Enhancing Development with ChatGPT: Revolutionizing the C/C++ STL
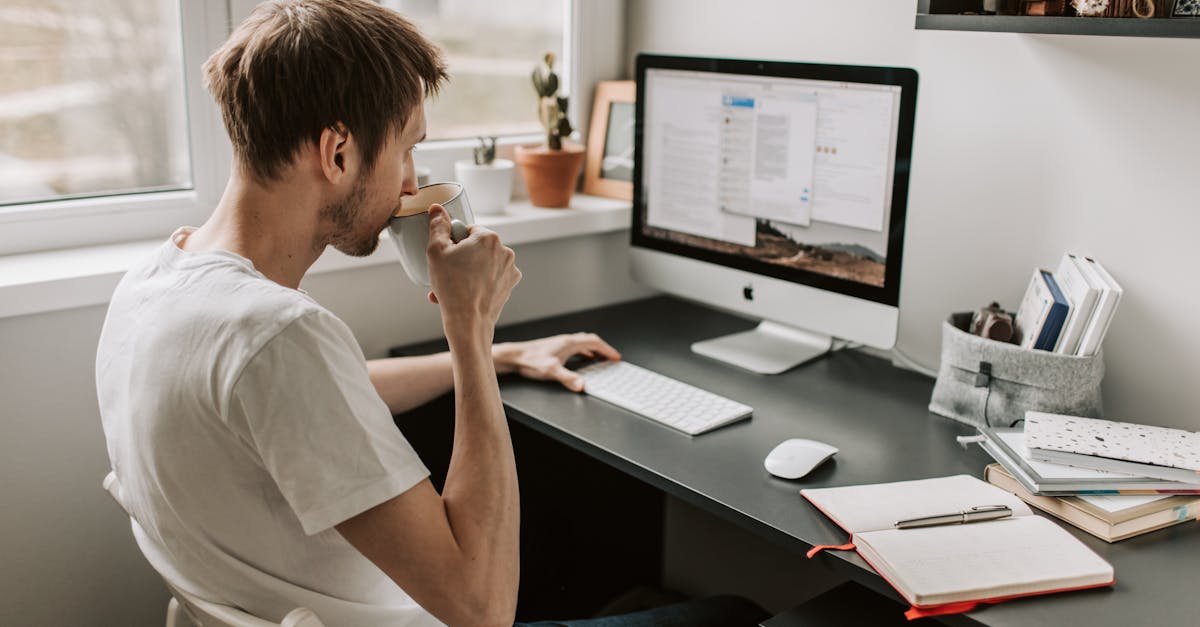
The C++ Standard Template Library (STL) provides a comprehensive set of algorithm functions that can greatly simplify the development process when working with C/C++ programs. These functions are part of the standard library and can be utilized to perform various operations efficiently and effectively.
Introduction to Algorithm Functions
The algorithm functions in C/C++ STL are designed to handle a wide range of tasks, including searching, sorting, manipulating, and modifying sequences of elements in containers. These functions are template-based, meaning they can work with different types of containers and elements.
Sort()
One of the most commonly used algorithm functions in C/C++ STL is sort(). This function can be used to sort elements in a container in ascending order. For example, if you have a vector of integers, you can simply call sort() to order the elements from the smallest to the largest.
#include
#include
#include
int main() {
std::vector numbers = {5, 3, 1, 4, 2};
std::sort(numbers.begin(), numbers.end());
for (const auto& number : numbers) {
std::cout << number << " ";
}
return 0;
}
This code snippet demonstrates the usage of sort() function in C++ STL. The output of the program will be: 1 2 3 4 5, indicating that the vector elements have been sorted in ascending order.
Reverse()
The reverse() function, as the name suggests, can be used to reverse the order of elements in a container. This function takes two iterators as arguments to define the range of elements to be reversed. By calling reverse() on a container, the order of its elements will be reversed.
#include
#include
#include
int main() {
std::list numbers = {1, 2, 3, 4, 5};
std::reverse(numbers.begin(), numbers.end());
for (const auto& number : numbers) {
std::cout << number << " ";
}
return 0;
}
In this example, the elements of a list will be reversed using reverse(). The output of the program will be: 5 4 3 2 1, indicating that the list elements have been reversed.
Next_permutation()
The next_permutation() function is a powerful tool for generating permutations of a range of elements. It rearranges the elements of the range to generate the next lexicographically greater permutation. This function can be used to explore and iterate through all possible permutations of a set of elements.
#include
#include
#include
int main() {
std::vector numbers = {1, 2, 3};
do {
for (const auto& number : numbers) {
std::cout << number << " ";
}
std::cout << std::endl;
} while (std::next_permutation(numbers.begin(), numbers.end()));
return 0;
}
In this example, next_permutation() is used to generate and print all the possible permutations of the numbers 1, 2, and 3. The output will be:
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
Conclusion
C/C++ STL algorithm functions, such as sort(), reverse(), and next_permutation(), provide powerful tools for performing various operations on sequences of elements. These functions enhance the productivity of C/C++ developers by simplifying complex tasks and providing efficient solutions. By utilizing these algorithm functions in combination with the ChatGPT-4 model, developers can explain and provide examples of usage for various algorithm functions in C++ STL to assist learners and programmers in understanding and implementing efficient algorithms.
Comments:
Thank you all for taking the time to read my article on enhancing development with ChatGPT! I'm excited to hear your thoughts and engage in a discussion.
Interesting article, Rey! I can definitely see the potential of ChatGPT in revolutionizing C/C++ development. Do you think it could also have applications in other programming languages?
Thanks, David! ChatGPT indeed has potential beyond C/C++. It can be adapted to work with other programming languages, as the underlying techniques are language-agnostic. It's quite versatile!
Thanks for the insight, Rey! The flexibility of ChatGPT to adapt to different programming languages is definitely a major advantage. It's incredible to think about the impact it could have on the coding process as a whole.
You're welcome, David! Indeed, the adaptability of ChatGPT across languages is a game-changer. Envisioning the potential impact on coding productivity is quite promising. Exciting times ahead!
Absolutely, Rey! It's fascinating to witness the rapid advancements in AI that can reshape the way we develop software. Looking forward to exploring the possibilities further!
Thank you, Rey! Your expertise and passion for AI-assisted development are truly inspiring. This discussion has been enlightening. Best of luck with your future endeavors!
You're very welcome, David! I'm grateful for your kind words and delighted that you found the discussion enlightening. Thank you and best of luck to you too in your coding adventures!
Thank you again, Rey! Your guidance and presence in this discussion have been highly valued. Best of luck moving forward!
You're most welcome, David! I'm pleased that you found my guidance valuable. Thank you for the kind words, and best of luck to you too in your coding and AI-assisted development endeavors!
Hi Rey, great article! I was wondering how ChatGPT handles complex data structures in the C/C++ STL. Can it provide solutions for optimizing the performance of containers like std::map or std::unordered_map?
Hi Kelly! Yes, ChatGPT can definitely provide assistance with optimizing the performance of C/C++ STL containers. It can suggest alternative approaches, highlight potential bottlenecks, and even help with identifying memory leaks.
That's impressive, Rey! Having assistance in identifying performance bottlenecks and memory leaks can be a great help. It seems like ChatGPT can be a valuable tool for C/C++ developers.
Definitely, Rey! Having real-time assistance in performance optimizations and bug detection can greatly enhance productivity and code quality. Great work on exploring this topic!
Thank you, Kelly! I'm glad you found the article helpful. Real-time assistance can indeed boost productivity and code quality. It's exciting to be part of this revolutionary technological advancement!
Thank you, Rey! Your article has shed light on the possibilities of using AI assistance for C/C++ development, and I certainly feel confident exploring these tools further.
You're welcome, Kelly! I'm glad you found the article informative and feel inspired to explore the AI-assisted development tools. Wishing you success in your coding endeavors!
Thank you, Rey! Your expertise and engagement have been invaluable. Best of luck with your ongoing work in AI-assisted development!
You're very welcome, Kelly! I'm grateful for your kind words and support. Best of luck to you too in your coding journey and future endeavors!
Thank you again, Rey! Your expertise and engagement have been greatly appreciated. Best of luck with your ongoing AI-assisted development projects!
However, it's worth mentioning that different programming languages have their own idioms and specific libraries, so certain adaptations and customizations would be necessary when using ChatGPT in different contexts.
I'm a bit skeptical about using AI for development tasks. How reliable is ChatGPT in providing accurate and safe suggestions for C/C++ code? Any potential risks or caveats to consider?
Valid concern, Mike! While ChatGPT has shown promising results, it's important to exercise caution. Since it's trained on large datasets from the internet, it may not always generate safe or bug-free code. It should be used as a helpful tool, not as a substitute for careful programming and code reviews.
Thanks for the clarification, Rey! Indeed, it's important to use AI tools responsibly and never rely solely on their suggestions without thoroughly reviewing the code. Still, it's exciting to see the potential advancements in development assistance.
Definitely, Rey! Responsible AI adoption is crucial to ensure safe and reliable development practices. Your article sheds light on the potential of ChatGPT while emphasizing the importance of human expertise. Well done!
Thank you, Rey! Your article strikes a good balance by highlighting the potential of ChatGPT while promoting the importance of careful coding practices. The future of AI-assisted development looks bright!
You're welcome, Mike! Maintaining a balance between AI assistance and human expertise is crucial for safe and reliable development. The future is indeed promising, and responsible AI adoption will propel it even further!
Rey, are there any limitations to using ChatGPT for C/C++ development? Are there specific scenarios where it may struggle or provide inaccurate suggestions?
Great question, Emily! ChatGPT has limitations, especially when dealing with very specific domain knowledge or handling large codebases. It may also struggle with complex algorithmic optimizations. In such cases, domain-specific tools and expert knowledge would still be essential.
I see, Rey! Having that awareness of ChatGPT's limitations is crucial for developers. It's impressive to see how AI can augment the development process without replacing the need for domain expertise.
Hi Rey, great article! How does ChatGPT handle C/C++ language libraries and their various versions? Does it provide accurate suggestions in different library contexts?
Thank you, Alex! ChatGPT can handle different C/C++ libraries and versions, but its knowledge is based on the data it was trained on, so there might be limitations. It's recommended to double-check library specifics, updates, and consult the official documentation for accurate suggestions in specific library contexts.
Got it, Rey! Relying on the official documentation alongside ChatGPT's suggestions seems like a great way to use the tool effectively. Thanks for the clarification!
Indeed, Rey! Relying on ChatGPT's suggestions alongside official documentation seems like the optimal approach. I'm thrilled to experiment with the possibilities!
Absolutely, Alex! Combining ChatGPT's suggestions with official documentation will help you harness its potential effectively. Enjoy experimenting and discovering the capabilities!
Thank you, Rey! Your enthusiasm and guidance are genuinely appreciated. Looking forward to this exciting journey of AI-assisted development!
Rey, can ChatGPT also provide assistance with debugging C/C++ code? It would be great to have an AI-powered 'pair programming' tool that can help in identifying and fixing bugs.
Hi Daniel! While ChatGPT can offer suggestions for debugging C/C++ code and point out potential issues, it's not specifically designed as a debugging tool. For precise debugging, dedicated debuggers and tools would still be the best choice.
Thanks for the response, Rey! Pairing ChatGPT with dedicated debuggers makes sense. It's exciting to see how AI can augment the development workflow in numerous ways.
I understand, Rey. Having a dedicated debugging tool is crucial for effective bug fixing. I'm still amazed by the potential applications of ChatGPT in various areas of development!
Absolutely, Daniel! ChatGPT opens up exciting possibilities, and its integration with dedicated tools can create a powerful combination. AI can truly be a valuable assistant in the hands of developers.
Indeed, Rey! AI-assisted development has tremendous potential, and I can't wait to see how it evolves in the coming years. Thank you for sharing your expertise!
You're welcome, Daniel! It's an exciting time for AI-assisted development, and I'm glad you found the discussion valuable. Don't hesitate to reach out if you have any more questions!
Thank you, Rey! I truly appreciate your engagement and expertise. I'll definitely reach out if I have any more questions. Keep up the amazing work!
Thank you, Rey! I appreciate your expertise and engagement in this conversation. It has been insightful, and I'm excited to explore the possibilities further!
You're most welcome, Daniel! I'm glad I could contribute to the conversation and provide insights. Feel free to explore the possibilities and reach out anytime. Happy coding!
Thank you, Rey! Your expertise and insights have been incredibly valuable. I'm sincerely grateful. Cheers to an exciting future of AI-assisted development!
Thank you, Rey! Your expertise and insights have been incredibly valuable. I appreciate your contribution to the conversation. Cheers!
Thank you, Rey! Your engagement and knowledge have been invaluable. Keep up the fantastic work!
Thank you, Rey! Your expertise and involvement have been truly appreciated. Wishing you continued success and exciting discoveries in AI-assisted development!
Thank you, Rey! Your involvement and insights have been exceptional. Best of luck in your ongoing endeavors!
Thank you, Daniel! I'm grateful for your kind words and support. Best of luck to you too in your coding and AI-assisted development pursuits. Keep pushing the boundaries!
Rey, I really enjoyed reading your article! Just curious, how would you recommend getting started with using ChatGPT for C/C++ development? Any specific resources or tools we should consider?
Thank you, Sophie! To get started with ChatGPT for C/C++ development, you can explore OpenAI's blog post on 'ChatGPT as a Development Environment,' which provides guidance and showcases example code. You can also experiment with OpenAI's API and client libraries for interfacing with ChatGPT effectively.
Thank you, Rey! I'll definitely check out the OpenAI resources and engage with the developer community. Excited to dive into the world of AI-assisted C/C++ development!
Thank you, Rey! Your expertise and guidance are highly appreciated. Looking forward to exploring AI-assisted development further. Best wishes on your endeavors!
You're most welcome, Sophie! Delving further into AI-assisted development will unlock numerous possibilities. Thank you for your well wishes. Feel free to reach out if you have any more questions!
Thank you, Rey! Your support and willingness to answer our questions are commendable. I'll definitely reach out if I need any further insights. Best of luck!
You're most welcome, Sophie! I'm here to help whenever you need further insights. Best of luck to you too in your AI-assisted development journey. Exciting times lie ahead!
Thank you so much, Rey! I'm grateful for your guidance and expertise. Wishing you continued success!
Thank you, Rey! Your insights and dedication to the field are commendable. Best of luck with your future endeavors in AI-assisted development!
Thank you, Sophie! I greatly appreciate your kind words. Best of luck to you too in your AI-assisted development journey. It's an exciting frontier to explore!
You're welcome, Rey! Thank you for your warm wishes. Looking forward to embracing the possibilities of AI-assisted development!
Thank you, Rey! Your expertise and engagement are truly commendable. I wish you success in all your future AI-assisted development projects!
You're welcome, Sophie! I appreciate your kind words and well wishes. Success to you too in your AI-assisted development projects. Let's shape the future together!
Absolutely, Sophie! ChatGPT can be a valuable resource for developers, providing guidance and suggestions during the development process. It's an exciting step towards enhancing the efficiency of programming endeavors.
Absolutely, Emily! Having an AI assistant that can provide suggestions and help streamline the programming process is a remarkable advancement. It can contribute to both individual productivity and collaborative development efforts.
Indeed, Sophie! The collaborative aspect of AI-assisted development is especially exciting. It can foster knowledge sharing and enable developers to learn from each other, ultimately leading to the growth of the entire community.
Absolutely, Emily! Collaboration and knowledge sharing among developers are vital for the growth of the entire community. AI-assisted development can greatly facilitate this process. Let's embrace this exciting change!
You're welcome, Emily! AI can indeed augment the development process without replacing the need for domain expertise. It's a valuable tool to drive productivity while ensuring quality code. Let's embrace this technological advancement responsibly!
Thank you, Rey! Your expertise and engagement have been truly valuable. Wishing you continued success in your AI-assisted development endeavors!
You're very welcome, Emily! Thank you for your kind words and well wishes. Continued success to you too in your AI-assisted development journey. Exciting things lie ahead!
Absolutely, Emily! Streamlining the programming process through AI assistance can have a profound impact on individual productivity and collaborative development. AI is truly transforming the way developers work!
Indeed, Sophie! The transformative power of AI in development is awe-inspiring. With AI as a helpful assistant, developers can navigate challenges more efficiently and push the boundaries of what we can achieve.
Absolutely, Emily! AI-assisted development is empowering both experienced and aspiring developers to reach new heights. I'm excited to be part of this journey!
Well said, Sophie and Emily! Embracing AI-assisted development opens doors to increased productivity, enhanced collaboration, and learning opportunities. It's an exciting time to be a developer!
Absolutely, Kelly! The advancements in AI-assisted development are empowering developers to thrive and create even more impactful solutions. It's a thrilling journey to be a part of!
Additionally, it would be valuable to join the developer communities and forums around ChatGPT and C/C++ development. Collaborating with fellow developers can elevate the learning experience and help in leveraging the tool optimally.
Rey, thanks for the informative article! While it's fascinating to integrate AI into development, I wonder about the availability of ChatGPT and its potential cost implications. Could you shed some light on that?
Great question, Tom! ChatGPT is available through OpenAI's API, and you can check their pricing details on the OpenAI website. The availability and cost depend on factors like usage volume, type of interaction, and desired features. It's best to refer to OpenAI's official documentation for the most accurate and up-to-date information.
Thanks for the detailed response, Rey! I'll check OpenAI's official documentation and assess the cost factors accordingly. Keep up the great work!
You're welcome, Rey! I'll make sure to delve deeper into OpenAI's resources and gain a better understanding. Thanks for the guidance!
No problem, Tom! Delving deeper into the resources will definitely give you a clearer picture. Feel free to ask if you have any further questions. Happy exploring!
Thank you, Rey! Your explanations and guidance have been illuminating. Best of luck with your AI-assisted development projects!
You're most welcome, Tom! I'm glad I could provide illuminating explanations. Thank you for your wishes, and best of luck to you too in your projects and coding endeavors!
Once again, thank you, Rey! Your explanations and insights have been truly helpful. Best of luck in all your future AI-assisted development projects!
You're most welcome, Tom! I'm grateful that you found my explanations helpful. I appreciate your kind words and well wishes. Continued success to you too in your endeavors, exploring the frontiers of AI-assisted development!
Hi Rey! Your article highlights the potential of ChatGPT in development. I'm curious, how does ChatGPT handle working with existing codebases? Can it provide insights in reviewing and refactoring existing code?
Hello, Olivia! ChatGPT can provide valuable insights when reviewing and refactoring existing code. It can offer suggestions on improving code readability, optimizing performance, and even identifying potential bugs or anti-patterns. However, as always, careful consideration and manual review by developers are crucial when making changes to existing codebases.
Thank you, Rey! Having AI-assisted insights during code reviews and refactoring can save time and ensure better code quality. I appreciate your explanation!
You're welcome, Olivia! AI-assisted insights can indeed be a great time-saver and quality enhancer during code reviews and refactoring. Keep up the excellent work in your development journey!
Thank you, Rey! Your advice and encouragement are greatly appreciated. Wishing you continued success in your endeavors!
Thank you, Rey! AI-assisted insights for code reviews and refactoring will certainly elevate the development process. Your inputs have been enlightening!
You're welcome, Olivia! AI-assisted insights can definitely enhance code reviews and refactoring, saving time and improving the quality of the codebase. Feel free to explore these tools further!
Thank you, Rey! Your guidance has been invaluable, and I look forward to incorporating AI assistance into my development workflow. Best wishes!
Thank you, Rey! Your advice and support are highly appreciated. Best of luck with your future undertakings!
You're welcome, Olivia! Your kind words mean a lot. Best of luck to you too in your programming journey and upcoming endeavors!
Thank you once again, Rey! Your expertise and encouragement are genuinely appreciated. Best of luck in your ongoing AI-assisted development journey!
You're welcome, Olivia! I'm glad I could provide expertise and encouragement. Thank you for your well wishes, and best of luck to you too in your coding and AI-assisted development journey!
Thank you all for an engaging discussion! Your questions and comments have been thought-provoking and insightful. I've thoroughly enjoyed participating in this conversation. Remember to harness the power of AI-assisted development responsibly. Best wishes to each and every one of you in your coding endeavors!
Thank you all for reading my article on enhancing development with ChatGPT. I'm excited to hear your thoughts and answer any questions you may have!
Great article, Rey! ChatGPT seems like a powerful tool for improving development productivity. How does it specifically revolutionize the C/C++ STL?
Thanks, Anna! ChatGPT revolutionizes the C/C++ STL by providing an interactive and conversational coding experience. It can assist developers with tasks like auto-completion, automatic error detection, and suggesting optimized code snippets.
That's impressive, Rey! I can see how having an AI-powered tool like ChatGPT can greatly enhance coding efficiency. Are there any limitations to consider?
Absolutely, Peter! While ChatGPT excels in assisting developers, it's important to keep in mind that it may not always provide the most optimal solutions. Its suggestions should be reviewed and validated by developers to ensure code quality and performance.
Interesting article, Rey! Do you have any examples of how ChatGPT has helped improve development in real-world scenarios?
Thank you, Lisa! One example is when a developer was struggling with a complex algorithm implementation. ChatGPT assisted by suggesting alternative approaches and highlighting potential errors, ultimately helping the developer find an optimized solution. It has also been useful for code refactoring and troubleshooting.
Thanks for sharing your insights, Rey! I'm curious about the learning curve for using ChatGPT effectively. How much time does it take for developers to get comfortable with it?
You're welcome, Samuel! The learning curve for ChatGPT varies depending on the developer's familiarity with natural language processing tools. Generally, developers can start using it with basic functionality quite quickly. However, mastering advanced features and understanding the AI model's limitations may take some practice.
Rey, I believe ChatGPT can be a game-changer for development. Are there any concerns about the model generating insecure or vulnerable code snippets?
Definitely, Michael! Generating secure and robust code is a critical concern. ChatGPT's model has been fine-tuned to prioritize safety and avoid generating insecure code. However, it's important for developers to review and validate the suggestions provided by ChatGPT, especially when it comes to security-related code snippets.
Great article, Rey! How does ChatGPT integrate with existing development environments? Can developers use it with popular IDEs?
Thank you, Emily! ChatGPT can be integrated with popular IDEs via plugins or APIs. These integrations allow developers to directly interact with ChatGPT within their preferred coding environment, making the experience seamless and enhancing productivity.
I've read about ethical concerns with AI models. How does OpenAI address such concerns when it comes to ChatGPT and its impact on the development process?
That's a crucial aspect, Daniel. OpenAI takes ethics seriously. They aim to ensure that AI systems like ChatGPT are designed to align with human values and avoid biased or harmful behavior. OpenAI actively encourages feedback and works on improvements to address ethical concerns and make the technology more robust and safe.
Rey, do you think ChatGPT will eventually replace traditional debugging and error detection tools?
That's an interesting question, Olivia! While ChatGPT can assist with debugging and error detection, it's designed to complement existing tools rather than replace them. Traditional debugging tools offer deep insights into code execution, which are crucial for effective debugging. ChatGPT focuses more on providing code suggestions and assisting with development tasks.
Thanks for this informative article, Rey! How does ChatGPT handle complex C/C++ template metaprogramming scenarios?
You're welcome, Sophia! ChatGPT can be helpful with complex C/C++ template metaprogramming scenarios by offering suggestions, explaining concepts, and helping developers refactor code. It can assist in making the code cleaner, more efficient, and easier to understand.
Hi Rey! Could you provide some guidance on when to rely on ChatGPT's suggestions versus when developers should rely on their own knowledge and expertise?
Certainly, Benjamin! Developers should consider ChatGPT's suggestions as helpful insights, but it's crucial to exercise critical thinking and use their own knowledge and expertise. While ChatGPT can provide valuable assistance, it's always essential to validate suggestions and prioritize code correctness, readability, and performance.
Rey, does ChatGPT support code generation for other programming languages apart from C/C++? Can it be extended to other domains?
That's a great question, Grace! As of now, ChatGPT primarily supports C/C++. However, there are ongoing efforts to extend its capabilities to other programming languages and domains. OpenAI aims to improve and expand the system to benefit developers working in various programming language ecosystems.
Rey, how does ChatGPT handle large codebases and complex projects? Can it provide valuable guidance in such cases?
Thanks for asking, Joshua! While ChatGPT can assist with large codebases and complex projects, its effectiveness may vary. In such cases, ChatGPT can still provide valuable guidance and suggestions, but developers should be mindful of reviewing and validating the suggestions in the context of the specific project requirements and constraints.
Rey, how does ChatGPT handle compatibility with different C/C++ compilers and versions? Are there any considerations for developers to keep in mind?
Great question, Isabella! ChatGPT is trained on a diverse dataset that includes code snippets compatible with different C/C++ compilers and versions. However, developers should still be cautious and validate compatibility with their specific target environments, especially when working with less commonly used compilers or older versions of the language.
Rey, what kind of hardware requirements are there for running ChatGPT effectively?
Good question, Max! ChatGPT's hardware requirements depend on the scale of usage. For individual developers, it can run on a typical development machine's hardware. However, for large-scale deployments or integrations into coding environments, more powerful hardware resources might be necessary to ensure optimal performance.
Thank you for this insightful article, Rey! How can developers provide feedback to OpenAI regarding ChatGPT's suggestions or report any issues they encounter?
You're welcome, Emma! Developers can provide feedback and report issues regarding ChatGPT's suggestions through OpenAI's platform. OpenAI encourages user feedback as it helps them understand the system's strengths and areas for improvement, ensuring a more robust and reliable tool for developers.
Rey, I'm concerned about possible biases in ChatGPT's suggestions. How does OpenAI address bias and ensure fairness in the system?
Addressing bias is a top priority for OpenAI, Nathan. They actively work on reducing both glaring and subtle biases in ChatGPT's responses. OpenAI utilizes a combination of techniques like fine-tuning, bias checks, and gathering user feedback to identify and mitigate bias, ensuring fairness and inclusivity in the system's output.
Hi Rey! Can developers customize ChatGPT's behavior based on their project requirements and coding best practices?
Hello, Sophie! Currently, developers can't directly customize ChatGPT's behavior, but OpenAI is actively exploring ways to allow users to have more control over the model's suggestions. While customization is not available yet, OpenAI values user feedback and considers it when further developing the system.
Rey, can ChatGPT assist with learning programming concepts for beginners?
Absolutely, Ethan! ChatGPT can be a valuable tool for beginners learning programming concepts. It can provide explanations, code examples, and step-by-step guidance, helping beginners understand concepts more easily and accelerate their learning process.
Thank you for the informative article, Rey! Are there any privacy concerns associated with using ChatGPT for development tasks?
You're welcome, Victoria! OpenAI takes privacy seriously. While using ChatGPT, the data exchanged with the model can improve its performance, but OpenAI retains the data only for a limited time. Moreover, OpenAI is actively working on reducing the amount of personal and sensitive information exchanged, minimizing privacy risks.
Great article, Rey! I'm excited to explore ChatGPT's capabilities for enhancing C/C++ development. Are there any known limitations with handling more niche or domain-specific libraries?
Thank you, Robert! ChatGPT has been trained on a wide range of code, including popular libraries. However, it may have limitations when working with more niche or domain-specific libraries. Developers should be aware of this and, if required, review the model's suggestions in those cases.
Rey, can ChatGPT be used offline, or is internet connectivity necessary for its functioning?
Good question, Julia! ChatGPT relies on an internet connection to function as it requires access to the model hosted on servers. While certain caching mechanisms can provide limited offline access, the full functionality of ChatGPT is predicated on a stable internet connection.
Thank you for sharing your insights, Rey! Could ChatGPT be used as a teaching tool for programming courses or coding bootcamps?
Absolutely, Liam! ChatGPT can be an excellent teaching tool for programming courses and coding bootcamps. Its ability to provide explanations, code examples, and assist with learning programming concepts can enhance the learning experience and provide valuable guidance to students.
Rey, does ChatGPT support real-time collaborative coding or is it primarily an individual developer tool?
Good question, Jacob! Currently, ChatGPT is primarily designed for individual developers. While it may be used in collaborative coding scenarios with multiple users, it lacks built-in real-time collaborative features. However, integrations and extensions can be developed to enable real-time collaboration in specific coding environments.
Hi Rey! Are there any costs associated with using ChatGPT for development tasks?
Hello, Ava! OpenAI provides both free and subscription-based access to ChatGPT. The availability and costs associated with using ChatGPT for development tasks depend on OpenAI's pricing models. I recommend checking OpenAI's platform for the latest information on costs and subscriptions.
Thank you all for your engaging comments and questions! I hope you found the article valuable, and I appreciate your interest in ChatGPT. Remember to provide your feedback and report any issues you encounter while using it. Let's revolutionize development together!