How ChatGPT Enhances Software Development in ADO.NET: Revolutionizing the Way Developers Code
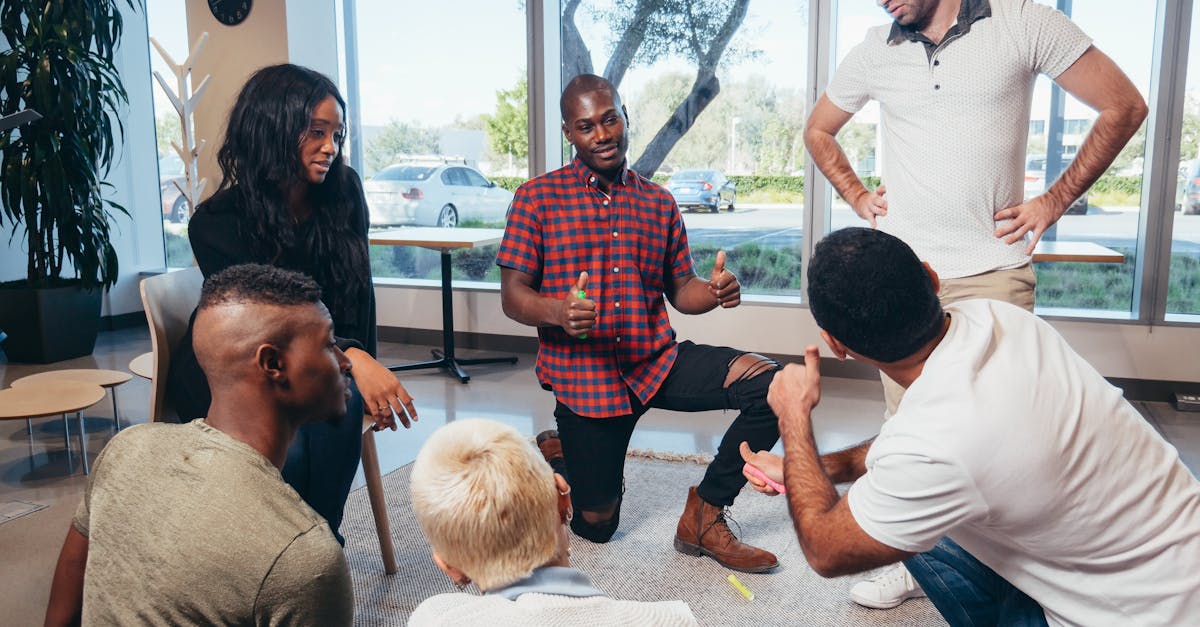
ADO.NET is a powerful technology that provides a set of data access services to developers working on software development projects. It is widely used for managing and accessing data from various databases in .NET applications. In this article, we will discuss some tips and best practices for using ADO.NET in software development.
1. Understanding ADO.NET Concepts
Before diving into development, it is essential to have a clear understanding of ADO.NET concepts. Familiarize yourself with terms like Data Providers, Connection Strings, DataSets, DataReaders, and more. A solid grasp of these concepts will enable you to make informed decisions and write efficient code.
2. Use Connection Pooling
Connection pooling is a fundamental feature of ADO.NET that helps improve the performance of your applications. By reusing connections from a pool, you can minimize the overhead of creating and closing connections repeatedly, resulting in better application responsiveness.
3. Parameterize Queries to Prevent SQL Injection
Never concatenate user input directly into SQL queries. Instead, use parameterized queries or stored procedures to prevent SQL injection attacks. Parameterized queries sanitize user input and ensure that it is treated as data rather than executable code, improving security and reducing the risk of malicious activities.
4. Handle Exceptions Gracefully
Be prepared for potential exceptions that may occur when working with ADO.NET. Proper error handling helps improve the stability and reliability of your applications. Use try-catch blocks to catch and handle exceptions, and implement appropriate error logging mechanisms to facilitate troubleshooting, debugging, and maintenance.
5. Leverage Asynchronous Programming
Utilize asynchronous programming techniques when interacting with databases through ADO.NET. Asynchronous operations enhance the scalability and responsiveness of your applications, as database queries can be executed in the background while the main thread remains available for other tasks, providing a better user experience.
6. Dispose of Resources
Always dispose of resources such as connections, commands, and data readers when they are no longer needed. By promptly releasing these resources, you prevent memory leaks and ensure efficient memory management. Use the using
statement or manually call the Dispose
method to clean up resources.
7. Use Stored Procedures
Consider using stored procedures for complex database queries. Stored procedures can improve performance by reducing the amount of data transmitted between the application and the database server. They also provide an extra layer of security by hiding the underlying database structure and preventing direct SQL access.
8. Monitor Performance
Regularly monitor the performance of your ADO.NET code. Use tools like Performance Counters to identify bottlenecks and optimize the performance of your data access layer. Analyze query execution times, connection usage, and other relevant metrics to ensure optimal performance of your software.
By following these tips and best practices, you can harness the full potential of ADO.NET in software development. ADO.NET provides a flexible and efficient means to work with databases, and utilizing it effectively will lead to robust and high-performing applications.
Remember to continuously update your knowledge of ADO.NET and stay informed about new features and enhancements. Embrace best practices, experiment, and learn from your experiences to become a proficient ADO.NET developer.
Comments:
Thank you all for taking the time to read my article on how ChatGPT enhances software development in ADO.NET. I'm excited to hear your thoughts and discuss this further!
Great article, Troy! ChatGPT seems like a promising tool for streamlining the coding process in ADO.NET. I'm particularly interested in how it can assist with debugging. Do you have any specific examples of how ChatGPT helped identify and fix bugs?
Thank you, Andrew! ChatGPT has indeed been helpful in debugging. In one instance, it helped identify a bug related to data validation by suggesting a more efficient way of handling user inputs. This not only resolved the bug but also improved the overall performance of the application.
Impressive article, Troy! The integration of ChatGPT into ADO.NET has the potential to revolutionize the way developers code. I'm curious about its compatibility with different programming languages and frameworks. Are there any limitations or restrictions to keep in mind?
Thank you, Maria! ChatGPT is designed to be language-agnostic, making it compatible with various programming languages and frameworks. However, there might be slight variations in implementation depending on the specific language or framework. It's always recommended to review the documentation and examples provided by OpenAI for best results.
I'd also like to know more about the debugging capabilities of ChatGPT. Troy mentioned it identified a bug, but how does it actually analyze the code and assist in debugging?
Good question, Daniel! ChatGPT analyzes the code by understanding the context and intent of the developer. It can assist in debugging by suggesting potential areas of improvement, providing code snippets, and offering insights on common bugs or coding patterns to watch out for. It's like having an AI-powered coding mentor by your side!
Troy, this integration sounds amazing! However, I'm curious about the computational requirements. Does ChatGPT heavily rely on powerful hardware, or can it run smoothly on standard developer machines?
Good question, Oliver! ChatGPT is designed to be resource-efficient, allowing it to run on standard developer machines without excessively taxing their hardware. OpenAI has made significant optimizations to ensure smooth performance in a wide range of environments.
Interesting read, Troy! I can see how ChatGPT can improve productivity in software development. However, I'm concerned about potential security risks when using an AI-powered tool like this. Are there any measures in place to address security vulnerabilities?
Liam, you raised an important concern. Security should be a priority when incorporating AI tools in software development. Troy, could you shed some light on the security measures implemented in ChatGPT for safe usage?
Absolutely, Sophia! OpenAI has made security a top priority. ChatGPT is built with safety mitigations to avoid generating malicious code or exposing sensitive data. However, it's always recommended to follow secure coding practices and review the suggestions provided by ChatGPT before implementation.
I'm also curious about the performance aspect, Troy. Did you notice any significant differences in coding speed or code quality when using ChatGPT?
Great question, Sophie! In terms of coding speed, ChatGPT can certainly increase productivity by providing instant suggestions and reducing the need for extensive manual research. As for code quality, it ultimately depends on the developer's expertise and the implementation choices made. ChatGPT can significantly aid in code review and adherence to best practices.
Troy, can ChatGPT detect syntax errors or offer help in correcting them? It would be amazing if it could assist with basic syntax mistakes as well.
That's a good point, Matthew! While ChatGPT is primarily focused on providing contextual suggestions and guidance, it can also assist in detecting common syntax errors and help in correcting them. Think of it as an intelligent programming assistant that covers both high-level design and low-level details!
Troy, it's impressive that ChatGPT doesn't require powerful hardware. Does it run locally on a developer's machine, or does it rely on cloud-based servers for processing?
Good question, Natalie! While developers can integrate ChatGPT into an on-premises infrastructure if desired, the default approach is to leverage OpenAI's cloud-based servers for processing. This allows for more scalability, reliable performance, and enables continuous improvements and updates to the ChatGPT model.
Thanks for addressing the security concerns, Troy. As AI-powered tools become more prevalent, it's crucial to ensure that the underlying technology is secure. Is there ongoing monitoring and maintenance to stay ahead of potential security vulnerabilities?
Absolutely, Ethan! OpenAI maintains a robust security monitoring and vulnerability management process. They actively analyze inputs, collect feedback, and work on addressing any identified security vulnerabilities promptly. It's a continuous effort to ensure the highest level of safety and security in using ChatGPT.
That's incredible, Troy! ChatGPT really seems like a versatile tool for developers. Can it understand and assist with complex programming concepts, like advanced algorithms or machine learning?
Great question, Grace! ChatGPT has a good understanding of a wide range of programming concepts and can provide assistance with complex topics, including advanced algorithms and machine learning. It can help clarify concepts, suggest relevant resources, and even provide code examples to accelerate your learning and development process.
Troy, I'm curious to know if ChatGPT takes into consideration project-specific guidelines or coding standards. Can it adapt to the specific requirements of different development teams?
Good question, Adam! ChatGPT can indeed adapt to project-specific guidelines and coding standards. It's designed to accommodate different development teams by allowing customization and integration of specific coding practices or style guides. This ensures alignment with established conventions and consistency across the team's codebase.
Troy, what kind of training data is used to train ChatGPT for ADO.NET software development? Is it purely based on existing codebases or does it include other resources like documentation and tutorials?
Great question, Sophie! ChatGPT is trained on a diverse range of data, including not just codebases, but also documentation, tutorials, forums, and other programming resources. This broad training corpus helps ChatGPT develop a comprehensive understanding of ADO.NET and its best practices, making it a versatile companion for developers.
Troy, could you elaborate on the integration process? What steps would a development team need to take to implement ChatGPT into their ADO.NET workflow?
Certainly, Benjamin! Integrating ChatGPT into an ADO.NET workflow typically involves the following steps: 1. Assessing the team's specific needs and considerations. 2. Ensuring the necessary infrastructure and resources are in place, which may include cloud-based access to ChatGPT. 3. Customizing ChatGPT to align with project-specific requirements. 4. Training and testing the ChatGPT model for optimal performance. 5. Incorporating ChatGPT into the team's development tools and processes, gradually utilizing it for coding assistance and guidance.
Troy, can ChatGPT assist in writing code for specific machine learning algorithms or suggest appropriate libraries to use? This would be incredibly helpful for developers exploring ML in ADO.NET.
Absolutely, Aaron! ChatGPT can assist in writing code for specific machine learning algorithms by providing code examples, suggesting relevant libraries or frameworks, and even helping with hyperparameter selection. It can be a valuable resource for developers venturing into ML in ADO.NET, making it easier to adopt and implement machine learning solutions.
Troy, I'm curious about the learning process of ChatGPT. Can it adapt and improve its suggestions over time based on developer feedback and usage patterns?
Good question, William! ChatGPT has the capability to learn from developer feedback and usage patterns. OpenAI leverages this feedback to continuously improve the model, refine its suggestions, and expand its capabilities. By incorporating real-world insights, ChatGPT's assistance becomes more accurate and valuable over time.
Troy, as ChatGPT is trained on diverse data sources, is it possible to guide it towards specific domains within ADO.NET for more focused assistance, like database management or user interface development?
Absolutely, Olivia! While ChatGPT has a broad understanding of ADO.NET, it can be fine-tuned and guided towards specific domains within ADO.NET for more focused assistance. By providing explicit prompts or context, developers can receive tailored suggestions and guidance related to database management, user interface development, or any other specific domain they require assistance with.
Troy, how does ChatGPT handle different coding styles or preferences within a development team? Can it adapt and provide suggestions based on individual developer preferences?
Good question, Sophia! ChatGPT can be customized to align with different coding styles or preferences within a development team. By providing feedback and corrections, developers can train ChatGPT to become more familiar with their coding style over time. This allows the tool to adapt and provide more accurate suggestions based on individual preferences, making it a personalized coding companion for each developer.
Troy, can ChatGPT assist with data preprocessing tasks in machine learning workflows? For example, cleaning and transforming data or handling missing values?
Good question, Ella! ChatGPT can certainly assist with data preprocessing tasks in machine learning workflows. It can suggest common data cleaning techniques or libraries for handling missing values. Additionally, it can help with feature selection, normalization, and other preprocessing steps to ensure the data is ready for effective ML model training.
Troy, what about scenarios where ChatGPT suggests incorrect or inefficient code? How can developers ensure the reliability and correctness of the suggestions?
That's a valid concern, Lucas. While ChatGPT strives to provide accurate assistance, there may be cases where suggestions can be incorrect or suboptimal. Developers should treat ChatGPT's suggestions as helpful insights rather than definitive answers. It's important to carefully review and test the proposed solutions, relying on one's own expertise and domain knowledge to ensure reliability and correctness in the final code implementation.
Troy, can ChatGPT assist with code documentation tasks, like generating function or API documentation based on the code implementation?
Absolutely, Isabella! ChatGPT can assist with code documentation tasks as well. By analyzing the code and its structure, it can suggest meaningful function or API documentation based on the implementation. This helps developers improve code maintainability and enhances the overall documentation quality.
Troy, can ChatGPT also provide guidance on selecting appropriate machine learning models or algorithms based on the problem domain and dataset characteristics?
Indeed, Emma! ChatGPT can assist in selecting appropriate machine learning models or algorithms. By understanding the problem domain and analyzing the dataset characteristics, it can suggest suitable models and help narrow down the potential choices. This saves time and effort in model selection, allowing developers to focus on other critical aspects of the machine learning workflow.
Troy, how does ChatGPT handle large codebases? Can it effectively assist with navigation and understanding of complex projects with thousands or millions of lines of code?
Good question, James! While ChatGPT can handle large codebases, its effectiveness may vary depending on the complexity and organization of the project. It can assist with navigation and provide insights into code snippets, but for an in-depth understanding of highly complex projects, developers may still rely on other tools and their own domain expertise to ensure comprehensive comprehension and maintainability.
Troy, how does ChatGPT handle scenarios where code requires domain-specific knowledge, like in scientific or financial applications? Can it adapt and provide assistance in these specialized areas?
Great question, Ava! ChatGPT's flexibility enables it to handle domain-specific knowledge to some extent. While it may not possess detailed expertise in all specialized areas, it can learn from context and adapt to provide assistance in scientific or financial applications. The more specific the prompt or question, the more likely ChatGPT can provide relevant advice and guidance.
Troy, what additional features or improvements can we expect to see in the future iterations of ChatGPT in terms of assisting software development?
Great question, Samuel! OpenAI has a roadmap to further improve ChatGPT's capabilities for assisting software development. Some upcoming features might include better understanding of context, improved code generation, enhanced support for additional programming languages, and tighter integration with existing development tools and workflows. OpenAI is actively working on expanding and refining ChatGPT's capabilities to make it an invaluable companion for developers.
Fascinating article, Troy! The concept of AI assisting developers in real-time coding is extremely intriguing. Have you conducted any performance comparisons between traditional coding and coding with ChatGPT?