Exploring ChatGPT for Enhanced Event Handling in Angular.js: Revolutionizing Interaction Development
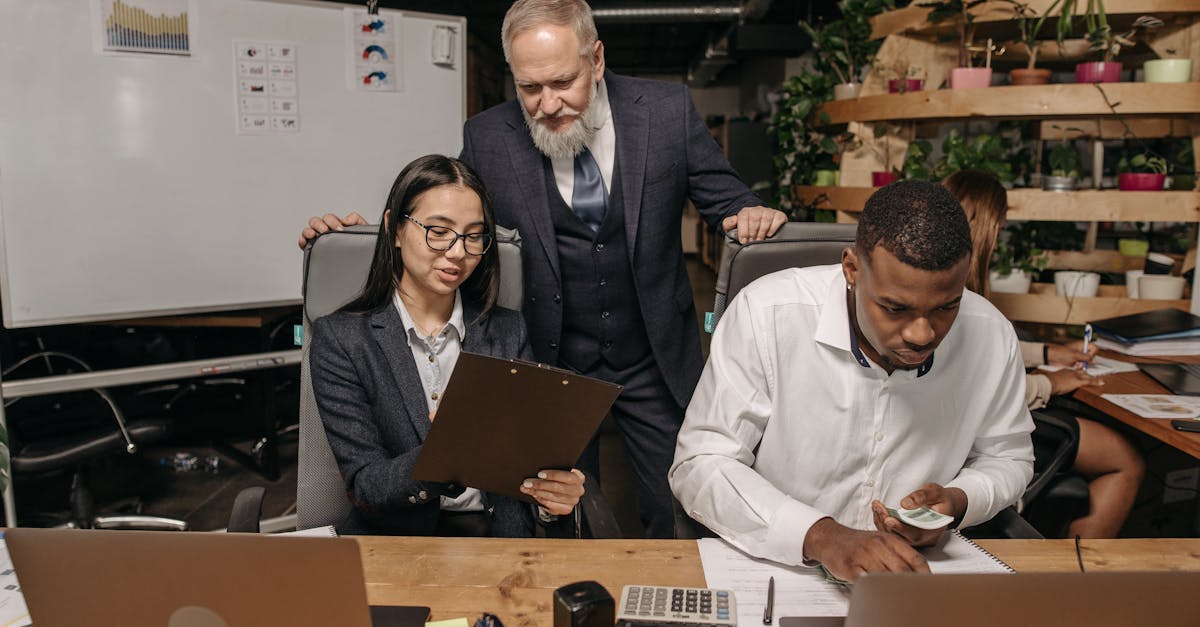
AngularJS is a powerful JavaScript framework that provides developers with a wide range of tools to build dynamic web applications. One of the key features of AngularJS is its event handling capabilities, which allow developers to handle user and system events seamlessly. In this article, we will explore how to effectively handle events in AngularJS applications.
User Events
AngularJS provides various directives to handle user events such as clicks, key press, mouse movements, etc. The most commonly used directive for handling user events is ng-click
, which is used to trigger a function when the element is clicked.
<button ng->Click Me</button>
In the above example, the ng-click
directive is bound to a function called onClick()
. When the button is clicked, the function will be executed. You can define the onClick()
function in your AngularJS controller to perform any desired actions.
Similarly, AngularJS provides other directives such as ng-keypress
, ng-mousemove
, ng-change
, etc., to handle various user events in an AngularJS application. These directives can be applied to different HTML elements like buttons, input fields, checkboxes, etc., allowing you to customize the behavior of your application based on user interactions.
System Events
In addition to user events, AngularJS also supports system events such as route changes, initialization, HTTP responses, etc. These events can be handled using various AngularJS services and events provided by the framework.
For example, you can use the $rootScope
service to listen for route changes in your AngularJS application:
angular.module('myApp', [])
.run(function($rootScope) {
$rootScope.$on('$routeChangeStart', function(event, next, current) {
// Handle route change logic here
});
});
In the above example, the $rootScope.$on()
method is used to listen for the $routeChangeStart
event. When this event is triggered, the provided callback function will be executed. Inside the callback function, you can write custom logic to handle the route change.
Similarly, you can use other services and events provided by AngularJS to handle system events such as HTTP responses, application initialization, etc. These events can be extremely useful in scenarios where you need to perform certain actions based on specific system events.
Conclusion
AngularJS offers powerful event handling capabilities that allow developers to handle both user and system events effortlessly. By leveraging AngularJS directives, services, and events, developers can create dynamic web applications that respond to user interactions and system events effectively. Whether it's capturing user clicks or performing actions based on route changes, AngularJS provides a robust event handling mechanism that simplifies the development of rich, interactive applications.
Comments:
Thank you all for taking the time to read my article on Exploring ChatGPT for Enhanced Event Handling in Angular.js: Revolutionizing Interaction Development. I'm excited to hear your thoughts and engage in a discussion!
Great article, Rowena! The potential of ChatGPT for enhancing event handling in Angular.js is intriguing. Looking forward to seeing how this revolutionizes interaction development.
I'm excited about the potential of ChatGPT too, Samantha! Do you think this approach can help improve user engagement and reduce bounce rates in Angular.js applications?
Absolutely, Victor! By incorporating intelligent chatbots powered by ChatGPT, we can offer personalized and interactive experiences to users, keeping them engaged and addressing their queries effectively. This can undoubtedly help reduce bounce rates and boost overall user satisfaction.
Samantha, do you have any specific examples or use cases where ChatGPT has already been successfully implemented in Angular.js?
Indeed, Nathan! One example is e-commerce applications utilizing ChatGPT for interactive product recommendations based on user preferences and requirements. Another use case is in customer support portals, where ChatGPT-powered chatbots can handle common queries, provide troubleshooting assistance, and escalate complex issues when necessary.
Samantha, do you foresee any challenges in convincing stakeholders to adopt ChatGPT for event handling in Angular.js apps, especially in industries with strict compliance requirements?
A valid concern, Sophia. In industries with strict compliance requirements, it may be challenging to convince stakeholders due to data privacy and security concerns. Conducting thorough risk assessments, implementing necessary encryption and access control measures, and highlighting the potential benefits and use cases specific to the industry can help mitigate concerns and facilitate adoption.
Samantha and Rowena, can you share any insights or limitations regarding user training for ChatGPT in Angular.js apps?
Certainly, Daniel! Training ChatGPT requires a substantial amount of high-quality conversational data to ensure effective responses. Generating diverse and realistic user inputs through techniques like data augmentation and active learning can help improve the model's performance. However, it's important to note that the model may still produce occasional incorrect or nonsensical responses due to inherent limitations.
I agree, Samantha. The concept of leveraging ChatGPT for better event handling in Angular.js seems promising. Rowena, can you provide some concrete examples of how this technology can be applied in practice?
Certainly, David! ChatGPT can be utilized to create intelligent chatbots within Angular.js applications, allowing for more dynamic and engaging interactions. For example, a chatbot powered by ChatGPT can handle user queries and guide them through a series of steps to complete a form efficiently. It can also be used for contextual help, providing real-time assistance based on user inputs.
That sounds amazing, Rowena! The potential of ChatGPT to enhance user experience is evident. How does it handle complex business logic and edge cases in event handling scenarios?
Good question, Emily! While ChatGPT is proficient in understanding and responding to various user inputs, including complex queries, it's important to consider proper error handling and validation mechanisms when integrating it into event handling workflows. Fallback options and error messages can be implemented to guide users in case of unexpected inputs or errors.
I see how ChatGPT can enhance straightforward interactions, but what about more nuanced conversations? Can it maintain context in a multi-turn conversation within an Angular.js app?
Excellent question, Adam! Yes, ChatGPT is designed to maintain context and handle multi-turn conversations effectively. By utilizing techniques like conversation history tracking and proper input formatting, the model can understand the conversation flow and generate relevant responses in an ongoing interaction.
That's fascinating, Rowena! Can ChatGPT also handle multiple languages within the scope of an Angular.js application?
Certainly, Marie! ChatGPT has shown impressive capabilities in multilingual understanding and generation. It can be leveraged to develop language-agnostic chatbots or support multiple languages within an Angular.js app through proper configuration and data pre-processing for different languages.
Impressive! Are there any limitations or challenges to consider when implementing ChatGPT in Angular.js applications?
Certainly, Liam! One crucial aspect to consider is data privacy and security, especially when handling sensitive user information within a chat interface. It's also essential to periodically evaluate and fine-tune the language models to ensure they align with expected behavior and avoid biases. Additionally, latency can be a concern when utilizing external APIs like ChatGPT.
Rowena, I'm curious about the potential scalability of ChatGPT. Can it handle a large number of concurrent users in an Angular.js app without significant performance issues?
Great question, Jonathan! While ChatGPT is powerful, handling a high volume of concurrent users can pose challenges. Scaling strategies, such as load balancing and distributed systems, should be considered to ensure optimal performance. Additionally, resource allocation and optimization techniques can be employed to enhance scalability.
Jonathan, what are the performance implications of utilizing ChatGPT for event handling in Angular.js apps, especially in terms of response time and server load?
Good question, Oliver! The performance implications depend on several factors, including the size and complexity of the chat model, the underlying infrastructure, and the concurrent user load. Caching frequently accessed responses, optimizing backend servers, and using techniques like response pagination can aid in managing server load and achieving acceptable response times.
Are there any specific Angular.js libraries or tools that you recommend for integrating ChatGPT effectively?
Absolutely, Beatrice! Some useful libraries for integrating ChatGPT in Angular.js applications include 'angular-chat-widget', 'ng-chat', and 'angular-ai-chat-bot'. These libraries provide convenient abstractions and components to streamline the integration process and enhance the overall user experience.
That's great, Rowena! I'm interested in exploring ChatGPT further. Are there any specific resources or tutorials you would recommend for getting started with this technology in Angular.js?
Definitely, Ella! You can check out the OpenAI documentation and blog posts on chat models to gain a better understanding of ChatGPT and its integration possibilities. Additionally, there are numerous online tutorials and GitHub repositories that provide step-by-step guides for implementing chatbots in Angular.js using ChatGPT as the underlying language model.
Rowena, how do you handle user intents and map them to appropriate actions in an Angular.js app utilizing ChatGPT?
Good question, Alexandra! Intent recognition plays a crucial role in mapping user queries to specific actions or responses. Techniques like natural language understanding (NLU), entity recognition, and intent classification can be employed in combination with ChatGPT to identify user intents and trigger appropriate event handling mechanisms in Angular.js applications.
Thank you for the library recommendations, Rowena! Are there any best practices for designing conversational interfaces with ChatGPT?
You're welcome, Alice! When designing conversational interfaces with ChatGPT, it's essential to keep the interactions natural and user-centric. Leveraging techniques like slot filling, providing helpful prompts, and acknowledging user inputs can enhance the conversational flow. Regular user testing and feedback collection also contribute to refining the interface and improving the overall user experience.
Privacy and bias are indeed important considerations, Rowena. Are there any guidelines or frameworks to help ensure ethical and unbiased use of ChatGPT in Angular.js apps?
Absolutely, Robert! OpenAI has provided guidelines and recommendations to address ethical concerns and mitigate biases in AI applications. The 'Responsible AI Practices' framework is a helpful resource that encourages transparency, fairness, and accountability when deploying AI models like ChatGPT. Adhering to such frameworks is essential for responsible and unbiased use of AI technologies.
Rowena, how do you handle potential biases in ChatGPT's responses, especially when it comes to sensitive topics or user demographics?
A critical aspect, Isabella! To address biases, it involves a combination of techniques like carefully curating training data, incorporating public input, and defining explicit guidelines during the fine-tuning process. OpenAI is actively researching and developing approaches to make ChatGPT configurable, empowering users to customize its behavior within certain ethical boundaries.
Thank you for the resources, Rowena! I'm looking forward to exploring ChatGPT in Angular.js apps further. Do you have any recommendations for evaluating the performance and effectiveness of a ChatGPT implementation?
You're welcome, Lily! Evaluating the performance and effectiveness of a ChatGPT implementation can involve metrics like response accuracy, user feedback ratings, and response time analytics. Conducting usability testing, gathering user satisfaction surveys, and monitoring server logs can provide valuable insights to refine the implementation and maximize its benefits.
Regarding user training, how frequently should the ChatGPT model be retrained to stay up to date with evolving user needs and industry trends?
An excellent question, Lily! The frequency of retraining depends on various factors like the rate of user interactions, the impact of new requirements on conversational flows, and the availability of updated or additional data. Regular evaluation of the model's performance and user feedback can help determine the appropriate retraining intervals for Angular.js applications utilizing ChatGPT.
Are there any specific strategies or optimizations you recommend to improve response times when using ChatGPT in Angular.js apps?
Certainly, Hannah! Some strategies to enhance response times include employing caching mechanisms for commonly accessed responses, utilizing efficient storage systems for chat history, and optimizing network communication through techniques like compression and batching. Load testing and performance monitoring can help identify bottlenecks and further optimize the system.
Can you shed some light on the computational resources required to deploy ChatGPT for event handling in Angular.js apps?
Certainly, Michael! The computational resources required depend on various factors such as the model size, the number of concurrent users, and the desired response times. Larger models may require more powerful hardware configurations, and techniques like model parallelism or distributed systems can be employed to efficiently utilize resources. Conducting load testing and resource profiling can help determine the necessary computational infrastructure.
In terms of cost, how does the deployment of ChatGPT and Angular.js interact? Are there any specific cost optimization strategies?
A valid concern, Sarah! The cost of deploying ChatGPT in Angular.js apps depends on factors like computational resources, the number of API calls, and the pricing structure of the AI service providers. Cost optimization strategies include intelligent caching, response pagination, and resource-aware load balancing. Understanding usage patterns, monitoring costs, and considering options like serverless deployments can further optimize the deployment costs.
Are there any specific cloud providers or platforms that are recommended for deploying and scaling Angular.js apps with integrated ChatGPT?
Certainly, Leo! Some popular cloud providers that offer platform-as-a-service (PaaS) solutions for deploying and scaling Angular.js apps, including those integrated with ChatGPT, include AWS, Google Cloud, and Azure. These platforms provide a range of services like serverless functions, scalable computing resources, and managed databases, facilitating seamless deployment and scalability for such applications.
In addition to user feedback, are there any other AI-specific metrics or evaluation techniques that can be applied to assess the quality of a ChatGPT-based event handling system in Angular.js?
Absolutely, Ethan! Some AI-specific metrics include perplexity scores to evaluate the model's understanding and generation capabilities, diversity measures to assess response variation, and context coherence scores to gauge the system's proficiency in maintaining contextual conversations. By combining these metrics with user feedback, it's possible to gain a comprehensive understanding of the system's quality and make necessary improvements.