Enhancing the Database-First Approach in Entity Framework with ChatGPT: A Powerful Combination for Simplified Development
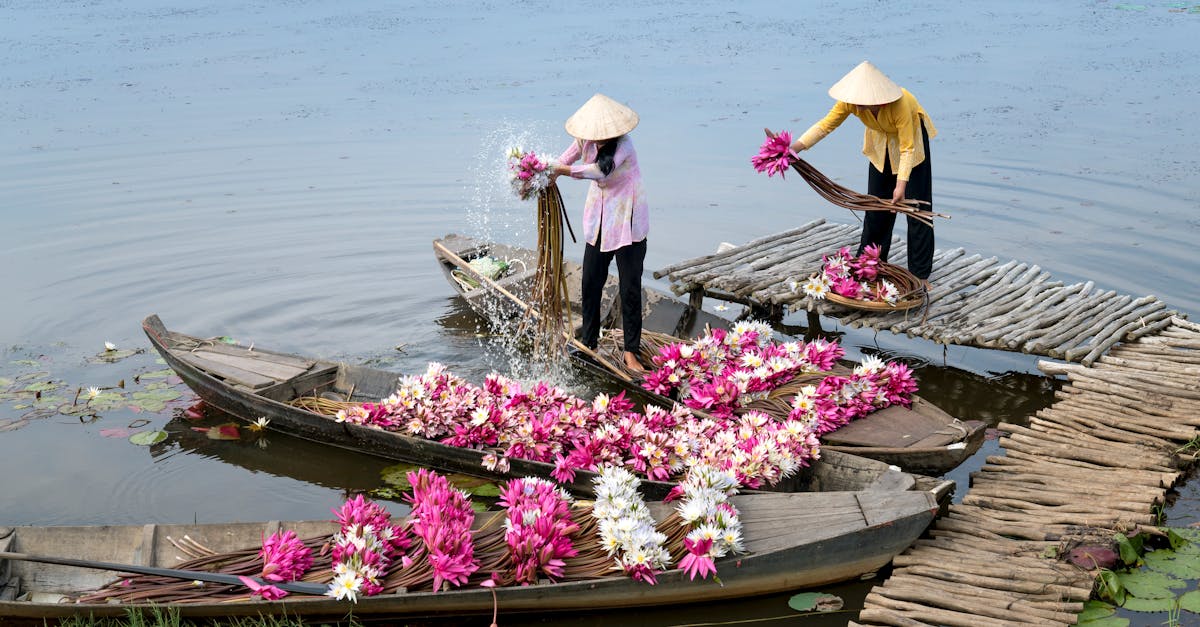
The database-first approach is a popular method used to create models from an existing database. It allows developers to leverage the power of Entity Framework, a widely adopted ORM (Object-Relational Mapping) tool, to interact with the database and perform CRUD operations easily.
One of the key advantages of using Entity Framework's database-first approach is that it simplifies the model creation process. Instead of designing the model from scratch, developers can generate the model classes, relationships, and mappings directly from the existing database structure. This saves time and effort, especially in scenarios where the database schema is already well-defined.
Getting Started
To begin implementing the database-first approach with Entity Framework, you will first need to install the Entity Framework package into your project. You can do this by running the following command in the NuGet Package Manager Console:
Install-Package EntityFramework
Once you have installed the Entity Framework package, you are ready to create your model from the database.
Generating the Model
Entity Framework provides various methods to generate the model classes. One of the commonly used approaches is the "Reverse Engineering" technique, which involves using the Entity Framework Power Tools or the EF Core Command-line Interface (CLI).
If you are using Visual Studio, you can install the Entity Framework Power Tools extension and follow these steps:
- Right-click on the project in Solution Explorer and select "Entity Framework" > "Reverse Engineer Code First..."
- Specify the database connection details and choose the desired tables/views to generate the model from.
- Review the generated model classes and customize them if necessary.
Alternatively, if you prefer to use the EF Core CLI, you can execute the following command in the terminal:
dotnet ef dbcontext scaffold "YourConnectionString" Microsoft.EntityFrameworkCore.SqlServer -o YourOutputFolder --context YourDbContextName
Replace "YourConnectionString" with the actual connection string to your database, "YourOutputFolder" with the desired output folder for the generated model classes, and "YourDbContextName" with the name you want to assign to the generated DbContext class.
Working with the Model
Once the model is generated, you can start using it to interact with the database. Entity Framework provides a rich set of APIs and features that simplify the data access layer implementation.
For example, you can use LINQ (Language Integrated Query) to query the database and retrieve data:
using (var context = new YourDbContextName())
{
var customers = context.Customers.Where(c => c.Age > 18).ToList();
}
You can also perform CRUD operations on the entities:
using (var context = new YourDbContextName())
{
var customer = new Customer
{
Name = "John Doe",
Age = 25,
Email = "johndoe@example.com"
};
context.Customers.Add(customer);
context.SaveChanges();
}
Remember to dispose of the DbContext after you are done with it, either by calling the Dispose()
method or using the using
statement, as shown in the examples above.
Conclusion
The database-first approach with Entity Framework is a powerful tool that simplifies the process of creating models from existing databases. It allows developers to quickly generate model classes and mappings, reducing the amount of manual effort required. By leveraging Entity Framework's rich feature set, developers can easily interact with the database and perform CRUD operations efficiently.
Whether you are building a new application or integrating an existing database into your project, the database-first approach with Entity Framework is worth considering. It provides a robust and convenient way to implement the data access layer and accelerates the development process.
Comments:
Thank you all for reading my article on enhancing the Database-First Approach in Entity Framework with ChatGPT! I'm excited to discuss this powerful combination further.
Great article, Cantrina! Combining the Database-First approach with ChatGPT can indeed simplify development. It's amazing how AI can assist in streamlining the process.
Thank you, Daniel! AI has opened up new possibilities, and leveraging ChatGPT in database development is definitely an exciting advancement.
I enjoyed reading your article, Cantrina! The Database-First approach is already great, but adding ChatGPT's capabilities makes it even better. It helps automate and simplify various tasks.
Absolutely, Emily! ChatGPT can assist in generating code, writing queries, and much more. It's a valuable tool for developers working with Entity Framework.
This combination sounds promising! Cantrina, have you personally used ChatGPT in your database development projects?
Yes, Jessica! I have utilized ChatGPT in some of my recent projects, and it has been incredibly helpful in speeding up the development process while maintaining code quality.
Could you provide some examples of how developers can benefit from integrating ChatGPT with Entity Framework's Database-First approach?
Certainly, Robert! ChatGPT can generate code snippets based on requirements, assist in writing LINQ queries, help with database schema design decisions, and provide suggestions for optimizing performance.
I'm curious about the learning curve involved in getting started with ChatGPT. Is it easy to use for developers with no prior AI experience?
Good question, Rachel! OpenAI has made efforts to improve usability, and while some AI knowledge can be beneficial, developers with no prior AI experience can still use ChatGPT effectively after familiarizing themselves with the documentation and APIs.
I'm concerned about the accuracy of generated code by ChatGPT. Can it reliably produce high-quality code without flaws?
Valid concern, David. While ChatGPT can assist in generating code, it's important to review and validate it before usage. OpenAI is continuously improving the models, but human review and quality checks remain an essential part of the development process.
The combination of AI and database development sounds intriguing. Can you share any real-world examples where ChatGPT has significantly sped up development tasks?
Definitely, Alex! ChatGPT has helped me automate the generation of repetitive code snippets, navigate complex database schemas, and even assisted in troubleshooting performance issues. It has saved me considerable time and effort throughout various projects.
I'm interested in security considerations when using ChatGPT during development. Are there any risks or precautions to be aware of?
Great point, Sophia! When using ChatGPT, developers should avoid sharing sensitive information or proprietary code. Additionally, it's essential to review and validate the output generated by ChatGPT to ensure security and overall project integrity.
Can ChatGPT assist in database migrations or schema updates as well?
Indeed, Daniel! ChatGPT can provide recommendations for database migrations and schema updates, helping developers plan and execute these tasks more efficiently.
Do you think the use of ChatGPT in the Database-First approach will impact the demand for manual database administrators and developers?
A valid concern, Michael. While ChatGPT can automate certain tasks and simplify development, manual database administrators and developers are still essential for tasks that require human decision-making, project management, and business-specific expertise.
Are there any limitations to be aware of when using ChatGPT in conjunction with Entity Framework?
Absolutely, Olivia! ChatGPT's responses are based on the provided context, but it may not have a complete understanding of the specific project requirements. Also, the quality and accuracy of suggestions can vary, requiring human review.
What would you recommend to developers who want to give ChatGPT a try in their projects?
Good question, Emily! I recommend starting with small experiments or non-critical parts of the project to get familiar with ChatGPT's capabilities. Gradually expand its usage based on the quality and accuracy of suggestions.
Are there any specific best practices or guidelines to follow when integrating ChatGPT with Entity Framework?
Certainly, Jessica! It's crucial to validate the generated code, customize outputs as per project requirements, and establish a feedback loop with ChatGPT to refine and improve its suggestions over time.
Regarding performance optimization, can ChatGPT provide insights or recommendations specific to optimizing Entity Framework queries?
Yes, Robert! ChatGPT can analyze query patterns, suggest indexing strategies, advise on eager or lazy loading, and recommend optimizations depending on the project's needs. It can greatly help in optimizing Entity Framework queries.
How does ChatGPT handle different database providers and versions? Is it limited to specific setups?
ChatGPT's capabilities are not limited to specific setups, Rachel. While it can provide general guidance and suggestions, it's recommended to validate the compatibility and specifics of the database provider and version being used in your project.
Considering the evolving nature of AI, do you anticipate any future enhancements or features that would further enhance the combination of ChatGPT and the Database-First approach?
Absolutely, David! Continued advancements in AI could improve code generation accuracy, expand the range of database-related suggestions, and also help in project documentation, data modeling, and data validation tasks.
What are the resource requirements for integrating ChatGPT with Entity Framework? Do developers need powerful hardware or extensive computational resources?
Good question, Alex! ChatGPT can be used through OpenAI's API, reducing the burden on local hardware. However, depending on the size and complexity of the project, developers might benefit from sufficient computational resources for efficient integration.
ChatGPT seems like an excellent tool. Are there any recommended ways to provide feedback or suggestions to make it even better?
Absolutely, Daniel! OpenAI encourages developers to provide feedback on problematic model outputs, false positives/negatives, and other nuances via their feedback channels. This helps in refining and improving ChatGPT's capabilities for the developer community.
Do you have any tips for developers to effectively collaborate with ChatGPT during development, especially in a team setting?
Great question, Sophia! When collaborating, it's essential to actively review and discuss the code and suggestions generated by ChatGPT, share feedback within the team, and establish clear guidelines and expectations to ensure seamless integration with the development process.
Can ChatGPT be used effectively in legacy systems that already have extensive codebases and databases?
Certainly, Olivia! ChatGPT can be leveraged in legacy systems as well. It can assist in understanding and working with existing codebases and databases, providing suggestions, and helping in code and schema updates.
How does the use of ChatGPT impact the learning curve for developers new to Entity Framework?
ChatGPT can help reduce the learning curve for developers new to Entity Framework, Michael. It can provide guidance, suggest code snippets, and assist in understanding database interactions. However, it's important to supplement its usage with gaining fundamental knowledge of Entity Framework concepts.
Are there any notable differences in integrating ChatGPT with Entity Framework in different programming languages?
Good question, Jessica! While the fundamental integration concepts remain similar across programming languages, there might be language-specific considerations. It's recommended to consult the programming language's documentation and explore language-specific libraries or frameworks that complement the integration.
Do you foresee any challenges in using ChatGPT for teams located in different time zones?
Time zone differences can pose challenges in real-time collaboration, David. However, asynchronous communication channels, shared documentation, and periodic sync-ups can help bridge the gap and ensure effective collaboration despite the time zone disparities.
Can ChatGPT be utilized in both small-scale and large-scale database projects?
Absolutely, Rachel! ChatGPT's benefits can be realized in both small-scale and large-scale database projects. Its assistance in code generation, query writing, optimizations, and other aspects can bring significant value regardless of the project's size.
Thank you, Cantrina, for providing valuable insights into enhancing the Database-First approach with ChatGPT. I can definitely see the potential benefits in our future projects.