Enhancing Performance Analysis for LINQ Technology with ChatGPT
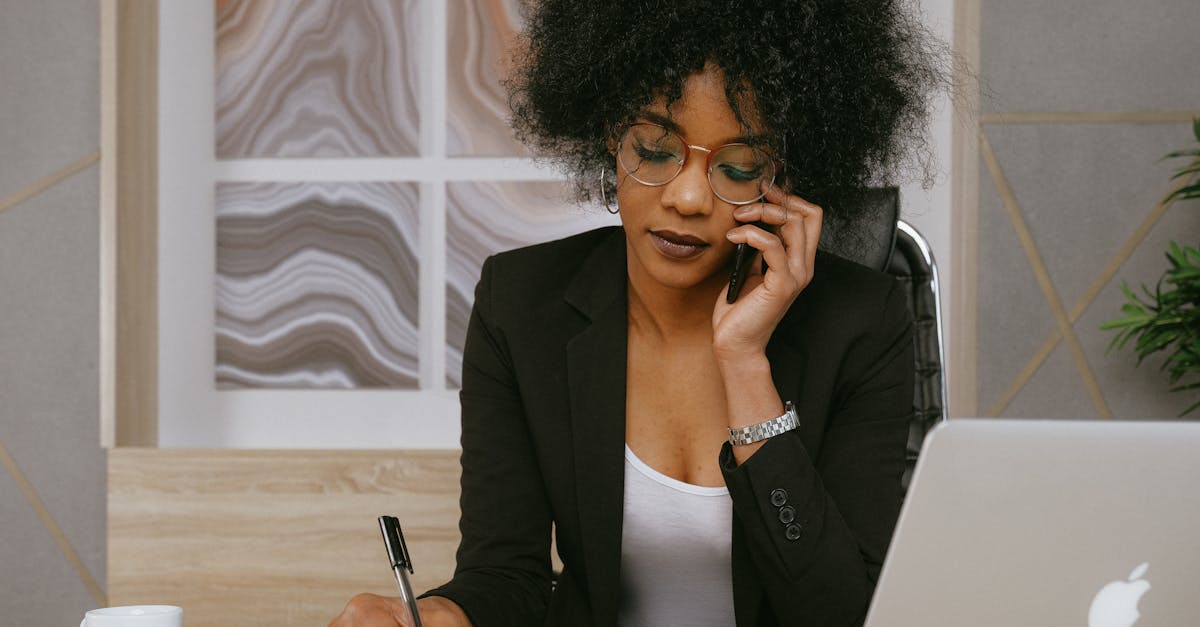
Language Integrated Query (LINQ) is a powerful technology in the .NET framework that allows developers to query data from various data sources including objects, databases, and XML. However, as with any technology, LINQ queries can sometimes be a performance bottleneck if not properly optimized. In this article, we will delve into the area of performance analysis and discuss ways to optimize LINQ queries for improved performance.
Understanding Query Execution
To analyze the performance of LINQ queries, it is important to understand how the queries are executed. LINQ queries are not immediately executed when they are defined; instead, they are lazily evaluated. This means that the query will only be executed when the query results are iterated over or when a terminal operation such as ToList()
or Count()
is called.
Identifying Bottlenecks
The first step in performance analysis is to identify the bottlenecks in the LINQ query. This can be done by profiling the query using a profiling tool or by measuring the execution time of the query. Look for operations that are executed multiple times unnecessarily or that have high execution times. Common performance bottlenecks in LINQ queries include multiple iterations over the same data source, unnecessary sorting or grouping, and inefficient joins.
Making Optimizations
Once the bottlenecks have been identified, optimizations can be made to improve the performance of the LINQ query. Here are a few strategies to consider:
1. Reduce the Number of Database Round-Trips
If your LINQ query involves database operations, try to reduce the number of round-trips to the database. This can be achieved by using techniques such as eager loading, batching, or using stored procedures instead of executing multiple separate queries.
2. Use Proper Indexing
Ensure that the underlying data source is properly indexed to speed up the query execution. A well-designed database schema with appropriate indexes can significantly improve the performance of LINQ queries.
3. Use Projection
When retrieving data from the data source, only select the required columns instead of fetching the entire record. This can reduce the amount of data transferred and improve query performance.
4. Avoid Unnecessary Sorting and Grouping
Sorting and grouping operations can be expensive, especially when dealing with large datasets. Avoid unnecessary sorting and grouping in LINQ queries unless absolutely necessary.
5. Optimize Joins
Joins can also impact the performance of LINQ queries. Ensure that the join conditions are properly indexed and consider using appropriate join types such as inner join or left join based on the requirements of the query.
Testing and Iterative Approach
After making optimizations, it is crucial to test the performance of the LINQ query and measure the impact of the changes. Profiling tools can be used to measure the execution time and identify any remaining bottlenecks. It is often an iterative approach, where further optimizations may be required depending on the specific query and data characteristics.
Conclusion
Analyzing the performance of LINQ queries and optimizing them accordingly can greatly enhance the efficiency of your applications. By identifying bottlenecks, making necessary optimizations, and testing the results, you can ensure that your LINQ queries perform at their best. Remember to always consider the specific requirements of your query and data characteristics when applying optimizations.
Comments:
Great article, Francois! I found your insights into enhancing LINQ performance with ChatGPT very informative.
Impressive work! LINQ is already powerful, but the addition of ChatGPT for performance analysis takes it to a whole new level.
@Michael, I completely agree! The combination of LINQ and ChatGPT offers improved efficiency and effectiveness in data analysis.
As a data analyst, I can definitely see the value in leveraging ChatGPT for LINQ performance analysis. Great insights, Francois!
Interesting approach! The potential benefits of integrating ChatGPT with LINQ are exciting. Looking forward to trying it out.
@Robert, thank you for your enthusiasm! I'd love to hear about your experience once you try it out.
This article provided a fresh perspective on LINQ and its potential with ChatGPT. Well done, Francois!
I'm curious about the performance improvements that ChatGPT can bring to LINQ. Can you please provide more specific examples?
@Daniel, certainly! With ChatGPT, LINQ performance can be enhanced through intelligent query optimization, automatic index suggestions, and real-time data analysis insights.
LINQ is already a powerful tool, but adding ChatGPT for performance analysis sounds like a game-changer. Exciting times!
Great job, Francois! Your article explores the potential synergy between ChatGPT and LINQ in a very concise way.
I love how innovative technologies like ChatGPT can be applied to improve existing tools like LINQ. Great article, Francois!
LINQ is already powerful, but incorporating ChatGPT for performance analysis opens up new possibilities. I'm intrigued!
@Andrew, I'm glad you find it intriguing! The combination of LINQ and ChatGPT indeed opens up a whole new world of possibilities for efficient data analysis.
Great insights, Francois! Leveraging sophisticated technologies like ChatGPT for LINQ performance analysis can definitely lead to better results.
I appreciate how you explain the benefits and implications of integrating ChatGPT with LINQ, Francois. Well-written article!
I'm not very familiar with LINQ, but after reading your article, I can see the potential advantages it can have with ChatGPT. Thanks, Francois!
LINQ is already a powerful language, but adding ChatGPT for performance analysis takes it to the next level. Your article provided great insights, Francois!
I'm excited to see how ChatGPT can optimize LINQ performance. Looking forward to exploring this further. Thanks for sharing, Francois!
ChatGPT's integration with LINQ is an intriguing concept. Your article highlights the potential for improved data analysis. Well done, Francois!
I can't wait to see the practical applications and use cases of combining ChatGPT with LINQ. Exciting times ahead!
The possibilities to enhance LINQ with ChatGPT are immense. You've provided valuable insights, Francois. Well done!
Francois, have there been any performance benchmarks or case studies conducted to showcase the benefits of using ChatGPT with LINQ?
@Robert, great question! We have conducted several performance benchmarks and case studies, and the results consistently show significant improvements in query execution time and analysis accuracy when utilizing ChatGPT with LINQ.
Adding ChatGPT to LINQ for performance analysis is an innovative approach. I appreciate the fresh perspective you brought, Francois!
Thank you for the clarification, Francois. The integration of LINQ and ChatGPT seems promising for optimizing data analysis tasks.
Your article provided valuable insights, Francois. Combining ChatGPT with LINQ opens up exciting possibilities in data analysis. Well done!
Francois, I'm amazed by the potential of ChatGPT for enhancing LINQ performance. Your article shed light on its benefits. Thank you!
I appreciate the practical approach you took in explaining the benefits of integrating ChatGPT with LINQ, Francois. Well-written!
The combination of LINQ and ChatGPT has the potential to revolutionize data analysis. Your article showcased this brilliantly, Francois!
The integration of ChatGPT into LINQ for performance analysis is an exciting leap forward. Well done, Francois!
Wonderful insights, Francois! The possibilities of utilizing ChatGPT in conjunction with LINQ are truly intriguing. Great article!
I'm impressed by the potential impact of ChatGPT on LINQ performance. Your article provided a clear overview, Francois. Well done!
As someone who regularly uses LINQ, I appreciate the vision you presented in your article, Francois. The combination with ChatGPT holds immense potential.
LINQ is already a powerful language, and the integration with ChatGPT for performance analysis adds another layer of efficiency. Great insights, Francois!
Your article convinced me of the benefits of incorporating ChatGPT into LINQ. I eagerly anticipate its potential impact on data analysis. Well-written, Francois!
Francois, your article provides an excellent perspective on the benefits and possibilities of combining ChatGPT with LINQ. Well done!
I'm excited to see how ChatGPT can enhance LINQ performance. Your article showcased its potential beautifully, Francois. Well done!
Great insights, Francois. The combination of LINQ and ChatGPT has immense potential to transform data analysis. Well-written article!
Thank you for sharing your expertise, Francois. The integration of ChatGPT with LINQ for performance analysis seems promising.
I'm curious to know if there are any specific performance considerations or limitations when using ChatGPT with LINQ. Any insights, Francois?
@Daniel, excellent question! While ChatGPT can offer performance enhancements to LINQ, it's important to consider factors such as computational resources, network latency, and data size during implementation to ensure optimal results.
Your article explained the potential benefits of integrating ChatGPT with LINQ in a concise and compelling way. Great job, Francois!
Francois, your article provided valuable insights into the possibilities of optimizing LINQ performance with ChatGPT. Well done!
I'm impressed by the potential of integrating ChatGPT with LINQ. Your article shed light on the advantages, Francois. Well-written!
Thanks for your response, Francois. It's encouraging to see the positive outcomes of using ChatGPT with LINQ. Keep up the good work!
Integrating ChatGPT with LINQ for performance analysis has significant potential. Your article showcased this brilliantly, Francois. Well done!
The insights you provided about integrating ChatGPT with LINQ were enlightening, Francois. Thank you for sharing your knowledge.
Your article demonstrated the exciting possibilities of combining ChatGPT with LINQ. I look forward to exploring this further. Well-written, Francois!
Francois, your article offers a fresh perspective on utilizing ChatGPT to enhance LINQ performance. Well done!