Mastering Efficiency: Transforming Lazy Loading in Angular with ChatGPT
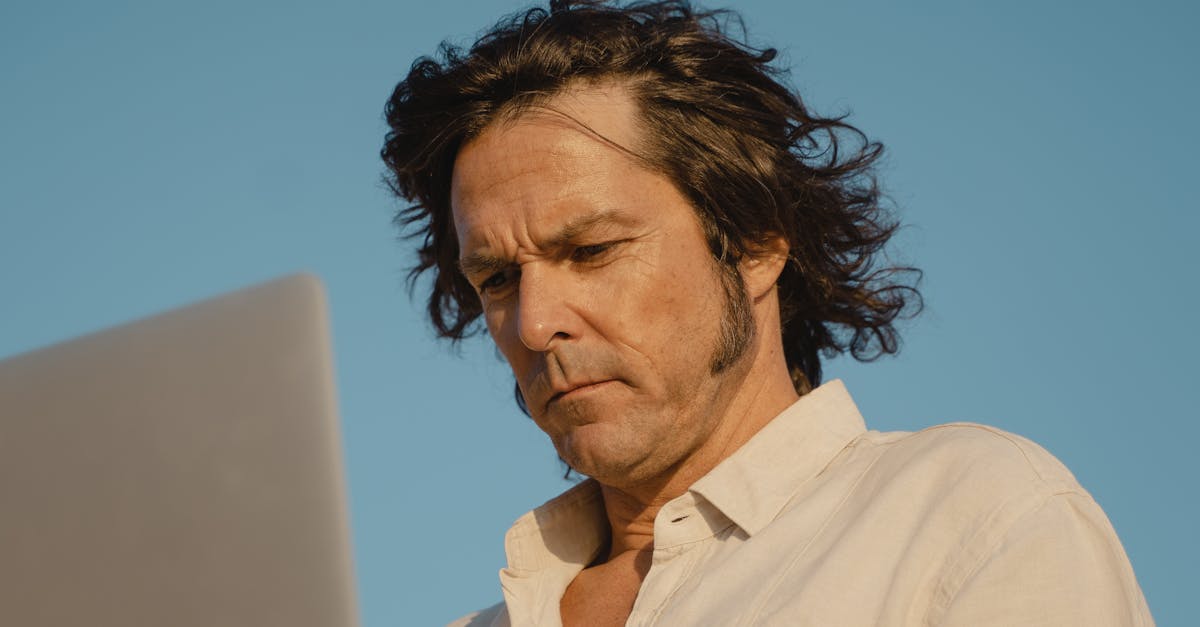
Lazy loading is a technique used in web development to improve performance by loading only the required resources when needed. In Angular, lazy loading allows developers to load a module or component on-demand, reducing the initial load time and optimizing the user experience.
What is Angular?
Angular is a popular TypeScript-based open-source framework for building web applications. It provides a set of tools and libraries that enable developers to create efficient and scalable applications. Angular follows the component-based architecture, making it easier to manage complex web applications.
What is Lazy Loading?
Lazy loading is a design pattern that defers the loading of resources until they are actually needed. In the context of Angular, lazy loading refers to loading modules or components only when the user navigates to a specific route or performs a particular action.
Why Use Lazy Loading in Angular?
Lazy loading is essential in Angular applications to improve performance and reduce the initial load time. With lazy loading, the browser only needs to load the necessary modules and components when they are required, rather than loading the entire application upfront.
This approach is particularly beneficial for large-scale applications that have multiple modules and complex routing. By using lazy loading, developers can optimize resource usage and provide a snappy user experience.
How to Implement Lazy Loading in Angular
Implementing lazy loading in Angular is straightforward and can be achieved by following a few simple steps:
- Create a new module for the feature or component you want to lazy load.
- Define the routes for lazy loading in the main routing module.
- Load the module or component using the Angular Router's lazy loading syntax.
Example Code
@NgModule({
imports: [
RouterModule.forRoot([
{ path: 'lazy', loadChildren: 'app/lazy/lazy.module#LazyModule' },
// Other routes...
])
],
exports: [
RouterModule
]
})
export class AppRoutingModule { }
Conclusion
Lazily loading modules and components in Angular is a powerful technique for improving performance and optimizing resource usage. By only loading what is necessary, you can significantly reduce the initial load time of your application, resulting in a better user experience. ChatGPT-4 can assist developers in implementing lazy loading in Angular and provide guidance on best practices for efficient application development.
Comments:
Thank you all for visiting my article on Mastering Efficiency: Transforming Lazy Loading in Angular with ChatGPT! I'm excited to hear your thoughts and answer any questions you may have.
Great article, Diego! I found your explanations very clear and easy to follow. Lazy loading can indeed greatly improve the performance of Angular applications.
Thank you, Alice! I'm glad you found the explanations helpful. Indeed, lazy loading can be a game-changer when it comes to performance optimization.
I've been hesitant to implement lazy loading in my Angular project due to concerns about increased complexity. Do you have any tips for managing lazy-loaded modules?
That's a valid concern, Bob. When working with lazy-loaded modules, it's important to have a well-defined module structure and establish clear boundaries. Also, make sure to keep an eye on your module sizes to avoid performance issues.
I've recently started learning Angular, and this article has given me a better understanding of lazy loading. Thank you, Diego!
You're welcome, Eva! I'm glad I could help you grasp the concept of lazy loading. If you have any specific questions, feel free to ask!
Lazy loading is definitely a powerful technique. It significantly improved the loading time of my Angular application. Thanks for the informative article, Diego!
You're welcome, Charlie! I'm thrilled to hear that lazy loading had a positive impact on your application's loading time. Feel free to share any challenges or experiences you encountered during the implementation.
Excellent article, Diego! Do you have any performance benchmarks or real-world examples to showcase the benefits of lazy loading?
Thank you, Grace! While I don't have specific benchmarks handy, I can assure you that lazy loading can greatly improve the initial loading time of large Angular applications by loading only the necessary modules. It's a technique widely adopted by many Angular projects.
Lazy loading does sound interesting, but I'm concerned about the impact on SEO. Can you shed some light on that aspect?
Sure, Daniel. While it's true that lazy loading might affect SEO to some extent, there are ways to ensure search engines can still crawl and index your application's content properly. One approach is to generate static HTML snapshots of the initial pages using tools like Angular Universal. These snapshots can be served to search engine crawlers while enabling lazy loading for regular users.
Lazy loading modules is a must in complex Angular applications. It helps keep the initial bundle size smaller and improves performance. Thanks for sharing your insights, Diego!
Absolutely, Frank! Complex Angular applications can significantly benefit from lazy loading. It's great to hear that you found my insights valuable. If you have any specific use cases or challenges you faced during implementation, feel free to share!
I agree that lazy loading is crucial for large projects, but what about smaller applications? Is it still worth implementing?
Good question, Hannah! While the impact may not be as noticeable in smaller applications, lazy loading can still contribute to better performance, especially if your application has multiple routes or feature-based modules. It's always a good practice to consider scalability from the start.
Lazy loading seems like a valuable technique, but are there any potential drawbacks or trade-offs we should be aware of?
Great question, Isabella! While lazy loading can offer significant benefits, it's essential to be mindful of the module architecture and bundle sizes. Poorly organized modules or large modules might lead to increased complexity and potentially impact the user experience. It's all about finding the right balance for your specific application.
I've been using Angular for a while, but I've never explored lazy loading. Your article has motivated me to give it a try. Thanks, Diego!
That's wonderful to hear, Oliver! Lazy loading can indeed open up new possibilities in optimizing your Angular project. If you have any questions during the implementation, feel free to reach out for assistance.
I enjoyed reading your article, Diego! Lazy loading is an essential technique for better performance and user experience in Angular web applications.
Thank you, Sarah! I'm delighted that you found the article enjoyable. Lazy loading can indeed make a significant difference in performance and user experience. If you have any specific examples or experiences related to lazy loading, feel free to share!
I've been implementing lazy loading in my project, and it has been a game-changer in terms of performance. Excellent article, Diego!
Thank you, Tom! It's fantastic to hear that lazy loading had such a positive impact on your project's performance. If you encountered any challenges or if there's anything specific you'd like to discuss about lazy loading implementation, feel free to share!
Lazy loading is definitely a technique worth considering for Angular applications. It optimizes the loading time and improves the overall user experience. Thanks for sharing your insights, Diego!
Absolutely, Sophia! Lazy loading is a powerful technique that can significantly enhance the loading time and user experience. I'm glad you found my insights valuable. If you have any additional questions or experiences to share, feel free to do so!
I've been using Angular for a while, but I've never dug into the concept of lazy loading. Your article has piqued my interest, Diego!
That's great to hear, Jack! Lazy loading can bring exciting improvements to your Angular projects. If you decide to give it a try or have any questions along the way, feel free to reach out for assistance.
Wonderful article, Diego! Lazy loading has become a must-know technique in Angular development. It really helps in optimizing performance.
Thank you, Emily! I'm thrilled to hear that you enjoyed the article. Indeed, lazy loading is widely adopted for optimizing performance in Angular applications. If you have any specific use cases or questions, feel free to share!
I'm fairly new to Angular, and your article was extremely informative, Diego! Lazy loading seems like a crucial aspect to learn. Thank you!
You're very welcome, Nathan! I'm glad to hear that you found the article informative and that lazy loading sparked your interest. Don't hesitate to ask any questions you may have as you delve into this technique!
I have been considering incorporating lazy loading into my Angular project, and your article clarified its benefits. Thank you, Diego!
You're welcome, Victoria! I'm happy to hear that the article helped you understand the benefits of lazy loading. If you have any specific concerns or questions while implementing it in your project, feel free to ask!
Lazy loading has significantly improved the performance of my Angular application. Thanks for sharing your insights and explaining it so well, Diego!
You're welcome, Lucas! I'm thrilled to hear that lazy loading had a positive impact on your application's performance. If you have any interesting experiences or insights related to lazy loading, feel free to share!
Lazy loading is an essential technique for large-scale Angular projects. It helps in managing resources efficiently. Thank you for the informative article, Diego!
Absolutely, Chloe! Lazy loading can be a game-changer when it comes to efficiently managing resources in large Angular projects. I'm glad you found the article informative. If you have any specific scenarios or questions, feel free to share!
I'm curious if there are any cases where lazy loading might not be suitable or recommended?
Good question, Liam! While lazy loading is generally beneficial, it might not be as critical for small, simple applications with fewer routes and module dependencies. In such cases, the initial bundle size may not be a concern. However, it's always worth assessing your application's scaling potential and considering future feature expansion.
Your article enlightened me about the potential of lazy loading in Angular. Thanks for sharing your knowledge, Diego!
You're welcome, Max! I'm glad the article enlightened you about the potential of lazy loading in Angular. If you have any further questions or specific topics you'd like to explore, feel free to let me know!
Excellent article, Diego! Lazy loading has become an integral part of modern Angular applications. Thank you for shedding light on its implementation.
Thank you, Julia! I'm delighted that you found the article excellent and gained insights into lazy loading's implementation. If you have any additional questions or interesting experiences to share, feel free to do so!
Your article on lazy loading in Angular was very informative, Diego. I appreciate the effort you put into explaining the concept and its benefits.
Thank you, Oscar! I'm glad you found the article informative and benefited from the explanations. If you have any specific questions or need further clarification, feel free to ask!
Lazy loading has helped me improve the performance of my Angular project. Your article provided valuable insights, Diego. Thank you!
You're welcome, Ava! I'm thrilled to hear that lazy loading made a positive difference in your project's performance. If you have any tips or challenges you'd like to discuss regarding lazy loading, feel free to share!
Thank you, everyone, for your engagement and valuable comments. I hope you found the article enlightening. Feel free to reach out with any further questions or insights you'd like to share. Happy coding!