Leveling Up State Management: Leveraging ChatGPT for Redux Technology's State Management Advice
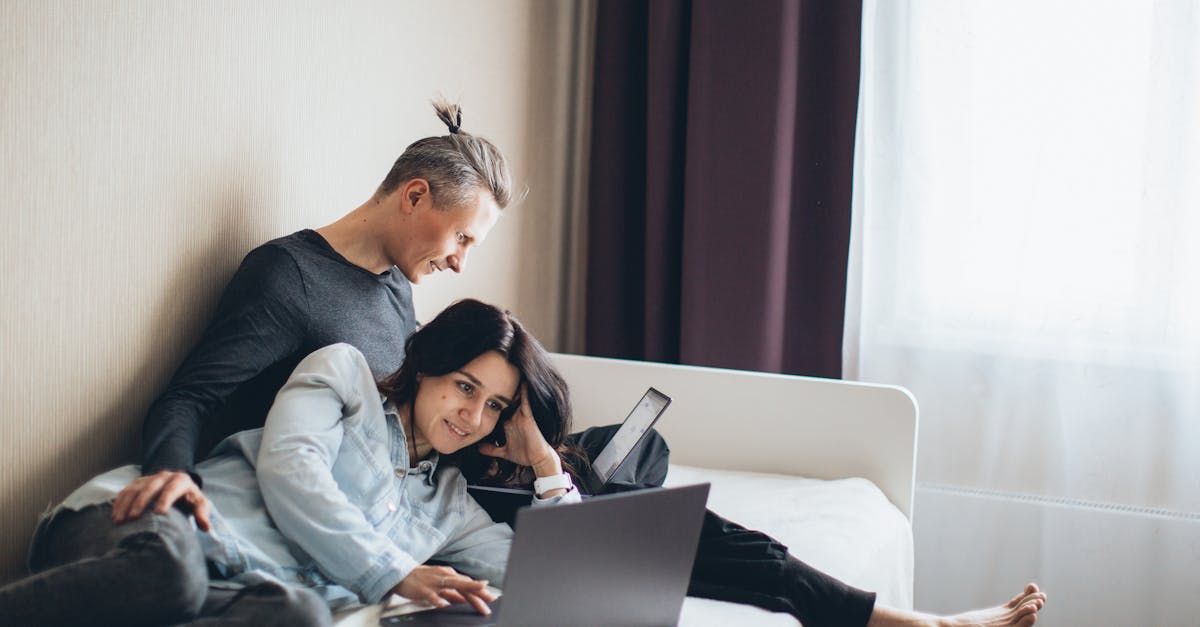
Redux is a popular state management library used primarily in JavaScript applications. It provides a predictable state container for managing the application state efficiently. In this article, we will discuss best practices and advice for managing state in Redux based on different contexts.
1. Keep the Store Structured
One of the key principles of Redux is to have a single source of truth in the application state. To achieve this, it is essential to keep the store structured in a logical manner. Divide the state into smaller and independent modules, each responsible for managing specific parts of the application. This modular approach helps in better organization and scaling of the application.
2. Normalize State Shape
When designing the state shape, it is advisable to normalize the data structure. This means keeping the data as flat as possible and avoiding nested objects or arrays. Normalizing the state makes it easier to update and query the data efficiently. Additionally, it simplifies the logic for handling updates and reduces the chance of bugs in the application.
3. Use Immutable Data
Redux encourages the use of immutability for state updates. Instead of directly mutating the state, create a copy of the previous state and make modifications on the new copy. This ensures that the state remains unchanged and facilitates better tracking of changes. Immutability also improves performance by leveraging reference equality checks.
4. Use Selectors for Data Retrieval
To avoid tightly coupling the components with the state structure, use selectors to retrieve data from the Redux store. Selectors are functions that encapsulate the access to specific parts of the state. By utilizing selectors, the components can remain unaware of the internal state structure changes, providing a cleaner and more maintainable codebase.
5. Use Middleware for Side Effects
Redux middleware can be employed to handle side effects, such as making API calls or dispatching additional actions. Middleware intercepts and allows modifications to the actions before they reach the reducers. This separation of concerns not only keeps the reducers pure but also provides a centralized place to manage side effects, leading to a more scalable and testable state management solution.
6. Optimize Performance
As the Redux store grows, it is crucial to optimize its performance. Avoid storing unnecessary data in the state to minimize memory usage. Use memoization techniques to prevent unnecessary re-renders in the components that depend on the state. By identifying and addressing performance bottlenecks, you can ensure a smoother and more responsive user experience.
7. Debugging with Redux DevTools
Redux DevTools is a browser extension that provides a powerful set of tools for debugging and inspecting the application state. It allows you to replay actions, revert state changes, and track the execution flow. Leveraging the capabilities of Redux DevTools simplifies the debugging process and greatly aids in identifying and resolving state-related issues.
Conclusion
Effective state management is crucial for building scalable and maintainable applications. The best practices outlined above provide a solid foundation for managing the state in Redux-based projects. By adhering to these practices, developers can ensure a cleaner, more efficient, and better-maintained state management solution, resulting in more robust applications.
Comments:
Thank you for reading my article on leveraging ChatGPT for Redux Technology's state management advice. I hope you found it insightful!
Great article, Rene! I especially liked how you explained the benefits of using ChatGPT for state management. It seems like an interesting approach to improving the development process.
I agree, Anna! The idea of using language models like ChatGPT for state management is quite innovative. It would be interesting to see how it compares to more traditional approaches like Redux.
I have some concerns about relying solely on ChatGPT for state management. What if it doesn't understand the context or gives incorrect suggestions?
That's a valid concern, Natalie. ChatGPT is powerful, but it does have limitations. It's important to carefully evaluate and validate the suggestions it provides to ensure they align with the desired state management goals.
Interesting article, Rene! Do you have any recommendations on how to effectively integrate ChatGPT for state management in an existing Redux codebase?
Thanks, Mark! Integrating ChatGPT with an existing Redux codebase may require some modifications. It's important to assess the specific needs of the project and identify the areas where ChatGPT can provide value. Gradual integration and testing can help uncover potential issues or conflicts.
I'm curious about the performance implications of using ChatGPT for state management. Will it introduce any noticeable overhead?
Good question, Lisa! ChatGPT poses performance considerations, especially for real-time applications. It's crucial to evaluate the response time and resource requirements of the model to ensure it doesn't impact the responsiveness of the system.
I'm not convinced that ChatGPT is suitable for state management. It seems like a complex solution for a problem that can be resolved with simpler approaches like Redux or MobX.
I understand your point, Michael. While traditional state management libraries like Redux or MobX are widely adopted, exploring alternative approaches can lead to new insights and possibilities. ChatGPT offers a different perspective worth considering.
I find ChatGPT fascinating, but I worry about the risks associated with relying on large language models. Security and privacy concerns come to mind. What are your thoughts on that, Rene?
Valid concern, Sophia. Security and privacy should always be a priority when working with large language models. It's essential to implement measures like data sanitization, proper access controls, and review processes to mitigate potential risks.
I see potential in using ChatGPT for state management, but how do you deal with the model's limitations in understanding domain-specific jargon or custom project requirements?
Great question, Peter! While ChatGPT is trained on a diverse range of internet text, it may struggle with specific jargon or custom requirements. One approach is to fine-tune the model using domain-specific data or provide additional context to improve its understanding.
Interesting concept, Rene! However, I wonder if using ChatGPT for state management could lead to code ambiguity or inconsistency due to the subjective nature of language processing. How do you address this concern?
Good point, Emily! Ensuring clarity and consistency in code is crucial. Proper documentation, clear coding standards, and a review process can help address the subjectivity of language processing and maintain code quality when leveraging ChatGPT for state management.
I'm not convinced that ChatGPT is reliable enough for state management. We can't solely rely on AI models for such critical components. What are your thoughts, Rene?
I understand your concern, Robert. ChatGPT is not meant to replace critical decision-making processes in state management. It's meant to assist and offer suggestions. It's essential to balance the use of AI with human expertise to ensure reliability.
I'm interested in trying out ChatGPT for state management in my next project. Are there any specific tools or libraries you recommend for implementing ChatGPT in a Redux-based application?
That's great to hear, Daniel! When integrating ChatGPT with Redux, you can utilize libraries like 'redux-api-middleware' or 'redux-saga' for handling asynchronous interactions with the model. These tools can help streamline the integration process.
I'm concerned about the ethical implications of using ChatGPT for state management. How do we ensure the model doesn't propagate biased or discriminatory suggestions?
Ethical considerations are paramount, Laura. When training and fine-tuning ChatGPT, it's important to use diverse and representative datasets, address biases in the training data, and implement evaluation mechanisms to ensure fair and unbiased suggestions.
I enjoyed reading your article, Rene. Have you compared ChatGPT's performance with other state management libraries in terms of development time or learning curve?
Thank you, Gregory! It's challenging to make direct performance comparisons between ChatGPT and traditional state management libraries. They serve different purposes, and the evaluation criteria can vary. However, you can assess the development time and learning curve based on your specific project requirements.
I worry about the scalability of ChatGPT for state management. Will it handle large-scale applications or complex systems efficiently?
Scalability is an important consideration, Jennifer. The scale of the application and the specific implementation details can impact ChatGPT's efficiency. It's advisable to conduct performance tests and monitor resource usage to ensure it meets the requirements of your application.
I find the idea of using ChatGPT for state management intriguing. It could potentially simplify development and reduce the need for explicit code changes. What are your thoughts, Rene?
I agree, Erica! The ability of ChatGPT to understand and generate code-related interactions could indeed simplify development tasks and reduce manual code changes. It opens up possibilities for more intuitive and interactive programming experiences.
This article presents an interesting alternative approach. However, I'm concerned about the learning curve and the effort required to train the model for specific state management needs. Could you shed some light on that, Rene?
Valid concern, Lucas. Training the model for specific state management needs may require effort and domain knowledge. Utilizing transfer learning and fine-tuning techniques can help reduce the training effort, but it's important to assess the trade-offs and scope of the desired interaction capabilities.
I'm excited about the potential of ChatGPT for state management! It could unlock new possibilities and inspire more interactive development workflows. Are there any community resources or tutorials you recommend to explore this further?
I share your excitement, Alex! To explore ChatGPT for state management further, you can check out the OpenAI forums, GitHub repositories, and resources on AI-assisted development. They provide valuable insights and examples contributed by the community.
I'm concerned about the potential limitations of ChatGPT in understanding complex state management requirements. Can it handle complicated scenarios effectively?
Complex state management scenarios can pose challenges, Olivia. While ChatGPT's capabilities are impressive, there may be scenarios where it struggles to grasp the intricacies. Iterative testing, feedback loops, and incorporating expert knowledge can help close the gap in understanding complex requirements.
Great article, Rene! I can see the potential of using ChatGPT for state management. It promotes a more conversational and interactive development experience. I'm excited to see how it evolves!
I'm a traditionalist when it comes to state management, but this article piqued my curiosity. The integration of AI in the development process is fascinating!
I'm intrigued by the concept of leveraging ChatGPT for state management, but I worry about the long-term maintenance and support. What if the model becomes obsolete or has compatibility issues?
Long-term maintenance and support are valid concerns, Liam. AI models can evolve, and compatibility issues may arise. It's crucial to keep an eye on updates, engage with the community, and have contingency plans to adapt or transition if needed.
Thank you for sharing your insights, Rene! Your article provided a unique perspective on state management and highlights the exciting possibilities of AI in development.
I'm still skeptical about the practicality of using ChatGPT for state management. The potential challenges seem too significant to overcome. How do you address those concerns, Rene?
I understand your skepticism, Chloe. Challenges exist, and the suitability of ChatGPT for state management depends on the specific requirements of each project. By carefully assessing trade-offs, validating suggestions, and balancing AI with conventional approaches, the concerns can be addressed to a great extent.
Great article, Rene! The idea of using ChatGPT for state management opens up new frontiers in software development. I look forward to exploring its potential!
I'm excited to see how the integration of language models like ChatGPT can reshape state management. Your article offered an intriguing glimpse into the future of development!
Interesting approach, Rene! I can see the benefits of using ChatGPT for state management, especially for rapidly evolving projects where maintaining traditional state management can become challenging.
It's fascinating to see the intersection of AI and software development expanding. Your article introduced an innovative use of ChatGPT in state management, Rene. Well done!
The topic of using ChatGPT for state management is intriguing. It highlights the potential for AI to augment the development process and provide new insights. I enjoyed reading your article, Rene!
ChatGPT's integration into state management is an interesting concept. It opens up possibilities for more intuitive and interactive programming experiences. Thank you for sharing your thoughts, Rene!
I appreciate your article, Rene. It made me think about the future potential of AI-assisted state management. ChatGPT provides an exciting approach worth exploring further!
As someone passionate about both AI and software development, I'm delighted to read about incorporating ChatGPT into state management. The possibilities are exciting, and your article offered valuable insights, Rene!