Exploring Design Patterns for ExtJS: Leveraging ChatGPT for Enhanced Development
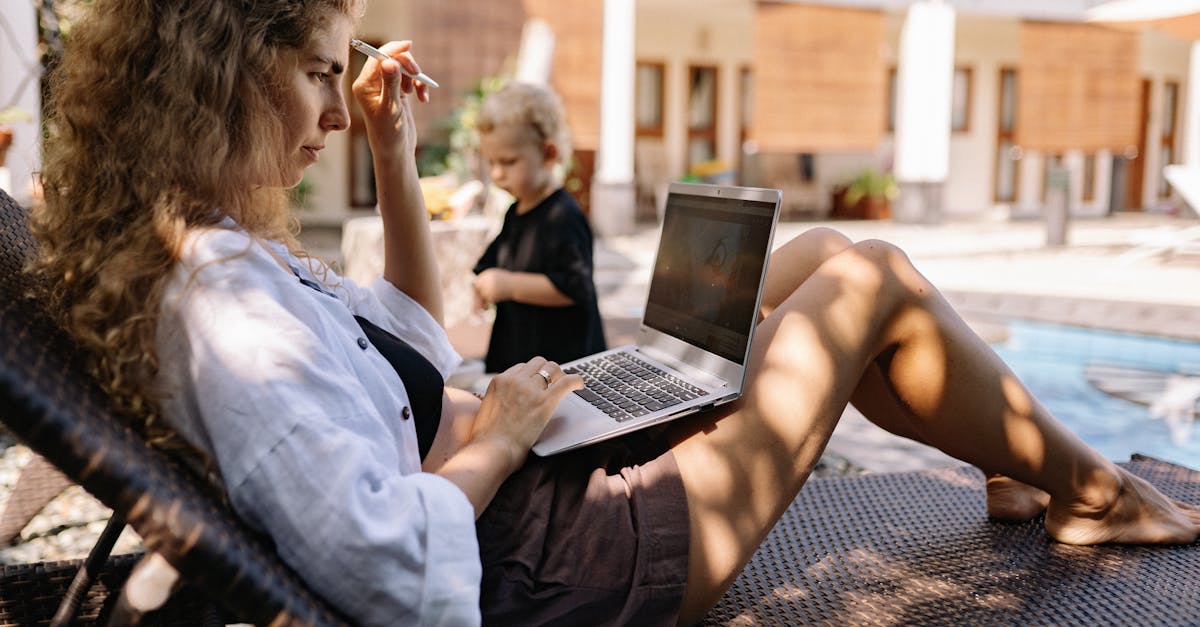
ExtJS is a comprehensive JavaScript framework used for building enterprise-grade web applications with rich user interfaces. In order to ensure maintainable and scalable code, it is important to follow established design patterns. Design patterns provide reusable solutions to commonly occurring problems and help improve the architectural structure of the application.
The Benefits of Design Patterns
Using design patterns in ExtJS has several advantages:
- Code Reusability: Design patterns promote code reusability by providing predefined solutions that can be applied to different scenarios without reinventing the wheel.
- Maintainability: By following established design patterns, the codebase becomes more structured and easier to understand, making it simpler to maintain and debug.
- Scalability: Design patterns ensure that the application's codebase can scale with growing requirements and complexity, allowing for easier integration of new features.
- Consistency: Design patterns enforce a consistent way of writing code, making it easier for multiple developers to collaborate and understand each other's work.
Design Patterns in ExtJS
ExtJS utilizes various design patterns to improve the architecture of its applications. Some commonly used design patterns in ExtJS include:
- MVC (Model-View-Controller): The MVC pattern separates the application into three main components - the model, view, and controller. This pattern helps in achieving separation of concerns and ensures a modular and scalable code structure.
- Singleton: The Singleton pattern restricts the instantiation of a class to a single instance. It allows easy access to the instance throughout the application and is commonly used for managing global resources or configuration settings.
- Observer: The Observer pattern allows objects to subscribe and receive notifications when changes occur in other objects. It provides a loosely coupled mechanism for communication between different components of the application.
- Facade: The Facade pattern provides a simplified interface to a complex system of components, making it easier to use and understand. It hides the complexity of the underlying subsystem and provides a unified interface for interacting with it.
- Factory: The Factory pattern provides an interface for creating objects without specifying their concrete classes. It allows the application to be more flexible by decoupling the object creation logic from the client code.
Implementing Design Patterns in ExtJS
The ExtJS framework provides built-in support for implementing various design patterns. The ExtJS documentation includes detailed explanations and examples of how to apply these patterns in your application.
For example, to implement the MVC pattern in ExtJS, you can define your models, views, and controllers using the ExtJS classes provided:
Ext.define('MyApp.model.User', { ... });
Ext.define('MyApp.view.UserList', { ... });
Ext.define('MyApp.controller.UserController', { ... });
Similarly, the Singleton pattern can be implemented using ExtJS's global namespace or application instance:
MyApp.singletonConfig = { ... };
The ExtJS documentation provides comprehensive examples and guidelines for implementing all the supported design patterns. It is highly recommended to refer to the official documentation for detailed explanations and best practices.
Conclusion
Incorporating design patterns in your ExtJS applications can significantly improve their architecture, maintainability, and scalability. The AI assistant is capable of providing detailed explanations and implementations of various design patterns applicable in ExtJS. Make sure to utilize the available resources and always follow best practices when using design patterns in ExtJS development.
Comments:
Thank you all for joining the discussion! I'm excited to hear your thoughts on exploring design patterns for ExtJS using ChatGPT.
Great article, Dale! I've been using ExtJS for a while now, and leveraging design patterns has definitely improved my development process. Looking forward to learning more from your insights!
Thank you, Alexandra! I'm glad to hear that design patterns have been beneficial to you. Is there any particular pattern you have found useful in your ExtJS projects?
I'm relatively new to ExtJS, but this article has inspired me to dive deeper into design patterns. It seems they can bring a lot of structure and maintainability to the codebase. Any recommendations for beginners?
Welcome, Michael! Design patterns can indeed enhance code maintainability. As a beginner, I recommend starting with the MVC (Model-View-Controller) pattern. It helps separate concerns and promotes code reusability.
Great article, Dale! I've been using ExtJS for a while now, and leveraging design patterns has definitely improved my development process. Looking forward to learning more from your insights!
Thank you, Adam! I'm glad to hear that design patterns have been beneficial to you. Is there any particular pattern you have found useful in your ExtJS projects?
The article provides a great practical approach for leveraging ChatGPT in ExtJS development. It would be interesting to see some code examples demonstrating the implementation of different design patterns.
Thank you, Linda! I appreciate your feedback. I'll definitely consider including some code examples in future articles to showcase the implementation of different design patterns in ExtJS.
This article expands on the versatility of ExtJS by combining it with ChatGPT to enhance development. It opens up a new realm of possibilities for building interactive and intelligent applications. Exciting stuff!
Absolutely, Nathan! By integrating ChatGPT into ExtJS, developers can create more intelligent and interactive applications. It's an exciting journey to explore.
As a UX/UI designer, I find the combination of ExtJS and design patterns fascinating. It not only ensures a better structure for development but also allows for more effective collaboration between designers and developers.
You're absolutely right, Emma! The combination of ExtJS and design patterns creates a solid foundation for collaboration between designers and developers. It helps bridge the gap between the two disciplines.
I've been using ExtJS without paying much attention to design patterns. This article has enlightened me about their importance and the benefits they bring. Time to refactor some code!
That's great to hear, Oliver! Refactoring your code to incorporate design patterns can greatly improve its maintainability and extensibility. Best of luck with your refactoring efforts!
I've been using ExtJS for a while, and design patterns have been a game-changer for me. They simplify complex applications and make them more manageable. Can't wait to explore the potential of ChatGPT with ExtJS!
Absolutely, Sophia! Design patterns provide a structured approach to tackle complexity and make applications more manageable. Adding ChatGPT to your arsenal can open up even more possibilities. Enjoy exploring!
Excellent article, Dale! Design patterns are essential for developing scalable and maintainable ExtJS applications. They save a lot of time in the long run. Can't wait for your future articles!
Thank you for your kind words, Ethan! I'm thrilled to hear that you found the article helpful. Stay tuned for more articles on ExtJS and design patterns!
I've recently started using ExtJS, and I must say this article has given me insights into leveraging design patterns effectively. Looking forward to applying them in my projects.
That's great, Isabella! Design patterns can significantly enhance the structure and maintainability of your ExtJS projects. Don't hesitate to reach out if you have any questions during your application process.
As a front-end developer, I really appreciate the practical suggestions given in this article. It's refreshing to see a discussion on design patterns specifically tailored to ExtJS.
I'm glad you found the article valuable, Sophie! ExtJS has its own unique characteristics, and exploring design patterns tailored for it can significantly enhance your development experience.
ExtJS has always been my go-to framework, but I've never delved deep into design patterns. This article has sparked my curiosity, and I'll definitely be implementing them in my future projects.
I'm glad to hear that, Charlie! Design patterns can bring a whole new level of structure and elegance to your ExtJS projects. Feel free to ask if you need any guidance during your implementation.
As a software engineer, I've found that applying design patterns to ExtJS has made my code more organized and maintainable. It's a valuable practice for any ExtJS developer!
Exactly, Samantha! Design patterns promote code organization and maintainability, which are crucial aspects of developing successful ExtJS applications. Keep up the good work!
I've been using ExtJS for a few years, and this article has reminded me of the importance of design patterns. They bring consistency and scalability to the codebase, making it easier to onboard new team members.
Absolutely, Aiden! Design patterns contribute to code consistency and scalability, making it easier for new team members to understand and contribute to ExtJS projects. They're a valuable asset!
This article provides a fantastic overview of design patterns in ExtJS development. Looking forward to seeing more specific examples in your future articles, Dale!
Thank you, Emily! I'm glad you enjoyed the article. I'll definitely include more specific examples in my future articles to provide practical insights for ExtJS developers. Stay tuned!
I'm relatively new to ExtJS, but this article has given me a head start in leveraging design patterns. Excited to explore their effectiveness in improving my development workflow.
Welcome to ExtJS, Henry! Design patterns can accelerate your development workflow significantly. Don't hesitate to ask if you need any assistance along the way. Enjoy exploring!
As someone who's been using ExtJS for years, I appreciate the focus on design patterns. They provide a standardized approach to solving common problems and help maintain a clean codebase.
Absolutely, David! Design patterns act as proven solutions to common problems, saving developers time and effort. They are instrumental in maintaining a clean, robust ExtJS codebase.
I've encountered some difficulties in working with ExtJS in the past. I believe leveraging the right design patterns can alleviate those pain points. Looking forward to learning more!
I understand, Alexandra! Design patterns can provide solutions to common challenges faced while working with ExtJS. Hopefully, the insights shared in this article will help address some of those pain points.
I totally agree with the significance of design patterns in ExtJS development. They promote code reusability and make the codebase more flexible for future changes.
Exactly, Michael! Design patterns enable code reusability and flexibility, allowing ExtJS applications to adapt and evolve over time. They're a powerful tool in a developer's toolkit.
The practical application of ChatGPT in the discussion adds a unique perspective to ExtJS development. It unveils new possibilities and sparks creativity. Great article!
Thank you, Linda! Integrating ChatGPT with ExtJS indeed opens up exciting possibilities for development. Feel free to share your creative ideas or any specific areas where you think ChatGPT can make a significant impact!
Design patterns serve as a guide to creating well-structured ExtJS code. They enhance collaboration within a team and make the codebase more maintainable. Looking forward to your future articles, Dale!
Thank you for your kind words, Nathan! I couldn't agree more—design patterns encourage collaboration and improve code maintainability. Stay tuned for more insightful articles!
The combination of ExtJS with ChatGPT for enhanced development sounds intriguing. I'm excited to learn more about how they can work together effectively.
I'm glad to hear your interest, Emma! ExtJS and ChatGPT indeed have the potential to work hand-in-hand, bringing enhanced development experiences. Feel free to ask any specific questions you may have!
This article has convinced me to explore design patterns in my ExtJS projects. They seem to provide a structured approach to solving common challenges efficiently.
That's great to hear, Oliver! Design patterns can bring a new level of efficiency and structure to your ExtJS projects. Don't hesitate to reach out if you need any guidance during your exploration!
I appreciate the practical insights you've shared in this article, Dale. Design patterns can save developers from reinventing the wheel and simplify complex applications.
Thank you, Sophia! Design patterns indeed act as proven solutions to common problems, allowing developers to build on existing knowledge and simplify application complexity. Keep up the great work!
ExtJS is a powerful framework, and design patterns further amplify its capabilities. This article provides valuable insights into combining the two. Looking forward to more articles, Dale!
Thank you, Ethan! I'm thrilled to know that you found the insights valuable. Stay tuned for more articles exploring the synergies between design patterns and ExtJS. Your continued support means a lot!