Boosting Performance with ChatGPT: Exploring Explicit Loading in Entity Framework for Enhanced Results
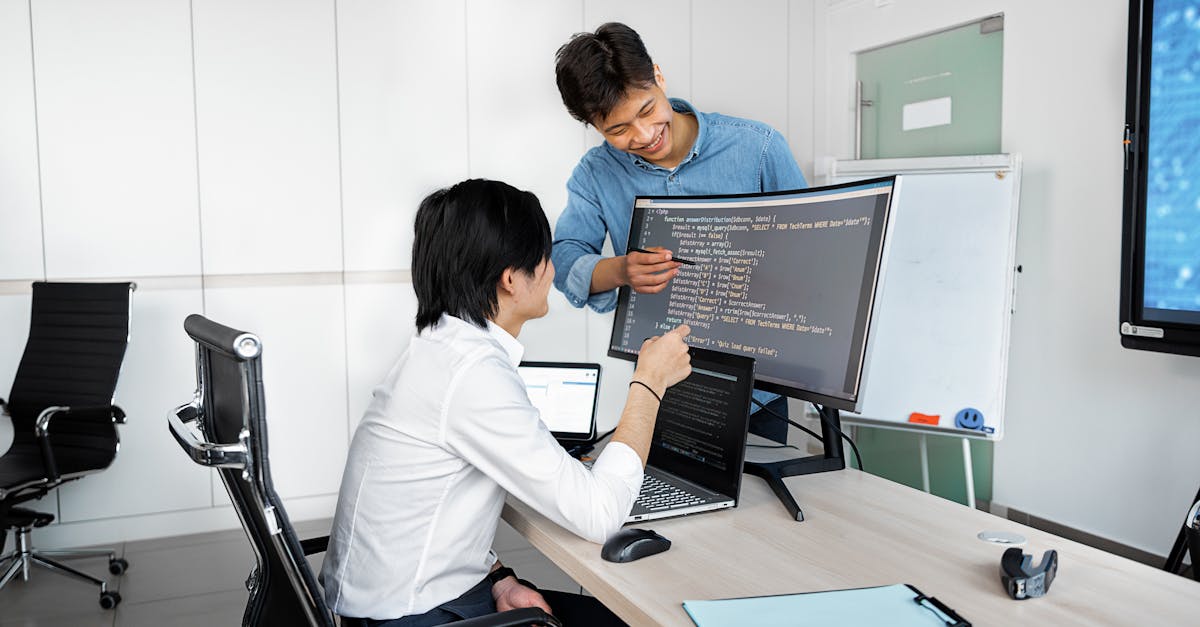
Introduction
Entity Framework is a well-known technology used for object-relational mapping in .NET applications. It provides a convenient way to work with databases by abstracting the underlying data access logic. Entity Framework has various features that make working with databases easier for developers, one of which is explicit loading.
What is Explicit Loading?
Explicit Loading is a technique in Entity Framework that allows developers to load related data into memory when needed. By default, Entity Framework uses lazy loading to load related entities automatically. However, with explicit loading, developers have more control over when and what related data is loaded.
Using Explicit Loading
Imagine you are building a chat application and using Entity Framework to store and manage your chat data. In this context, Explicit Loading can be useful when retrieving chat messages and their associated user information.
Here's an example code snippet that demonstrates how to use Explicit Loading in Entity Framework:
using (var context = new ChatDbContext())
{
// Retrieve the chat message
var chatMessage = context.ChatMessages.Find(chatMessageId);
// Explicit loading of related user information
context.Entry(chatMessage)
.Reference(c => c.User)
.Load();
}
In the code above, we first retrieve the chat message based on its ID. Then, using the Entry()
method of the DbContext
, we specify which related entity we want to load (in this case, the user information associated with the chat message). Finally, we call the Load()
method to explicitly load the related data into memory.
Benefits of Explicit Loading
Explicit Loading provides several benefits for developers:
- Reduced overhead: With explicit loading, you can avoid loading unnecessary related data, thereby improving performance.
- Control over relationships: Explicit Loading gives you more control over relationships between entities and allows you to load related data on-demand, leading to more efficient memory management.
- Flexibility in querying: Using explicit loading, you can load related entities conditionally, giving you more flexibility in querying and filtering.
Conclusion
Entity Framework's explicit loading feature is a powerful tool that can greatly enhance your ability to work with related data. Whether you are building a chat application or any other application that requires efficient loading of related entities, explicit loading provides you with more control and flexibility. By leveraging the capabilities of explicit loading, you can optimize performance, reduce overhead, and create more efficient and responsive applications.
Comments:
Thank you all for taking the time to read my article on Boosting Performance with ChatGPT. I hope you find it informative and useful!
Great article, Cantrina! I have been using Entity Framework for a while, and I'm excited to learn about explicit loading. It seems like it can really help improve performance.
Thank you, Michael! I'm glad you found the article useful. Explicit loading can indeed be a powerful technique to optimize performance in Entity Framework.
I had heard about explicit loading before, but I wasn't sure how to use it effectively. Your article explained it very well, Cantrina. Thank you!
You're welcome, Sarah! I'm happy to hear that my explanation helped clarify the concept of explicit loading. If you have any further questions, feel free to ask.
I appreciate the examples you provided, Cantrina. It really helped me understand explicit loading in Entity Framework better. Excellent article!
Thank you, Robert! I believe using examples is essential to grasp the concepts more easily. I'm glad you found them helpful.
I've been struggling with performance issues in my Entity Framework project, and this article came at the perfect time. The explicit loading technique seems promising.
I'm glad the timing worked out for you, Emily! Hopefully, incorporating explicit loading into your project will help address the performance issues you've been facing.
Cantrina, do you have any tips for identifying when to use explicit loading in Entity Framework? Sometimes it's not clear to me which scenario it's most suitable for.
That's a great question, Daniel! In general, explicit loading is beneficial when you have a scenario where you want to load related entities selectively rather than loading everything at once.
I found explicit loading especially helpful in scenarios where navigation properties might load unnecessary data by default. It allows me to control what gets loaded.
Exactly, John! Explicit loading provides more control over data loading, ensuring that only the necessary information is fetched, which can significantly improve performance.
Thanks for sharing this article, Cantrina! I've been using Entity Framework for a while now, but I haven't explored explicit loading before. I'm excited to give it a try.
You're welcome, Ryan! It's great to hear that you're willing to explore explicit loading. I'm sure it will open up new opportunities for improving performance in your projects.
I had a chance to implement explicit loading after reading your article, Cantrina. It made a noticeable difference in my Entity Framework application's speed. Thanks!
That's fantastic to hear, Olivia! I'm thrilled that the implementation of explicit loading had a tangible impact on your application's speed. Keep up the great work!
I loved how you explained the benefits of explicit loading, Cantrina. It not only improves performance but also helps reduce unnecessary data retrieval. Very insightful!
Thank you for your kind words, Maxwell! Indeed, explicit loading brings both performance benefits and the advantage of fetching only the data you need at a given moment.
Hey Cantrina, do you have any advice on when explicit loading might not be the best approach? Are there any scenarios where it's better to stick with eager or lazy loading?
Great question, Samantha! While explicit loading can be very effective in many scenarios, it may not be the best choice if you know in advance that you will always need all related data or if you're working in a context where eager or lazy loading offers better performance.
I've heard that explicit loading can cause additional round trips to the database. Can you shed some light on that, Cantrina?
Certainly, Rachel! Explicit loading can result in additional round trips to the database, as you are fetching related data on-demand. However, it's crucial to balance the number of round trips with the performance improvements gained by avoiding unnecessary data loading.
I appreciate the thorough explanation, Cantrina. Your article has given me a clear understanding of explicit loading. Looking forward to implementing it in my projects.
You're welcome, Joshua! I'm glad the explanation resonated with you. I'm confident that implementing explicit loading in your projects will have a positive impact.
Cantrina, do you have any recommendations for optimizing explicit loading further, especially when working with large data sets?
Certainly, Laura! When working with large data sets, consider using pagination or implementing caching mechanisms to further enhance performance. Additionally, make sure to profile and analyze the application's behavior to identify any bottlenecks.
Cantrina, thank you for sharing this detailed article. Explicit loading was always a bit confusing to me, but your explanations cleared things up. Much appreciated!
You're welcome, Andrew! I'm glad my explanations helped clarify any confusion you had regarding explicit loading. Feel free to reach out if you have any further questions.
I loved how you provided both code examples and explanations in the article, Cantrina. It really helped me understand the implementation better. Thank you!
Thank you for your feedback, Sophia! I find that combining code examples and explanations makes it easier for readers to grasp the concepts and apply them practically. I'm glad you found it helpful.
I had not considered explicit loading before, but your article convinced me to give it a try. I can see the potential benefits it can bring. Thanks!
You're welcome, Thomas! It's great to hear that the article convinced you to explore explicit loading. I believe you'll find it valuable in your projects.
Do you have any recommendations for ensuring maintainability when using explicit loading, Cantrina? I'm concerned it might make the code more complex.
Maintainability is an important aspect, Jessica. To improve it, consider encapsulating explicit loading logic in dedicated methods or classes and follow consistent architectural patterns. This way, the code complexity can be managed effectively.
Hi Cantrina! Your article was well-written and easy to follow. Explicit loading seems like a powerful technique, and I can't wait to try it out in my projects. Thank you!
Thank you for your kind words, Adam! I'm thrilled that you found the article easy to follow, and I hope explicit loading brings significant improvements to your projects. Let me know if you have any questions along the way.
I've been struggling with performance issues in my Entity Framework application, Cantrina. Your article gave me new insights, and I'm excited to implement explicit loading. Thank you!
I'm delighted to hear that my article provided new insights, Ethan! I'm confident that implementing explicit loading will help you address the performance issues you've been facing. Best of luck!
Explicit loading seems like an excellent solution to avoid loading excessive data, Cantrina. Your article was thorough and well-explained. Thank you!
You're welcome, Melissa! I'm glad you found the article thorough and that it shed light on the benefits of explicit loading. If you have any further questions, feel free to ask.
I've been using Entity Framework for a while, and I must say I learned something new from your article, Cantrina. Explicit loading will definitely be part of my toolbox from now on. Thank you!
I'm glad to hear that you learned something new, Kevin! Adding explicit loading to your toolbox can certainly unlock new possibilities for optimizing performance in your Entity Framework projects. You're welcome!
Your article was insightful, Cantrina. I had a basic understanding of explicit loading, but your explanations provided a clearer picture. Thank you for sharing!
Thank you for your kind words, Ava! I'm glad my explanations helped provide a clearer understanding of explicit loading. I appreciate your feedback!
I had never heard of explicit loading before, Cantrina. Your article presented it in an easy-to-understand manner. Thank you for sharing your knowledge!
You're welcome, Sophie! I'm pleased that you found the article easy to understand, even if you were unfamiliar with explicit loading. It's always a joy to share knowledge.
Great article, Cantrina! I'm an avid reader of your work, and you never disappoint. Explicit loading will definitely find its way into my development arsenal!
Thank you for your continuous support, Matthew! I'm thrilled to hear that explicit loading resonated with you and will become part of your development arsenal. I appreciate your kind words!
I've been looking for ways to enhance performance in my Entity Framework projects. Your article, Cantrina, provided valuable insights into explicit loading. Thank you!