Powering Angular Pipes with ChatGPT: Enhancing Data Transformation and Manipulation
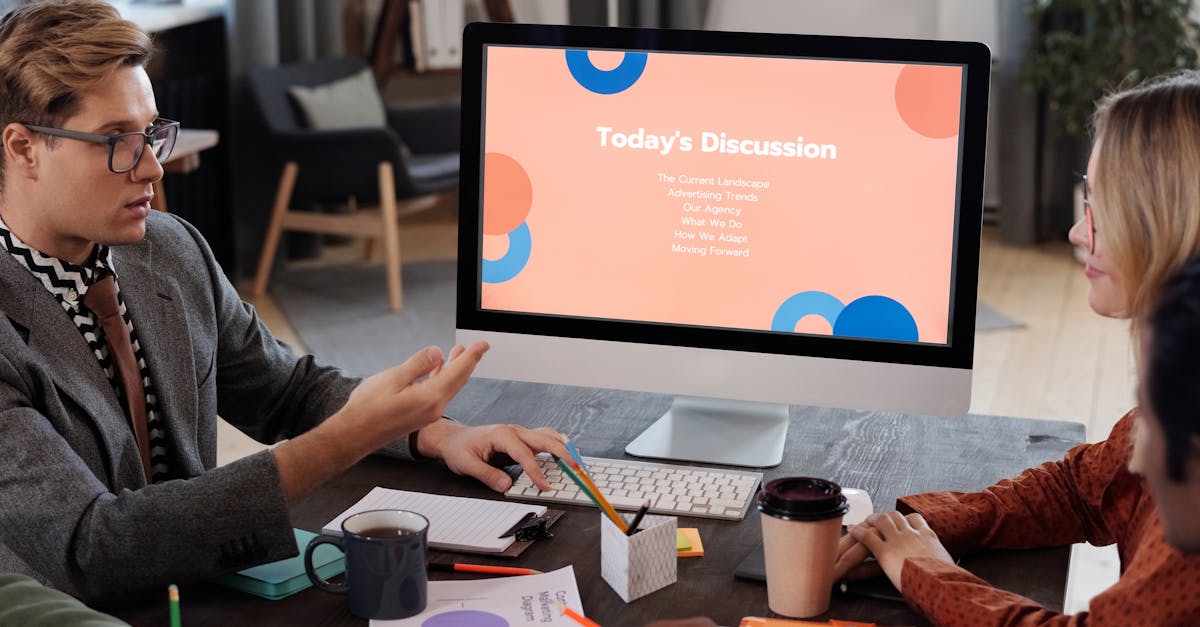
Angular is a popular JavaScript framework that allows developers to build dynamic web applications. One of the key features of Angular is its flexibility in transforming values within templates using pipes.
What are Pipes?
Pipes in Angular are a way to transform or modify data before displaying it in the template. They provide a convenient way to format data, filter arrays, transform strings, and perform various other transformations.
Usage of Pipes in Angular
One common use case for pipes is in formatting dates. Angular provides a built-in DatePipe
that allows you to format dates in various ways. For example, you can display a date in a specific format such as "MMM dd, yyyy" or "yyyy-MM-dd".
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: 'Today is {{ currentDate | date:"MMM dd, yyyy" }}
'
})
export class AppComponent {
currentDate: Date = new Date();
}
This code snippet shows how you can use the DatePipe
to format the current date in your Angular application. By using the pipe in the template, you can easily transform and display the date in the desired format.
Another common use case for pipes is in filtering arrays of data. Angular provides a built-in FilterPipe
that allows you to filter arrays based on specific criteria. For example, you can filter an array of objects based on a certain property value.
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
- {{ user.name }}
`
})
export class AppComponent {
users: User[] = [
{ name: 'John', active: true },
{ name: 'Jane', active: false },
{ name: 'Jake', active: true }
];
}
interface User {
name: string;
active: boolean;
}
In this code snippet, the FilterPipe
is used to filter the array of users based on the value of the "active" property. Only the users with "active" set to true will be displayed in the template.
Implementing Custom Pipes
While Angular provides a set of built-in pipes, you can also create custom pipes to perform specific transformations. Custom pipes allow you to encapsulate complex logic and reuse it across your application.
// capitalize.pipe.ts
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'capitalize'
})
export class CapitalizePipe implements PipeTransform {
transform(value: string): string {
return value.charAt(0).toUpperCase() + value.slice(1);
}
}
In this example, a custom pipe called CapitalizePipe
is created to capitalize the first letter of a given string. You can then use this pipe in your templates to transform strings.
Conclusion
Pipes in Angular are a powerful feature that allows you to transform values before displaying them in templates. Whether you need to format dates, filter arrays, or perform custom transformations, pipes provide a convenient way to achieve these tasks. By understanding the usage and implementing custom pipes, you can enhance the functionality and user experience of your Angular applications.
Comments:
Thank you all for reading my article on Powering Angular Pipes with ChatGPT! I hope you found it informative.
Great article, Diego! I really enjoyed learning about using ChatGPT to enhance data transformation in Angular pipes. It seems like a powerful tool.
Completely agree, Jessica! ChatGPT can certainly revolutionize the way we manipulate data in Angular pipes. Thanks for sharing, Diego!
I've been using Angular for a while now, but I hadn't thought about leveraging NLP models like ChatGPT for data transformation. This post opened my eyes to new possibilities. Thanks, Diego!
You're welcome, Sara! It's always great to help fellow developers discover new ways to improve their workflows with Angular.
ChatGPT seems like a game-changer! Can you provide more examples of how it can be used in Angular pipes, Diego?
Of course, Mark! With ChatGPT, you can perform tasks like sentiment analysis, language translation, and even content generation within Angular pipes. It opens up a wide range of possibilities!
Wow, I didn't realize Angular pipes could be taken to this level. It's incredible how AI can enhance our frontend development workflows. Thanks for sharing this, Diego!
I agree, Nina. Integrating AI capabilities into Angular pipes can significantly improve our development efficiency. Thanks for the article, Diego!
This is fascinating, Diego! I'm excited to explore using ChatGPT in my Angular projects. Thanks for the insightful article!
You're welcome, Michelle! Feel free to reach out if you have any questions while integrating ChatGPT into your Angular projects. Happy coding!
The possibilities with ChatGPT in Angular pipes are indeed impressive! Diego, are there any limitations we should be aware of when using it?
Great question, Alex! While ChatGPT is powerful, it's important to be mindful of potential bias and context-related limitations. Additionally, working with large datasets may require optimizations. It's crucial to thoroughly test and monitor its performance!
Diego, thanks for the valuable insights. I really appreciate that you pointed out the potential limitations. It's vital to be aware of those when integrating AI models into our pipelines.
Absolutely, Eva! Being conscious of both the possibilities and limitations of AI models helps us make informed decisions and ensure the best outcomes. Happy to provide insights!
Wonderful article, Diego! It's inspiring to see how AI can revolutionize Angular development. Can you recommend any resources to further explore integrating ChatGPT in Angular?
Thank you, Robert! To further explore integrating ChatGPT in Angular, I suggest checking out OpenAI's documentation and sample projects available online. They provide a wealth of information and examples to get started.
Diego, your article shed light on a whole new world of possibilities. I had no idea ChatGPT could transform data in Angular pipes. I'm excited to experiment with it!
Thank you, Emily! The power of imagination combined with the capabilities of AI can lead to amazing innovations. Best of luck with your experiments!
Great article, Diego! I'm curious, though, if there are any performance considerations when integrating ChatGPT into Angular pipes?
Absolutely, Liam. While ChatGPT provides powerful functionality, it's important to consider performance impact. Processing large amounts of data can introduce latency. It's recommended to benchmark and optimize your implementation based on the specific requirements of your Angular projects.
Thank you, Diego, for emphasizing the performance aspect. It's crucial to strike a balance between functionality and efficiency when integrating AI models like ChatGPT into our frontend workflows.
Well said, Eric! Ultimately, finding that balance is key to delivering high-quality user experiences while leveraging the capabilities of AI models. Thanks for your comment!
Diego, I loved your article! It's amazing how AI technologies like ChatGPT can transform the way we work with data in Angular pipes. Thank you for sharing your expertise!
Thank you, Laura! I'm thrilled to hear that you found the article informative. AI technologies indeed provide exciting opportunities in the world of Angular development. It's my pleasure to share my expertise!
Excellent read, Diego! ChatGPT holds tremendous potential for enhancing data manipulation in Angular. Can't wait to explore it further.
Thank you, Gregory! I share your excitement for the potential of ChatGPT in Angular. It's a remarkable tool that can empower developers to do more with their data transformations. Enjoy exploring it further!
Diego, your article was a great read. It's fascinating how AI can unlock new possibilities in frontend development. Thanks for sharing your knowledge!
You're very welcome, Sophie! AI is indeed transforming the way we approach frontend development, and it's exciting to explore the potential it holds. I'm glad you enjoyed the article!
This article caught my attention, Diego. Incorporating ChatGPT into Angular pipes seems like an innovative approach. How does it compare to traditional data manipulation techniques?
Great question, Jerry! ChatGPT offers new ways to manipulate and transform data, especially when dealing with unstructured text or language-related tasks. Compared to traditional techniques, it introduces powerful NLP capabilities that can greatly enhance your data manipulation workflows.
Diego, your article was a real eye-opener! Angular pipes paired with ChatGPT can bring remarkable possibilities. Thanks for sharing this insightful piece!
Thank you, Stella! It's always rewarding to hear that my article brought new insights. Angular pipes with ChatGPT indeed offer exciting possibilities for data manipulation. Happy to share the insights!
Diego, this was a fascinating read! It's incredible to think about the potential of using ChatGPT for data manipulation. Can it be seamlessly integrated into existing Angular projects?
Absolutely, Maxwell! ChatGPT can be integrated into existing Angular projects without much hassle. OpenAI provides comprehensive documentation that guides you through the integration process. Give it a try!
I'm glad I stumbled upon your article, Diego! ChatGPT seems like a powerful tool for transforming and manipulating data in Angular pipes. Can't wait to give it a try!
Thank you, Anna! I'm thrilled that you found my article helpful. ChatGPT can indeed augment your data transformation capabilities in Angular pipes. Enjoy exploring its potential!
Diego, thanks for shedding light on this exciting topic. The possibilities with ChatGPT and Angular pipes seem limitless. Looking forward to experimenting with it!
You're welcome, Isaac! The combination of ChatGPT and Angular pipes indeed opens up a world of possibilities. I'm excited for your experiments and discoveries!
Diego, your article was both enlightening and thought-provoking. Thanks for sharing your knowledge on leveraging ChatGPT in Angular pipes!
Thank you, Olivia! I'm glad my article provided valuable insights. Feel free to reach out if you have any further questions or need guidance on using ChatGPT in Angular pipes.
Diego, your article was a fantastic read! Integrating ChatGPT with Angular pipes seems like a powerful combination. Thanks for sharing your expertise!
You're welcome, Maria! It's great to hear that you enjoyed the article. ChatGPT can indeed enhance Angular pipes in unique ways. Happy coding!
Great article, Diego! ChatGPT in Angular pipes can certainly streamline data transformation tasks. Thanks for sharing this wonderful read!
Thank you, Sophia! I'm glad you found the article insightful. ChatGPT in Angular pipes is a powerful combination that can make data transformation tasks more efficient. Enjoy implementing it!
Diego, your article was an inspiration. The potential of ChatGPT in Angular pipes is unbelievable. Thanks for sharing your expertise!
You're very welcome, Daniel! I'm thrilled to hear that my article inspired you. ChatGPT in Angular pipes can indeed unlock a whole new realm of possibilities. Happy exploring!
Diego, your article was a fascinating read! I had never thought about leveraging ChatGPT in Angular pipes. Thanks for enlightening me!
Thank you, Sophie! It's always a pleasure to introduce new concepts and possibilities. Leveraging ChatGPT in Angular pipes can indeed take your data transformation workflows to the next level. Enjoy exploring!
Diego, your article was an eye-opener! ChatGPT in Angular pipes seems like an amazing combination. Thanks for sharing this valuable information!