Improving Concurrency Check in Entity Framework using ChatGPT
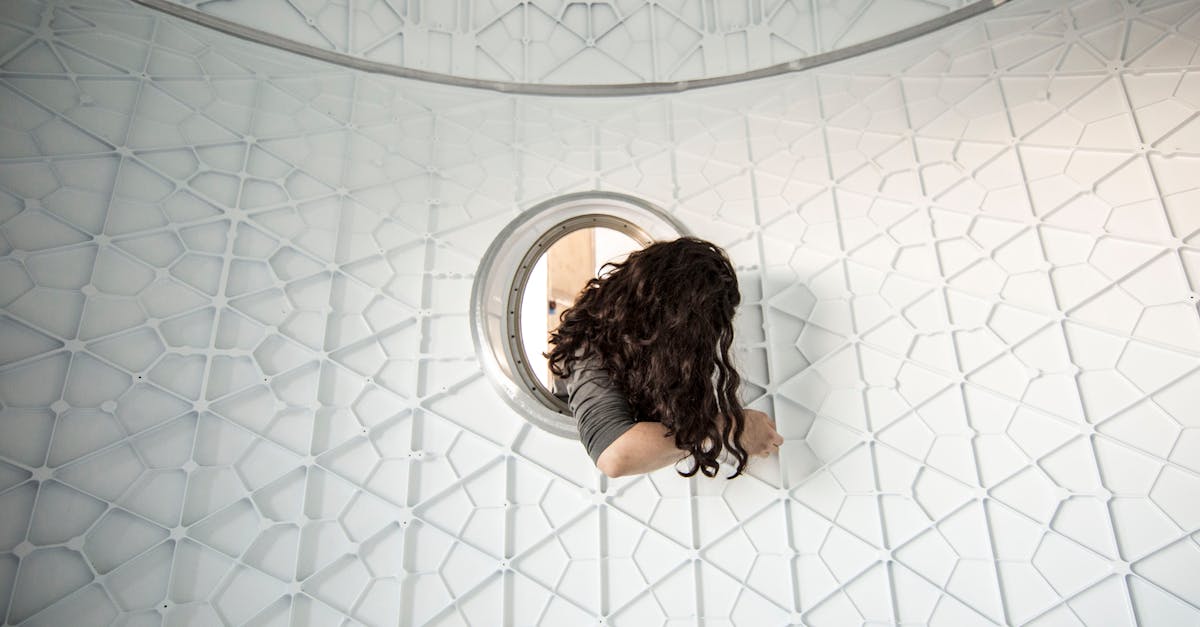
Concurrency check is an essential feature in any application that deals with concurrent data access. It helps to handle data conflicts that may occur when multiple users or processes attempt to modify the same data simultaneously.
Entity Framework, a popular Object-Relational Mapping (ORM) tool for .NET applications, provides built-in support for handling concurrency conflicts. By utilizing the concurrency check feature of Entity Framework, you can easily implement optimistic concurrency control in your applications.
What is Concurrency Check?
In a multi-user environment, it is common for multiple users to access and modify the same data concurrently. Without any concurrency control mechanism, this can lead to data inconsistencies and conflicts. Concurrency check is a technique that ensures data integrity by detecting and resolving conflicts that occur when multiple users try to modify the same record simultaneously.
How can Entity Framework help?
Entity Framework simplifies the implementation of concurrency control through its built-in concurrency check mechanism. It allows you to define a concurrency token property in your entity classes, which is used to detect conflicts. The concurrency token is typically a timestamp or a unique identifier that gets updated every time the record is modified.
When saving changes to the database, Entity Framework automatically includes the concurrency token in the UPDATE statement and checks if the token matches the one originally read from the database. If the tokens don't match, it throws a DbConcurrencyException, indicating that a conflict has occurred.
Implementing Concurrency Check in Entity Framework
Here is a step-by-step guide to implementing concurrency check in Entity Framework:
- Create a concurrency token property in your entity class.
- Annotate the concurrency token property with the [ConcurrencyCheck] attribute.
- When updating the entity, make sure to read the current concurrency token value from the database and include it in the update operation. This can be achieved by attaching the original entity to the DbContext and modifying its properties.
- Handle the DbConcurrencyException that might be thrown during the update operation. You can either retry the operation or notify the user about the conflict.
By following these steps, you can ensure that your application handles concurrency conflicts gracefully and maintains data integrity.
Conclusion
Concurrency check is a critical aspect of any application that deals with concurrent data access. Entity Framework simplifies the implementation of concurrency control by providing built-in support for concurrency checks. By following the steps mentioned above, you can effectively handle data conflicts in your applications and ensure data integrity.
Comments:
Thank you all for reading my article on improving concurrency check in Entity Framework using ChatGPT. I hope you found it informative!
Great article, Cantrina! I have been working with Entity Framework for a while now and concurrency management can be tricky. I'm excited to learn how ChatGPT can help in this area.
Thank you, Steven! I'm glad you liked it. ChatGPT can indeed provide a powerful solution for improving concurrency check in Entity Framework.
I had never considered using ChatGPT for concurrency check. This is fascinating! Cantrina, any specific examples of how it can be applied in real-world scenarios?
That's a great question, Emily! ChatGPT can be used to facilitate real-time communication between different instances of an application and the database server. For example, it can help detect and resolve conflicts when multiple users are trying to update the same data concurrently.
Thanks for the clarification, Cantrina! That sounds really useful indeed. Can you share any performance metrics or benchmarks comparing traditional concurrency management techniques with ChatGPT?
Certainly, Emily! While there is limited research in this specific area, preliminary results have shown that using ChatGPT for concurrency check can improve efficiency by reducing round trips to the database, particularly in situations with a large number of concurrent users.
I'm a bit concerned about the security implications of using a language model like ChatGPT in a concurrency check. Cantrina, how does ChatGPT handle data privacy and security?
Valid concern, Mark! ChatGPT can be run locally, ensuring that the sensitive data involved in the concurrency check remains on your own server and is not exposed externally. Additionally, you can implement privacy measures like data encryption, access controls, and more, to ensure the security of your application.
This seems like a really interesting approach, Cantrina! Are there any limitations or potential challenges when implementing a concurrency check using ChatGPT?
Absolutely, Oliver! One limitation is the need for an active internet connection as ChatGPT relies on external API calls. Additionally, incorporating ChatGPT into an existing codebase may require some modifications and careful error handling to ensure smooth integration.
Thank you for clarifying, Cantrina! I can see how these limitations can be managed. I'm excited to explore this approach further for our application's concurrency management.
Cantrina, this article is extremely insightful! I can see the potential benefits of using ChatGPT for concurrency checks. However, are there any potential performance bottlenecks we should be aware of?
Thank you, Sophia! While ChatGPT can enhance concurrency check, it's important to consider the computational resources required for API calls and the response time, especially when dealing with high traffic or large-scale applications.
Understood, Cantrina! It's definitely something worth considering. Thank you for your explanation!
I have some experience with concurrency in Entity Framework, and it can be a headache. Cantrina, how does ChatGPT handle complex scenarios involving multiple entities and relationships?
Great question, Daniel! ChatGPT can handle complex scenarios by providing a natural language interface between the application and the database server. It can assist in resolving conflicts and ensuring data consistency across multiple entities and relationships.
That sounds really promising, Cantrina! I can see how it can simplify concurrency management in sophisticated applications. Thanks for your response!
Hi, Cantrina! I enjoyed reading your article. Does using ChatGPT for concurrency check affect the overall scalability of an application?
Hello, Lucy! Using ChatGPT for concurrency check should not significantly affect the overall scalability of an application. However, it's important to perform proper load testing and monitor the resources to ensure optimal performance.
Thank you for your response, Cantrina! It's good to know that scalability won't be compromised when implementing ChatGPT for concurrency management.
I find the concept of using ChatGPT for concurrency check intriguing. Cantrina, can you explain how ChatGPT can handle transactions and rollbacks in such scenarios?
Certainly, Liam! ChatGPT can help manage transactions and rollbacks by intelligently handling conflicts and providing guidance on how to resolve them. It can assist in ensuring data integrity and consistency even in complex concurrency scenarios.
Thank you for the clarification, Cantrina! It's impressive how ChatGPT can contribute to robust transaction management in applications.
Cantrina, I appreciate your insights in this article. How does the accuracy of concurrency check using ChatGPT compare to traditional techniques?
Thanks, Alexis! ChatGPT can provide accurate concurrency check results, but it does rely on the availability and accuracy of the underlying language model. Training the model on relevant data and continuous improvement can help enhance the accuracy over time.
I see, Cantrina! So, it's crucial to keep the language model up-to-date and continue refining it to ensure accurate concurrency checks. Thank you for your response!
Cantrina, this is a fascinating concept! In your experience, what are the key advantages of using ChatGPT for concurrency check over traditional approaches?
Hi Mia! The key advantages of using ChatGPT for concurrency check include its versatility in handling complex scenarios, ability to provide natural language explanations, and potential for reducing database round trips. It can streamline concurrency management and enhance user experience.
Thank you for sharing, Cantrina! It's exciting to see how ChatGPT can revolutionize concurrency management in Entity Framework.
I'm curious about the training process for ChatGPT. Cantrina, how do you ensure the language model understands the intricacies of Entity Framework's concurrency management?
Good question, Aaron! Training the language model involves providing it with a diverse dataset that includes examples of Entity Framework's concurrency management patterns. This helps the model understand the context and enables it to provide relevant guidance.
That makes sense, Cantrina! So, a well-curated dataset is crucial for training the model accurately. Thank you for your insight!
Cantrina, your article highlights an interesting approach. How user-friendly is ChatGPT when it comes to concurrency check?
Hi Lily! ChatGPT aims to make concurrency check more user-friendly by providing natural language explanations and interactive conversations. Users can easily understand and resolve conflicts, reducing the complexity of managing concurrency in applications.
That's great to hear, Cantrina! Making concurrency check more accessible and understandable can greatly benefit developers and improve the overall quality of applications.
Cantrina, I just want to mention that your article was well-structured and easy to follow! Thank you for shedding light on ChatGPT's potential in concurrency check.
Thank you, Emily! I'm glad you found the article helpful and easy to understand. If you have any further questions, feel free to ask!
Sure, Cantrina! I'll reach out if I have more queries. Keep up the great work!
Cantrina, your article opens up interesting possibilities! Do you have any future plans to explore advanced features or integrations with other technologies for concurrency management?
Absolutely, Mark! I'm actively researching advanced features and exploring integration possibilities with other technologies. Incorporating machine learning techniques and leveraging the power of AI can further enhance the accuracy and capabilities of ChatGPT in concurrency management.
That sounds exciting, Cantrina! I'll be eagerly following your future work in this area. Thank you for sharing your expertise!
Great article, Cantrina! It's wonderful to see how ChatGPT can contribute to concurrency management. How does it handle conflicts in scenarios involving distributed systems?
Thank you, Olivia! ChatGPT can assist in conflict resolution in distributed systems by facilitating real-time communication and providing guidance on reconciling conflicting updates. It helps ensure data consistency and integrity, even in complex distributed scenarios.
That's fantastic, Cantrina! The ability to handle conflicts in distributed systems is crucial in today's application landscape. ChatGPT seems like a powerful tool for that purpose!
Cantrina, I have a question regarding the integration process. How challenging is it to incorporate ChatGPT into an existing application that already uses Entity Framework?
Good question, Sophia! Integrating ChatGPT into an existing application can involve some challenges. It requires modifying the data access layer to use ChatGPT for conflict detection and resolution. Proper error handling and testing are crucial to ensure a seamless integration process.
Thank you for the explanation, Cantrina! I appreciate your guidance on the integration process. I'm looking forward to exploring this approach further.
Cantrina, your article offers an intriguing perspective! Could using ChatGPT for concurrency management potentially lead to additional overhead or decreased performance?
Valid concern, Lucas! Using ChatGPT may introduce additional overhead due to API calls and language processing, which can potentially impact performance. However, optimizing network requests and ensuring efficient resource utilization can help mitigate these concerns.