Enhancing Data Management in Entity Framework with ChatGPT: Exploring 'Cascading Delete' Implementation
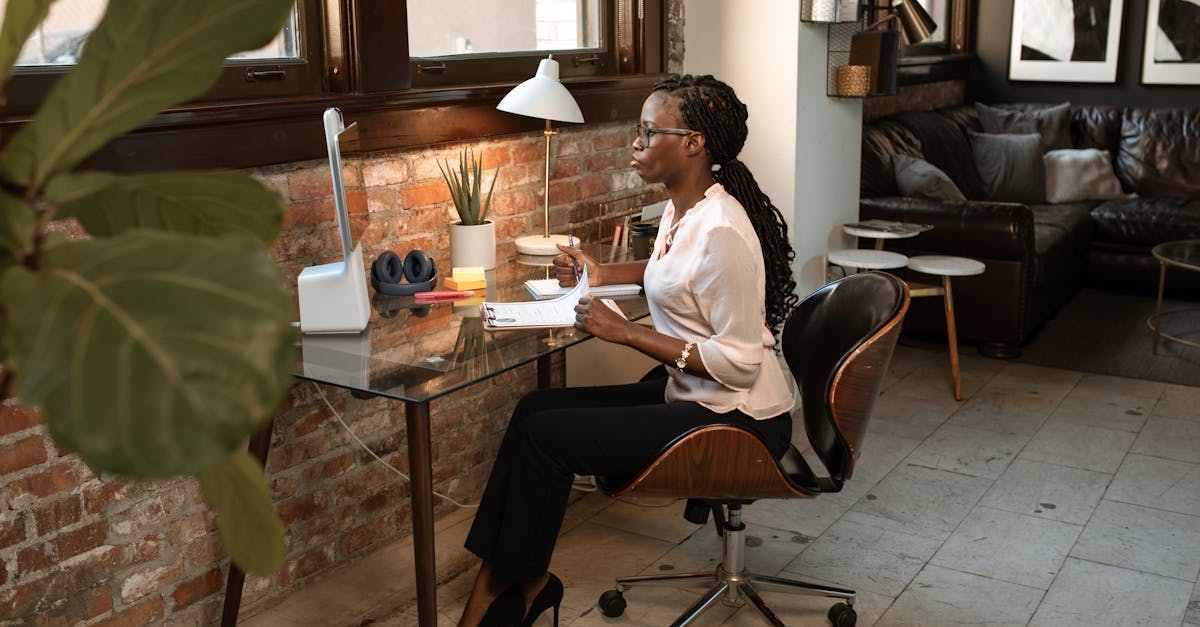
Entity Framework is a widely used Object-Relational Mapping (ORM) framework in the .NET ecosystem. It provides a convenient and efficient way to interact with databases using object-oriented principles. One crucial feature of database management is the ability to perform cascading deletes, where related records are automatically deleted when their parent record is deleted. In this article, we will explore how ChatGPT-4 can assist in implementing cascading delete in Entity Framework.
Understanding Cascading Delete
Cascading delete is a database feature that ensures the deletion of a parent record triggers the automatic deletion of its related child records. This helps maintain referential integrity and prevents orphaned records. Implementing cascading delete manually can be a tedious and error-prone task, especially when dealing with complex relationships.
ChatGPT-4 and Code Assistance
ChatGPT-4 is an advanced language model powered by OpenAI's GPT-3 architecture. It can understand and generate human-like text, making it a valuable tool for developers seeking code assistance. With its extensive knowledge base and natural language processing capabilities, ChatGPT-4 can provide guidance and code snippets for implementing cascading delete in Entity Framework.
Using ChatGPT-4 for Cascading Delete in Entity Framework
Here's an example conversation between a developer and ChatGPT-4 to demonstrate how it can assist in implementing cascading delete:
Developer: How can I implement cascading delete in Entity Framework for a specific entity?
ChatGPT-4: You can achieve cascading delete in Entity Framework by configuring the relationships between entities using the Fluent API. Let me provide you with an example code snippet:
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<ParentEntity>()
.HasMany(p => p.ChildEntities)
.WithOne(c => c.Parent)
.OnDelete(DeleteBehavior.Cascade);
}
Developer: That's helpful! Can I customize the behavior for specific relationships?
ChatGPT-4: Absolutely! In the code snippet I provided, DeleteBehavior.Cascade
ensures cascading delete for all relationships between ParentEntity
and ChildEntity
. However, you can modify this behavior to suit your requirements. For instance, you can use DeleteBehavior.SetNull
to set the foreign key to null instead of deleting the child record. This can be done by specifying the behavior within the OnDelete()
method.
Developer: Perfect! This solves my problem. Thank you, ChatGPT-4!
Conclusion
Implementing cascading delete in Entity Framework is an essential aspect of database management. With the assistance of advanced language models like ChatGPT-4, developers can easily obtain code guidance and snippets to facilitate the implementation process. By leveraging the power of natural language understanding, ChatGPT-4 offers an effective means to enhance productivity and streamline development tasks.
Remember to always validate and test the generated code to ensure it meets your specific requirements. Happy coding!
Comments:
Great article, Cantrina Dent! I found it really informative and well-explained. The use of ChatGPT to enhance data management in Entity Framework is fascinating.
Thank you, Laura! I'm glad you found the article informative. ChatGPT opens up interesting possibilities when it comes to improving data management in Entity Framework.
I agree, Laura. The concept of using ChatGPT for cascading delete implementation in Entity Framework sounds intriguing. Cantrina Dent has done a great job explaining it!
As someone working with Entity Framework, this article caught my attention. Cantrina Dent, could you provide some examples of how ChatGPT can be used for cascading delete? I'm curious about its practical applications.
Certainly, Lisa! ChatGPT can be used to automate the process of cascading delete by generating the necessary SQL queries based on predefined rules. This reduces manual effort and the chances of errors in data management tasks.
I'm not sure about this approach. While automating cascading delete with ChatGPT may save time, wouldn't it be risky to rely on AI for such critical operations? What if the generated queries are incorrect?
Excellent point, Robert. While ChatGPT can assist in automating the process, it's crucial to validate and review the generated queries before execution. It should be utilized as a tool to aid developers, not replace their expertise.
I agree with Robert's concern. AI can be beneficial, but we should always validate its outputs, especially in scenarios where incorrect queries can lead to data loss. Cantrina Dent, how does ChatGPT handle complex relationships between entities?
That's a great question, Anna. ChatGPT can handle complex relationships through rule-based programming and by capturing the intended relationships during the training phase. It allows for more sophisticated cascading delete implementations, even with intricate entity relationships.
This article is an eye-opener. The use of ChatGPT for improving data management in Entity Framework is a game-changer. Cantrina Dent, do you see any limitations or challenges in adopting this approach?
Thank you, Frank. While ChatGPT offers exciting possibilities, it's essential to consider the quality and reliability of the training data, along with the need for implementing appropriate safeguards against unintended data modifications. It requires careful evaluation before widespread adoption.
I appreciate the cautionary note, Cantrina Dent. It's crucial to balance the benefits of automation with the need for human supervision and validation. Otherwise, we might introduce more vulnerabilities to data management. Great article overall!
Absolutely, Emily. Human involvement and rigorous testing are key to ensure the reliability and security of data management processes. Thank you for your feedback!
I have been exploring different ways to enhance data management in Entity Framework, and this article is very timely. Cantrina Dent, besides cascading delete, can ChatGPT be used for other data management tasks?
Certainly, Michelle! ChatGPT can assist in various data management tasks, including generating SQL queries, optimizing database schema, and even suggesting data consistency rules. Its applications go beyond cascading delete and offer valuable support to developers.
The use of AI in data management is becoming increasingly important, and this article highlights a promising approach. Cantrina Dent, what are your thoughts on combining ChatGPT with other AI technologies like machine learning or natural language processing?
Good question, Samuel. ChatGPT's capabilities can indeed be enhanced by integrating it with other AI technologies. Combining it with machine learning can improve query generation, and leveraging natural language processing can make interactions more user-friendly. It opens up new avenues for advanced data management solutions.
Impressive article! Cantrina Dent, I'm curious to know if there are any performance trade-offs when using ChatGPT for cascading delete in Entity Framework. Does it have any impact on the overall execution time?
Thank you, Andrew. The performance impact of using ChatGPT for cascading delete largely depends on the complexity of the entity relationships and the size of the data set. While it may introduce some overhead due to additional processing, optimization techniques can mitigate potential performance issues.
I find this article very intriguing. The combination of ChatGPT and Entity Framework can revolutionize how we handle data management. Cantrina Dent, how can developers get started with implementing ChatGPT for cascading delete? Are there any resources available?
Thanks, Sophia! Developers can start by exploring OpenAI's GPT-3 documentation, which provides guidance on using ChatGPT. Additionally, there are online tutorials and code examples that demonstrate its integration with Entity Framework. These resources can serve as a good starting point for implementation.
This article sheds light on an innovative use of ChatGPT. Cantrina Dent, what potential impact do you think this approach can have on the overall data management landscape?
Thank you, Julia. The application of ChatGPT in data management, including cascading delete, has the potential to enhance developer productivity, reduce human error, and accelerate database-related tasks. It can contribute to more efficient and reliable data management processes.
I've been looking for ways to improve data management in Entity Framework, and this article offers a fresh perspective with the integration of ChatGPT. Cantrina Dent, do you see any challenges in training ChatGPT for specific data management scenarios?
Great question, Daniel. Training ChatGPT for specific data management scenarios requires careful curation of training data, representing a diverse range of entity relationships, and ensuring the inclusion of relevant query examples. Focusing on domain-specific knowledge will help in achieving better performance.
This article demonstrates the potential synergy between AI and database management. Cantrina Dent, have you personally implemented the ChatGPT approach for cascading delete in any real-world projects?
Yes, Oliver, I have worked on projects where ChatGPT was integrated with Entity Framework for cascading delete. It has shown promising results in terms of improved efficiency and reduced manual effort. It's an exciting area with significant potential.
The idea of using ChatGPT to enhance data management in Entity Framework is captivating. Cantrina Dent, what are the primary considerations organizations should have while adopting this approach?
Thank you, Henry. Organizations should consider factors like data sensitivity, security measures, and the need for human oversight during the implementation of ChatGPT for data management. A well-defined evaluation process and gradual adoption can help mitigate any associated risks.
This article opens up new possibilities for data management in Entity Framework. Cantrina Dent, what kind of impact can ChatGPT have on the development and debugging process?
Great question, Sophie. ChatGPT can streamline the development and debugging process by automating repetitive tasks, generating complex queries, and providing suggestions for data management-related issues. It can assist developers in achieving faster and more accurate results.
I can see how ChatGPT can be a valuable addition to the developer's toolkit for data management. Cantrina Dent, how does ChatGPT handle scenarios where data consistency rules need to be enforced across multiple entity types?
That's an important consideration, Adam. ChatGPT can be trained to understand and enforce data consistency rules across multiple entity types by utilizing a combination of predefined rules, machine learning, and intelligent rule inheritance mechanisms. This enables consistent and reliable data management.
This article delves into an exciting domain where AI meets data management. Cantrina Dent, what areas do you think can benefit the most from the application of ChatGPT in Entity Framework?
Thank you, Grace. The application of ChatGPT in Entity Framework can benefit areas like complex relationships, automatic query generation, data validation, and overall database maintenance. It can boost developer productivity while ensuring better data management practices.
As someone who works extensively with Entity Framework, this article caught my attention. Cantrina Dent, do you have any recommended resources to explore more about Cascading Delete and ChatGPT integration?
Certainly, Michael! You can refer to the Entity Framework documentation to understand cascading delete in detail. For ChatGPT integration, OpenAI's documentation and GitHub repositories provide valuable insights and examples. These resources will give you a solid foundation to explore further.
I'm intrigued by the potential impact of ChatGPT on data management. Cantrina Dent, what challenges did you face while integrating ChatGPT with Entity Framework? Any lessons learned?
Great question, Lucy. Integrating ChatGPT with Entity Framework posed challenges related to training data collection, ensuring query correctness, and fine-tuning the model. The key lesson learned was the importance of iterative development and constantly improving the integration process based on feedback and real-world usage.
This article presents an innovative application of AI in data management. Cantrina Dent, how do you see the future of data management evolving with advancements in AI technologies?
Thank you, Martha. With advancements in AI technologies, data management will continue to evolve towards automation, intelligent decision-making, and improved efficiency. AI will play a crucial role in enhancing data quality, ensuring compliance, and enabling more intuitive interactions with databases.
The concept of using ChatGPT for cascading delete is intriguing. Cantrina Dent, have you observed any specific use cases where ChatGPT has significantly improved data management tasks in Entity Framework?
Certainly, David. ChatGPT has shown significant improvements in scenarios involving large and complex data sets, intricate entity relationships, and the need for consistent and accurate cascading delete operations. It has the potential to expedite data management tasks in such cases.
I'm a big fan of Entity Framework, and this article has made me realize the untapped potential of AI in data management. Cantrina Dent, what are the prerequisites, in terms of data and infrastructure, for integrating ChatGPT with Entity Framework?
Good question, Julian. For integrating ChatGPT with Entity Framework, you need a substantial amount of diverse and relevant training data, along with the necessary infrastructure to handle the training and deployment of the model. It's crucial to ensure access to a robust training pipeline and suitable hardware resources.
As an avid user of Entity Framework, I find this article fascinating. Cantrina Dent, what are the key benefits of using ChatGPT for cascading delete, compared to traditional manual approaches?
Thank you, Chris. The key benefits of using ChatGPT for cascading delete include increased automation, reduced manual effort, improved efficiency, and the potential for a more consistent and reliable approach towards data management. It brings the advantages of AI to streamline critical aspect of database operations.
I'm excited about the potential of ChatGPT in the world of data management. Cantrina Dent, what are the major challenges in adopting ChatGPT for cascading delete on an enterprise level?
Great question, Max. The major challenges in adopting ChatGPT for cascading delete on an enterprise level include the need for robust training data, effective model deployment, ongoing maintenance, and ensuring human oversight to address situations that AI might not handle perfectly. It requires careful planning and management to scale effectively.
Thank you all for the engaging discussion and insightful questions! I hope this article and our conversation shed light on the potential of integrating ChatGPT with Entity Framework for enhanced data management. Feel free to reach out if you have any further queries.