Integrating ChatGPT in the Redux Community Guidelines: Enhancing the Redux Technology Experience
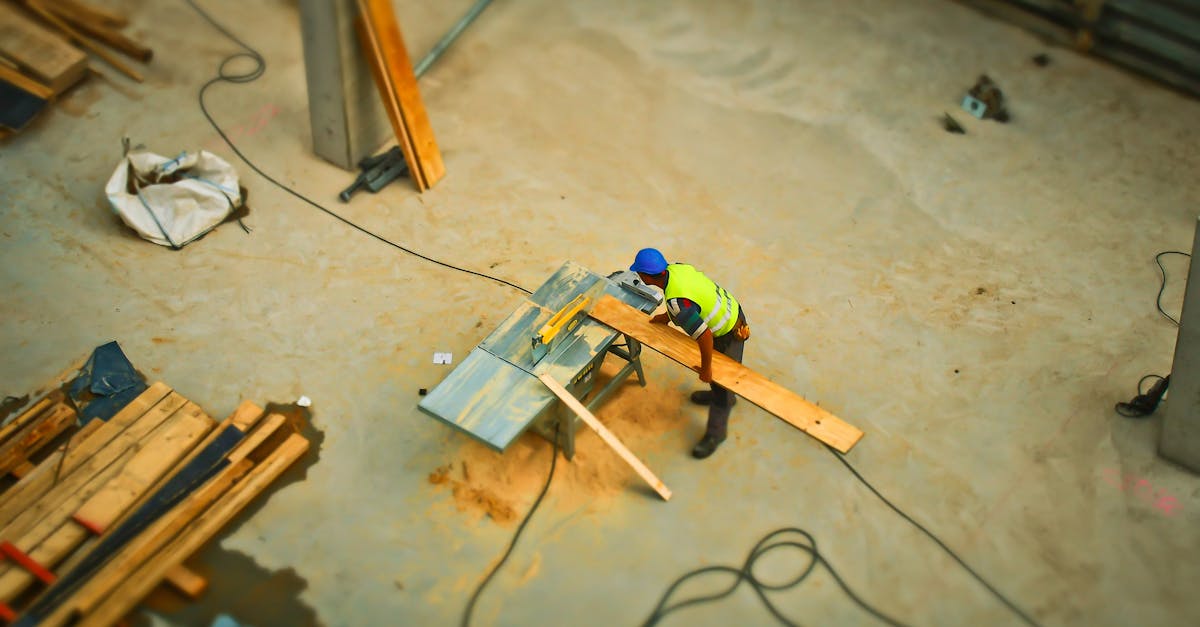
Welcome to the Redux community! If you're new to Redux or want to ensure you're following the best practices established by the community, this article will provide you with the necessary details. Whether you're using Redux in your personal projects or for enterprise-level applications, adhering to these guidelines will promote consistency, maintainability, and readability of your Redux codebase.
What is Redux?
Redux is a predictable state container for JavaScript applications. It is commonly used with React but can be used with other frameworks or libraries. Redux helps manage the state of an application in a centralized store, making it easier to reason about how data changes over time.
Redux Community Guidelines
The Redux community has established a set of guidelines and best practices to ensure that developers can collaborate effectively and build scalable and maintainable Redux applications. Here are some key principles:
1. Single Source of Truth
In Redux, the state of your whole application is stored in an object tree within a single store. This ensures that your application has a single source of truth, making it easier to understand and debug state changes.
2. Read-only State
The state in Redux is read-only. The only way to modify the state is by dispatching actions. This reduces the chances of introducing hard-to-track bugs caused by accidentally mutating the state.
3. Pure Functions and Immutable Updates
In Redux, reducers are pure functions that take the current state and an action and return a new state object. It is crucial to keep your reducers pure and avoid mutating the state directly. Instead, make use of immutable data structures or utility libraries like Immutable.js or Immer.
4. Use Action Creators and Async Actions
Action creators are functions that create and return actions. By using action creators, you can centralize your action creation logic and make it easier to test and reuse. Async actions allow you to handle asynchronous operations such as fetching data from an API and dispatching multiple actions in response.
5. Structuring Your Redux Code
Organizing your Redux code is essential for maintainability. Divide your code into separate files for actions, reducers, and selectors. Consider using the Ducks pattern or feature-based directory structure to keep related code in one place.
6. Middleware for Extensibility
Redux middleware provides a way to extend the capabilities of Redux by intercepting and modifying actions or adding custom behavior to the dispatch process. Popular middleware includes Redux Thunk for handling async actions and Redux Logger for logging actions and state changes.
Conclusion
Following the Redux community guidelines and best practices outlined in this article will help you write clean, maintainable, and scalable Redux code. Remember, the goal is to make your code more readable, predictable, and easier to collaborate with other developers.
Now that you have a better understanding of the Redux community guidelines and best practices, you can confidently build Redux-powered applications and contribute to the growing Redux ecosystem.
Comments:
Thank you for taking the time to read my article on integrating ChatGPT in the Redux Community Guidelines! I'd love to hear your thoughts and opinions on this topic.
This is a great article, Rene! I think integrating ChatGPT into the Redux Community Guidelines could enhance the overall technology experience. It could help users troubleshoot issues and get more personalized support.
I'm not entirely convinced about integrating ChatGPT in the Redux Community Guidelines. It might introduce more complexities and require additional maintenance. What do you think, Rene?
That's a valid concern, Joshua. While integrating ChatGPT would require some maintenance, it has the potential to improve user experience by providing instant assistance. We could find a balance by implementing it in a controlled manner.
I agree with Melissa. ChatGPT could greatly benefit Redux users, especially those who are new to the technology. It would be like having a virtual mentor.
I can see the potential benefits of integrating ChatGPT, but we should also consider potential downsides. How can we address security and privacy concerns that come with using AI-powered chatbots?
Excellent point, Peter! Addressing security and privacy concerns is crucial. We would need to implement strict measures to protect user data and ensure that the chatbot doesn't compromise sensitive information.
I think ChatGPT integration in Redux Community Guidelines would be a game-changer. It could help users find solutions faster and improve the overall support system.
The idea of integrating ChatGPT in Redux Community Guidelines is interesting, but I worry that it might lead to over-reliance on AI instead of encouraging users to develop problem-solving skills on their own.
That's a valid concern, Michael. The goal is to provide supplemental assistance while still encouraging users to understand the technology. ChatGPT can act as a guide, not a crutch.
I'm a Redux beginner, and I think having ChatGPT integrated with the community guidelines would be a huge help. It would make it easier to find answers to my questions without spending hours searching through documentation.
Thanks for sharing your perspective, Olivia! As a beginner, having a chatbot to provide guidance and answer your questions would definitely be valuable.
While AI-powered chatbots can be helpful, they lack human touch and understanding. Sometimes, a real person's response can address a user's concerns more effectively.
I agree with you, David. AI can never fully replace human interaction. A combined approach of human support and AI chatbot assistance might be better.
The idea of having ChatGPT integrated in Redux Community Guidelines sounds intriguing. It could make the learning process more interactive and engaging.
Absolutely, Marissa! An interactive learning experience would be a huge advantage for Redux users.
This article raises important points about the benefits of integrating ChatGPT. However, we should also consider the costs involved and the potential impact on the community's resources.
You're right, Susan. The costs and impact on resources need to be taken into account. It would require careful planning and allocation of resources to ensure the community can handle the integration.
The integration of ChatGPT in Redux Community Guidelines could be a valuable addition. It would provide a centralized knowledge base accessible anytime.
Exactly, Alex! Having a centralized knowledge base with an intelligent chatbot would make it easier for users to access relevant information.
I think the integration of ChatGPT could be beneficial, but we should also be cautious about potential biases and misinformation that AI chatbots might propagate.
That's a valid concern, Sarah. To mitigate biases and misinformation, the chatbot's responses would need to go through rigorous testing and validation before being integrated.
Integrating ChatGPT could also provide an opportunity for the community to give feedback and enhance the chatbot's responses over time.
Absolutely, Nathan! Feedback from the community would be invaluable in improving the chatbot's performance and refining its responses.
I'm excited about the potential of integrating ChatGPT. It could make the Redux experience more user-friendly and accessible for everyone.
Although ChatGPT integration seems promising, we shouldn't overlook the potential impact on the community's inclusivity. Some users may not be comfortable interacting with a chatbot.
Good point, Michelle. We need to ensure that the chatbot doesn't alienate users who prefer human interaction. Making it optional could be one possible solution.
ChatGPT integration might give Redux an edge over other technologies. It shows the community's commitment to innovation and user experience enhancement.
Exactly, Adam! Staying ahead of the curve and embracing innovative solutions can help Redux maintain its relevance and attract a wider user base.
I'm all for integrating ChatGPT. It could save a lot of time for both users and the support team by providing instant answers to common questions.
Indeed, Jennifer! Faster resolution of common questions would lead to improved productivity and more efficient support for the Redux community.
ChatGPT integration could also help bridge the gap between developers and non-technical users. It could provide clearer explanations and make the technology more accessible.
Absolutely, Brian! Making Redux more accessible to non-technical users is an important goal, and an AI-powered chatbot can play a crucial role in achieving that.
I'm concerned about the potential misuse of AI chatbots by spammers or trolls. How do we prevent them from exploiting the system?
That's a valid concern, Lucy. Implementing robust moderation tools and incorporating user feedback can help in identifying and mitigating misuse of the chatbot.
ChatGPT integration sounds exciting, but let's not forget the importance of maintaining up-to-date documentation alongside the chatbot.
Absolutely, Daniel! The chatbot should complement, not replace, the documentation. Keeping the documentation up-to-date will remain a priority.
I'm curious about how the integration of ChatGPT would be implemented. Would it be separate from the Redux website or fully integrated within it?
Great question, Anna! While the specifics would need to be determined, ideally, the integration would be seamless within the Redux website to provide a cohesive user experience.
Integrating ChatGPT in Redux Community Guidelines could be a significant step forward. It's worth exploring further and piloting in a controlled environment.
I'm glad you think so, Samuel! Piloting the integration in a controlled environment would help us assess its effectiveness and fine-tune the implementation.
I see the potential benefits of ChatGPT integration, but we should also be prepared for the learning curve associated with training the chatbot effectively.
Indeed, Sophia! Training the chatbot to understand the nuances of Redux and provide accurate responses can be a challenge, but it's a worthwhile endeavor.
ChatGPT could be useful when it comes to debugging and troubleshooting issues. It could save a lot of time for both developers and support staff.
Absolutely, Jacob! Enabling users to troubleshoot issues with the help of the chatbot can streamline the support process and reduce the burden on support staff.
I think integrating ChatGPT would bring the Redux community closer together. Users could interact, share knowledge, and help each other through the chatbot.
That's a great perspective, Julia! The chatbot can act as a hub for community interaction and foster collaboration among Redux users.
I'm excited about the potential of ChatGPT integration. The technology is advancing rapidly, and it's important for Redux to stay at the forefront of these developments.
You're absolutely right, Michael! Embracing cutting-edge technologies like ChatGPT can help Redux remain relevant and future-proof.
Thank you all for your insightful comments and thoughtful discussion! Your feedback has given me much to consider as we explore the possibility of integrating ChatGPT into the Redux Community Guidelines.