Enhancing Component Usage in ExtJS Technology with ChatGPT: An Innovative Approach
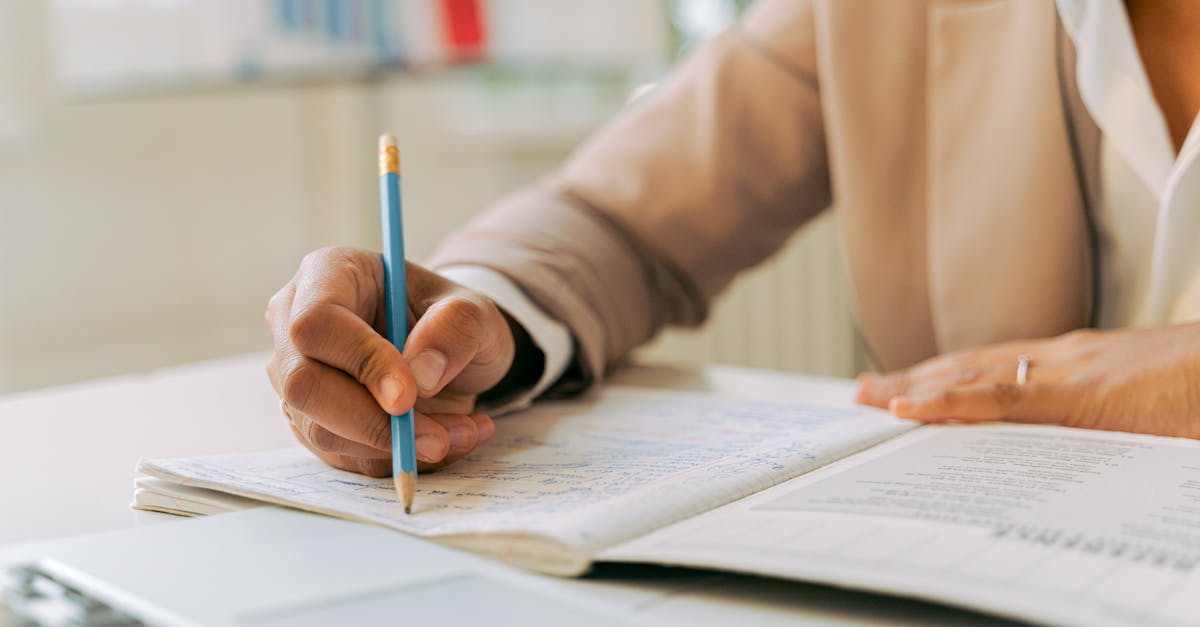
ExtJS is a powerful JavaScript framework that provides a wide range of interactive and customizable UI components. These components can be utilized to create sophisticated web applications with rich user experiences. In this article, we will explore the usage of various ExtJS components, as well as their properties, events, and methods.
1. Window Component
The Window component is used to create draggable and resizable dialog windows. It can be instantiated with various configuration options, such as title, width, height, and buttons. For example:
var win = new Ext.Window({
title: 'My Window',
width: 400,
height: 300,
items: [
{
xtype: 'panel',
html: 'Content goes here...'
}
]
});
win.show();
2. Grid Component
The Grid component is a powerful tool for displaying and editing tabular data. It supports features like sorting, filtering, grouping, and pagination. To create a simple grid, you can define a store with data and a column model with columns:
var store = new Ext.data.Store({
fields: ['name', 'email', 'phone'],
data: [
{ name: 'John Doe', email: 'john@example.com', phone: '123-456-7890' },
{ name: 'Jane Smith', email: 'jane@example.com', phone: '987-654-3210' }
]
});
var grid = new Ext.grid.GridPanel({
store: store,
columns: [
{ header: 'Name', dataIndex: 'name' },
{ header: 'Email', dataIndex: 'email' },
{ header: 'Phone', dataIndex: 'phone' }
]
});
grid.render('myGrid');
3. Form Component
The Form component provides a convenient way to create input forms with various field types such as text fields, checkboxes, radio buttons, and more. Here's an example of creating a simple form:
var form = new Ext.form.FormPanel({
items: [
{
xtype: 'textfield',
fieldLabel: 'Name',
name: 'name'
},
{
xtype: 'textfield',
fieldLabel: 'Email',
name: 'email'
},
{
xtype: 'button',
text: 'Submit',
handler: function () {
// Handle form submission
}
}
]
});
form.render('myForm');
4. Tree Component
The Tree component allows you to display hierarchical data in a tree-like structure. It is useful for representing directory structures, navigation menus, or any other data with parent-child relationships. Here's an example of creating a simple tree:
var tree = new Ext.tree.TreePanel({
root: {
text: 'Root',
expanded: true,
children: [
{
text: 'Child 1',
leaf: true
},
{
text: 'Child 2',
children: [
{
text: 'Grandchild 1',
leaf: true
}
]
}
]
}
});
tree.render('myTree');
Conclusion
ExtJS provides an extensive set of components that enable developers to build feature-rich web applications. The examples above only scratch the surface of what's possible with ExtJS. By understanding the usage of these components and their properties, events, and methods, you can leverage the full potential of the framework and create highly interactive user interfaces.
Whether you need draggable windows, data grids, input forms, or hierarchical trees, ExtJS has got you covered. Start exploring the documentation and unleash the power of ExtJS in your next web development project!
Comments:
Thank you all for reading my article on enhancing component usage in ExtJS Technology with ChatGPT! I'm excited to hear your thoughts and answer any questions you may have.
Great article, Dale! I found your insights on using ChatGPT to improve component usage really interesting. It seems like a powerful way to enhance the user experience.
Thank you, Megan! I appreciate your feedback. Yes, ChatGPT can indeed revolutionize how components are utilized in ExtJS Technology.
I've been using ExtJS for a while, and I must say this article opened up new possibilities. The combination of ChatGPT with ExtJS can definitely improve the development process.
Absolutely, Jacob! ChatGPT can help developers streamline their workflow and build more efficient and user-friendly applications with ExtJS.
I'm curious about the specific ways in which ChatGPT can be integrated into ExtJS. Are there any examples or use cases that you can share, Dale?
Great question, Olivia! One example is using ChatGPT to provide real-time code suggestions while working with ExtJS components. It can analyze the code context and offer relevant suggestions to improve coding efficiency.
I like the idea of leveraging ChatGPT in ExtJS, but what about privacy concerns? How is the data handled?
Valid concern, Nathan. When using ChatGPT, data is only used to generate responses and not stored. OpenAI takes privacy seriously and follows strict data protection practices.
I'm impressed by the potential of combining AI with ExtJS. It could make the development process much more efficient and precise.
Absolutely, Emily! AI integration can save developers time, reduce errors, and improve the overall user experience.
I would love to see some examples of how ChatGPT improves ExtJS component usage. Are there any demos available?
Definitely, Ethan! I'll be publishing a follow-up article soon that includes demos and code snippets showcasing the integration of ChatGPT with ExtJS components.
How does ChatGPT handle complex component interactions in ExtJS? Can it provide assistance for those scenarios?
Good question, Lily! ChatGPT can analyze and understand complex interactions, offering suggestions for proper component usage and guiding developers through intricate scenarios.
Do you think using ChatGPT with ExtJS will require a steep learning curve for developers?
That's a valid concern, David. While there may be a learning curve initially, once developers get familiar with ChatGPT and its capabilities, they can harness its power to accelerate their workflow effectively.
I can see how ChatGPT would be beneficial, but can it really understand the intricacies of ExtJS components like an experienced developer does?
Great point, Sophia. ChatGPT utilizes advanced language models and can provide valuable insights. However, it's important to remember that experienced developers' expertise should always be valued alongside ChatGPT's assistance to achieve the best results.
This article has definitely piqued my interest in exploring the combination of ChatGPT and ExtJS. Thanks for sharing your knowledge, Dale!
You're welcome, Emma! I'm glad you found it interesting. Feel free to reach out if you have any further questions.
The possibilities seem endless with ChatGPT and ExtJS. It has the potential to greatly improve the productivity of developers.
Absolutely, Benjamin! The synergy between ChatGPT and ExtJS can take development productivity to new heights, benefiting both developers and end-users.
What are the hardware and software requirements for implementing ChatGPT with ExtJS?
Good question, Grace! To implement ChatGPT with ExtJS, you need a machine capable of running ExtJS and a reliable internet connection for seamless integration with the OpenAI API.
Could ChatGPT also help with troubleshooting and debugging ExtJS components?
Absolutely, Isaac! ChatGPT's contextual understanding can assist developers in troubleshooting and debugging ExtJS components, helping to resolve issues more efficiently.
I'm concerned about the potential bias in AI-generated suggestions. How does ChatGPT address this?
Valid concern, Nora. OpenAI places a strong emphasis on reducing biases in ChatGPT's responses. They continuously work to make the models better and more inclusive, and they appreciate user feedback to identify and improve any biases that may arise.
Apart from ExtJS, can ChatGPT be integrated into other JavaScript frameworks as well?
Indeed, William! While this article focuses on ExtJS, ChatGPT can be integrated into various JavaScript frameworks, facilitating the development process and improving component usage across different technologies.
The possibilities seem vast. What do you think the future holds for the combination of AI and frontend frameworks like ExtJS?
A great question, Hailey! The future holds tremendous potential for AI integration in frontend frameworks like ExtJS. With advancements in AI models, we can expect more intelligent and context-aware assistance to developers, making frontend development even more efficient and user-friendly.
I can't wait to try out ChatGPT with ExtJS. It has the potential to revolutionize how we build applications.
That's exciting, Kayla! I hope you find the integration of ChatGPT with ExtJS as transformative and beneficial as many other developers have.
How does ChatGPT handle different versions of ExtJS? Will it adapt to specific framework changes and updates?
Great question, Jonathan. ChatGPT's training enables it to learn from different versions of ExtJS and adapt to specific framework changes. However, it's always recommended to stay up to date with the latest versions for the best compatibility and assistance.
This integration sounds promising! Just wondering, how can developers get started with using ChatGPT in ExtJS?
Thanks for asking, Michael! Getting started involves integrating the OpenAI API with your ExtJS development environment and making API calls based on your desired use cases. OpenAI's documentation provides a comprehensive guide to help developers successfully implement ChatGPT in their ExtJS projects.
I've thoroughly enjoyed reading your article, Dale. It's fascinating to see the potential of AI in frontend development!
Thank you, Mason! It's an exciting time for AI and frontend development, and I'm thrilled to see the positive impact ChatGPT can have on ExtJS and other frameworks.
Will using ChatGPT with ExtJS require significant modifications to existing codebases?
Good question, Rachel! Integrating ChatGPT with ExtJS typically doesn't require significant modifications to existing codebases. It's designed to seamlessly complement the development process without causing major disruptions.
I appreciate how your article highlights the benefits of combining AI with ExtJS. It's an innovative approach that can provide developers with valuable assistance.
Thank you, Andrew! AI can indeed act as a valuable assistant for developers, enhancing their productivity and allowing them to focus on building great applications.
Do you have any tips or best practices to share when integrating ChatGPT with ExtJS?
Certainly, Sophie! When integrating ChatGPT with ExtJS, ensure clear communication with the AI model by specifying your requirements and desired use cases. Additionally, it's beneficial to test and iterate on the integration to achieve the best results.
I'm amazed at how AI continues to reshape various industries. The combination of AI and ExtJS seems like a game-changer for frontend developers.
Absolutely, Christopher! AI's transformative potential extends to frontend development, and with the combination of AI and ExtJS, developers can unlock new possibilities and streamline their workflows.
Thank you all for your valuable comments and engaging in this discussion. I appreciate your interest in the application of ChatGPT in ExtJS. If you have any further questions, feel free to ask!