ChatGPT: Enhancing Redux with Functional Programming Techniques
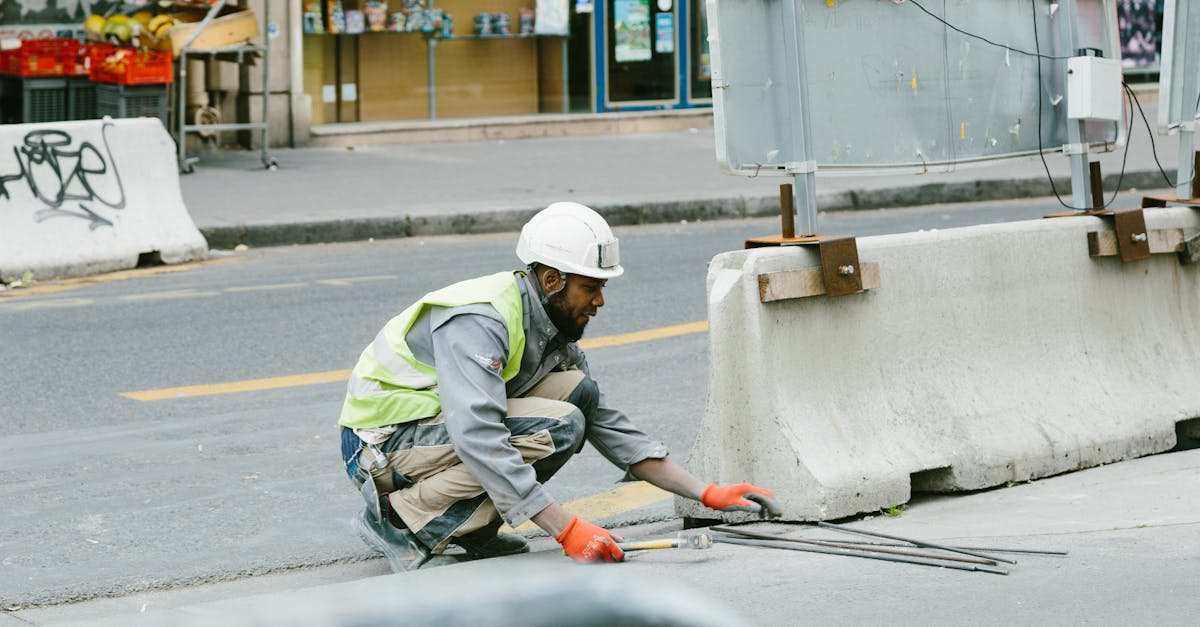
Redux is a powerful state management library widely used in modern web development projects. It follows the principles of functional programming, which emphasizes immutability, pure functions, and composability. Understanding and applying functional programming techniques can greatly enhance the effectiveness of Redux in managing complex application state.
ChatGPT-4, an AI-powered language model, can provide guidance on how to apply functional programming techniques in Redux. With its vast knowledge and understanding of programming concepts, ChatGPT-4 can help developers gain insights into Redux and functional programming best practices.
Note: It's important to have a basic understanding of Redux and functional programming concepts before diving deep into applying these techniques in Redux.
1. Immutability
Redux relies heavily on immutability to ensure predictable state changes. Instead of mutating the state directly, functional programming encourages creating new objects with updated properties. This avoids unintended side effects and makes debugging easier.
ChatGPT-4 can guide you on how to handle immutable updates in Redux applications. It can help you understand techniques like spread syntax and object.assign to create new state objects.
2. Pure Functions
Pure functions are the foundation of functional programming. In Redux, reducers are pure functions that take the current state and an action as arguments and return a new state based on the action's type and payload.
ChatGPT-4 can assist you in writing pure reducers that follow functional programming principles. It can help you understand concepts like state normalization, action patterns, and how to avoid mutating the state within reducers.
3. Composability
One of the core principles of functional programming is composability, which allows you to break down complex problems into smaller, reusable functions. This approach helps in creating maintainable and testable Redux code.
ChatGPT-4 can provide insights into composing Redux middleware and how to structure your application to maximize reusability and modularity. It can guide you in understanding concepts like higher-order functions, middleware composition, and middleware chaining.
Conclusion
Applying functional programming techniques in Redux can greatly enhance the development process and make your code more maintainable and predictable. With the help of ChatGPT-4, you can dive into the intricacies of functional programming and gain a deeper understanding of Redux best practices.
Remember to leverage the immense knowledge and guidance ChatGPT-4 offers. Keep exploring and applying functional programming techniques in Redux to unleash the full potential of this powerful state management library.
Comments:
Thank you for reading my blog article on enhancing Redux with functional programming techniques! I'm excited to hear your thoughts and engage in a discussion.
Great article, Rene! I found the examples you provided very helpful in understanding how functional programming can enhance Redux. Do you have any other recommendations for learning more about functional programming?
Thank you, Alice! I'm glad you found the examples helpful. If you're looking to dive deeper into functional programming, I recommend checking out books like 'Functional JavaScript' by Michael Fogus and 'Functional Programming in JavaScript' by Luis Atencio.
I enjoyed reading your article, Rene. One question I have is how functional programming techniques impact performance in a Redux application. Can you shed some light on that?
Hi Bob! That's a valid concern. While functional programming can introduce some overhead, it's generally negligible and can even lead to performance improvements in certain scenarios. By reducing mutation and embracing immutability, functional programming can enable better caching and memoization, resulting in faster code execution.
I'm relatively new to Redux and functional programming, but your article made it easier for me to grasp the concepts. It would be great if you could provide more real-world examples showcasing the benefits of functional programming in Redux.
Thanks for your feedback, Carol! I'm happy to hear that the article helped you understand the concepts better. I'll definitely consider writing another article with more real-world examples to showcase the benefits of functional programming in Redux.
Excellent article, Rene! I've been using Redux for a while now, and your insights on functional programming have opened up new possibilities for me. Keep up the great work!
Thank you, David! I'm glad to hear that my article has been helpful to you. If you have any specific topics in mind that you'd like me to explore in future articles, feel free to let me know!
Your article was very well-written, Rene. I appreciate that you explained the concepts clearly and provided practical tips. I have a question though - how does functional programming fit into a team development environment?
Thank you, Eva! In a team development environment, adopting functional programming in Redux can lead to more predictable and maintainable code. By avoiding side effects and making the codebase more declarative, it becomes easier for multiple team members to collaborate, review each other's code, and reason about the application's behavior.
Your article convinced me to give functional programming a try in my Redux projects. However, can you share some common pitfalls or challenges that developers might face when incorporating functional programming in Redux?
I'm glad to hear that, Frank! When incorporating functional programming in Redux, one common challenge is dealing with asynchronous actions, where immutability may not be straightforward. Additionally, the learning curve for functional programming concepts can be steep for developers with limited exposure. However, with practice and patience, these challenges can be overcome, and the benefits are worth it.
Good to hear that functional programming can potentially offer performance benefits, Rene. It's always crucial to balance performance and maintainability.
You're absolutely right, Frank. Striking the right balance between performance and maintainability is crucial, and the characteristics of functional programming can help achieve that balance in many scenarios.
Thank you for writing this article, Rene. I've been trying to incorporate more functional programming techniques in my Redux projects, and your explanations have been valuable. Can you provide any advice on how to convince team members or stakeholders to embrace functional programming?
You're welcome, Grace! When convincing team members or stakeholders to embrace functional programming in Redux, it can be helpful to showcase the potential benefits such as improved code quality, easier maintenance, and reduced bugs. Additionally, highlighting success stories and case studies from other teams or companies that have adopted functional programming can provide concrete evidence of its effectiveness.
Your article was a great introduction to functional programming in Redux, Rene. I'm excited to start applying these techniques in my projects. Do you have any recommendations on testing strategies for functional Redux code?
Thank you, Hannah! Testing functional Redux code often involves writing pure functions that are easier to test since they don't depend on external state or introduce side effects. By leveraging tools like Jest and Redux's built-in testing utilities, you can write comprehensive unit tests for your functional Redux code.
I've been using Redux for a while, but I haven't explored functional programming much. Your article has sparked my interest. How can I migrate an existing Redux codebase to embrace functional programming techniques?
I'm glad to hear that, Ivy! When migrating an existing Redux codebase to embrace functional programming, you can start by refactoring your reducers to be pure functions and embracing immutability. Additionally, you can gradually introduce functional programming concepts to your action creators and selectors. It's important to take an incremental approach and thoroughly test the changes as you go.
Your article was just what I needed, Rene. It provided a clear understanding of functional programming and how it can enhance Redux. Do you believe functional programming is suitable for all Redux projects, regardless of their size or complexity?
Thank you, Jack! Functional programming can bring benefits to Redux projects of all sizes and complexity. However, it's important to strike a balance and consider the specific needs of your project. In some cases, adopting functional programming techniques may not be necessary or may require more effort than the perceived benefits justify. It's always a good idea to evaluate the trade-offs and make an informed decision based on the project's requirements.
I really enjoyed your article, Rene. I'm curious to know how functional programming can simplify debugging in Redux applications.
Thanks, Karen! Functional programming can simplify debugging in Redux applications by making the code more predictable. Since functional programming advocates for pure functions that don't produce side effects, it becomes easier to isolate and test individual parts of the application. Unit testing can help catch bugs early on, and the absence of mutable state can reduce the complexity of debugging.
Your article was well-explained, Rene. I appreciate the clarity you brought to the topic of functional programming in Redux. Can you recommend any best practices for integrating Redux with functional programming techniques?
Thank you, Laura! When integrating Redux with functional programming techniques, it's best to emphasize immutability throughout the codebase. Avoid directly mutating state and utilize tools like Redux Toolkit, which encourages immutable updates by default. It's also important to break down complex logic into smaller pure functions and leverage composability to make the codebase more maintainable.
I've been exploring functional programming concepts lately, and your article provided a clear connection to Redux. Do you have any thoughts on integrating TypeScript with Redux and functional programming?
I'm glad you found the connection, Mark! Integrating TypeScript with Redux and functional programming can provide additional benefits by adding static type checking to your codebase. TypeScript can help catch type-related errors at compile-time, especially when working with functional code that relies heavily on function signatures. It's a powerful combination that can enhance both the safety and maintainability of your Redux projects.
Your article was a great introduction to functional programming in Redux, Rene. I'm looking forward to exploring more about this topic. Do you have any recommended online resources for further learning?
Thank you, Nancy! If you're looking for further resources on functional programming in Redux, I recommend checking out the official Redux documentation, which has a section dedicated to functional programming concepts. Additionally, the Functional Programming in JavaScript course on Pluralsight by Cory House is highly recommended for a comprehensive understanding of functional programming principles.
Your article provided a clear and concise overview of functional programming in Redux, Rene. I appreciate how you explained the benefits and trade-offs. Are there any scenarios where functional programming might not be the best approach?
Thank you, Oliver! While functional programming has its benefits, there are scenarios where it might not be the best approach. For example, if you're working on a small and simple project with minimal state management needs, adopting functional programming techniques may introduce unnecessary complexity. It's important to evaluate the project's specific requirements and choose an approach that strikes the right balance between simplicity and maintainability.
Your article was a great read, Rene. I'm curious if there are any performance benchmarks comparing functional programming with Redux to other state management solutions.
Thanks, Patricia! While there may not be specific performance benchmarks comparing functional programming with Redux to other state management solutions, the inherent characteristics of functional programming, such as immutability and pure functions, can lead to code that is more performant. However, it's worth noting that the performance of an application depends on various factors, including the specific use case, implementation details, and the efficiency of the underlying algorithms.
Your article was an eye-opener, Rene. It made me realize how functional programming can enhance the predictability of Redux code. Thank you for sharing your insights!
I'm glad my article resonated with you, Quentin! Functional programming can indeed contribute to the predictability, testability, and overall quality of Redux code. Thank you for your feedback!
Great article, Rene! I particularly enjoyed the practical examples you shared. They made understanding functional programming concepts in Redux much easier. Looking forward to more of your articles!
Thank you, Rachel! I'm delighted that the practical examples helped you understand functional programming concepts better. I'll be sure to continue sharing more articles on related topics. Stay tuned!
Your article shed light on the benefits of functional programming in Redux, Rene. It got me thinking about how I can refactor my existing codebases. Any tips on dealing with legacy code?
I'm glad you found the benefits insightful, Sam! Dealing with legacy code when adopting functional programming in Redux can be challenging. Start by identifying critical areas where functional programming can make an immediate impact and refactor those gradually. Writing comprehensive tests is crucial to ensure that refactored code behaves correctly. Embrace an incremental approach, and over time, you'll be able to reduce the reliance on legacy code and reap the benefits of functional programming.
Your article was a fantastic introduction to functional programming in Redux, Rene. I appreciate the clarity in your explanations. How does functional programming align with the goal of code reusability?
Thank you, Tom! Functional programming aligns well with the goal of code reusability. By breaking down code into smaller, pure functions that solve specific problems, you're creating highly composable and reusable building blocks. These reusable functions can be combined to solve different problems, leading to more modular and maintainable code.
Your article provided great insights into functional programming, Rene. I have one concern - wouldn't adopting functional programming in Redux lead to a steeper learning curve for new team members?
That's a valid concern, Ursula. Adopting functional programming in Redux can indeed introduce a steeper learning curve for new team members who are not familiar with functional concepts. However, with proper documentation, training resources, and mentorship, the learning curve can be managed effectively. Additionally, the long-term benefits, such as improved code quality and maintainability, can outweigh the initial learning investment.
Your article was a great starting point for me to explore functional programming in Redux, Rene. It gave me a clear understanding of the benefits it can bring. Thank you!
You're welcome, Vera! I'm thrilled to hear that my article provided a clear starting point for your exploration of functional programming in Redux. If you have any further questions or need guidance along the way, don't hesitate to ask.
I found your article very informative, Rene. It inspired me to further explore functional programming techniques in my Redux projects. Thank you for sharing your knowledge!
Thank you, Will! I'm glad my article inspired you to explore functional programming techniques further. Best of luck with implementing them in your Redux projects, and don't hesitate to reach out if you need any assistance along the way.
Your article was an excellent introduction to functional programming in Redux, Rene. It made me realize the potential benefits of adopting these techniques. Thank you!
I'm delighted to hear that, Xavier! Exploring functional programming in Redux can indeed yield many benefits. Thank you for reading and sharing your feedback!