Enhancing C++ Language: Exploring Design Patterns with ChatGPT
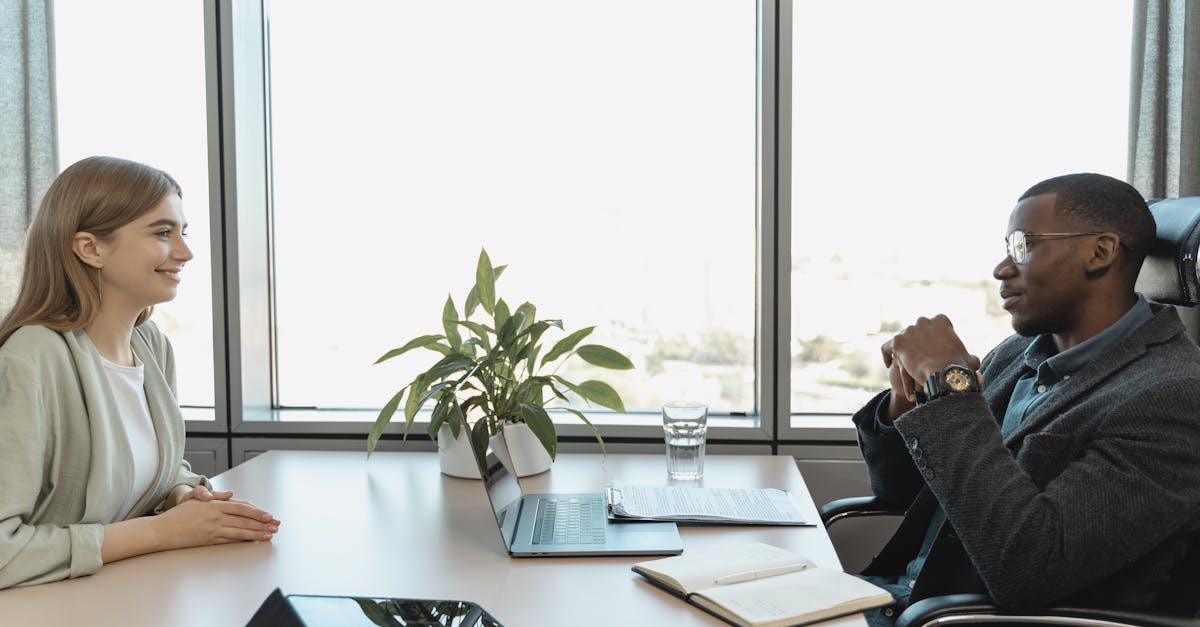
C++ is a powerful programming language that provides a wide range of features and tools to developers. One particularly useful aspect of C++ is its ability to implement various design patterns, which are proven solutions for common software design problems. In this article, we will explore the usage of C++ language in implementing design patterns.
What are Design Patterns?
Design patterns are reusable solutions to common problems that occur in software design. They represent best practices for solving these problems and have been proven to be effective. Design patterns can assist in improving the efficiency, flexibility, and maintainability of the codebase.
Why use Design Patterns in C++?
C++ is a versatile programming language that allows developers to utilize various design patterns. By using design patterns in C++, developers can create flexible and modular code that is easy to understand and maintain.
Implementing Design Patterns in C++
Here are some of the popular design patterns that can be implemented using C++ language:
1. Creational Design Patterns
Creational design patterns focus on the process of object creation. Examples of creational design patterns include:
- Singleton pattern
- Factory pattern
- Builder pattern
- Prototype pattern
2. Structural Design Patterns
Structural design patterns deal with the composition of objects and classes. Examples of structural design patterns include:
- Adapter pattern
- Decorator pattern
- Composite pattern
- Facade pattern
3. Behavioral Design Patterns
Behavioral design patterns focus on the interaction between objects. Examples of behavioral design patterns include:
- Observer pattern
- Strategy pattern
- Command pattern
- Visitor pattern
Benefits of Using Design Patterns in C++
Implementing design patterns in C++ offers several benefits:
- Code reusability: Design patterns promote reusable code, making it easier to develop and maintain large-scale applications.
- Modularity: Design patterns encourage code modularity, allowing components to be easily separated and developed independently.
- Maintainability: By utilizing design patterns, code becomes easier to understand, making it simpler to maintain and debug.
- Flexibility: Design patterns make the code more flexible by providing a structure that can be easily modified or extended.
- Scalability: With design patterns, it is easier to scale the application by adding new features or functionalities.
Conclusion
C++ language offers a powerful platform for implementing design patterns. By utilizing design patterns, developers can improve the efficiency, flexibility, and maintainability of their code. Understanding and implementing design patterns play a crucial role in becoming an effective C++ developer.
References:
- Gamma, E., Helm, R., Johnson, R., & Vlissides, J. (1994). Design patterns: elements of reusable object-oriented software. Addison-Wesley Professional.
- Sobel, J., & Lee, R. (2009). C++ in a nutshell: A desktop quick reference. " O'Reilly Media, Inc.".
Comments:
Thank you all for joining this discussion on enhancing the C++ language through design patterns with ChatGPT! I'm excited to hear your thoughts and ideas.
Great article, Amanda! I really enjoyed reading about how design patterns can be applied to C++. It's a powerful language, and using design patterns can make code more organized and maintainable.
Thank you, David! I appreciate your feedback. Design patterns indeed play a crucial role in improving code quality and reusability. Do you have any favorite design patterns in C++?
Hi everyone! I found this article fascinating. Design patterns offer elegant solutions to common programming problems. I'm particularly interested in exploring how ChatGPT can enhance the implementation of these patterns.
Hi Emily! Thanks for joining the discussion. ChatGPT does provide an interesting perspective when it comes to implementing design patterns. Which design patterns do you think could benefit the most from ChatGPT's capabilities?
This is a very well-written article, Amanda. It's impressive how design patterns can help in designing robust and efficient software systems. I'd love to hear more about how ChatGPT specifically contributes to this.
Thank you, Mark! ChatGPT can assist in various ways, such as generating boilerplate code for design pattern implementation, suggesting alternative pattern approaches, or even helping in refactoring existing patterns. It adds a new level of intelligent automation to the development process.
As a beginner in C++, this article was very informative and accessible. I've started learning about design patterns recently, and it's exciting to see how they can improve code structure. Are there any design patterns you'd recommend starting with?
Hi Sophia! I'm glad you found the article helpful. For beginners in C++, I would recommend starting with the Singleton, Factory, and Observer patterns. They are relatively simple and widely used. Once you're comfortable with those, you can explore more complex patterns like Decorator and Facade.
This article elevated my understanding of C++ design patterns. Well done, Amanda! I find the Decorator pattern particularly interesting, as it allows for dynamic modification of an object's functionality.
Thank you, Anna! The Decorator pattern is indeed quite powerful. Its ability to add or modify object behavior at runtime provides a lot of flexibility. Do you have any specific use cases where you've applied the Decorator pattern in C++?
Really enjoyed the article, Amanda! Design patterns are essential tools in any developer's toolkit. I would love to see a follow-up article discussing how ChatGPT can assist with detecting anti-patterns and suggesting alternative approaches.
Thank you, Chris! That's a great suggestion. Detecting anti-patterns and providing alternative approaches is definitely an area where ChatGPT can make a significant impact. I'll consider it for a future article.
Fantastic write-up, Amanda! One question I have is how ChatGPT can help in choosing the most suitable design pattern for a given problem. Sometimes there are multiple patterns that seem applicable.
Thank you, Lisa! Choosing the most suitable design pattern can be challenging, especially when there are multiple viable options. ChatGPT can offer guidance by analyzing the problem requirements and providing insights into potential trade-offs, helping developers make informed decisions.
This is a fascinating article, Amanda! I'm curious about the impact of using ChatGPT on the performance of the code. Does it introduce any overhead or affect runtime efficiency?
Hi Alex! ChatGPT itself does not introduce any runtime overhead since it operates separately from the compiled code. However, generating and analyzing code with ChatGPT might take additional time during development. In production, the generated code remains independent of ChatGPT.
Kudos, Amanda, for this comprehensive article! The Bridge pattern caught my attention. It's a great way to decouple abstractions from their implementations, making them more flexible to evolve independently.
Thanks, Sam! The Bridge pattern is indeed an excellent choice when there is a need to separate the abstraction and implementation hierarchies. It allows both to evolve independently, minimizing the impact of changes to one on the other.
Well done on the article, Amanda! I'm an experienced C++ developer, but it's always good to revisit design patterns and explore new perspectives like incorporating ChatGPT. It inspires fresh ideas.
Thank you, Daniel! It's great to hear that even experienced developers find value in revisiting design patterns. Incorporating AI models like ChatGPT can indeed open up new possibilities and spark innovative ideas.
Incredible article, Amanda! I appreciate the practical examples provided for each design pattern. They really helped solidify my understanding. Do you plan to write more in-depth articles on specific patterns?
Thank you, Olivia! I'm glad the practical examples were helpful. I do plan to write more in-depth articles on specific design patterns, discussing their implementation nuances and real-world use cases. Stay tuned!
Great job on the article, Amanda! As someone who works on large-scale projects, I'm always looking for ways to improve code maintainability. Design patterns play a significant role in that aspect.
Thank you, Mike! Design patterns indeed contribute to code maintainability, especially in large-scale projects. Is there a specific design pattern you find most useful for improving code maintainability?
I thoroughly enjoyed reading this article, Amanda! The Proxy pattern caught my attention. It's a powerful way to control object access and add additional functionality when needed.
Thank you, Roberta! The Proxy pattern is indeed powerful, especially when it comes to controlling and managing access to objects. It allows for additional functionality without modifying the underlying implementation.
Excellent article, Amanda! It reminded me of the power of design patterns and got me excited to implement them in my projects. I'd love to hear about any challenges you faced when incorporating ChatGPT.
Thanks, Justin! Incorporating ChatGPT did come with its own set of challenges, especially in training and fine-tuning the models to understand C++ code patterns effectively. Ensuring the generated code is error-free and optimal also required thorough testing.
Amanda, your article was superb! The Flyweight pattern stood out for me. It's fascinating how it tackles memory usage optimization by sharing common state between objects.
Thank you, Grace! The Flyweight pattern indeed provides an efficient way to optimize memory usage by sharing common state among multiple objects. It can greatly impact performance, especially in scenarios with a large number of similar objects.
Well-written article, Amanda! The Command pattern has always been one of my favorites in C++. It encapsulates both behavior and state, making it ideal for decoupling object execution from the operation requesters.
Thank you, Dylan! The Command pattern is indeed powerful for decoupling objects and encapsulating behavior and state. It provides flexibility and extensibility, allowing for the composition and execution of complex operations.
Amanda, thanks for sharing such an insightful article on design patterns in C++. It made me realize the depth of the language and the possibilities these patterns offer.
You're welcome, Julia! I'm glad the article showcased the potential of design patterns in C++. The rich features of C++ make it a great language for utilizing powerful design patterns to enhance code quality and maintainability.
Excellent article, Amanda! It's impressive to see how ChatGPT can augment the design pattern implementation process. Do you think AI models like ChatGPT will eventually become standard tools for developers?
Thanks, Gabriel! AI models like ChatGPT are already making an impact, and I believe they will become integral tools for developers in the future. The ability to assist with code generation, refactoring, and offering intelligent insights has tremendous potential.
Thanks for this informative article, Amanda! It broadened my understanding of design patterns and their implications in C++. The Proxy pattern seems very useful for implementing access control.
You're welcome, Eric! The Proxy pattern is indeed useful for implementing access control and additional functionality, acting as a surrogate for other objects. It's often used to manage object access in a controlled manner.
Great article, Amanda! Design patterns are invaluable tools for developers, and incorporating ChatGPT to enhance their implementation is fascinating. Do you plan to explore other programming languages in future posts?
Thank you, Brandon! I'm glad you found it fascinating. Yes, I do plan to explore design patterns in other programming languages in future posts. It's exciting to observe how different languages can leverage design patterns.
As a student studying C++, this article was incredibly informative, Amanda. It sparked my curiosity to learn more about design patterns and how they can improve my coding skills.
Hi Tessa! I'm glad the article sparked your curiosity. Design patterns can indeed greatly improve your coding skills by providing reusable solutions to common problems. Wishing you success in your C++ studies!
Informative article, Amanda! It's fascinating to see how ChatGPT can bring a new dimension to the implementation of design patterns. I look forward to more content like this!
Thank you, Harry! ChatGPT certainly offers exciting possibilities for improving the implementation and understanding of design patterns. I'll continue exploring such topics in future articles.
Amanda, this article was well-researched and explained. I'm curious if there are any scenarios where ChatGPT's suggestions might not align with the best practices of design patterns.
Hi Nicole! While ChatGPT provides valuable insights, it's important to consider its suggestions critically. There might be scenarios where the best practices or specific requirements of a project deviate from ChatGPT's suggestions. It's always good to evaluate generated code against established design principles.
Well-done, Amanda! This article made me reflect on the design patterns I frequently use in my C++ projects. Incorporating ChatGPT seems like a promising way to streamline and enhance the process.
Thank you, Tom! ChatGPT can indeed streamline the process by providing code suggestions, assisting in refactoring, and facilitating a deeper understanding of design patterns. It opens up new possibilities for developers.
Great article, Amanda! Design patterns are timeless tools in software development. Incorporating ChatGPT adds intelligence to the process. I'm excited to experiment with these ideas.
Thanks, Sarah! Design patterns indeed stand the test of time and remain powerful tools. Incorporating ChatGPT's capabilities can definitely fuel experimentation and innovation. Have fun exploring these ideas!