Boosting Performance: Leveraging ChatGPT for Performance Optimization in Angular Technology
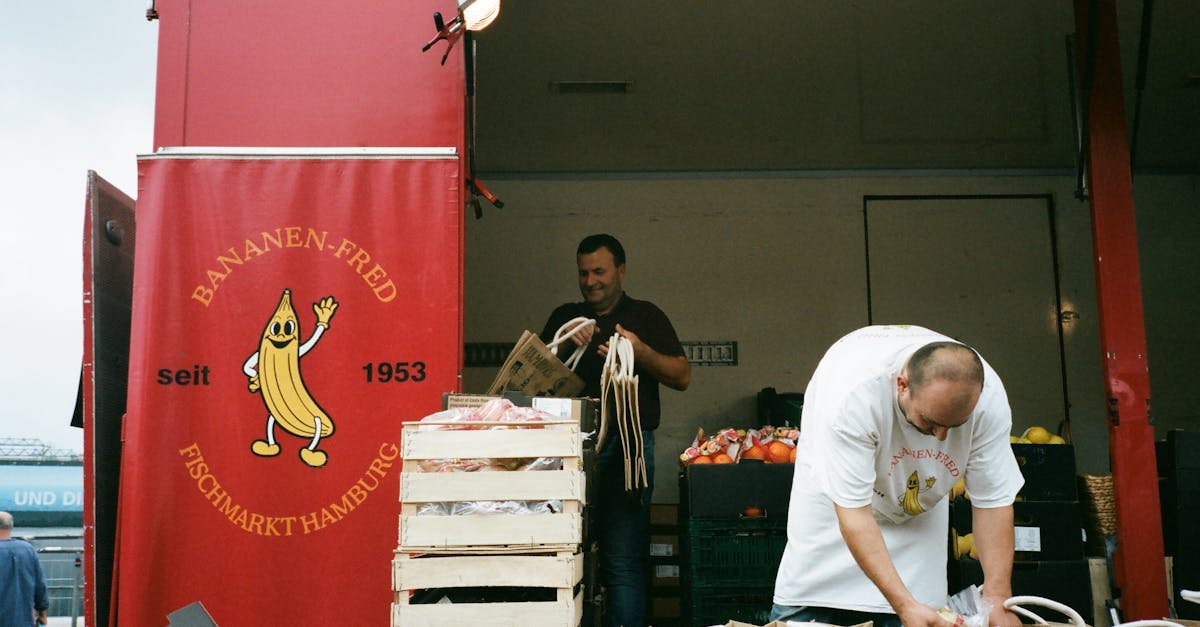
Angular, a popular JavaScript framework, is widely used for building complex web applications. However, as the application grows in size and complexity, the performance can start to degrade. This article explores various practices and approaches to optimize Angular applications, ensuring a smooth and responsive user experience.
1. Lazy Loading
Lazy loading is a technique that allows loading specific parts of the application only when needed, reducing the initial load time and improving performance. With Angular, you can implement lazy loading by splitting your application into feature modules and loading them on-demand. This approach eliminates unnecessary network requests and improves the overall performance of the application.
2. Change Detection
Angular's change detection mechanism can be a performance bottleneck, especially when dealing with large data sets or frequent updates. To optimize change detection, you can consider the following:
- Use OnPush change detection strategy for components that don't require frequent updates.
- Avoid unnecessary subscriptions and utilize observables with async pipe.
- Optimize data bindings by using one-time bindings when appropriate.
3. AOT Compilation
Ahead-of-Time (AOT) compilation reduces the size of your application and improves the initial load time. By pre-compiling Angular templates during the build process, you eliminate the need for runtime template compilation, which speeds up the rendering process. AOT compilation also enables better tree shaking and eliminates unused code, further optimizing the application's performance.
4. Code Optimization
Optimizing the codebase can significantly enhance the performance of an Angular application. Some best practices include:
- Minify and gzip the JavaScript and CSS files to reduce file sizes.
- Use lazy loading for external libraries and third-party dependencies.
- Avoid unnecessary imports and dependencies.
- Remove dead code and unused assets.
5. Caching and HTTP Interceptors
Implementing caching and HTTP interceptors can minimize network requests and reduce server load. You can utilize Angular's HTTP interceptors to cache requests, allowing subsequent requests to be served from the cache instead of hitting the server. This technique improves the application's response time and reduces unnecessary network traffic.
6. Performance Monitoring
Regular performance monitoring helps to identify performance bottlenecks and optimize the application accordingly. Utilize tools like Angular's built-in performance profiler or third-party tools to analyze and track performance metrics. This enables you to identify areas that need improvement and make data-driven optimizations for better overall performance.
Conclusion
Optimizing the performance of an Angular application is crucial for delivering a smooth user experience. By implementing practices such as lazy loading, optimizing change detection, enabling AOT compilation, optimizing code, implementing caching, and monitoring performance, you can significantly enhance the performance of your Angular application. Keep in mind that performance optimization is an ongoing process, and regular analysis and improvements are necessary to ensure optimal performance.
Comments:
Thank you all for taking the time to read my article on leveraging ChatGPT for performance optimization in Angular technology. I'm excited to discuss this topic with you!
Great article, Diego! The use of ChatGPT for performance optimization in Angular is an interesting idea. I'd love to hear more about your experiences with it.
Thanks, Lisa! I've found that leveraging ChatGPT has helped in optimizing Angular performance by making complex code optimizations more accessible. It has allowed me to identify and address potential bottlenecks quicker.
This is an intriguing approach, Diego! How would you compare leveraging ChatGPT with traditional methods of performance optimization in Angular?
Good question, Mark! Traditional methods typically involve manual code analysis and performance profiling, which can be time-consuming. ChatGPT allows for a more interactive and conversational approach, making it easier to explore optimization possibilities.
Diego, have you encountered any challenges or limitations when using ChatGPT for performance optimization?
Certainly, Emily! ChatGPT provides helpful insights and suggestions, but it still requires human judgment to apply optimizations. It's important to closely analyze and test the recommended changes before implementation.
I'm curious, Diego, how does leveraging ChatGPT impact the development workflow in an Angular project?
That's a good question, Mike! Introducing ChatGPT into the development workflow does add an extra step, but the benefits can outweigh the additional effort. It enhances the optimization process by providing alternative perspectives on improving performance.
As someone new to Angular, this article has given me valuable insights into performance optimization. Thank you, Diego!
I'm curious if ChatGPT can help optimize specific aspects of Angular, like rendering performance. Any thoughts, Diego?
Absolutely, Kevin! ChatGPT can help identify potential rendering bottlenecks by analyzing code and suggesting optimizations. It has been particularly useful in improving rendering performance in my projects.
Diego, could you provide some examples of how ChatGPT has helped you optimize Angular projects?
Sure, Sophia! ChatGPT has helped me identify unnecessarily complex code patterns, suggesting simpler alternatives that improve performance. It has also guided me in addressing memory leaks and improving overall application responsiveness.
This article presents an innovative concept, but I wonder about the potential risks of relying too heavily on ChatGPT for performance optimization. Any thoughts, Diego?
Valid concern, Robert. While ChatGPT is a helpful tool, it's important to strike a balance and not solely rely on its suggestions. Human expertise and thorough testing are crucial to ensure the suggested optimizations are appropriate and effective.
Diego, how does ChatGPT handle performance optimization suggestions for larger Angular codebases?
Good question, Grace! ChatGPT can handle larger codebases, but the optimization suggestions might require more validation and testing due to the increased complexity. It's crucial to separate and prioritize the optimizations based on their impact.
I'm curious about the implementation process of leveraging ChatGPT for performance optimization. Could you provide some insights, Diego?
Certainly, Daniel! To leverage ChatGPT, you can start by training it on a relevant dataset of Angular code examples. Then, using the trained model, you can engage in conversation-like interactions to explore optimization possibilities.
Diego, what tools or frameworks do you recommend for implementing ChatGPT-based performance optimization in an Angular project?
Great question, Olivia! There are several libraries and frameworks available for integrating ChatGPT into an Angular project. Some popular options include using TensorFlow.js for model deployment and Angular Material for the user interface.
Diego, how would you compare leveraging ChatGPT with other AI-based approaches to performance optimization in Angular?
Good question, Ethan! ChatGPT offers a more conversational and interactive approach compared to other AI-based approaches, which may involve automated code analysis or rule-based optimizations. It allows for a more iterative exploration of optimization possibilities.
Diego, what are your thoughts on the future of leveraging AI models like ChatGPT for performance optimization in Angular?
I believe leveraging AI models like ChatGPT for performance optimization in Angular will continue to evolve and become more sophisticated. It has the potential to become an integral part of the development process, helping developers create more efficient and performant applications.
This article has opened up a new perspective for me on performance optimization in Angular. Thanks, Diego!
Diego, I appreciate your insights on leveraging ChatGPT for performance optimization. It seems like a promising approach.
Great article, Diego! I would be interested in hearing more about the potential limitations of using ChatGPT for performance optimization in Angular.
Thanks, Alan! While ChatGPT is helpful, it is important to note that it's a tool to assist in the optimization process. It's not a magic solution and requires human judgment to prioritize and validate the suggested optimizations.
As an Angular developer, I find the idea of leveraging ChatGPT for performance optimization quite intriguing. Can't wait to give it a try!
Diego, have you found any specific scenarios where ChatGPT has provided unexpected insights or suggestions for performance optimization in Angular?
Definitely, Sophie! ChatGPT has sometimes suggested optimizations that I hadn't considered before, leading to notable performance improvements. Its ability to analyze code patterns comprehensively can reveal unique optimization opportunities.
Interesting read, Diego! What are your thoughts on the learning curve associated with leveraging ChatGPT for performance optimization in Angular?
Thanks, Lucas! The learning curve for leveraging ChatGPT depends on the developer's familiarity with AI models and natural language processing. However, the availability of resources and tutorials can help reduce the initial learning curve.
Diego, how would you recommend developers get started with leveraging ChatGPT for performance optimization in Angular?
To get started, Anna, developers can explore available pre-trained models or train their own using relevant Angular code examples. They can then integrate the model into their workflow and gradually incorporate ChatGPT-based optimization suggestions.
Diego, do you have any tips for developers on effectively utilizing ChatGPT for performance optimization in Angular?
Certainly, Emma! It's important to start with smaller chunks of code and validate the optimization suggestions before applying them to the entire codebase. Additionally, engaging in conversations with ChatGPT by asking specific questions can yield more targeted optimization insights.
This article has given me a new perspective on performance optimization in Angular. Thank you, Diego!
Diego, I found your article fascinating! Can you discuss any potential drawbacks of leveraging ChatGPT for performance optimization in Angular?
Certainly, Julia! One potential drawback is the need for manual validation and testing of the suggested optimizations since ChatGPT's suggestions might not always be optimal or suitable. It's crucial to exercise caution and consider the broader context.
Diego, I'm curious if ChatGPT can suggest performance optimizations specific to Angular libraries or external dependencies.
Good question, Sophie! ChatGPT can indeed provide suggestions for performance optimizations in Angular libraries or external dependencies. Its ability to analyze code patterns allows it to identify areas of potential optimization across different parts of the codebase.
As someone who's worked on performance optimization in Angular projects, I see great potential in leveraging ChatGPT. Thanks for sharing your insights, Diego!
Diego, do you think leveraging ChatGPT for performance optimization might become a standard practice among Angular developers in the future?
Absolutely, Mia! As the technology evolves and developers become more comfortable with AI models, leveraging ChatGPT for performance optimization has the potential to become a standard practice among Angular developers.
Diego, I appreciate your article on leveraging ChatGPT for performance optimization in Angular. It's an interesting approach that I'm excited to explore.
Diego, have you found that ChatGPT's suggestions for performance optimization in Angular align well with Angular best practices?
Yes, David! ChatGPT's suggestions often align well with Angular best practices. However, it's important to review the suggestions in the context of the specific project and consider any project-specific conventions.
Thank you all for the engaging discussion! I appreciate your thoughts and questions. If you have any further inquiries, feel free to ask.
Diego, thank you for sharing your insights on leveraging ChatGPT for performance optimization in Angular.
You're welcome, Lucy! I'm glad you found the insights valuable.
Great article, Diego! I'm eager to experiment with leveraging ChatGPT in my Angular projects.
Thank you, Anthony! I hope you find success in leveraging ChatGPT for performance optimization.
Diego, your article has inspired me to explore new ways of optimizing performance in Angular!
That's wonderful to hear, Sophia! It's always great to explore and innovate with new performance optimization methods.
This article has given me valuable insights into utilizing AI for performance optimization in Angular. Thank you, Diego!
Diego, how do you handle potential trade-offs between performance optimization and code readability/maintainability when leveraging ChatGPT?
Great question, Sarah! Balancing performance optimization with code readability and maintainability requires careful consideration. It's important to evaluate the impact of suggested optimizations on code readability and assess whether the benefits outweigh any potential trade-offs.
Diego, I'm excited to explore leveraging ChatGPT for performance optimization in my Angular projects. Any tips on getting started?
Certainly, Emily! I would recommend familiarizing yourself with AI models and natural language processing concepts. Then, start by understanding how to train and use ChatGPT with relevant Angular code examples. Taking small steps will help you gain confidence and expertise.
This article has broadened my perspective on performance optimization in Angular. Thanks for sharing your insights, Diego!
Diego, I found your article on leveraging ChatGPT for performance optimization in Angular fascinating. It's an approach I haven't considered before.
As an Angular developer, I appreciate your article on using ChatGPT for performance optimization. It has opened up new possibilities for me.
I'm glad to hear that, Brandon! Exploring new possibilities for performance optimization is always exciting.
Diego, have you faced any challenges when integrating ChatGPT-based optimization suggestions into existing Angular codebases?
Certainly, Grace! Integrating optimization suggestions into existing codebases can require careful consideration. It's essential to evaluate potential impacts on existing functionality and thoroughly test the changes to ensure they don't introduce regressions.
This article has given me a fresh perspective on performance optimization in Angular. Thank you, Diego!
Diego, do you have any recommendations for developers who want to train their own ChatGPT model for performance optimization in Angular?
Certainly, Hannah! For training your own ChatGPT model, gathering a relevant dataset of Angular code examples is crucial. You can collect code snippets that cover various performance scenarios and train the model using frameworks like OpenAI's GPT or fine-tune pre-existing models.
Thank you all for your valuable contributions to the discussion. I hope you found the article informative and inspiring. Keep exploring and leveraging AI for performance optimization in Angular!
Diego, thank you for sharing your experiences and insights on leveraging ChatGPT for performance optimization in Angular.
You're welcome, Dylan! I'm glad you found the insights helpful.