Improving User Experiences with ChatGPT: The Power of Responsive UI Design in Xamarin
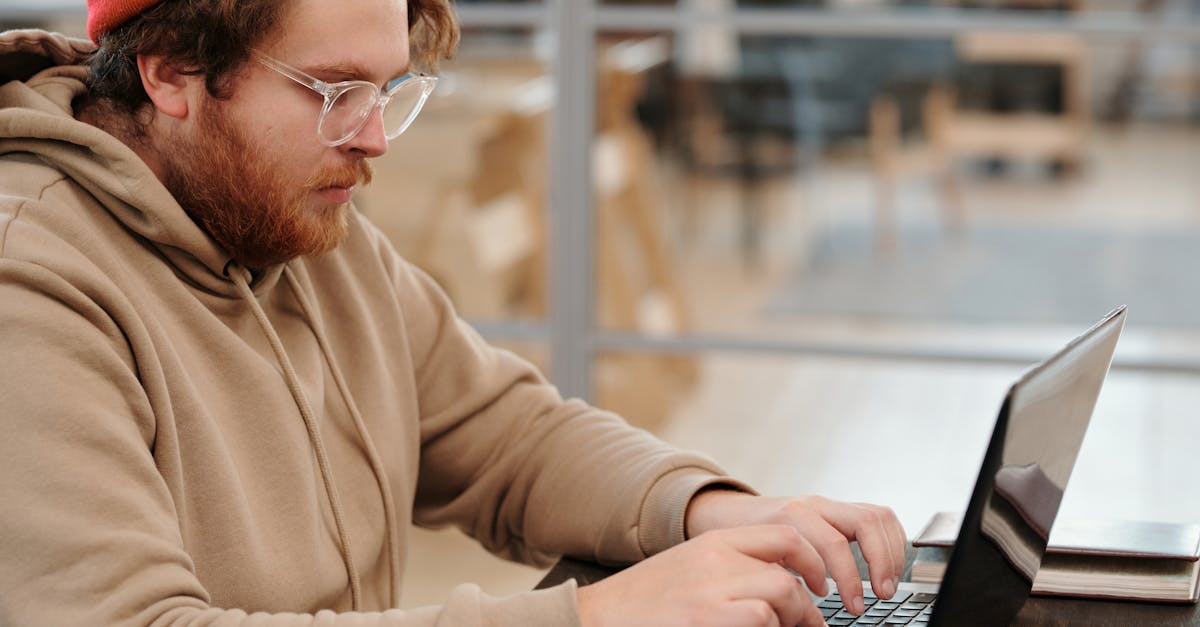
Xamarin is a popular technology that allows developers to build cross-platform applications using .NET and C#. One important aspect of modern application development is creating a responsive user interface (UI) that adapts to different screen sizes and orientations. With Xamarin's support for responsive design, developers can ensure their apps look and function well across various devices.
Responsive UI Design with Xamarin
Responsive UI design aims to create user interfaces that automatically adjust and adapt to the user's device. By implementing responsive UI design in Xamarin applications, developers can provide a consistent user experience across different platforms, such as Android, iOS, and Windows.
Xamarin has built-in features and tools that simplify the development of responsive UIs. Developers can leverage Xamarin.Forms, which is a UI toolkit that allows for the creation of shared UI code across multiple platforms. Xamarin.Forms provides a flexible layout system that enables the UI to respond to screen changes, ensuring optimal usability and visual appeal.
// Example of a responsive layout using Xamarin.Forms ```csharp var stackLayout = new Xamarin.Forms.StackLayout { Orientation = Xamarin.Forms.StackOrientation.Vertical, HorizontalOptions = Xamarin.Forms.LayoutOptions.Center, VerticalOptions = Xamarin.Forms.LayoutOptions.Center, Padding = new Xamarin.Forms.Thickness(20), Spacing = 10, }; var label = new Xamarin.Forms.Label { Text = "Hello, Xamarin!", FontSize = Device.GetNamedSize(Xamarin.Forms.NamedSize.Large, typeof(Xamarin.Forms.Label)), }; var button = new Xamarin.Forms.Button { Text = "Click Me", }; stackLayout.Children.Add(label); stackLayout.Children.Add(button); this.Content = stackLayout; ```
In the example above, a responsive layout is created using Xamarin.Forms' StackLayout. The StackLayout is set to center its contents and includes padding and spacing to enhance the UI's appearance. The label and button are added to the layout, and the layout is set as the content for the page. As the device's screen size or orientation changes, the UI elements within the StackLayout will adapt accordingly.
The Benefits of Responsive UI Design
Responsive UI design offers several benefits for Xamarin application development:
- Improved User Experience: Responsive UI ensures that the app looks and functions well on different devices. Users can have a consistent experience, regardless of whether they use a phone, tablet, or desktop.
- Time and Cost Efficiency: With Xamarin.Forms' shared UI code, developers can save time and effort spent on designing separate UIs for each platform. This results in cost savings and quicker development cycles.
- Scalability: Responsive UI design allows applications to scale seamlessly as new devices with varying screen sizes and resolutions become available.
Usage of ChatGPT-4 for Responsive Design in Xamarin
ChatGPT-4, an advanced language model powered by artificial intelligence, can be employed within Xamarin applications to generate instructions and respond to user commands for responsive design. By integrating ChatGPT-4, developers can enhance the responsiveness of their UIs in real-time, providing dynamic user experiences based on user input and preferences.
ChatGPT-4 can offer suggestions for layout adjustments, provide guidance on handling different screen sizes, and even assist in automating responsive design tasks. Developers can integrate ChatGPT-4 in the Xamarin application's backend to deliver personalized and intelligent adaptive UIs.
Conclusion
Xamarin, combined with responsive UI design principles, allows developers to create applications that provide a seamless user experience across various platforms and devices. By utilizing Xamarin.Forms and its built-in responsive layout system, developers can save time and efforts, ensure consistent usability, and future-proof their applications. Additionally, integrating ChatGPT-4 within Xamarin applications can further enhance the responsiveness and adaptiveness of the UIs, providing dynamic and personalized experiences to the users.
Article written by: Your Helpful Assistant
Comments:
Great article! I found it very informative and well-written.
I agree, the article explains the importance of responsive UI design really well.
As a Xamarin developer, this article is incredibly helpful. Thanks for sharing!
I'm new to Xamarin, but this article gave me a good understanding of how to improve user experiences using ChatGPT and responsive UI design.
The examples provided in the article were really useful. I'll definitely apply these principles in my projects.
Thank you all for your positive feedback! I'm glad you found the article helpful.
I have a question regarding UI responsiveness. How do you handle complex UI layouts while maintaining responsiveness?
Great question, Frank! In my experience, breaking down the UI into smaller, reusable components and utilizing threading to handle heavy computations helps maintain responsiveness.
Thanks, Ivan! I'll try implementing those techniques in my next project.
The article also highlights the importance of considering different screen sizes and orientations. That's crucial for providing a seamless experience across devices.
Absolutely, Grace! Responsive UI design ensures that the app adapts well to various screen resolutions and orientations.
I loved the practical tips mentioned in the article. It's always helpful to have a checklist while designing and testing for responsiveness.
The article provides a comprehensive understanding of how to leverage ChatGPT for improving user experiences. Thanks for sharing!
Being a Xamarin developer, I found this article incredibly practical and insightful. Thank you!
I had heard about Xamarin before, but this article really demonstrates its power in enhancing user experiences.
The article breaks down the implementation steps well. It's a great resource for beginners like me.
The examples provided in the article really bring the concepts to life. Kudos to the author!
Responsive UI design is essential in today's mobile-driven world. This article offers helpful insights.
The article clearly explains how to create a delightful user experience through thoughtful UI design. Well done!
I would also suggest utilizing async and await patterns to keep the UI responsive while performing long-running operations.
Thanks for the suggestion, Peter! I'll keep that in mind.
I agree with Peter. Utilizing async programming techniques allows you to keep the UI responsive during long-running operations by leveraging background threads.
I implemented the techniques you suggested, Ivan. They worked like a charm! Thanks again.
You're welcome, Frank! I'm glad it worked out for you.
The article's emphasis on user feedback and continuous iteration aligns well with developing user-centered applications.
I appreciate how the article combines ChatGPT and Xamarin to improve user experiences. Definitely worth implementing!
True, Robert! The article not only addresses responsiveness but also covers other important aspects of UI design.
Indeed, Susie! It's crucial for developers to have a holistic approach towards user experience.
The article addresses common challenges faced by Xamarin developers and provides practical solutions.
The tips and best practices mentioned in the article are applicable not just to Xamarin, but also to other mobile development frameworks.
Yes, Tom! The concepts discussed in the article can be applied to various mobile development frameworks, making it even more valuable.
I loved how the article emphasized the importance of user testing. It's crucial to gather feedback and make iterative improvements.
Absolutely, Victoria! User testing helps identify pain points and refine the user experience.
I'm glad to see a lively discussion here! Feel free to ask any questions related to the article. I'm here to help.
The article showcases the power of Xamarin and ChatGPT in creating intuitive and responsive apps. Well-written!
As a fellow Xamarin developer, this article resonates with me. It's always good to stay updated on UI design trends.
Definitely, David! This article provides practical tips to enhance the user experience while leveraging Xamarin and ChatGPT.
I loved the way the article explained the benefits of responsive UI design and its impact on user satisfaction.
The article has given me clear guidance on how to implement responsive UI design in Xamarin projects. Thank you!
The code snippets provided in the article are really helpful for implementing the concepts discussed.
I appreciate how the article highlights the importance of clear and concise error handling in responsive UI design.
I'm glad the article was helpful to you too, Henry!
Thank you, Ivan and Peter! I will explore async programming to ensure better responsiveness.
The article presents a clear roadmap for implementing responsive UI designs in Xamarin projects. Thank you for sharing the knowledge!
This article has motivated me to enhance my Xamarin apps with responsive UI design. It's definitely a game-changer!
The examples provided in the article make it easier to understand the concepts and apply them in real-world scenarios.
The article's explanation of accessibility considerations in responsive UI design is commendable. It ensures inclusivity for all users.
Thank you all for your contributions to the discussion. If you have any further questions, feel free to ask!
The article not only covers the technical aspects but also emphasizes the importance of creating delightful user experiences. Well-rounded content!
The article was a great read. It inspired me to prioritize responsive UI design in my Xamarin projects.