How ChatGPT is Transforming Data Structures in Object-Oriented Programming
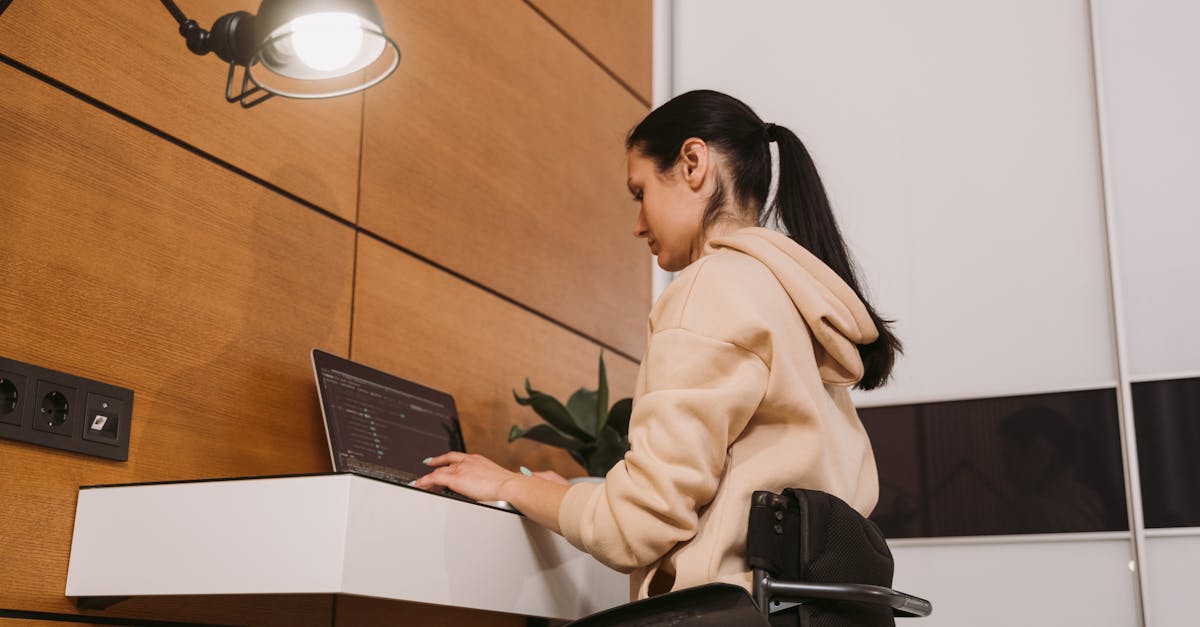
Introduction
Object-oriented programming (OOP) is a popular programming paradigm that focuses on using objects to design and implement software systems. It allows developers to organize code into reusable and maintainable structures, promoting modular and efficient development.
Data Structures in OOP
Data structures play a crucial role in managing and manipulating data efficiently in OOP. They provide a way to organize and store data in memory, allowing for easy access, retrieval, and modification.
1. Arrays
Arrays are one of the fundamental data structures used in OOP. They are containers that hold a fixed number of elements of the same type. Arrays provide fast access to elements using index-based retrieval and are widely used for storing and accessing collections of data.
2. Lists
Lists are dynamic data structures that allow for the efficient insertion and deletion of elements. They can grow and shrink as needed, making them suitable for scenarios requiring flexible data storage. In OOP, lists are often implemented using linked lists or dynamic arrays.
3. Stacks
Stacks follow the Last-In-First-Out (LIFO) principle, meaning that the last element added to the stack is the first one to be removed. They are used to manage function calls, undo/redo operations, and expression evaluation. Stacks are particularly useful when implementing recursion or parsing algorithms.
4. Queues
Queues follow the First-In-First-Out (FIFO) principle, wherein the first element added to the queue is the first one to be removed. They are commonly used in scenarios involving scheduling, task management, and resource allocation. Queues can be implemented using arrays or linked lists.
5. Trees
Trees are hierarchical data structures used extensively in OOP. They consist of nodes connected by edges and are used to represent hierarchies and relationships between elements. Trees have applications in database systems, file systems, and various searching and sorting algorithms.
6. Graphs
Graphs are a versatile data structure that represents a set of interconnected nodes. They are used to model complex relationships and are employed in social networks, network routing, and recommendation systems. Graphs can be implemented using adjacency matrices or adjacency lists.
Conclusion
Data structures are essential in object-oriented programming for efficient data organization and manipulation. By understanding and utilizing various data structures, developers can optimize their code and design robust software systems. Whether it's arrays, lists, stacks, queues, trees, or graphs, each data structure has its own strengths and use cases that make them valuable tools in software development.
Comments:
Great article, Andrew! I've been using ChatGPT in my object-oriented programming projects and it has truly transformed the way I work with data structures.
I completely agree, Sarah. ChatGPT has made it much easier to manipulate complex data structures in my code. It has definitely sped up my development process.
Yes, I've experienced the same, David. ChatGPT provides a more intuitive way to handle data structures. It's a game-changer for sure.
George, I agree that ChatGPT is a game-changer. The ability to converse with the model and get assistance with data structures has been incredibly beneficial.
That's great to hear, David! ChatGPT has definitely made working with complex data structures less daunting for me too.
Thanks for your kind words, David! I'm glad ChatGPT has been a valuable tool for you as well. Let's keep utilizing its power in our programming projects.
Sarah, I'm glad to hear that ChatGPT has had a positive impact on your work as well. It's truly a game-changer in the field of programming.
David, I appreciate your feedback! It's truly rewarding to see ChatGPT making a positive impact on developers' efficiency and productivity.
David, I completely agree. ChatGPT has made coding more enjoyable by abstracting away the complexities of data structure manipulation.
I haven't tried ChatGPT yet, but after reading this article, I'm definitely excited to see how it can enhance my object-oriented programming skills.
Andrew, thanks for shedding light on how ChatGPT integrates with object-oriented programming. It's fascinating to see how AI is impacting software development.
Mark, I couldn't agree more. AI technologies like ChatGPT are transforming various aspects of our daily lives, including programming.
Mark, AI's influence on programming is truly remarkable. It's exciting to be part of this transformative era.
This is an interesting perspective, Andrew. I'm curious to know how ChatGPT handles complex data structures compared to traditional programming methods.
Sophia, ChatGPT utilizes natural language processing capabilities to understand and manipulate complex data structures. It simplifies the code and reduces manual effort.
Andrew, thanks for the explanation. It sounds like an exciting tool for improving productivity in programming tasks that involve data structures.
You're welcome, Sophia. Indeed, ChatGPT enhances productivity by providing intelligent assistance in handling data structures, resulting in more efficient code.
I wonder how reliable ChatGPT is when it comes to recommending optimal data structure choices based on specific program requirements.
That's an important point, Daniel. While ChatGPT can provide suggestions, it's always crucial to apply domain knowledge and consider individual program needs.
Oliver, I completely agree. ChatGPT should be seen as a helpful tool, but the final decision on data structure choices should ultimately come from the programmer's expertise.
Oliver, perfect point. Ultimately, the programmer's expertise and understanding of the program requirements should guide the data structure choices, with ChatGPT serving as a valuable assistance tool.
I've been using ChatGPT and it has definitely simplified my work with data structures. It provides a more interactive and efficient approach.
Sophie, I'm curious to know if you've encountered any limitations or challenges when using ChatGPT for data structure transformations.
Sophia, one minor limitation I've noticed is ChatGPT's occasional lack of understanding of very specific data structure intricacies. However, it still significantly improves my workflow.
As a beginner in programming, I find it fascinating how AI like ChatGPT can make complex concepts like data structures more accessible and understandable for newcomers.
Alex, I couldn't agree more. AI-powered tools, including ChatGPT, can play a significant role in reducing the learning curve for beginners entering the programming field.
Michael, it's great to see how technology is evolving to assist beginners like me. It motivates me to continue learning and exploring programming.
Absolutely, Alex! Embrace the opportunities that technologies like ChatGPT offer, and you'll continue to grow as a programmer.
I agree with you, Michael. AI tools are paving the way for beginners to dive into programming with more confidence and less intimidation.
Thank you all for your valuable comments! It's inspiring to see the positive impact ChatGPT is having on programmers' workflows. Keep exploring and leveraging this powerful tool!
Andrew, your article has sparked great discussion. It's evident that ChatGPT is revolutionizing how we work with data structures in object-oriented programming. Exciting times!
It's fascinating to witness advancements in AI-enhanced programming tools like ChatGPT. The integration of ChatGPT with data structures opens up new possibilities for developers.
Precisely, Oliver. ChatGPT's suggestions should be evaluated in the context of the program's specific needs. It's a powerful tool, but human judgment remains vital.
Interesting point, Oliver. It's important to strike a balance between relying on AI recommendations and applying human expertise based on the program's unique requirements.
Indeed, Daniel. The collaboration between AI tools like ChatGPT and human expertise leads to optimal results when it comes to data structures in programming.
Absolutely, Oliver. It's all about striking the right balance and leveraging the strengths of both AI and human capabilities.
I'm glad I came across this article. ChatGPT's potential in transforming data structures is captivating. Can't wait to give it a try in my upcoming projects!
Sophia, you won't be disappointed! ChatGPT will make a positive difference in your programming workflow. It's definitely worth exploring.
Great to hear your firsthand experience, Sophie! I'll definitely incorporate ChatGPT into my future projects.
Absolutely, Sophie. ChatGPT opens up opportunities for more interactive and efficient handling of data structures, minimizing the risk of manual errors.
Sophie, I highly recommend exploring the integration of ChatGPT with data structures. It adds a whole new level of dynamism to your programming projects.
Well said, Sophie Martin. Leveraging ChatGPT as an assistance tool, while combining it with human judgment, ensures optimal decision-making in selecting data structures.
Exactly, Daniel. AI tools like ChatGPT should be seen as guides rather than decision-makers when it comes to data structure choices.
Oliver, I'll definitely explore integrating ChatGPT with data structures. It sounds like a powerful combination to improve efficiency and code quality.
You're welcome, Sophie Martin! I'm delighted to hear that you found the article informative. ChatGPT indeed revolutionizes data structures in object-oriented programming.
Andrew, thank you for writing such an informative article. It's fascinating to see how ChatGPT is transforming data structures in object-oriented programming.