Enhancing C++ Language Interfacing with ChatGPT: Revolutionizing the Interaction Experience
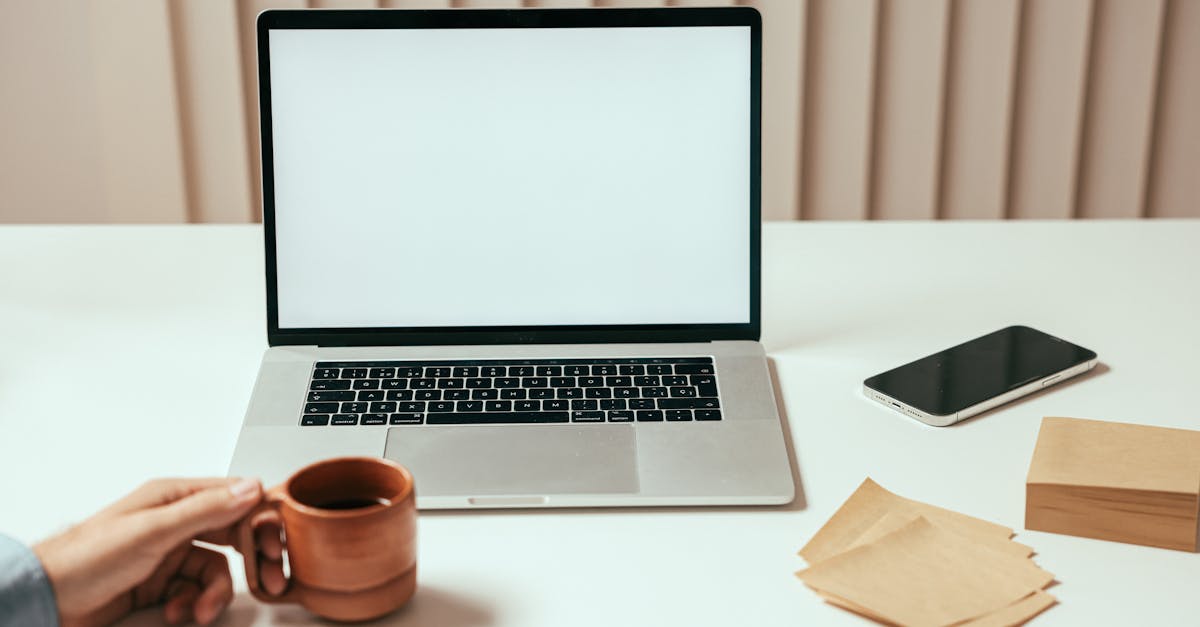
C++ is a powerful programming language widely used for developing software applications. It is known for its high performance and low-level control, making it suitable for various tasks, including interfacing. In this article, we will discuss how ChatGPT-4 can assist in writing interfaces in C++ to communicate with other software applications.
What is Interfacing in C++?
Interfacing in C++ refers to the process of creating connections and communication channels between different software applications or systems. It enables the exchange of data and functionalities between these applications, allowing them to work together seamlessly.
Using C++ for Interfacing with ChatGPT-4
ChatGPT-4, an advanced AI language model, can be utilized to simplify the development of interfaces in C++. By incorporating ChatGPT-4's natural language processing capabilities, developers can create intuitive and interactive interfaces for their applications.
Step 1: Obtaining the ChatGPT-4 API
Before integrating ChatGPT-4 into your C++ interface, you need to obtain the ChatGPT-4 API. This API provides the necessary functionalities to communicate with the AI model hosted on OpenAI servers. You can sign up for an API key and follow the documentation to set up your environment.
Step 2: Setting Up the C++ Environment
To start building the interface, you need to set up a C++ development environment. Make sure you have a compatible C++ compiler installed on your system. You can choose an Integrated Development Environment (IDE) such as Visual Studio or use a text editor and command-line tools for compilation and execution.
Step 3: Writing the C++ Interface Code
Once your development environment is ready, you can start writing the C++ code for the interface. Here's a simple example:
#include <iostream>
#include <string>
extern "C" {
// Include the ChatGPT-4 API header file
#include "chatgpt4_api.h"
}
int main() {
std::string userInput;
std::string response;
// Initialize the ChatGPT-4 API
chatgpt4_api_init();
while (true) {
std::cout << "User: ";
std::getline(std::cin, userInput);
// Call the ChatGPT-4 API to generate a response
response = chatgpt4_api_generate_response(userInput);
std::cout << "ChatGPT-4: " << response << std::endl;
}
// Clean up and release resources
chatgpt4_api_cleanup();
return 0;
}
In this code snippet, we first include the necessary libraries and headers. The main function initializes the ChatGPT-4 API and enters a loop where it waits for user input. It then calls the ChatGPT-4 API to process the user input and generates a response. The response is then displayed on the console. The loop continues until the program is terminated.
Step 4: Building and Running the Interface
After writing the C++ code, you need to compile and build the interface. Use the appropriate commands or settings specific to your development environment to compile the code. Once successfully compiled, you can run the interface and start interacting with ChatGPT-4 through the console.
Conclusion
C++ provides a powerful and efficient language for developing interfaces to connect software applications. By utilizing the capabilities of ChatGPT-4, developers can enhance the user experience by creating interactive and intelligent interfaces in C++. With ChatGPT-4's natural language processing abilities, the possibilities for creating sophisticated interfaces are vast. Whether you are building a chatbot, virtual assistant, or any other application that requires user interactions, integrating ChatGPT-4 into your C++ interface can greatly simplify the development process.
Comments:
Great article, Amanda! I'm excited to learn how ChatGPT can enhance C++ interfacing. Can you share some examples of how this revolutionizes the interaction experience?
I've been using C++ for years, and this sounds very interesting! Amanda, could you explain how ChatGPT can improve the interaction compared to traditional methods?
I'm curious about whether ChatGPT can just be used for basic interactions or if it can handle more complex tasks. Amanda, do you have any insights on this?
Thank you, Marcia, Jamie, and Jonathan! ChatGPT offers a more conversational and interactive experience for language interfacing. Instead of purely functional interactions, developers can now have natural language conversations with their C++ programs.
I'm also interested in some concrete use cases. Amanda, could you provide examples of how ChatGPT can be applied in C++ projects?
Certainly, Emma! With ChatGPT, developers can create command-line interfaces that understand and respond to natural language inputs. For example, you can build a chatbot that interacts with users using text-based conversations to navigate through a program's functionalities or provide assistance.
How does ChatGPT handle potentially ambiguous or incorrect user inputs? Amanda, I'm curious to know how it handles such scenarios.
Good question, Laura! ChatGPT is trained on vast amounts of data, which helps it generate relevant responses. However, ambiguity can sometimes arise. Developers have the flexibility to handle ambiguous inputs by implementing strategies like asking clarifying questions or providing multiple options for the user to choose from.
Can ChatGPT understand and utilize complex C++ libraries and frameworks? Amanda, I'd love to know if there are limitations in terms of the complexity it can handle.
Great question, Julian! ChatGPT's ability to handle complex C++ libraries depends on the training data and fine-tuning. While it can understand high-level details about popular libraries, it might struggle with more specific or niche ones. However, developers can train and fine-tune ChatGPT with domain-specific data to enhance its proficiency with custom libraries.
Are there any limitations when integrating ChatGPT into existing C++ codebases? Amanda, I'd like to know if there are any challenges in adopting this technology in real-world projects.
Excellent question, Nathan! Integrating ChatGPT into C++ codebases might require certain adjustments and considerations. For instance, developers need to establish secure communication channels with the model to maintain data integrity. Additionally, optimizing the performance of the interaction system is crucial to avoid any potential slowdowns.
In instances where ChatGPT cannot understand the user input, does it gracefully handle the situation? Amanda, I'm curious to know how it resolves such scenarios.
Great question, Olivia! When ChatGPT encounters unsupported or unclear inputs, developers can design fallback mechanisms to handle such situations gracefully. This can involve providing helpful error messages, suggesting alternate ways to phrase the query, or requesting more information from the user to improve understanding.
How does ChatGPT handle potential security concerns when interfacing with C++ programs? Amanda, I'm interested in knowing how this technology addresses security aspects.
Security is an important aspect, Samuel. Developers should carefully consider potential risks when integrating ChatGPT. Techniques like input validation, access control, and sanitization of user inputs are essential to mitigate security vulnerabilities. It's crucial to ensure that the system is designed to protect confidential information and prevent malicious activities.
What kind of tools or libraries would be useful for developers when working with ChatGPT in C++? Amanda, I'd appreciate any insights on what resources to explore.
Absolutely, Rebecca! Developers can explore libraries like OpenAI's GPT API to integrate ChatGPT into their C++ projects. Additionally, documentation and guides provided by OpenAI can assist in understanding the implementation process and best practices.
Are there any open-source projects or frameworks available that help developers with integrating ChatGPT into their C++ applications? Amanda, I'd like to know if there are any community-driven resources.
Good question, Daniel! While I'm not aware of any specific open-source projects or frameworks tailored for C++ integration, the vibrant developer community often creates various tools, libraries, and frameworks that can help streamline the integration process. Exploring developer forums, GitHub repositories, or reaching out to the community can be beneficial.
How much computational power is required to use ChatGPT in C++? Amanda, I'd like to understand the hardware requirements.
When it comes to hardware requirements, Sophia, it depends on the specific setup and the scale of usage. Running ChatGPT efficiently might involve utilizing GPUs or specialized hardware accelerators. However, OpenAI's GPT API enables developers to offload the heavy computation to the cloud, simplifying the hardware requirements on the local systems.
How can developers ensure that the ChatGPT interaction remains fast and responsive with C++ programs? Amanda, I'd appreciate any insights on optimizing the performance.
Optimizing performance is crucial, Lucas. Developers should consider techniques like caching commonly requested information, ensuring efficient data retrieval strategies, and parallelizing computationally intensive tasks when possible. By fine-tuning the system's architecture and leveraging optimization strategies, developers can achieve fast and responsive interactions with C++ programs.
Are there any examples or tutorials available that demonstrate the integration of ChatGPT in C++? Amanda, I'm interested in practical resources for implementation guidance.
Certainly, Grace! OpenAI offers comprehensive documentation, tutorials, and examples that cover the integration of ChatGPT in various programming languages, including C++. Exploring their resources can provide practical implementation guidance for incorporating ChatGPT into your C++ projects.
Does leveraging GPUs significantly improve the performance of ChatGPT in C++ applications? Amanda, I'm curious about the impact of hardware acceleration.
Hardware acceleration, like leveraging GPUs, can have a significant impact on ChatGPT's performance, Max. GPUs are designed to handle parallel processing tasks efficiently, which aligns well with the nature of neural network computations. Utilizing GPUs can accelerate the response generation process, making the overall system more responsive.
When it comes to optimizing performance, are there any trade-offs developers should consider? Amanda, I'd like to know if there are any potential downsides or challenges associated with optimization strategies.
Indeed, Megan. While optimization strategies can improve performance, developers need to carefully balance them with system complexity and resource usage. Over-optimization might introduce architectural complexities, making the system harder to maintain and debug. It's essential to strike a balance between performance improvements and maintaining a manageable codebase.
Are there any particular development environments or IDEs that developers recommend for working with ChatGPT in C++? Amanda, I'm interested in the preferred tools.
While the choice of development environments or IDEs is subjective, Lea, there are some popular options among C++ developers. IDEs like Visual Studio, CLion, and Eclipse offer robust C++ support and development features. It's a matter of personal preference to choose the most suitable environment for your ChatGPT integration project.
If GPUs are not accessible, are there any alternative hardware accelerators that can enhance ChatGPT's performance in C++? Amanda, I'd like to explore other possibilities.
Certainly, Emily! In addition to GPUs, other hardware accelerators like TPUs (Tensor Processing Units) can also enhance ChatGPT's performance. TPUs are specifically designed for deep learning workloads and can provide fast and efficient computations. However, availability and compatibility may vary based on the specific project requirements.
Are there any strategies to identify potential bottlenecks in the performance of ChatGPT? Amanda, I'm interested in how developers can pinpoint areas that need optimization.
Analyzing the performance of ChatGPT involves profiling and monitoring, Liam. Developers can use profiling tools to identify areas where the system spends the most time or consumes excessive resources. This helps in pinpointing potential bottlenecks and areas that require further optimization. Continuous monitoring and benchmarking also aid in tracking performance improvements over time.
Are there any specific practices or design patterns that can assist developers in effectively integrating ChatGPT into their C++ codebases? Amanda, I'd appreciate any suggestions to streamline the process.
Definitely, Zoe! One effective practice is to separate business logic from the interaction layer. This allows easier maintenance and updates to the language interfacing part without impacting the core functionality of the program. Additionally, adopting modular design patterns and using well-defined interfaces can enhance the reusability and scalability of the ChatGPT integration.
Is fine-tuning ChatGPT with domain-specific data an involved process? Amanda, I'm wondering about the effort required to train it for optimal performance in a specific C++ context.
Fine-tuning ChatGPT with domain-specific data can require some effort, Carter. It involves curating a dataset specific to the desired use case, following OpenAI's guidelines for data collection, and training the model. The level of effort depends on factors like the size of the dataset, the complexity of the domain, and the time required for experimentation and iteration to achieve optimal performance.
What are some common patterns or strategies for optimizing the performance of ChatGPT in C++ applications? Amanda, I'd appreciate any tips in this regard.
Certainly, Alex! Some common strategies for optimizing ChatGPT's performance include batch processing, where multiple queries are sent together for efficient computation, and result caching to avoid re-computation for identical or similar queries. Additionally, using efficient data structures and algorithms, along with parallelization, can further enhance performance in C++ applications.
What are the potential challenges in maintaining and updating the ChatGPT integration over time in C++ projects? Amanda, I'm interested in understanding the long-term considerations.
Maintaining the ChatGPT integration involves ensuring compatibility with newer versions of the underlying libraries, ongoing updates to language models, and addressing potential issues arising from interface changes in C++ frameworks. Long-term considerations also include retraining the model to stay up-to-date with evolving requirements and data to ensure optimal performance.
Are there any specific profiling tools or techniques that developers can use to identify performance bottlenecks in their ChatGPT integration? Amanda, I'd appreciate any recommendations for effective profiling.
Certainly, Connor! Profiling tools like Valgrind with its 'callgrind' tool or Intel's VTune can aid in identifying performance bottlenecks and locating areas for optimization. These tools provide insights into CPU time usage, memory usage, and function-level profiling, helping developers make informed decisions to improve the performance of their ChatGPT integration.
Are there any best practices for interpreting and acting on the profiling results obtained when optimizing ChatGPT in C++? Amanda, I'm interested in understanding how developers can effectively utilize the profiling data.
When interpreting profiling results, Hannah, developers should focus on identifying the most time-consuming parts of the code. By prioritizing those areas, they can target optimizations effectively. It's important to consider the broader context, assess potential trade-offs, and carefully validate any changes made based on the profiling data. Profiling results should guide developers towards making informed decisions for performance improvements.
How frequently should developers update the language models or fine-tune ChatGPT to keep up with evolving requirements? Amanda, I'd appreciate any insights on managing long-term updates.
The frequency of updating language models and fine-tuning depends on the specific project, Isabella. It's generally good practice to evaluate and update the model periodically with fresh data or when significant changes in requirements occur. Regularly checking for updates from OpenAI, exploring new research advancements, and staying engaged with the developer community can help in effectively managing long-term updates.