Enhancing User Experience: Harnessing the Power of ChatGPT in jQuery Mobile's Selectmenu Widget
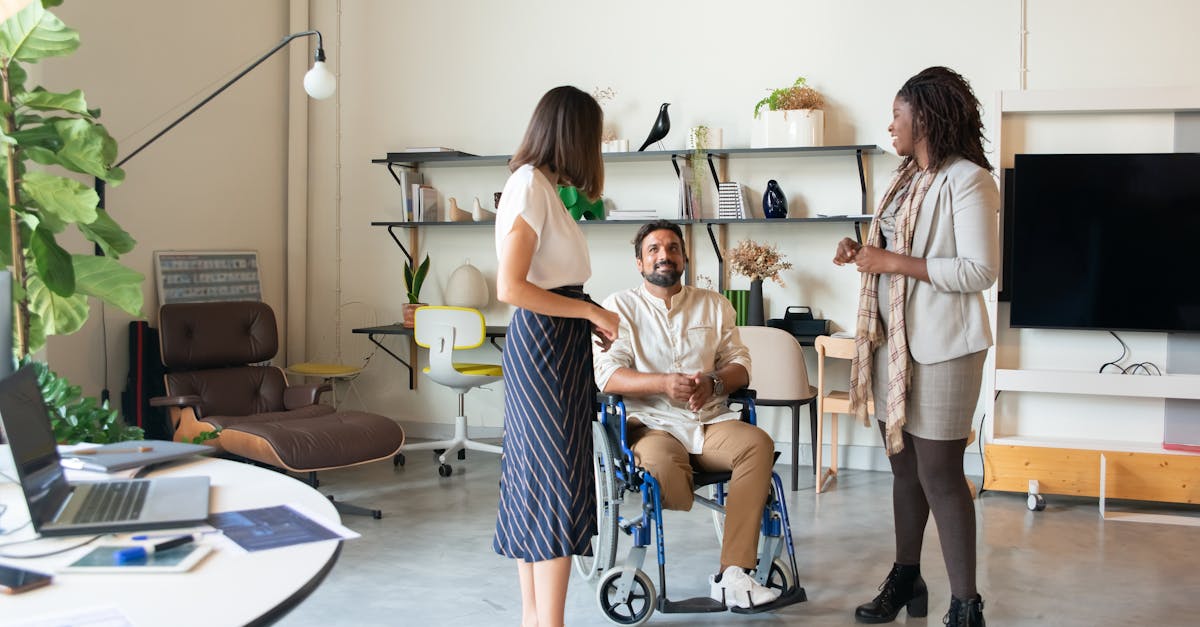
jQuery Mobile is a powerful JavaScript framework that allows developers to build mobile-friendly web applications with ease. One of its useful widgets is the Selectmenu widget, which provides a simple and customizable dropdown menu for selecting options.
In this article, we will explore how ChatGPT-4, the latest version of the conversational AI model, can guide users on how to use the Selectmenu widget in jQuery Mobile.
ChatGPT-4: Your Guide to Selectmenu Widget
ChatGPT-4 is designed to offer real-time assistance and support by simulating human-like conversations with users. It has been trained on a vast amount of data, including coding-related information, making it an excellent resource for developers.
Let's dive into the usage of the Selectmenu widget with the help of ChatGPT-4:
Step 1: Including jQuery Mobile
To begin, make sure you have included the jQuery Mobile library in your HTML file. You can either download it locally or use a CDN (Content Delivery Network) to include it.
<head>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<link href="https://code.jquery.com/mobile/1.5.0-alpha.1/jquery.mobile-1.5.0-alpha.1.min.css">
<script src="https://code.jquery.com/mobile/1.5.0-alpha.1/jquery.mobile-1.5.0-alpha.1.min.js"></script>
</head>
Step 2: Creating a Selectmenu
Next, you need to create a select menu element using the appropriate HTML markup. The select menu should have an ID for easy manipulation.
<select >
<option >Option 1</option>
<option >Option 2</option>
<option >Option 3</option>
<option >Option 4</option>
</select>
Step 3: Enhancing the Selectmenu
To transform the standard select menu into a jQuery Mobile selectmenu, you need to initialize it using JavaScript.
This is done by calling the .selectmenu()
method on the select menu.
<script>
$(document).ready(function() {
$('#selectmenu').selectmenu();
});
</script>
Step 4: Customizing the Selectmenu
The Selectmenu widget in jQuery Mobile provides various options to customize its appearance and behavior. These
options can be configured by passing an object with key-value pairs to the .selectmenu()
method during
initialization.
<script>
$(document).ready(function() {
$('#selectmenu').selectmenu({
theme: "a",
icon: "arrow-r",
hidePlaceholderMenuItems: false
});
});
</script>
Step 5: Handling Selectmenu Events
You can also handle events associated with the Selectmenu widget. For example, you might want to perform certain actions when a user selects an option or when the select menu value changes.
<script>
$(document).ready(function() {
$('#selectmenu').selectmenu({
change: function(event, data) {
console.log("Selected option: " + data.item.value);
}
});
});
</script>
Conclusion
The Selectmenu widget in jQuery Mobile is a versatile tool for creating dropdown menus in mobile web applications. With the guidance of ChatGPT-4, you can quickly grasp how to use this widget and make the most out of its features.
Remember to always refer to the official jQuery Mobile documentation for more detailed information on the Selectmenu widget and other available options.
Happy coding!
Comments:
Thank you all for your interest in my article! I'm excited to discuss further about enhancing user experience with ChatGPT and jQuery Mobile's Selectmenu Widget.
Great article, Bill! I've always been a fan of jQuery Mobile, and combining it with ChatGPT sounds like a fantastic way to improve user experience.
I agree, Erica! The power of ChatGPT can really take the user experience to the next level. Looking forward to trying it out.
Bill, your article is very insightful. I'm curious though, how easy is it to integrate ChatGPT into the Selectmenu Widget? Any challenges you encountered?
That's a good question, Liam. Integrating ChatGPT into the Selectmenu Widget requires some additional coding, but the process is well-documented. I'll be happy to share some resources if you're interested!
Thanks for the reply, Bill! I'd definitely appreciate some resources to get started. Looking forward to experimenting with it.
This article couldn't have come at a better time! I was just about to start working on improving the user experience of a web app using jQuery Mobile. Thanks for the inspiration, Bill!
You're welcome, Rachel! I'm glad the article could help you. If you have any specific questions or need guidance while working on your web app, feel free to ask.
I have been using jQuery Mobile for a while now, and it's great to see new ways of enhancing it. Can't wait to see the combination with ChatGPT in action!
I'm interested in knowing how ChatGPT can handle complex user interactions and dynamically adapt to different scenarios in the Selectmenu Widget.
Good point, Nathan. ChatGPT is designed to handle a wide range of user interactions by using a combination of pre-training and fine-tuning techniques. It can adapt to different scenarios within the Selectmenu Widget based on the training data and the given user input.
I'm concerned about potential limitations in terms of language understanding. Can ChatGPT accurately interpret user commands and anticipate their needs in real-time?
Language understanding is indeed a vital aspect, Daniel. While ChatGPT has shown impressive capabilities, it may still encounter challenges in accurately interpreting complex commands and anticipating user needs. It's crucial to provide clear instructions and conduct thorough testing during the implementation phase.
Thanks, Bill! I'll keep that in mind. It's important to set realistic expectations and iterate as needed.
Bill, could you share some use cases where the combination of ChatGPT and jQuery Mobile's Selectmenu Widget has significantly improved user experience?
Absolutely, Sara! One notable use case is in e-commerce applications where users can have conversational interactions while selecting products or customizing options. It adds a natural and engaging touch to the user experience, resulting in increased satisfaction and potentially higher conversion rates.
That sounds intriguing, Bill! I can see various industries benefiting from such an approach. It opens up possibilities for interactive surveys, guided questionnaires, and more.
Are there any potential security concerns when using ChatGPT in tandem with the Selectmenu Widget? How can we ensure user data is protected?
Security is crucial, Oliver. When integrating ChatGPT, it's essential to follow standard security practices, such as input sanitization, data encryption, and user authentication. By properly implementing these measures, user data can be effectively protected.
Bill, could you recommend any specific security resources or best practices to ensure a robust implementation?
Certainly, Sophia! Here are a few resources to get you started: - OWASP (Open Web Application Security Project) provides a wealth of information on web application security best practices. - The jQuery Mobile documentation includes security-related guidelines specific to the framework. - The ChatGPT API documentation provides guidance on securing the communication channels between your web app and the ChatGPT service.
Thank you, Bill! It's essential to prioritize security when dealing with user data. Your recommendations will definitely help us ensure a robust implementation.
Bill, do you have any tips on optimizing performance when using ChatGPT with the Selectmenu Widget? Are there any potential bottlenecks to watch out for?
Optimizing performance is indeed important, David. When using ChatGPT, consider factors like response time and server load. Caching frequently used responses, using server-side processing for intensive tasks, and setting appropriate timeouts can help manage performance. It's also essential to monitor and analyze your application's performance to identify and address any bottlenecks that may arise.
Thanks for the tips, Bill! Performance optimization is often overlooked but can significantly impact the user experience. I'll keep these suggestions in mind while working with ChatGPT and the Selectmenu Widget.
Bill, I'm curious about the scalability of ChatGPT in the context of the Selectmenu Widget. Can it handle a large number of concurrent users without compromising performance?
Scalability is an important aspect, Grace. ChatGPT can handle a considerable number of concurrent users, but it's crucial to architect your system appropriately. Employing load balancing techniques, utilizing serverless computing, and optimizing resource allocation can help accommodate a large user base without compromising performance.
That's reassuring to hear, Bill. Scalability is often a concern when incorporating new technologies. Your recommendations offer valuable insights for managing high user loads.
I'm interested in the training aspect of ChatGPT. How can we ensure that the ChatGPT model is well-trained and provides accurate responses in the context of the Selectmenu Widget?
Training the ChatGPT model requires a large dataset of conversations that cover various scenarios in the context of the Selectmenu Widget. Validating and curating the training data is crucial to ensure accurate responses. Additionally, fine-tuning the model on specific prompts and iteratively evaluating its performance can further improve its accuracy.
Bill, I would like to know more about the potential limitations or challenges one might encounter when implementing the ChatGPT-Selectmenu combination.
Certainly, Laura! When implementing the ChatGPT-Selectmenu combination, some potential challenges include: - Understanding complex user queries and intents accurately - Handling unexpected user inputs or out-of-context requests - Balancing user guidance and customization options Overall, thorough testing, user feedback collection, and continuous refinements are essential for overcoming these challenges.
Thanks for addressing the challenges, Bill! It's important to be aware of potential limitations and plan accordingly for a successful implementation.
How would you recommend organizations go about user training and onboarding when utilizing the ChatGPT-Selectmenu approach?
User training and onboarding are crucial for ensuring a smooth user experience, Sophie. It's beneficial to provide interactive tutorials, tooltips, and clear documentation on how to interact with the ChatGPT-Selectmenu combination. Additionally, collecting user feedback during the initial stages and continuously refining the system based on user needs can contribute to a better onboarding experience.
Bill, what are the potential privacy concerns associated with using ChatGPT in conjunction with jQuery Mobile's Selectmenu Widget?
Privacy is indeed a concern, Emma. When using ChatGPT, it's crucial to handle user data with care. Clearly communicate your data handling practices to your users, obtain explicit consent if needed, and follow relevant privacy regulations. By prioritizing user privacy, organizations can maintain trust and ensure compliance with applicable laws.
Thanks for highlighting the privacy aspect, Bill. Respecting users' privacy and providing transparent data handling practices is essential for maintaining a trustworthy relationship.
Bill, in your opinion, what exciting advancements can we expect in the future regarding user experience enhancement with ChatGPT and frameworks like jQuery Mobile's Selectmenu?
The future looks promising, Jason! We can expect further advancements in natural language understanding, context-awareness, and personalization. This will allow for more sophisticated interactions, dynamic adaptations, and tailored experiences using ChatGPT in conjunction with frameworks like jQuery Mobile's Selectmenu. Additionally, as ChatGPT continues to evolve, we can look forward to improved training methodologies and accessibility.
Bill, thank you for sharing your expertise and insights through this article. It has sparked my enthusiasm to explore the combination of ChatGPT and jQuery Mobile's Selectmenu for enhancing user experiences!
You're very welcome, Isabella! I'm thrilled to hear that the article has inspired you. Feel free to reach out if you have any further questions or require assistance during your exploration. Best of luck!
Bill, as someone new to the field, I appreciate how well you explained the concept and benefits. This article has motivated me to delve deeper into user experience design!
I'm glad to hear that, Emma! User experience design is an exciting and ever-evolving field. If you have any specific questions or need further guidance while delving into it, feel free to ask. Enjoy exploring!
Bill, excellent article! It's amazing to see how artificial intelligence can be harnessed to improve user experiences. I look forward to experimenting with the ChatGPT-Selectmenu combination.
Thank you, Aaron! The combination of artificial intelligence and user experience enhancement offers a wide range of possibilities. I'm sure you'll enjoy the experimentation process. Feel free to share your experiences and any insights you gather along the way!
Bill, your article has given me some great ideas on how to enhance our existing web applications with ChatGPT and jQuery Mobile's Selectmenu. It's exciting to think about the potential improvements we can achieve!
I'm thrilled to hear that, Lucas! Incorporating ChatGPT and jQuery Mobile's Selectmenu into existing web applications can indeed bring exciting improvements. Don't hesitate to share your progress or any challenges you encounter. Good luck!
Bill, I'm impressed with how you've explained the concept in a concise and easily understandable manner. Your article has piqued my curiosity, and I'm eager to explore the combination further.
Thank you, Hailey! I'm glad you found the article helpful. If you have any questions or need further information while exploring the combination of ChatGPT and jQuery Mobile's Selectmenu, feel free to ask. Enjoy your exploration!