How ChatGPT Revolutionizes RxJS Observables in Angular: Transforming the Way Developers Leverage Real-time Data
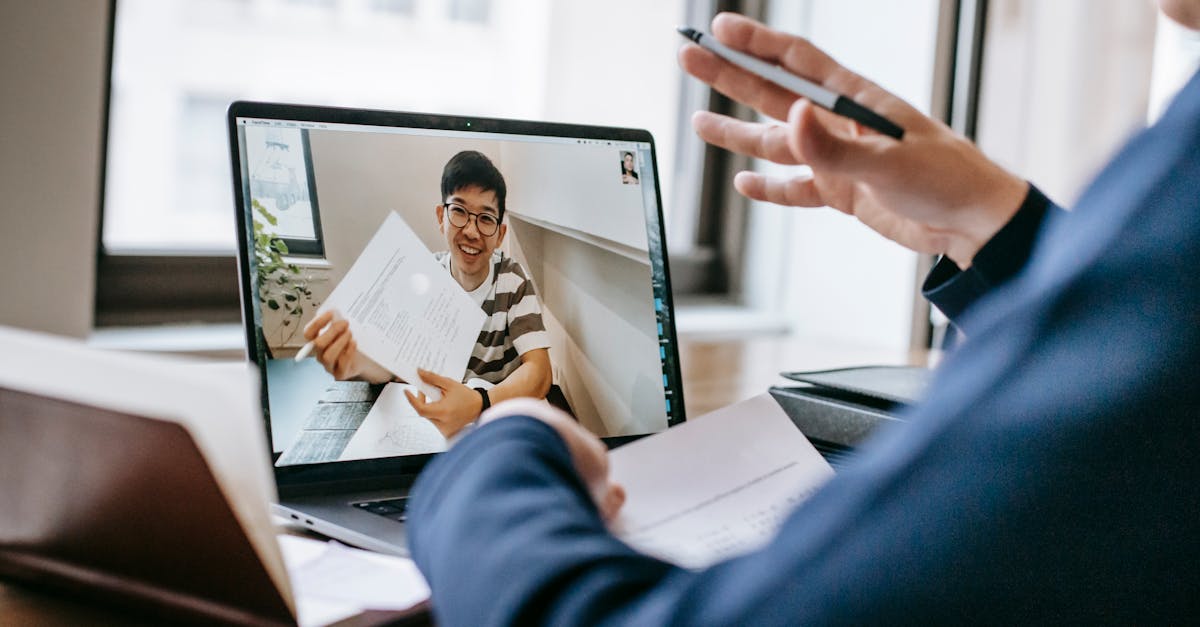
Angular is a popular JavaScript framework utilized for building dynamic web applications. One of the key features Angular provides is the powerful RxJS library for handling asynchronous operations using Observables. In this article, we will explore how the combination of Angular and RxJS Observables can be leveraged to improve the functionality and user experience of your application.
What are Observables?
Observables are a fundamental part of the RxJS library, which is a reactive programming library for handling asynchronous data streams. They represent a sequence of events or values that can be observed over time. With Observables, we can deal with asynchronous operations in a more streamlined manner, making it easier to manage data streams and handle events.
Why use Observables in Angular?
Angular applications often require handling asynchronous operations such as HTTP requests, user input, and data streams from various sources. Observables provide an elegant and efficient way to handle these operations, allowing developers to easily write clean and concise code. The use of Observables also promotes better code organization, enhanced maintainability, and improved testability of Angular applications.
How to use Observables in Angular?
Using Observables in Angular is straightforward. Angular leverages RxJS Observables natively, allowing you to easily subscribe to and manipulate data streams in your components. Here is a simple example of using Observables in an Angular component:
import { Component, OnInit } from '@angular/core';
import { Observable } from 'rxjs';
@Component({
selector: 'app-example',
template: `
Data Stream:
- {{ data }}
`
})
export class ExampleComponent implements OnInit {
dataStream: Observable;
constructor() { }
ngOnInit(): void {
this.dataStream = this.getDataStream();
}
getDataStream(): Observable {
// Simulate an asynchronous data stream
return new Observable(observer => {
setTimeout(() => {
observer.next(['Data 1', 'Data 2', 'Data 3']);
observer.complete();
}, 2000);
});
}
}
In the above example, we define a component that initializes an Observable dataStream in the ngOnInit lifecycle hook. The dataStream is then bound to the component's template using the async pipe, which subscribes and unsubscribes from the Observable automatically. The data emitted by the Observable is displayed as a list.
Benefits of using Observables in Angular
There are several benefits to using Observables in Angular with RxJS:
- Efficient handling of asynchronous operations
- Improved code organization and maintainability
- Streamlined error handling
- Easy composition of complex data flows
- Better support for real-time and interactive applications
Conclusion
Angular, combined with RxJS Observables, provides a powerful and flexible solution for handling asynchronous operations in web applications. Observables allow you to manage data streams, handle asynchronous events, and create more responsive and interactive user experiences. By using Observables in your Angular projects, you can enhance your application's functionality and improve overall development efficiency.
Start leveraging the benefits of Observables in Angular today and unlock the full potential of your web applications!
Comments:
Great article, Diego! I've been using RxJS Observables in Angular, and the idea of ChatGPT revolutionizing it sounds really promising.
I totally agree, Sara! Real-time data is so important in modern web applications. Can't wait to see how ChatGPT enhances it.
Thank you, Sara and Andrew! I appreciate your positive feedback. I'm excited to share more about ChatGPT's potential in transforming Angular development.
This is fascinating stuff, Diego! As a developer, I'm always looking for new ways to optimize my code and improve real-time data handling. Looking forward to learning more!
Hi, Maria! I'm glad you find it fascinating. ChatGPT indeed offers exciting possibilities for developers to leverage real-time data in Angular applications. Stay tuned for more information!
I'm curious about how ChatGPT handles RxJS Observables. Could you provide more technical details, Diego?
Of course, Peter! ChatGPT allows for more intuitive ways to transform and manipulate RxJS Observables. It simplifies the process and enhances code readability. I'll be discussing this in detail in an upcoming article. Keep an eye out!
As an Angular developer, I'm really excited about this new development! Can't wait to see the practical implementation examples.
Thanks for your enthusiasm, Olivia! I'll definitely include practical implementation examples to help developers grasp how ChatGPT can streamline their workflow with RxJS Observables.
This could be a game-changer! Real-time data handling can sometimes be complex. Looking forward to seeing how ChatGPT simplifies it.
Absolutely, Liam! ChatGPT offers a potential game-changer in real-time data handling. It aims to simplify the complex aspects, making it more accessible for developers. Stay tuned for more updates!
Diego, this is brilliant! I've always enjoyed working with Angular, and if ChatGPT can make RxJS Observables even better, count me in!
Thank you, Charlotte! It's great to hear your excitement. ChatGPT aims to enhance the development experience with RxJS Observables in Angular. I'm looking forward to showcasing its benefits.
Will ChatGPT provide any performance improvements when working with real-time data?
Great question, Brian! ChatGPT doesn't directly provide performance improvements. However, it offers a more streamlined approach to working with real-time data, which can indirectly alleviate potential performance bottlenecks. I'll cover this topic in more detail soon!
This sounds really exciting! Looking forward to experimenting with it in my Angular projects.
I'm thrilled to hear that, Emily! It's always exciting to experiment with new tools and technologies. ChatGPT can help you take your Angular projects to the next level. Let me know if you have any questions along the way!
I wonder how ChatGPT compares to other libraries or frameworks that enhance real-time data handling in Angular?
That's a valid point, Michael. ChatGPT is designed specifically to enhance real-time data handling with RxJS Observables in Angular. While there are other libraries out there, ChatGPT focuses on providing a more intuitive and streamlined development experience. I'll explore this comparison further in a future article.
This is intriguing! Can't wait to dive deeper into the possibilities of ChatGPT in real-time data handling.
I'm glad you find it intriguing, Lisa! Dive deeper with me as we explore the possibilities of ChatGPT in real-time data handling. Make sure to follow along for more insights!
Will there be any tutorials or examples available to learn how to use ChatGPT with RxJS Observables?
Absolutely, Alex! Tutorials and examples are crucial for developers to understand how to leverage ChatGPT with RxJS Observables effectively. I'll include hands-on tutorials and practical examples in upcoming articles. Stay tuned!
Can't wait to see how ChatGPT simplifies real-time data handling. As a developer, I'm always looking for tools that can improve my productivity.
Hi, Erica! ChatGPT aims to simplify real-time data handling to boost developers' productivity. I'm excited to demonstrate its capabilities in future articles. Let me know if you have any specific questions or areas of interest!
Looking forward to seeing how ChatGPT empowers developers to build more efficient and scalable real-time applications.
Thanks for your anticipation, Joshua! Building efficient and scalable real-time applications is crucial, and ChatGPT aims to empower developers in achieving those goals. I'll delve deeper into this topic soon!
Diego, as an Angular enthusiast, I'm excited to see how ChatGPT elevates the use of RxJS Observables. Can't wait for your next article!
Thank you, Sophia! It's great to have fellow Angular enthusiasts embrace the potential of ChatGPT. I'm eager to share more insights and knowledge through the upcoming article. Stay tuned!
How does ChatGPT handle errors or exceptions in the context of real-time data handling in Angular?
That's an important consideration, Jason. ChatGPT simplifies error handling by providing intuitive mechanisms to catch exceptions and handle errors in real-time data handling with Angular applications. I'll dive deeper into this topic soon!
This could be a game-changer for developers working with real-time data in Angular. Can't wait to learn more!
Absolutely, Lily! ChatGPT holds the potential to be a game-changer for developers working with real-time data in Angular. Stay tuned for more insights on how it can revolutionize your development workflow!
Is ChatGPT compatible with older versions of Angular, or is it built specifically for the latest versions?
Lucas, ChatGPT is designed to be compatible with both older and newer versions of Angular. The goal is to provide a seamless integration with RxJS Observables, regardless of the framework version you're using. I'll cover this topic more comprehensively in a future article.
I'm really excited about ChatGPT's potential in real-time data handling. Looking forward to exploring it further!
Hi, Eva! It's great to hear your excitement about ChatGPT's potential in real-time data handling. Follow me on this journey as we explore its capabilities. Let's make real-time data handling a breeze!
Are there any use cases where RxJS Observables combined with ChatGPT can provide more advantages than traditional data handling methods?
Absolutely, Ryan! RxJS Observables combined with ChatGPT can offer advantages in scenarios where real-time data plays a crucial role. From reactive UI updates to complex event handling, it opens up new possibilities for developers. I'll shed more light on this topic soon!
I'm genuinely excited about the potential of using ChatGPT with RxJS Observables. This could greatly impact the way we build real-time applications in Angular.
Thank you, Grace! I share your excitement about the potential impact of ChatGPT with RxJS Observables in real-time applications built with Angular. Together, we can revolutionize the way we build and handle real-time data!
Will ChatGPT offer seamless integration with existing projects that already use RxJS Observables?
Absolutely, Daniel! ChatGPT is designed to offer seamless integration with existing projects that use RxJS Observables. You won't have to start from scratch, and can easily incorporate its benefits into your projects. Stay tuned for more details on how to leverage this integration!
This is fascinating! I can see how ChatGPT can simplify the development process of real-time data handling. Can't wait to see practical examples.
Hi, Isabella! I'm glad you find it fascinating. Practical examples will be an important part of showcasing how ChatGPT simplifies the development process of real-time data handling. Let's explore its possibilities together!
What are the main challenges that ChatGPT addresses when it comes to leveraging real-time data in Angular?
Great question, Aiden! ChatGPT addresses several challenges, such as handling complex event streams, managing asynchronous data flows, and providing a more expressive and intuitive API for real-time data manipulation in Angular. Stay tuned as I examine these challenges in more detail!
I've mostly used traditional methods for handling real-time data. Looking forward to seeing how ChatGPT can provide a more efficient alternative in Angular.
Hi, Sophie! ChatGPT holds the potential to provide a more efficient alternative to traditional methods in Angular for handling real-time data. I'm excited to illustrate its benefits and how it can streamline your workflow. Let me know if you have any specific questions or concerns!
Is ChatGPT compatible with other popular frontend frameworks, or is it focused solely on Angular?
Great question, Avery! While ChatGPT is designed with Angular in mind, its principles can be applied to other popular frontend frameworks leveraging RxJS Observables. Although the context of my articles will focus on Angular, the concepts can be adapted. I appreciate your curiosity!