Improving Debugging Efficiency: Harnessing ChatGPT for C++ Language Debugging
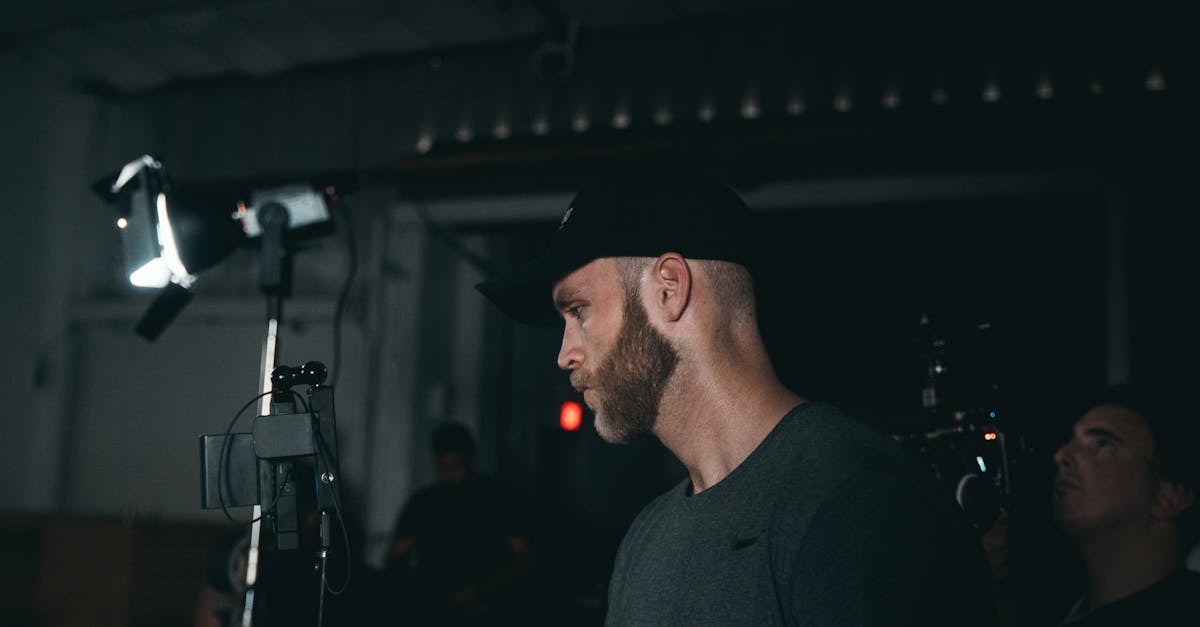
Debugging is an essential part of the software development process. It involves identifying and fixing errors or bugs in a program. The C++ language provides a powerful set of tools and techniques that can predict and help debug errors efficiently.
C++ is a widely used programming language for developing various applications, and with its strong typing, high performance, and extensive library support, it offers great potential for software development. However, like any programming language, C++ programs may contain errors or bugs that prevent them from functioning correctly.
Predicting Errors in C++ Programs
C++ provides several features that can help identify and predict errors before the program is executed. The compiler, for example, performs a static analysis of the code and can catch syntax errors, type mismatches, and other potential issues. It checks for any violations of the language's rules and generates error messages to alert the developer.
Additionally, C++ provides static code analyzers that can detect potential errors based on coding conventions, best practices, and common mistakes. These analyzers can flag issues such as uninitialized variables, unused code, memory leaks, and more, helping developers catch errors even before running the program.
Debugging Techniques in C++
Despite the best efforts to predict errors, they may still occur during program execution. C++ provides a range of powerful debugging features that can facilitate the process of identifying and fixing these errors.
One of the most commonly used techniques is the use of breakpoints. Breakpoints allow developers to pause program execution at a specific line of code and inspect the state of variables, evaluate expressions, and analyze the control flow. By strategically placing breakpoints in the code, developers can isolate the problematic areas and step through the program to understand its behavior.
Another helpful debugging technique is the use of log statements. By adding print statements at various points in the code, developers can output the values of variables, trace the program's execution path, and analyze the program flow. This technique can be particularly helpful in identifying issues that occur intermittently or in complex scenarios.
C++ also provides the ability to perform runtime assertions. These assertions allow developers to validate assumptions about the program's state at specific points in the code. If an assertion fails, an error message is generated, which helps pinpoint the cause of the error. Runtime assertions are especially useful for testing and debugging complex algorithms and data structures.
Integrated Development Environments (IDEs)
To aid in the debugging process, various Integrated Development Environments (IDEs) provide advanced debugging features specifically designed for C++ development. These IDEs offer features such as advanced breakpoints, variable inspection, call stack analysis, and real-time debugging, making the process of identifying and fixing errors more efficient and effective.
Some popular IDEs for C++ development with robust debugging capabilities include Microsoft Visual Studio, Code::Blocks, Qt Creator, and Eclipse with the CDT (C/C++ Development Tools) plugin.
Conclusion
Debugging is an integral part of the software development process, and C++ provides a range of powerful tools and techniques to aid in this process. By utilizing the predictive capabilities of the compiler and static analyzers, as well as employing debugging techniques such as breakpoints, log statements, and runtime assertions, developers can efficiently identify and fix errors in C++ programs.
Moreover, the availability of advanced debugging features in modern IDEs further enhances the debugging experience and accelerates the development cycle. With the use of these debugging resources, developers can ensure the reliability and stability of their C++ applications.
Comments:
Thank you all for taking the time to read my article on improving debugging efficiency using ChatGPT for C++ language debugging. I'm excited to hear your thoughts and opinions!
Great article, Amanda! I particularly liked how you explained the potential of using natural language in debugging. It could definitely help bridge the gap between experienced programmers and those who are just starting out.
Thank you, Lisa! Yes, that's one of the key advantages of using ChatGPT for debugging. It can make the process more accessible to beginners and less intimidating.
I'm not convinced that AI-powered debugging tools can be as effective as traditional debugging techniques. Sometimes, simple and systematic approaches work better.
That's a valid concern, Michael. While AI-powered tools like ChatGPT can be helpful, they are not meant to replace traditional debugging techniques. It's more about augmenting and enhancing the debugging process.
I have to agree with Michael. It's hard to trust an AI system to debug complex code accurately. I'd rather rely on my own debugging skills and experience.
I understand your concern, Jennifer. AI systems like ChatGPT are not infallible, but they can provide valuable insights and suggestions that may complement your own debugging skills.
This article opened my eyes to the potential of AI in debugging. With ChatGPT, I can see how it would significantly speed up the process of finding and fixing bugs.
I'm glad to hear that, Robert! Indeed, by harnessing the power of AI, we can reduce the time spent on debugging and make the development process more efficient.
What about the risk of over-reliance on AI tools? Won't developers become too dependent on them and neglect their own critical thinking?
That's a valid concern, David. It's important for developers to strike a balance and not solely rely on AI tools. Critical thinking and manual debugging skills remain essential in ensuring the quality and reliability of code.
I can see how ChatGPT would be helpful for beginners, but what about experienced programmers? Can it bring any value to them?
Absolutely, Sarah! Even experienced programmers can benefit from the natural language capabilities of ChatGPT. It can help them think about code in different ways and provide fresh insights during difficult debugging sessions.
This article got me excited about trying out ChatGPT for debugging! Are there any specific tools or libraries you recommend to get started?
I'm glad you're enthusiastic, Mark! To get started with ChatGPT for debugging, you can explore OpenAI's GPT-3 API and integrate it into your existing development environment. OpenAI provides helpful documentation and examples to guide you along the way.
I'm curious about the limitations of ChatGPT in the context of C++ language debugging. Are there any known challenges or areas where it struggles?
Good question, Emily! While ChatGPT can be useful, it has limitations. It may have difficulty understanding very specific or complex code, and its suggestions should always be reviewed critically. It's best to start with small code snippets and gradually test its effectiveness.
I'm concerned about the potential security risks of using an AI system like ChatGPT for debugging. How can we ensure the privacy and integrity of our code?
That's an important concern, Peter. OpenAI takes privacy and security seriously. When using the GPT-3 API, you should follow the best practices recommended by OpenAI to protect the confidentiality of your code and data.
I'm amazed by the potential of AI to transform the debugging process. It will be interesting to see how AI-powered tools evolve in the future. Great article, Amanda!
Thank you, Daniel! Indeed, the future of AI-powered debugging tools is promising. As AI technology advances, we can expect even more sophisticated and effective solutions.
I wonder if ChatGPT can help with optimization issues in C++ code. Sometimes it's not just about debugging, but also improving performance. Any insights on that?
That's a great point, Olivia! While ChatGPT primarily focuses on debugging, it can potentially provide suggestions for optimization as well. It's worth exploring and experimenting with different use cases.
I appreciate how this article highlights the importance of leveraging AI to empower developers. It's about using technology to enhance our skills, not replace us. Well said, Amanda!
Thank you, Karen! That's exactly the message I wanted to convey. AI can amplify our capabilities and improve our productivity as developers.
I have mixed feelings about relying on an AI system for debugging. Can it really understand all the intricacies and intentions behind the code?
I understand your concern, Jacob. While ChatGPT may not comprehend the intricacies at the same level as a human, it can still provide valuable insights and suggestions. It's important to use it as a tool to augment your debugging process rather than rely on it entirely.
This article made me realize the untapped potential of AI in the field of debugging. It's exciting to think about how it can revolutionize the way we develop and maintain software.
I'm glad you found it eye-opening, Sophia! The possibilities are indeed exciting, and with the collaborative efforts of developers and AI systems, we can usher in a new era of more efficient software development.
I appreciate the balanced perspective of this article. It acknowledges the potential of AI while acknowledging the importance of traditional debugging skills. Well done, Amanda!
Thank you, George! It's crucial to strike a balance and leverage both AI and traditional debugging techniques to ensure the best outcomes in software development.
As a beginner, this article gives me hope that debugging won't always be an insurmountable mountain to climb. Excited to explore the possibilities of ChatGPT!
I'm glad to hear that, Jennifer! ChatGPT can certainly make debugging more accessible and less daunting for beginners. Best of luck in your debugging journey!
What are the potential downsides of using AI-powered debugging tools like ChatGPT? Are there any trade-offs?
Good question, Kevin! One potential downside is the need for a reliable internet connection when using AI-powered tools. Additionally, like any AI system, ChatGPT may produce incorrect or misleading suggestions, so it's crucial to verify and analyze its outputs.
I'm wondering how ChatGPT handles large codebases. Can it effectively handle complex projects, or is it more suitable for smaller code snippets?
That's a valid question, Michelle. While ChatGPT can handle larger codebases to some extent, it's generally more effective for smaller code snippets. Starting with smaller portions of the code can provide better results and insights.
I can see how ChatGPT can be beneficial, especially for those projects where the original developer is no longer available to provide insights. It can bridge the knowledge gap.
Exactly, Julia! ChatGPT can be a valuable resource in such situations, helping other developers understand and maintain code even when the original developer is unavailable.
This article has inspired me to explore AI further in my debugging process. Are there any other AI models or tools you recommend alongside ChatGPT?
I'm glad to hear that, Ryan! Alongside ChatGPT, you can also explore other AI models like GPT-4 in the future, as well as other debugging-specific tools and frameworks that may emerge in the AI community.
I'm concerned about the potential biases in AI systems like ChatGPT. How can we ensure fairness and prevent biases from affecting the debugging process?
That's an important aspect to consider, Natalie. OpenAI is actively working on reducing biases in their models and ensuring fairness. Developers using AI-powered debugging tools should also be aware of potential biases and apply critical thinking when interpreting suggestions.
I appreciate how this article highlights the practical applications of AI in the developer's workflow. It's exciting to see the potential unfold in real-world scenarios.
Thank you, Ethan! AI has indeed come a long way, and its practical applications can greatly benefit developers and make their workflows more efficient.
I love how you laid out the advantages and considerations of using ChatGPT for debugging. It helps developers make informed decisions about incorporating AI in their workflow.
Thank you, Patricia! I wanted to provide a balanced view so that developers can evaluate the advantages and considerations of using AI-powered debugging tools in their specific contexts and projects.
The potential of AI in debugging is undeniable. It will be interesting to see how it evolves and becomes more integrated into the development process.
Indeed, Christopher! The evolution and integration of AI in the development process will likely lead to more efficient debugging and software development practices.
Thank you all for your valuable comments and engaging in this discussion! I appreciate your perspectives and insights on using ChatGPT for C++ language debugging. If you have any further questions or thoughts, feel free to ask!