Enhancing Secure Coding in C++ Language: Leveraging the Power of ChatGPT
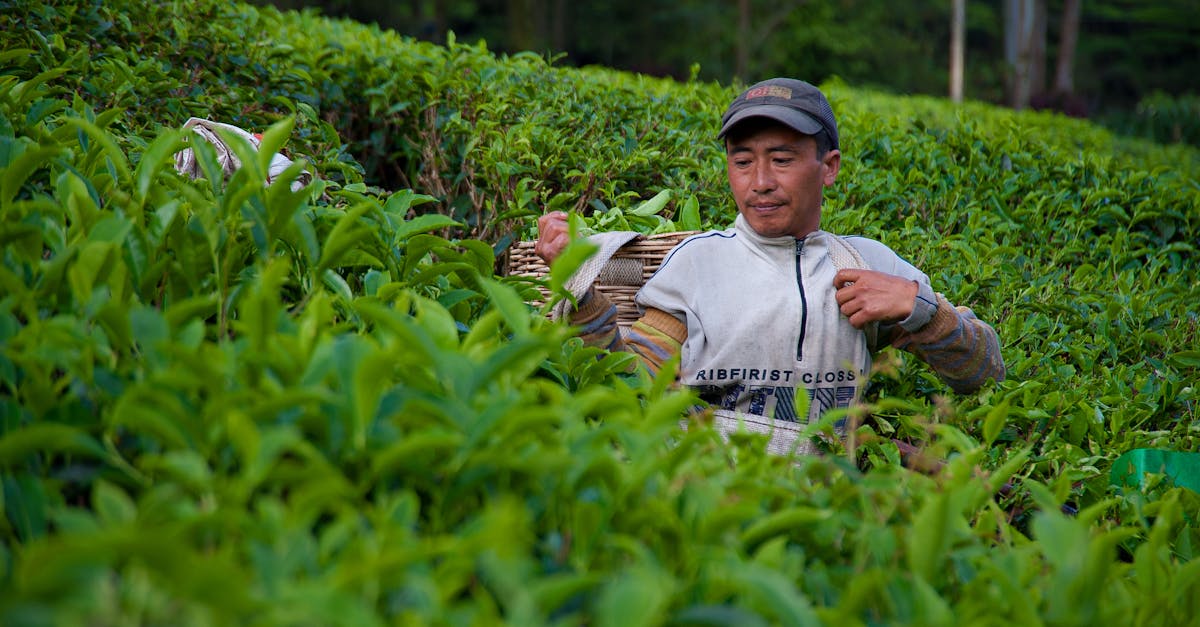
With the increasing demand for reliable and secure software applications, it is essential for developers to follow secure coding practices. C++ is a powerful programming language widely used for developing both system and application-level software. In this article, we will explore some best practices for secure coding in C++.
1. Input Validation
Properly validating user input is paramount to prevent security vulnerabilities such as buffer overflows, SQL injection, and cross-site scripting. Always validate and sanitize user input before using it in your code. Use functions provided by the C++ Standard Library, such as std::cin
and std::getline
, to read input safely.
2. Memory Management
Memory management is crucial to prevent common vulnerabilities like memory leaks and buffer overflows. Use modern C++ features like smart pointers (std::unique_ptr
and std::shared_ptr
) to handle memory allocation and deallocation automatically. Avoid using raw pointers whenever possible.
3. String Manipulation
Improper string manipulation can lead to security vulnerabilities such as buffer overflows, format string attacks, and SQL injections. Always use secure string manipulation functions provided by the Standard Library, such as std::string
and std::string_view
, instead of C-style string functions like strcpy
and strcat
.
4. Error Handling
Effective error handling plays a significant role in preventing security vulnerabilities and maintaining the integrity of your code. Always check the return values of functions and handle errors appropriately. Use exceptions for exceptional situations and ensure that sensitive information is not exposed in error messages.
5. Encryption and Cryptography
When dealing with sensitive data, it's crucial to implement proper encryption and cryptography techniques. Utilize well-vetted libraries or algorithms for encryption tasks and ensure that keys, passwords, and other confidential information are stored securely.
6. Access Control
Implement proper access control mechanisms to prevent unauthorized access to sensitive resources. Use access modifiers, such as private
and public
, to encapsulate data and restrict its access. Additionally, employ authentication and authorization mechanisms to ensure that only authorized users can access critical functionalities.
7. Regular Code Review and Testing
Regularly review your code for security vulnerabilities and perform thorough testing to identify any potential security risks. Use static code analysis tools to detect common coding mistakes and security flaws. Additionally, conduct penetration testing to verify the robustness and security of your application.
Conclusion
Secure coding practices are crucial in C++ to ensure the development of reliable and secure software applications. By adhering to the best practices mentioned in this article, developers can significantly reduce the chances of introducing security vulnerabilities into their code. Stay updated with the latest security advancements and continuously enhance your knowledge to stay ahead of potential threats.
Comments:
Great article, Amanda! I completely agree that leveraging the power of ChatGPT can be a game-changer for enhancing secure coding in C++.
I'm curious, Amanda, how is ChatGPT specifically used to enhance secure coding? Could you provide some examples?
Thank you, Michael! I appreciate your support. Emma, ChatGPT can be used during code reviews to identify potential security vulnerabilities in the code. It can also assist developers in generating secure code snippets or providing guidance on best coding practices to prevent common security issues.
This sounds interesting, Amanda. Are there any limitations or challenges we should be aware of when using ChatGPT for secure coding?
Good question, David! While ChatGPT can be helpful, it's important to note that it relies on patterns and examples from the training data. It may not catch all edge cases or novel attack vectors. It's crucial to supplement it with manual reviews and other security tools for a comprehensive approach.
I think leveraging AI for secure coding is a great idea. It could potentially save developers a lot of time and effort. Thanks for the informative article, Amanda!
I have concerns about overreliance on AI for secure coding. It's important for developers to understand the underlying security principles. AI can assist, but it shouldn't replace the knowledge and expertise of the coders.
Amanda, could you please share any real-world examples or success stories where ChatGPT has proven valuable in enhancing secure coding?
Certainly, Laura! One example is using ChatGPT to identify common security vulnerabilities in existing codebases, helping developers prioritize remediation efforts. It has also been successful in providing automated code reviews, catching potential security flaws that might have been overlooked.
I'm a C++ developer and always looking for ways to improve secure coding practices. Amanda, any recommendations on how to get started with leveraging ChatGPT for this purpose?
That's fantastic, Jessica! To get started, you can integrate ChatGPT into your code review process. Use it to analyze snippets of code or ask it questions related to secure coding practices. It's important to fine-tune the model on relevant security-related data to improve its suggestions and accuracy over time.
Amanda, as AI continuously evolves, how do you see the future of secure coding practices? Will AI play an even more significant role?
Great question, Robert! I believe AI will indeed play a more significant role in the future of secure coding practices. As AI models become more advanced and trained on larger datasets, they will be able to provide even better guidance and identify increasingly complex security issues. However, human expertise and judgment will always remain crucial.
Amanda, are there any privacy concerns associated with using ChatGPT for secure coding? How is user data handled?
Valid concern, Daniel. User data is an important aspect. OpenAI has taken steps to improve privacy by providing the option for organizations to deploy ChatGPT on their infrastructure. This allows better control over sensitive code and reduces the exposure of data to external parties.
Amanda, what specific security-related scenarios do you think ChatGPT is best suited for in the context of secure coding?
Great question, Michael! ChatGPT is well-suited for identifying common security vulnerabilities like SQL injection, cross-site scripting, or buffer overflows in the code. It can also assist with generating secure authentication mechanisms or recommending appropriate encryption algorithms.
Thank you for elaborating, Amanda! It seems ChatGPT can be a valuable tool in the secure coding process by providing automated feedback and code analysis.
I appreciate your insights, Amanda. It's important for organizations to strike a balance between utilizing AI and maintaining a strong foundation of secure coding practices within their development teams.
Amanda, what are the resources or reference materials you recommend for developers who want to dive deeper into the topic of secure coding with AI?
Good question, Adam! Developers interested in secure coding with AI can explore resources like OpenAI's documentation, security-related forums, and research papers on the topic. It's also valuable to participate in relevant communities to exchange ideas and experiences with fellow developers.
I think organizations should strive to create a culture of secure coding practices where developers can benefit from AI tools like ChatGPT while also continuously improving their own knowledge and skills.
Absolutely, Sophie! AI tools can be valuable aids, but they should supplement, not replace, the human intelligence and expertise in secure coding.
Amanda, thank you for sharing your expertise on secure coding with ChatGPT in this article. It has definitely sparked my interest in exploring this area more.
I agree, Laura. It's an intriguing field, and I'm excited to see how AI can contribute to enhancing secure coding practices.
Thank you, Amanda, for shedding light on the potential of ChatGPT in secure coding. It's clear that the combination of AI and human expertise is the way forward.
Well said, Daniel. Let's embrace the potential while staying grounded in good coding practices.
Thank you, Amanda, for addressing our questions and sharing your knowledge. This discussion has been enlightening.
Indeed, Emma. It was a pleasure to be part of this discussion regarding the exciting prospect of AI in secure coding.
Thanks again, Amanda, for this informative article. I look forward to exploring secure coding with AI further.
I couldn't agree more, Adam. Amanda has given us a lot to consider when it comes to leveraging AI for secure coding.
Thank you, Amanda, for taking the time to interact with us and provide valuable insights. It's been a great discussion.
I completely agree with Ryan. Thanks, Amanda, for your expertise and for answering our questions.
This article and discussion have been eye-opening. Secure coding with AI is certainly an exciting area to explore.
Absolutely, Laura. The future holds great possibilities for the intersection of AI and secure coding practices.
I'm grateful for this opportunity to learn from Amanda and engage in this discussion. Thank you all for your valuable contributions!
Indeed, Emma. It was a pleasure to be a part of this enlightening conversation. Thank you, Amanda!
Thank you, Amanda, for guiding us through the benefits and considerations of using AI in secure coding. It has been an informative exchange.
Thank you, Amanda, for sharing your expertise. This discussion has definitely given me valuable insights into the role of AI in secure coding.
Thank you, Amanda, for shedding light on how AI can enhance secure coding practices. It's an exciting and promising field.
This discussion has been both engaging and educational. Thank you, Amanda, for sharing your knowledge and perspectives.
Thank you all for the insightful comments and questions. It's refreshing to see such a vibrant discussion around secure coding with AI.
Absolutely, Ryan. It's been a pleasure exchanging thoughts with everyone here. Thank you, Amanda, for this valuable discussion.
Thank you, Amanda, for sharing your expertise and guiding this discussion. Secure coding with AI is an area worth exploring.
Indeed, Michael. The insights shared here will undoubtedly inspire further exploration and research in this field.
Thank you again, Amanda, and everyone else. This discussion has broadened my perspective on the potential use of AI in secure coding.
I couldn't agree more, Emma. It's been an enriching conversation. Thank you, Amanda, for facilitating this.
Thank you, Amanda, for gathering us all here for this fruitful discussion. Let's continue exploring the possibilities of AI in secure coding.
Absolutely, Jessica. The ideas and insights shared here will surely contribute to achieving more secure coding practices in the future.
Thank you, Amanda, for your expertise and for bringing us together in this discussion on AI and secure coding. It's been a pleasure.