Improving Form Validation in Bootstrap with ChatGPT: A Breakthrough in User Experience
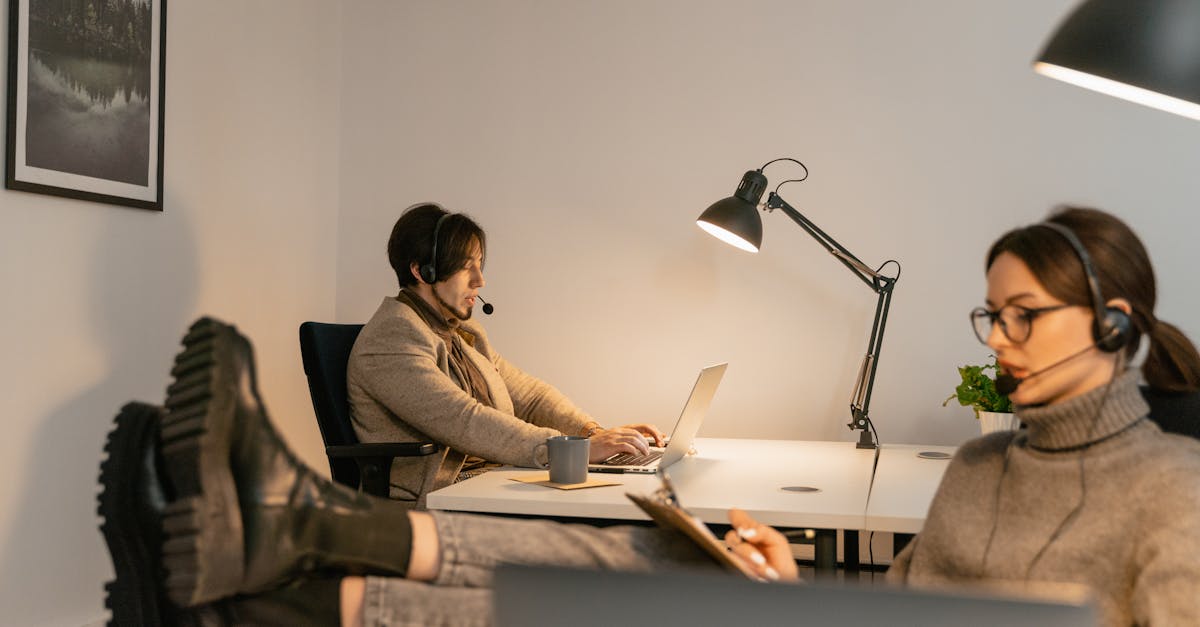
Form validation is an essential part of web development to ensure the accuracy and reliability of user-submitted data. Bootstrap, a popular frontend framework, provides built-in form validation features that can help developers implement effective form validation in their projects.
Getting Started
To use Bootstrap's form validation, include the Bootstrap CSS file in your project:
<link href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
Once you have included the CSS file, you can start using Bootstrap's form validation classes and attributes in your HTML forms.
HTML Form Structure
Here's an example of a simple HTML form structure:
<form novalidate>
<div >
<label >Name</label>
<input required>
<div >Please enter your name.</div>
</div>
<div >
<label >Email address</label>
<input required>
<div >Please enter a valid email address.</div>
</div>
<button >Submit</button>
</form>
Form Validation Classes
Bootstrap provides several CSS classes for form validation:
.needs-validation
: Add this class to the<form>
element to enable form validation..valid
: Apply this class to form controls that pass validation..invalid
: Apply this class to form controls that fail validation..invalid-feedback
: Add this class to the feedback message element for invalid controls.
Validating Form Controls
By default, Bootstrap uses HTML5 validation attributes such as required
, , etc. to validate form controls. The
.needs-validation
class ensures that these attributes are enforced.
When a form control fails validation, Bootstrap automatically applies the .invalid
class to the control and displays the associated .invalid-feedback
message. Conversely, when a control passes validation, the .valid
class is applied.
Custom Validation Styles
You can customize the appearance of invalid and valid form controls by adding custom styles to your CSS file. For example:
.needs-validation input:invalid {
border-color: red;
}
.needs-validation input.valid {
border-color: green;
}
.needs-validation .invalid-feedback {
display: block;
}
In this example, invalid form controls will have a red border color, valid controls will have a green border color, and the invalid feedback message will be displayed.
Conclusion
With Bootstrap's built-in form validation features, implementing effective form validation in your project becomes easier. By making use of the .needs-validation
class, along with HTML5 validation attributes, you can ensure that user-submitted data is accurate and reliable. Furthermore, you can customize the visual appearance of invalid and valid form controls to match your project's design.
Start using Bootstrap's form validation today and deliver a more user-friendly experience to your website visitors!
Comments:
Thank you all for reading my article on improving form validation in Bootstrap with ChatGPT. I'm really excited to hear your thoughts and opinions!
Great article, Joseph! I really enjoyed reading it. The idea of using ChatGPT for form validation is intriguing. Can you provide more details on how it works?
Thank you, Sarah! Sure, ChatGPT is a language model developed by OpenAI. We can fine-tune it to understand and respond to specific prompts, such as form validation. It uses natural language processing techniques to provide dynamic and interactive feedback to users.
I like the concept of using ChatGPT for better form validation. It could definitely enhance the user experience. Are there any specific advantages of using ChatGPT over traditional validation methods?
Thanks, Michael! One advantage is that ChatGPT can provide more contextual and conversational feedback to users. It can understand user queries and respond accordingly, guiding users through the validation process with personalized assistance.
Interesting approach, Joseph! However, I'm a bit concerned about the accuracy and reliability of ChatGPT. How does it handle complex validation scenarios?
Valid concern, Emily. ChatGPT performs well in general, but it may have limitations in handling extremely complex validation scenarios. In such cases, it can still offer suggestions and guidelines, but it's essential to ensure that critical validations are also implemented on the server-side to maintain data integrity.
Nice article, Joseph! I can see how using ChatGPT can make form validation more user-friendly. Do you have any live examples where ChatGPT is being used successfully?
Thank you, Nathan! Currently, there aren't many live examples of ChatGPT being used for form validation, as it's a relatively new concept. However, there are successful implementations of ChatGPT in various other domains, such as customer support and content generation.
I like the idea of improving user experience, but won't using ChatGPT for form validation increase the load on the server and potentially slow down the process?
That's a valid concern, Alex. While using ChatGPT may have some impact on server load and response time, it can be mitigated by optimizing the implementation and leveraging caching mechanisms. Proper infrastructure planning can help ensure smooth functionality.
I'm excited about the potential of ChatGPT for form validation. Joseph, do you have any recommendations on the best practices for implementing this approach?
Absolutely, Sophia! Here are a few recommendations: 1) Start with well-defined prompts to guide the user inputs, 2) Train the model on relevant data to improve accuracy, 3) Implement smooth error handling and fallback mechanisms, and 4) Continuously evaluate and improve the system based on user feedback and real-world usage.
Interesting article, Joseph! Do you foresee any potential challenges or limitations when incorporating ChatGPT for form validation?
Certainly, Robert! Some potential challenges include ensuring data privacy and security, addressing bias in the model's responses, and handling extreme edge cases that may not be well-suited for a language model. It's crucial to strike a balance and supplement ChatGPT's capabilities with other validation techniques when needed.
Thanks for sharing your insights, Joseph. I have a question about scalability. How well does ChatGPT handle a high volume of concurrent form validation requests?
Good question, Olivia! The scalability of ChatGPT depends on the underlying infrastructure and resource allocation. With proper scaling and provisioning, it can handle a significant number of concurrent requests. However, it's essential to monitor the system to ensure optimal performance and make adjustments as needed.
This article got me thinking about using ChatGPT for other user interactions beyond form validation. What are your thoughts, Joseph?
Absolutely, Daniel! ChatGPT can be a powerful tool for various user interactions, such as onboarding processes, interactive product tours, and conversational assistance. Its flexibility and ability to understand and respond to natural language make it well-suited for enhancing user experiences in many domains.
Joseph, what do you think about incorporating user feedback into the ChatGPT model to improve its accuracy and effectiveness?
Great point, Michelle! Incorporating user feedback is crucial for model improvement. OpenAI is actively working on approaches to solicit and leverage user feedback to address biases, improve responses, and ensure the model aligns with user expectations. It's an ongoing process that requires collaboration between developers and users.
As a UX designer, I'm always looking for ways to improve the user experience. Joseph, do you have any design considerations when implementing ChatGPT for form validation?
Absolutely, Rachel! Some design considerations include providing clear instructions and prompts, ensuring the ChatGPT responses are visually distinct from validation errors, considering accessibility requirements, and offering a fallback mechanism in case ChatGPT encounters a problem. Good design will provide a seamless and intuitive experience for users.
Interesting article, Joseph! I wonder how ChatGPT handles multilingual form validation. Any insights on that?
Thanks, Gabriel! ChatGPT can handle multilingual scenarios to some extent. By providing translated prompts and training the model on multilingual data, it can provide validation feedback in different languages. However, there may be limitations in understanding certain languages or cultural nuances, so it's important to test and refine the system as needed.
Joseph, I must say this is an innovative approach! Can ChatGPT differentiate between optional and mandatory form fields?
Thank you, Anna! ChatGPT can be trained to differentiate between optional and mandatory fields by providing clear instructions and prompts. By leveraging this distinction, it can guide users to complete mandatory fields and provide contextual feedback for optional ones.
Joseph, I enjoyed reading your article. How does ChatGPT handle real-time validation as users type?
Thanks, David! Real-time validation can be achieved by continuously feeding user input to ChatGPT and obtaining immediate feedback. This allows users to receive validation suggestions as they type or make changes, providing a seamless and proactive validation experience.
Joseph, what are your thoughts on the potential use of ChatGPT for more complex forms, such as multi-step or conditional forms?
Good question, Sophie! ChatGPT can certainly be used for more complex forms with multiple steps or conditional logic. By adapting the prompts and training data, it can provide guidance throughout the form completion process, ensuring users have a smooth experience while handling complex validation scenarios.
Joseph, can ChatGPT handle validation rules that require interaction with external services or APIs?
Certainly, Maxwell! ChatGPT can integrate with external services or APIs to perform validation tasks that require interaction or data retrieval from external sources. This enables it to handle more advanced validation rules and provide accurate feedback based on real-time data.
Hi Joseph, I loved your article! Would you recommend using ChatGPT for all types of form validation or are there specific cases where it shines the most?
Thank you, Amy! While ChatGPT can add value to various types of form validation, it is particularly useful in scenarios where a more conversational and interactive approach is desired. For complex forms or situations where personalized assistance is beneficial, ChatGPT can shine and greatly enhance the user experience.
Joseph, great article! I'm curious to know if there any performance implications when using ChatGPT for form validation?
Thank you, Liam! ChatGPT's performance depends on the computational resources and infrastructure allocated to it. By optimizing the implementation and leveraging techniques like caching and precomputation, it's possible to ensure fast response times and a smooth user experience.
Joseph, I appreciate your insights on improving form validation. Do you have any tips for developers who want to get started with implementing ChatGPT for this purpose?
Absolutely, Ella! To get started, developers can explore OpenAI's documentation and guides on using ChatGPT. It's important to define clear prompts, gather relevant training data, fine-tune the model, and iterate based on user feedback. Experimentation and continuous improvement are key in realizing the full potential of ChatGPT for form validation.
Thank you all for your engaging comments and questions! I appreciate your time and your interest in improving form validation with ChatGPT.