehensive guide to improving the efficiency of our applications. Thank you!
Rowena, your article was a fantastic resource for anyone working with Angular.js. Your insights on leveraging ChatGPT for performance optimization were enlightening. Keep up the great work!
Rowena, your article was a breath of fresh air. Your explanations were clear and concise, making it easy for me to follow along. Thank you for sharing your expertise!
Rowena, your article on boosting Angular.js performance by utilizing ChatGPT was spot on. It provided valuable insights and practical strategies. Well done!
Rowena, your article was an excellent read. The way you explained the potential of ChatGPT in Angular.js performance optimization was impressive. Thank you for sharing your knowledge!
I'm glad you found it impressive, Victoria! ChatGPT indeed has the potential to revolutionize performance optimization in Angular.js. If you have any questions or need further examples, feel free to ask!
Rowena, your article was a game-changer for me. The insights you provided on leveraging ChatGPT for performance optimization in Angular.js were eye-opening. Thank you!
Rowena, your article was a masterclass in optimizing Angular.js performance. The way you explained complex concepts with real-world examples made it easy to follow along. Well done!
Thank you, Rowena, for sharing your expertise on leveraging ChatGPT for performance optimization in Angular.js. Your article provided a clear roadmap for developers looking to improve efficiency. Keep up the great work!
Rowena, your article was spot on! The use of ChatGPT for performance optimization in Angular.js is a game-changer. Thank you for sharing your expertise with the community!
Rowena, your article on optimizing Angular.js performance through ChatGPT was brilliant! Your explanations were clear, and the examples resonated well. Kudos to you!
Rowena, your article was a breath of fresh air. The way you explained the concepts and provided practical examples made it easy for me to comprehend. Thank you for sharing your knowledge!