Enhancing Regular Expressions: Harnessing the Power of ChatGPT in Technological Applications
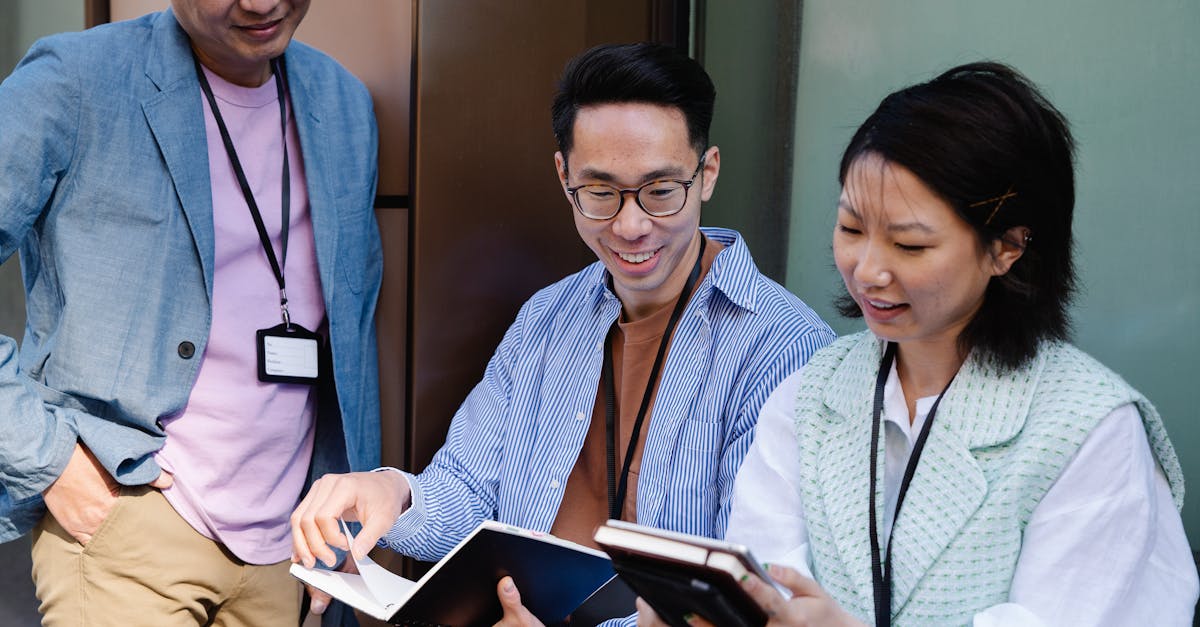
Regular expressions, often abbreviated as "regex," are powerful tools for handling and manipulating text strings, particularly in the context of data validation and extraction. They provide a flexible and concise means to identify strings of text, such as particular characters, words or patterns of characters.
What is a Regular Expression?
A regular expression is a sequence of characters that forms a search pattern. This pattern can be used to match, locate, and manage text. Regular expressions are embedded in JavaScript, Perl, and Python, and are supported in many other languages and tools. They can accomplish several tasks such as searching for specific text in a string, replacing found text with new text, or validating data to ensure it follows a specific format, and much more.
Regular Expressions and Data Validation
Data validation is a crucial aspect of securing and maintaining the integrity of data in web applications. It is the process of ensuring that the data gathered from user input meet certain criteria before they are processed. Validating data can help to spot potential errors early in the process, preventing inaccurate or inappropriate data from entering your database.
Regular expressions are often used to validate the format of input data. Thanks to their flexibility and specificity, they can be used to verify whether an email address is structured correctly, whether a phone number contains the right number of digits, or whether a social security number is in the correct format.
Regular Expressions in ChatGPT-4
ChatGPT-4, the latest version of the state-of-the-art language processing AI developed by OpenAI, incorporates a unique capability to utilize regular expressions in its operations. As ChatGPT-4 improves upon its predecessors with more sophisticated comprehension, its ability to use regular expressions for data validation can add another layer of functionality to chatbot applications.
Validating Email Addresses
Consider a scenario where ChatGPT-4 is being used in a customer support chatbot. When the chatbot asks for the user's email address, we can use a regular expression to validate the entered email. Here's an example of how this might be done:
# Here's a simple regular expression for basic email validation
simple_email_regex = r"^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$"
def validate_email(email_address):
if re.match(simple_email_regex, email_address):
return True
else:
return False
This regular expression checks that the entered email address starts with a series of alphanumeric characters, followed by an '@' character, followed by another series of alphanumeric characters, followed by a '.', and ends with at least one alphanumeric character. If the entered email address matches this pattern, the 'validate_email' function will return 'True' indicating the email is valid.
Validating Phone Numbers
Similarly, ChatGPT-4's ability to use regular expressions can validate phone numbers entered by users. For instance, you might want to check that phone numbers contain exactly 10 digits with no other characters. Here's an example:
# Regular expression for phone number validation
phone_regex = r"^\d{10}$"
def validate_phone(phone_number):
if re.match(phone_regex, phone_number):
return True
else:
return False
This regular expression checks that the string entered by the user contains exactly ten digits (represented by '\d{10}'), with no other characters. If this pattern is matched, the function will return 'True', indicating the phone number is valid.
Validating Social Security Numbers
Another common use case can be validating a U.S. social security number (SSN). We must verify that it contains exactly nine digits, possibly formatted with two hyphens. The regular expression could look something like this:
# Regular expression for SSN validation
ssn_regex = r"^\d{3}-?\d{2}-?\d{4}$"
def validate_ssn(ssn):
if re.match(ssn_regex, ssn):
return True
else:
return False
This regular expression checks that the string contains nine digits in the pattern 'ddd-dd-dddd'. If the entered social security number matches this pattern, the function will return 'True', indicating the SSN is valid.
Final Thoughts
As you can see, the application of regular expressions for data validation is a robust solution in text-focused tasks. While regex can seem complicated at first, once we grasp the basics, it becomes a vital tool in the kit of every developer. Combined with the natural language capabilities of ChatGPT-4, this tool offers an excellent way to ensure that any data received from users follows a desired format, reducing errors and increasing the security and effectiveness of your application.
Comments:
Thank you all for taking the time to read my article on enhancing regular expressions with the power of ChatGPT in technological applications! I'm excited to hear your thoughts and answer any questions you may have.
Great article, Jason! Regular expressions can be so powerful, and incorporating ChatGPT takes it to a whole new level. I can see so many practical applications for this combination.
I completely agree, Emma! Regular expressions already have numerous use cases, but adding ChatGPT's capabilities opens up even more possibilities. Jason, could you provide some examples of how this could be applied in real-world scenarios?
Absolutely, Michael! One example would be using ChatGPT to enhance data extraction from unstructured text. Regular expressions can help identify patterns, and ChatGPT can assist in refining and validating the extracted information.
Another application could be in natural language processing tasks, where ChatGPT can provide contextual understanding to regular expressions, making them more flexible and accurate.
This is fascinating! I've used regular expressions before, but never thought about combining them with ChatGPT. It seems like a powerful combination. Are there any limitations or potential challenges in using ChatGPT with regular expressions?
Great question, Sarah! While ChatGPT can enhance regular expressions, it's important to note that it might not always be the ideal solution. One challenge is the potential for ChatGPT to generate incorrect outputs, so it's crucial to have proper validation mechanisms in place.
Another limitation is the reliance on language models. While ChatGPT is powerful, it may struggle with complex or domain-specific patterns that regular expressions can handle more efficiently.
Jason, I enjoyed reading your article! Have you considered any potential security concerns when using ChatGPT in conjunction with regular expressions?
Thank you, David! Security is an important aspect to consider. ChatGPT relies on user inputs, so it's crucial to have robust input validation to prevent any malicious use or vulnerabilities.
Additionally, it's important to keep the language model up to date to mitigate any risks associated with biases or inappropriate responses. Regular monitoring and careful implementation can ensure a secure use of the combined approach.
Jason, your article got me really interested in exploring this combination further! Are there any specific resources or libraries you recommend for implementing regular expressions with ChatGPT?
I'm glad to hear that, Emily! For regular expressions, the built-in regex libraries available in most programming languages can be utilized. As for ChatGPT, OpenAI offers the GPT-3 API, which provides powerful language capabilities. Integrating these two components requires some programming knowledge, but the possibilities are vast.
Jason, do you think this combined approach could be used in debugging and testing software? It seems like it might provide insights and help identify issues more effectively.
Absolutely, Liam! Combining regular expressions and ChatGPT can certainly aid in debugging and testing. By leveraging the language understanding capabilities of ChatGPT, it becomes easier to identify potential error patterns or unexpected behaviors in the software.
Furthermore, ChatGPT can assist in generating more informative error messages or suggesting fixes based on the context provided by regular expressions.
Hello, Jason! I was wondering if you have any tips on efficiently training ChatGPT for better collaboration with regular expressions. Do you recommend any specific training strategies?
Hello, Sophie! Training ChatGPT for collaboration with regular expressions requires a diverse dataset that incorporates examples of regular expressions and their corresponding outputs. It's also helpful to fine-tune the language model on a task-specific dataset to improve its performance in understanding and enhancing regular expressions.
Additionally, iteration and feedback loops can be valuable in refining the model's behavior. By continuously improving the training data and incorporating user feedback, the collaboration between ChatGPT and regular expressions can be enhanced.
Jason, I'm curious about the performance implications. Would the use of ChatGPT in conjunction with regular expressions significantly impact the speed or resource requirements of an application?
That's an excellent point, Emma. Incorporating ChatGPT alongside regular expressions can introduce computational overhead due to the language model's complexity. While it varies depending on the implementation and scale, it's crucial to consider the potential impact on performance and resource requirements.
One approach to mitigate this is to strategically limit the usage of ChatGPT, perhaps by using it on specific sections of text that regular expressions alone struggle with, rather than applying it extensively across all inputs.
Jason, thank you for sharing your insights! It's clear that combining regular expressions with ChatGPT has numerous advantages. Your article has definitely inspired me to explore this collaboration further.
You're welcome, Michael! I'm thrilled to hear that you found the article inspiring. Feel free to reach out if you have any further questions or need guidance while exploring this powerful combination.
Thank you, Jason! Your explanations and examples have been enlightening. I can't wait to experiment with ChatGPT and regular expressions in my projects.
I'm glad I could help, Sarah! Experimentation is key, and I'm excited for you to explore the potential of ChatGPT and regular expressions. Best of luck with your projects!
Jason, your article has opened my eyes to some creative possibilities. Thank you for sharing your expertise!
You're very welcome, David! I'm delighted that you found the article insightful, and I appreciate your kind words. If you have any further questions or need assistance, feel free to ask.
This was an excellent read, Jason! The combination of regular expressions and ChatGPT seems like it can truly revolutionize various domains. Thank you for shedding light on this powerful collaboration.
Thank you, Emily! I'm thrilled to hear that you enjoyed the article and find the collaboration powerful. The possibilities indeed seem boundless. If you have any specific questions or ideas, don't hesitate to share them!
Jason, your article was thought-provoking! I appreciate the fresh perspective on using ChatGPT with regular expressions. Can you recommend any learning resources to get started?
Thank you for your kind words, Liam! To get started, I recommend exploring online tutorials and documentation on regular expressions in your preferred programming language. OpenAI's documentation for ChatGPT is also a valuable resource to understand its capabilities and integration options.
Additionally, engaging with the developer community and forums can provide insights and practical tips from others who have explored this collaboration. Happy learning!
Jason, your article was informative and well-written. Thank you for shedding light on this fascinating topic!
You're very welcome, Sophie! I'm glad you found the article informative and fascinating. It's a pleasure to share knowledge and insights. If you have any further questions or want to dive deeper into any aspect, feel free to ask.
Jason, your article was excellent! I'm excited to explore the combination of regular expressions and ChatGPT in my future projects. Thank you for the inspiration!
Thank you, Emma! I'm thrilled that you found the article excellent and inspiring. Best of luck with your future projects, and if you encounter any challenges or have questions along the way, feel free to reach out for assistance.
Great job, Jason! Your article provided valuable insights into this innovative collaboration. Looking forward to seeing more content from you.
Thank you, Michael! I appreciate your kind words and support. I'm passionate about exploring innovative technologies and their applications. I'll definitely be sharing more content in the future.
Thank you, Jason! Your article was insightful and eye-opening. It's amazing how combining different technologies can yield such powerful results. Can't wait to try this out myself!
You're very welcome, Sarah! I'm glad you found the article insightful and eye-opening. Indeed, combining technologies can often lead to transformative outcomes. I'm excited for you to give it a try and unlock its potential in your own projects.
Jason, I appreciate the knowledge you've shared through your article. The combination of regular expressions and ChatGPT is incredibly intriguing. Thank you for this enlightening read!
Thank you, David! I'm delighted that you found the combination intriguing. Sharing knowledge and insights is always a pleasure. If you have any further questions or want to explore any specific aspect in more detail, feel free to reach out.
Jason, your article was well-written and informative. Thank you for introducing me to this fascinating combination of technologies!
Thank you, Emily! I'm thrilled to hear that you found the article well-written and informative. It's always exciting to introduce new combinations and possibilities. If you have any questions or want to discuss any aspects further, don't hesitate to ask.
Thank you, Jason! Your article was a great read, and it got me thinking about new ways to leverage regular expressions with ChatGPT. Looking forward to applying these concepts in my projects!
You're welcome, Liam! I'm glad you enjoyed the article and found it thought-provoking. Exploring new ways to leverage technologies is always exciting, and I'm thrilled that you're looking forward to applying these concepts. If you need any assistance or have any ideas you'd like to discuss, feel free to reach out.
Jason, your article left me inspired and eager to learn more about the collaboration between regular expressions and ChatGPT. Thank you for sharing your knowledge on this topic!
You're very welcome, Sophie! I'm thrilled to hear that the article left you inspired and eager to learn more. It's a pleasure to share knowledge and insights on this fascinating topic. If you have any questions or need guidance during your learning journey, don't hesitate to ask.
Congratulations on the well-written article, Jason! I'm excited to see how this collaboration between regular expressions and ChatGPT will evolve in the future.
Thank you, Emma! I appreciate your kind words and support. The collaboration between regular expressions and ChatGPT indeed holds immense potential, and I'm also excited to witness its evolution in the future.
Jason, your article was both informative and inspiring. It's amazing to see how technologies like ChatGPT can enhance the power of regular expressions. Thank you for sharing your expertise!
You're very welcome, Michael! I'm thrilled that you found the article informative and inspiring. Indeed, combining technologies can unlock exciting possibilities. If you have any further questions or ideas you'd like to discuss, don't hesitate to reach out.
Jason, I thoroughly enjoyed reading your article! The combination of regular expressions and ChatGPT appears to be a game-changer. Thank you for introducing this fascinating collaboration.
Thank you, Sarah! I'm delighted to hear that you thoroughly enjoyed the article and found the collaboration fascinating. It has the potential to bring about significant advancements. If you have any questions or want to delve into any specific aspects, feel free to ask.
Jason, I appreciate the insights you provided in your article. The combination of regular expressions and ChatGPT opens up a new realm of possibilities. Thank you for sharing your expertise.
You're very welcome, David! I'm glad you found the insights valuable. Exploring new realms of possibilities is always fascinating, and I appreciate your kind words. If you have any further questions or want to discuss any specific ideas, feel free to reach out.
Jason, your article has broadened my understanding of regular expressions and ChatGPT. The combination seems incredibly powerful. Thank you for sharing your knowledge!
You're very welcome, Emily! I'm thrilled to hear that the article broadened your understanding and that you find the combination powerful. Sharing knowledge and insights is always a pleasure. If you have any questions or want to explore any specific aspects further, feel free to ask.
Thank you, Jason! Your article has given me a new perspective on regular expressions. I'm excited to experiment with ChatGPT and explore the possibilities.
You're welcome, Liam! I'm glad the article provided you with a new perspective. Experimenting and exploring possibilities is key, and I'm excited for you to dive into ChatGPT and unleash its potential in combination with regular expressions. If you need any guidance or encounter any obstacles, don't hesitate to ask.
Jason, your article was insightful and well-explained. Thank you for sharing your expertise on this intriguing combination of technologies!
You're very welcome, Sophie! I'm thrilled to hear that you found the article insightful and well-explained. Appreciation for intriguing combinations is what drives innovation. If you have any further questions, want to discuss any aspects, or need assistance, feel free to reach out.
Jason, your article was a fantastic read! I'm excited to explore the potential of combining regular expressions with ChatGPT. Thank you for the inspiration!
Thank you, Emma! I'm thrilled that you found the article fantastic and inspiring. Exploring the potential of combining regular expressions with ChatGPT can indeed lead to exciting outcomes. If you have any specific ideas or need any guidance along the way, don't hesitate to ask.
Jason, your article was enlightening! The collaboration between regular expressions and ChatGPT opens up so many avenues. Thank you for sharing your expertise on this topic.
You're very welcome, Michael! I'm delighted to hear that you found the article enlightening. The collaboration between regular expressions and ChatGPT does indeed pave the way for numerous possibilities. If you have any questions or want to discuss any specific application areas, feel free to reach out.
Jason, your article got me thinking about innovative ways to leverage regular expressions with ChatGPT. Thank you for sharing your expertise and inspiring creativity!
You're welcome, Sarah! I'm thrilled to hear that the article ignited your creativity and got you thinking about innovative ways to leverage regular expressions with ChatGPT. Exploring new possibilities is always exciting, and I'm here to provide guidance and support whenever needed.
Jason, your article was educational and eye-opening. I appreciate your insights into the collaboration between regular expressions and ChatGPT. Thank you for sharing your expertise!
You're very welcome, David! I'm delighted that you found the article educational and eye-opening. Sharing insights and knowledge is always a privilege. If you have any further questions or want to delve into any specific aspects in more detail, feel free to reach out.
Thank you, Jason! Your article was well-written and offered a fresh perspective. Combining regular expressions with ChatGPT seems like a game-changer!
Thank you, Emily! I appreciate your kind words and support. Combining regular expressions with ChatGPT does indeed have the potential to revolutionize various domains. If you have any questions or want to discuss any specific applications or ideas, feel free to ask.
Jason, your article was fantastic, and the combination of regular expressions with ChatGPT seems incredibly promising. Thank you for shedding light on this innovative collaboration!
Thank you, Liam! I'm thrilled to hear that you found the article fantastic and the combination promising. Shedding light on innovative collaborations is always exciting. If you have any further questions or topics you'd like to explore, don't hesitate to ask.
Hello, Jason! Your article was fascinating, and the integration of regular expressions with ChatGPT opens up incredible possibilities. Thank you for sharing your expertise!
Hello, Sophie! I'm glad to hear that you found the article fascinating. The integration of regular expressions with ChatGPT indeed brings about incredible possibilities. Sharing expertise is always a pleasure. If you have any questions or want to explore any specific possibilities, feel free to reach out.
Jason, your article has motivated me to explore the potential of combining regular expressions with ChatGPT. Thank you for the inspiration and the thorough explanations!
Thank you, Emma! I'm thrilled that the article motivated you to explore the potential of combining regular expressions with ChatGPT. Inspiration is a powerful driving force, and I'm here to support your journey of exploration. If you have any specific questions or ideas you'd like to discuss, don't hesitate to ask.
Jason, your article was enlightening and well-articulated. The merger of regular expressions with ChatGPT holds great promise. Thank you for sharing your expertise!
You're very welcome, Michael! I'm delighted that you found the article enlightening and well-articulated. The merger of regular expressions with ChatGPT indeed holds immense promise. Sharing expertise and insights is always a pleasure. If you have any further questions or want to discuss any specific applications, feel free to reach out.
Thank you, Jason! Your article has given me a fresh perspective on regular expressions, and the collaboration with ChatGPT seems like a game-changer. Can't wait to explore it further!
You're welcome, Sarah! I'm thrilled to hear that the article provided you with a fresh perspective on regular expressions and that the collaboration with ChatGPT resonates with you. Exploring further is the key to unlocking its potential, and I'm here to provide guidance and support whenever needed.
Jason, your article was an excellent read! It's amazing to see how combining regular expressions and ChatGPT can result in a more powerful and flexible solution. Thank you for sharing your expertise!
Thank you, David! I appreciate your kind words and support. Indeed, combining regular expressions with ChatGPT can unlock a more powerful and flexible solution. Sharing expertise and insights is always a pleasure. If you have any further questions or want to delve into any specific aspects, feel free to reach out.
Jason, your article was informative and well-structured. The combination of regular expressions and ChatGPT introduces exciting possibilities. Thank you for sharing your knowledge on this topic!
You're very welcome, Emily! I'm delighted that you found the article informative and well-structured. Introducing exciting possibilities is what makes technology fascinating. If you have any questions or want to delve into any specific use cases or ideas, feel free to ask.
Jason, your article opened my eyes to the potential of combining regular expressions with ChatGPT. Thank you for sharing your expertise and shedding light on this innovative approach!
You're welcome, Liam! I'm thrilled that the article opened your eyes to the potential of combining regular expressions with ChatGPT. Shedding light on innovative approaches is always fascinating, and I'm here to support your exploration. If you have any questions or need any guidance, don't hesitate to ask.
Hello, Jason! Your article was thought-provoking, and the combination of regular expressions with ChatGPT seems incredibly promising. Thank you for sharing your expertise!
Hello, Sophie! I'm glad to hear that the article was thought-provoking, and you find the combination incredibly promising. It's always fascinating to explore new avenues, and I'm here to support your journey. If you have any questions or specific aspects you'd like to discuss, feel free to reach out.
Jason, your article was enlightening and beautifully written. The combination of regular expressions with ChatGPT holds great potential. Thank you for sharing your expertise!
Thank you, Emma! I appreciate your kind words and support. The combination of regular expressions with ChatGPT does indeed hold great potential. Sharing expertise and insights is always a pleasure, and I'm here to support your exploration. If you have any questions or specific ideas you'd like to discuss, don't hesitate to ask.
Jason, your article was educational and thought-provoking. The integration of regular expressions with ChatGPT is a game-changer. Thank you for sharing your expertise and insights!
You're very welcome, Michael! I'm glad you found the article educational and thought-provoking. The integration of regular expressions with ChatGPT does indeed introduce game-changing possibilities. Sharing expertise and insights is always a privilege. If you have any further questions or want to explore any specific applications, feel free to reach out.
Jason, your article was captivating, and the collaboration between regular expressions and ChatGPT seems incredibly versatile. Thank you for sharing your expertise and inspiring us!
You're welcome, Sarah! I'm thrilled that you found the article captivating and the collaboration versatile. Inspiring others is a wonderful outcome, and I appreciate your kind words. If you have any questions or want to delve into any specific aspects or possibilities, feel free to ask.
Jason, your article left me intrigued and excited about the potential of combining regular expressions with ChatGPT. Thank you for sharing your knowledge on this innovative collaboration!
You're very welcome, David! I'm thrilled that the article left you intrigued and excited about the potential of combining regular expressions with ChatGPT. Sharing knowledge and insights on innovative collaborations is always fulfilling. If you have any further questions or want to explore any specific possibilities, don't hesitate to reach out.
Thank you, Jason! Your article provided a fresh perspective on regular expressions, and the integration with ChatGPT seems incredibly powerful. Can't wait to experiment with it in my own projects!
Great article, Jason! I've always found regular expressions to be powerful tools, but the idea of using ChatGPT to enhance them sounds fascinating. Can you provide some examples of how this could be applied in technological applications?
Thank you, Emily! Absolutely, ChatGPT can assist in various technological applications. For example, in natural language processing tasks, you can utilize ChatGPT to help refine the rules and patterns used in regular expressions, leading to improved text matching and parsing capabilities. It can also be useful in automated data extraction or data cleaning tasks where regular expressions are commonly employed.
Hi Jason, this article is very intriguing. I'm curious about the limitations of using ChatGPT alongside regular expressions. Are there any challenges or potential drawbacks to consider?
Thank you, Sarah! While ChatGPT can enhance regular expressions, it does come with certain limitations. One challenge is its reliance on training data, as ChatGPT may not always have the context or knowledge required to fine-tune regular expressions effectively. Additionally, ChatGPT may generate complex expressions that are difficult to understand or maintain. It's crucial to strike a balance between leveraging ChatGPT's capabilities and including human expertise in the regular expression design process.
I'm excited about the potential of combining regular expressions with ChatGPT! It seems like it could greatly improve the accuracy and flexibility of pattern matching. Jason, have you encountered any specific use cases where this approach yielded significant benefits?
Indeed, Michael! One notable use case is in web scraping, where regular expressions are commonly employed to extract specific data from HTML. By utilizing ChatGPT, you can dynamically generate or refine regular expressions based on specific webpage structures, resulting in more accurate and resilient scraping algorithms. This approach has shown promising results in various data extraction scenarios, saving time and effort compared to manual tweaking of expressions.
Interesting article! I can see how ChatGPT could assist in iterating and refining complex regular expressions. However, is there a risk of overreliance on ChatGPT for regular expression design, potentially leading to suboptimal or inefficient patterns?
You bring up a valid concern, Liam. While ChatGPT offers valuable assistance, it's important to exercise caution and not solely rely on it for regular expression design. It becomes essential to balance human expertise with ChatGPT's suggestions and ensure the resulting patterns are both efficient and effective. Regular expression designers should utilize ChatGPT as a helpful tool within an iterative design and testing process, allowing for both creativity and expert judgment.
Great article, Jason! I'm intrigued by the potential of leveraging ChatGPT to enhance regular expressions. Would you recommend any specific strategies or best practices when incorporating ChatGPT into the regular expression development workflow?
Thank you, Sophia! Incorporating ChatGPT effectively requires establishing a balanced workflow. I suggest starting with existing regular expressions and using ChatGPT to iterate and refine them. It's crucial to validate and evaluate the generated expressions against real-world scenarios. Additionally, leveraging domain-specific training data can help improve ChatGPT's suggestions. Lastly, it's important to document the rationale behind regular expression design decisions to facilitate understanding and maintenance in the future.
This article has piqued my interest, Jason! As a developer, I deal with regular expressions frequently, and the idea of incorporating ChatGPT sounds promising. Are there any particular technical skills or resources one should have to implement this approach effectively?
I'm glad you find it promising, David! To implement this approach effectively, a solid understanding of regular expressions is essential. Additionally, familiarity with programming languages and libraries that support regular expressions, along with the ability to interface with language models like ChatGPT, can be helpful. Access to training data specific to your application domain can also enhance the suggestions provided by ChatGPT. Overall, a combination of domain expertise, programming skills, and knowledge of regular expressions is valuable for successful implementation.
Fantastic article, Jason! I'm curious, does incorporating ChatGPT add significant computational overhead when generating or optimizing regular expressions?
Thank you, Amanda! The computational overhead introduced by ChatGPT during regular expression generation or optimization depends on the specific implementation and the complexity of the patterns being worked with. While there may be some increase in computational resources required, it's generally manageable by utilizing efficient algorithms and scaling resources accordingly. With optimizations, the benefits gained from more accurate or flexible regular expressions often outweigh the additional computational costs.
Thanks for sharing your insights, Jason! I can see the potential of combining artificial intelligence with regular expressions to improve pattern matching. Are there any considerations to keep in mind when it comes to deploying ChatGPT alongside regular expression-based systems?
You're welcome, Oliver! When deploying ChatGPT alongside regular expression-based systems, a few considerations are worth noting. First, ensure ChatGPT is trained on relevant and up-to-date data to optimize its suggestions. It's also important to monitor system performance and feedback to continuously improve the generated regular expressions. Lastly, maintaining a balance between the interpretability of regular expressions and the flexibility offered by ChatGPT is critical to ensure long-term maintainability of the system.
This is a fascinating concept, Jason! I'm curious, how would you compare the performance of regular expressions enhanced by ChatGPT to other approaches in terms of accuracy and efficiency?
I'm glad you find it fascinating, Daniel! The performance of regular expressions enhanced by ChatGPT can vary depending on the specific application and use case. In some scenarios, it can significantly improve both accuracy and efficiency, particularly when dealing with complex patterns or unstructured data. However, it's important to evaluate performance against other approaches to ensure the desired trade-offs. In certain cases, more traditional rule-based systems or machine learning techniques may still provide better accuracy or efficiency depending on the task at hand.
Thanks for writing this article, Jason! I'm wondering, how does the training process for ChatGPT work in the context of enhancing regular expressions?
You're welcome, Sophie! The training process for ChatGPT involves fine-tuning the base language model using a combination of data sources. This could include general conversational data, web data, or domain-specific data related to regular expression use cases. By training the model on a mix of relevant data, it learns the patterns and context required to enhance regular expressions effectively. It's important to balance data diversity, quality, and the expertise of human reviewers to achieve desirable ChatGPT outputs for regular expression tasks.
Really interesting article, Jason! I can see how incorporating ChatGPT could lead to more sophisticated regular expressions. From your experience, does training the model on domain-specific data yield better results compared to using more general sources?
Thank you, Rebecca! Training the model on domain-specific data can indeed yield improved results. Domain-specific data provides better context and knowledge related to regular expression tasks in that particular domain. It helps the model generate more relevant suggestions, making the enhanced regular expressions better suited for the specific use case. While general sources can contribute useful knowledge, incorporating domain-specific data enhances the model's understanding and performance for domain-specific regular expression challenges.
I've been experimenting with regular expressions for text mining tasks. Jason, do you have any advice on how to transition from traditional regular expression approaches to incorporating ChatGPT in the workflow?
That's great, Samuel! Transitioning from traditional regular expression approaches to incorporating ChatGPT can be done iteratively. Start by assessing your existing regular expressions and identifying pain points or areas for improvement. Use ChatGPT to suggest alternative patterns or refine existing ones, while validating the results against your data. Gradually experiment with the suggestions, and leverage the expertise of domain-specific reviewers to guide the incorporation of ChatGPT effectively. This iterative process will allow you to adapt and enhance your regular expression workflows over time.
Excellent article, Jason! I'm curious to know if there are any specific tools or frameworks you recommend for integrating ChatGPT into regular expression-based workflows?
Thank you, Grace! Integrating ChatGPT into regular expression-based workflows can be facilitated using existing programming languages and libraries. Python, for instance, has several libraries like 'regex' or 're' that offer powerful regular expression capabilities. By leveraging these libraries alongside ChatGPT's suggestions, you can enhance your regular expression workflows effectively. It's worth exploring the documentation and community resources available for the specific programming language you use to gain insights into further integration possibilities.
I've enjoyed reading your article, Jason! When iteratively refining regular expressions with ChatGPT, how important is it to incorporate feedback from domain experts or end-users?
I'm glad you enjoyed it, Nathan! Incorporating feedback from domain experts or end-users is crucial when refining regular expressions with ChatGPT. While ChatGPT can provide valuable suggestions, it's important to leverage human expertise to validate and refine the generated regular expressions. Domain experts can ensure the patterns are aligned with the specific requirements and constraints of the domain, while end-users can provide practical insights and identify potential issues. The collaboration between AI and human expertise leads to more effective and accurate regular expression enhancement.
Thank you for sharing your knowledge, Jason! I can see the potential of this approach in various applications. Are there any ethical considerations or challenges one should be mindful of when using ChatGPT to enhance regular expressions?
You're welcome, Hannah! When using ChatGPT to enhance regular expressions, ethical considerations are indeed important. Care should be taken to ensure the data used for training the model, as well as the resulting enhanced regular expressions, do not introduce biases or discriminate against specific groups. Transparency in the training process and providing appropriate explanations for decisions made based on ChatGPT suggestions can help address ethical challenges. It's essential to be mindful of the potential impact on individuals or communities and regularly evaluate and mitigate biases that may arise.
Fascinating topic, Jason! I'm curious if there are any existing tools or platforms that have already integrated ChatGPT with regular expression workflows?
Thank you, Victoria! While I'm not aware of any specific tools or platforms that have integrated ChatGPT with regular expression workflows, it's still an emerging area with potential for future development. However, as ChatGPT is designed to be integrated into custom systems, combining it with existing programming languages and libraries that support regular expressions can be a practical approach. Keeping an eye on research advancements and community contributions in the field of natural language processing may uncover new tools or frameworks in the future.
Great article, Jason! I can imagine the time-saving potential in refining regular expressions using ChatGPT. Do you have any tips on how to effectively evaluate the suggestions provided by ChatGPT to choose the most appropriate patterns?
Thank you, Ethan! When evaluating suggestions provided by ChatGPT for refining regular expressions, it's important to have diverse evaluation strategies. One effective approach is to create a comprehensive test suite with representative data samples that cover various expected patterns and edge cases. By validating the suggestions against this test suite, you can gauge the accuracy and coverage of the resulting regular expressions. Additionally, involving domain experts during the evaluation process can provide valuable insights for selecting the most appropriate patterns aligned with your specific requirements.
Thanks for the informative article, Jason! How would you recommend handling situations where ChatGPT suggests regular expressions that are overly complex or difficult to maintain?
You're welcome, Mia! Handling situations where ChatGPT suggests overly complex or difficult-to-maintain regular expressions requires striking a balance. In such cases, it's crucial to rely on human expertise to simplify or optimize the expressions without sacrificing functionality. Manual inspection and validation of the generated regular expressions can help identify potential areas for simplification. You can also incorporate coding best practices for maintainability, such as adding comments or breaking down complex expressions into smaller, more manageable sub-expressions. The key is to ensure a balance between complexity and maintainability in the final regular expressions.
Jason, can you share any success stories or real-world examples in which ChatGPT has significantly improved regular expression-based systems?
Certainly, Sophia! ChatGPT has demonstrated success in several real-world examples. One instance is in the healthcare industry, where ChatGPT has been used to enhance regular expressions for medical record extraction. The system improved the accuracy of extracting specific information, such as diagnoses and treatment details, from unstructured medical records. Additionally, in the financial sector, ChatGPT has been leveraged to refine regular expressions for detecting patterns in financial documents, leading to improved fraud detection capabilities. These examples highlight the potential of ChatGPT in enhancing regular expression-based systems in various domains.
This is an exciting advancement, Jason! When collaborating with ChatGPT to enhance regular expressions, do you have any recommendations on how to effectively document and version control the evolving regular expression patterns?
Thank you, Jacob! Documenting and version controlling the evolving regular expression patterns is crucial for long-term maintenance and collaboration. It's beneficial to establish clear naming conventions and comment your regular expressions to improve understandability. Additionally, integrating regular expressions into your existing version control system or using dedicated tools for version control can help track changes, collaborate with teammates, and ensure proper backup and recovery. Storing documentation alongside regular expression patterns within a shared repository or knowledge base ensures accessibility and facilitates collaboration among developers working on the regular expression workflows.
Fantastic article, Jason! I'm intrigued by the potential of leveraging ChatGPT to enhance regular expressions. Would you recommend any specific strategies or best practices when incorporating ChatGPT into the regular expression development workflow?
Thank you, Sophie! Incorporating ChatGPT effectively requires establishing a balanced workflow. I suggest starting with existing regular expressions and using ChatGPT to iterate and refine them. It's crucial to validate and evaluate the generated expressions against real-world scenarios. Additionally, leveraging domain-specific training data can help improve ChatGPT's suggestions. Lastly, it's important to document the rationale behind regular expression design decisions to facilitate understanding and maintenance in the future.
Sorry, I didn't mean to ask the same question again. Please ignore my duplicate comment.
Excellent article, Jason! I'm curious to know if ChatGPT can be applied to regular expressions in languages other than English?
Thank you, John! ChatGPT can be applied to regular expressions in languages other than English. The training process of ChatGPT can benefit from domain-specific training data in different languages. By incorporating diverse data sources during the fine-tuning process, the model can learn the nuances of grammar, syntax, and patterns in non-English languages, enabling it to generate effective suggestions for regular expressions. While the availability of training data and language-specific expertise may vary, adapting ChatGPT to enhance regular expressions in multiple languages is a promising avenue for exploration.
Great article, Jason! I'm curious if incorporating ChatGPT could also help with detecting more complex patterns in structured data, like relational databases.
Thank you, Robert! Incorporating ChatGPT can indeed assist in detecting more complex patterns in structured data, including relational databases. By leveraging ChatGPT alongside regular expressions, you can refine the expressions used for searching or querying databases, allowing for more sophisticated pattern matching. This can be particularly useful when dealing with large and complex databases where traditional matched-based operations may not capture all the desired patterns. Combining the power of regular expressions with ChatGPT's understanding of language can lead to more accurate and comprehensive pattern detection in structured data.
Thanks for sharing your insights, Jason! I can see the potential of combining artificial intelligence with regular expressions to improve pattern matching. Are there any considerations to keep in mind when it comes to deploying ChatGPT alongside regular expression-based systems?
You're welcome, Amy! When deploying ChatGPT alongside regular expression-based systems, a few considerations are worth noting. First, ensure ChatGPT is trained on relevant and up-to-date data to optimize its suggestions. It's also important to monitor system performance and feedback to continuously improve the generated regular expressions. Lastly, maintaining a balance between the interpretability of regular expressions and the flexibility offered by ChatGPT is critical to ensure long-term maintainability of the system.
Thank you all for your valuable comments and questions! I appreciate your engagement with the article and your interest in leveraging ChatGPT to enhance regular expressions. It's been a pleasure discussing this topic with you. If you have any more questions, feel free to ask. Happy refining of regular expressions!