Revolutionizing API Development Support: Harnessing the Power of ChatGPT in Coding Languages Technology
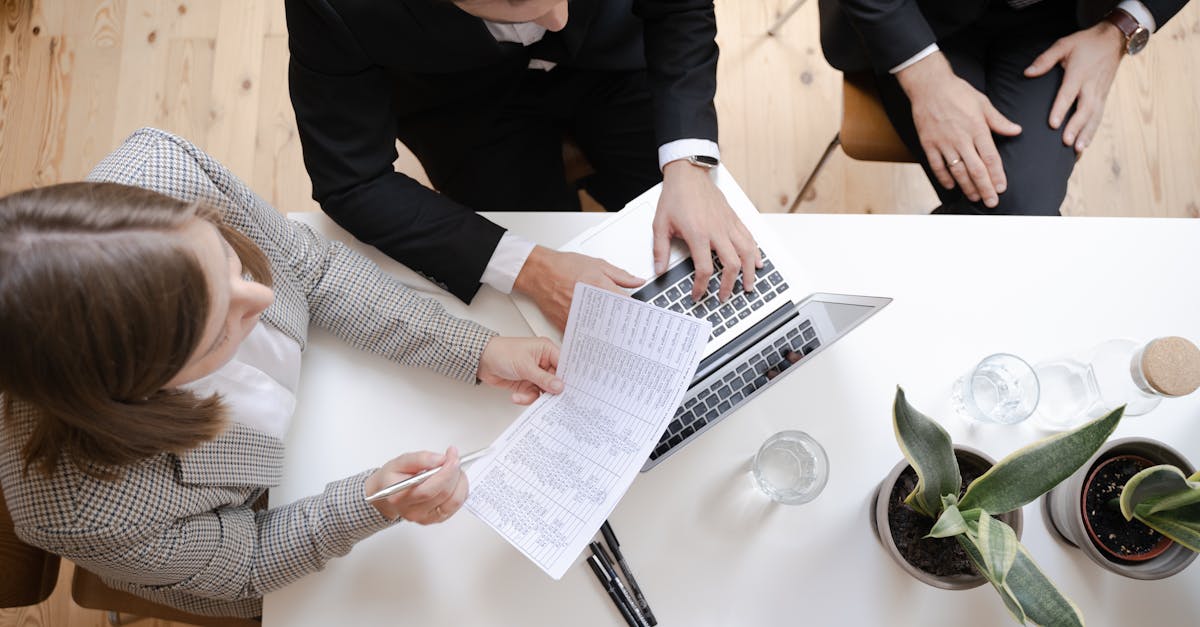
APIs (Application Programming Interfaces) play a crucial role in modern software development. They allow different software systems to communicate with each other, enabling developers to enhance functionalities and foster integration. API development support becomes paramount when it comes to ChatGPT-4, an AI model developed by OpenAI.
Why API Development Support Matters
API development support provides valuable assistance to developers in utilizing APIs effectively within their coding languages. This support equips them with the necessary knowledge and tools to integrate APIs securely and seamlessly into their applications. With the rapid growth of ChatGPT-4, such support becomes even more essential.
ChatGPT-4 API Usage Examples
ChatGPT-4 offers an extensive API that developers can leverage to incorporate AI chat capabilities into their applications. The API allows users to interact with the model by sending a list of messages as input and receiving the model's generated message as output.
Let's explore some API usage examples:
Example 1: Basic Chat Interaction
import openai
response = openai.Completion.create(
engine='davinci-codex',
prompt='What is the capital city of France?',
max_tokens=50,
n=1,
stop=None,
temperature=0.7
)
print(response.choices[0].text.strip())
The above example demonstrates a basic chat interaction using the ChatGPT-4 API. By utilizing the openai.Completion.create()
method and providing a prompt, developers can obtain an AI-generated completion in response.
Example 2: ChatGPT-4 Integration in Web Applications
async function getChatResponse(message) {
const response = await fetch('https://api.openai.com/v1/engines/davinci-codex/completions', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_API_KEY'
},
body: JSON.stringify({
'prompt': message,
})
});
const data = await response.json();
return data.choices[0].text.trim();
}
In this example, a JavaScript function is used to integrate ChatGPT-4 into web applications. By making an HTTP POST request to the API endpoint, developers can receive an AI-generated completion as a response, which can then be displayed or used in the application as desired.
Proper API Utilization
While the ChatGPT-4 API provides powerful capabilities, developers must ensure proper utilization to achieve the best results. Here are some guidelines:
- Clearly define the input prompt to obtain accurate and desired responses.
- Experiment with different values for parameters like
max_tokens
andtemperature
to control response length and randomness, respectively. - Implement error handling and rate limiting to prevent abuse and ensure optimal performance.
Conclusion
API development support is crucial for developers utilizing coding languages and aiming to leverage the power of AI through models like ChatGPT-4. Through API usage examples and proper guidance, developers can confidently incorporate AI-powered chat functionalities into their applications, expanding possibilities and transforming user experiences.
Comments:
Thank you all for your interest in my article! I'm glad to see such an active discussion. If you have any questions or need further clarification, feel free to ask.
This article is quite intriguing! I never thought AI could be used to support API development. Can you provide more details on how ChatGPT can assist in coding languages technology?
Hi Sara, thanks for your question. ChatGPT is a language model developed by OpenAI which can understand and generate human-like text. In the context of API development, it can provide developers with assistance, code suggestions, and even help with debugging. It's like having a coding buddy who can understand your programming queries and provide helpful responses.
I'm skeptical about using AI for coding tasks. How accurate and reliable is ChatGPT in providing assistance? Can it truly understand complex programming problems?
Hi Michael, that's a valid concern. While ChatGPT is a powerful language model, it may not always understand complex programming problems perfectly. It's important to note that it learns from data available on the internet and may lack domain-specific knowledge. However, with continuous training and improvements, it can still provide valuable assistance to developers by offering suggestions, initiating code completion, and providing general coding help.
As a developer, I'm curious about the integration process. How easy or difficult is it to incorporate ChatGPT into API development projects?
Hi Emily, integrating ChatGPT into API development projects can vary in complexity depending on the specific implementation. OpenAI provides tools and libraries to facilitate the integration, making it relatively straightforward. However, some customization and fine-tuning might be required to align the model's responses with the needs of the developer community. It's an evolving field, and ongoing developer feedback is crucial to improve the integration process.
This sounds like a promising tool for developers. I can see the potential benefits in terms of productivity and learning. Are there any limitations or challenges that developers should be aware of when using ChatGPT?
Great question, Chris! While ChatGPT offers significant benefits, there are some limitations to be aware of. It can sometimes generate incorrect or nonsensical code suggestions, and it may not fully understand context-based queries. Additionally, it does not have access to proprietary or confidential codebases and could potentially provide incorrect advice in certain cases. It's important to exercise caution and review suggestions before implementing them. Collaborative feedback from users is essential to improve the system's accuracy and safety.
I'm curious about the potential impact on code security. Does ChatGPT analyze the security implications of code suggestions, or could it unintentionally suggest insecure practices?
Hi Sophia, that's an excellent concern. Currently, ChatGPT primarily focuses on providing code assistance and does not specifically analyze security implications. It's crucial for developers to review and validate the suggestions for security best practices independently. Incorporating security-focused analyses could be an avenue for future improvement, but for now, it's vital to prioritize code security by following established practices and performing rigorous testing.
I can see the potential time-saving benefits, but could relying too much on AI assistance like ChatGPT limit developers' growth and problem-solving skills?
Good point, David. While AI assistance can save time and offer valuable suggestions, it's crucial for developers to continue learning and problem-solving independently. Relying solely on AI assistance can lead to dependency and limit personal growth. ChatGPT should be seen as a complementary tool that augments developers' abilities and helps them explore new solutions more efficiently.
This technology sounds fascinating! Are there any real-world case studies or success stories where ChatGPT has been implemented in API development projects?
Hi Adam, indeed! ChatGPT has been utilized in several API development projects to enhance support and code completion. While specific case studies may not be available in this context yet, it has been successful in various natural language processing tasks. Its potential in API development is being actively explored, and I'm excited to see how it evolves as more projects adopt and benefit from it.
What are the future plans for ChatGPT in API development? Are there any upcoming features or improvements in the pipeline?
Hi Sophia, the future of ChatGPT in API development looks bright. OpenAI plans to refine and expand its capabilities based on user feedback. This includes reducing biases, making the system safer to use, and enhancing the model's understanding of code-related queries. OpenAI is also exploring ways to allow fine-tuning of models to better suit specific user needs. The roadmap emphasizes collaboration with the developer community for continuous improvement and innovation.
Is there any concern about potential misuse of ChatGPT in API development? How can OpenAI ensure responsible use?
Valid question, Ethan. OpenAI recognizes the importance of responsible and ethical use of AI technologies like ChatGPT. They are dedicated to creating safeguards to prevent potential misuse. OpenAI aims to involve the developer community in shaping the rules and limits of the system, seeking feedback and guidance to address concerns effectively. OpenAI's commitment to transparency and accountability acts as a foundation for responsible AI development in the API domain.
I'm concerned about the accessibility of ChatGPT. Is it accessible to developers of all programming skill levels, including beginners?
Hi Lucy, accessibility is an important aspect. While ChatGPT can benefit developers of all skill levels, including beginners, it's more likely to be valuable for intermediate to advanced programmers who can better understand and evaluate its suggestions. However, efforts are underway to make it more accessible and beginner-friendly, ensuring that everyone can leverage its potential. User feedback plays a vital role in shaping the features and accessibility of ChatGPT.
Are there any potential use cases where ChatGPT could be applied beyond API development? How versatile is this technology?
Great question, Oliver. The versatility of ChatGPT extends beyond API development. It can assist in a wide range of tasks, such as generating conversational agents, drafting content, answering questions, and much more. OpenAI is actively exploring different use cases and continually improving the system to enhance its capabilities. The technology has the potential to revolutionize various industries by providing human-like language understanding and generation.
What is the main advantage of using ChatGPT instead of traditional programming documentation or help forums?
Hi Jennifer, the main advantage of using ChatGPT lies in its ability to provide interactive and dynamic assistance. Unlike traditional programming documentation, which can be static and might not address specific queries, ChatGPT understands natural language and can generate responses similar to human conversations. This allows developers to ask specific questions, get code suggestions, and foster a more interactive and personalized approach to problem-solving in the API development space.
Can ChatGPT be used collaboratively, allowing multiple developers to benefit from its assistance simultaneously?
Hi Jake, currently, ChatGPT's collaborative usage is not explicitly mentioned in the article. It's primarily designed to assist individual developers. However, there is potential for future improvements and integrations that might allow collaborative usage, enabling multiple developers to leverage its assistance simultaneously. As development tools evolve, collaborative features can bring additional benefits to the API coding process.
How well does ChatGPT handle different programming languages? Are there any limitations in terms of language support?
Hi Maria, ChatGPT has been trained on large amounts of diverse data, including code snippets from various programming languages. While it can offer assistance for different languages, its level of expertise may vary across them. It performs best in more popular programming languages with abundant training data available. As the model evolves, OpenAI intends to expand its language support and improve its understanding and assistance for a broader range of languages.
How would you compare ChatGPT with other existing code analysis tools and developer assistance platforms available in the market?
Hi Sophie, ChatGPT offers a unique approach to developer assistance compared to traditional code analysis tools and platforms. While it may not have the same level of domain-specific knowledge or accuracy as some existing tools, it focuses on providing more interactive, conversational assistance. This can be advantageous when developers seek customized code suggestions or encounter specific coding challenges. Exploring a balance between existing tools and ChatGPT's dynamic assistance can lead to better development experiences.
What kind of computational resources are required to utilize ChatGPT effectively? Are there any specific hardware or processing requirements?
Hi Emma, utilizing ChatGPT effectively requires a reasonably powerful computational system. While OpenAI suggests using a GPU for faster responses, it can still be used on CPUs but with potentially longer response times. There are no specific hardware or processing requirements mentioned, but having a system with ample memory, processing capabilities, and a stable internet connection would enhance the overall experience of utilizing ChatGPT in API development projects.
Do developers need to train or fine-tune ChatGPT specifically for their projects, or is it already trained and ready to assist?
Hi Daniel, developers don't need to train ChatGPT specifically for their projects. ChatGPT is a pre-trained language model that comes ready to assist in various tasks, including API development support. However, developers can potentially fine-tune the model using their own datasets to better align it with their specific application domain. Fine-tuning allows customization but isn't necessary for utilizing ChatGPT's core functionality in API development.
I'm thrilled to see AI advancing in the realm of API development! Can you provide any insights into the performance and stability of ChatGPT?
Hi Liam, ChatGPT has shown remarkable performance and stability in generating human-like text and providing assistance. However, it's vital to understand that like any AI model, it has certain limitations and can occasionally make errors or provide incorrect suggestions. Continuous improvements and fine-tuning are ongoing to enhance its performance, increase stability, and minimize any potential shortcomings. The focus remains on ensuring reliable and useful assistance for developers in API development.
How does ChatGPT handle niche or lesser-known programming languages and frameworks? Is it adaptable to less mainstream coding environments too?
Hi Ruby, while ChatGPT can provide assistance in various programming languages, its proficiency might vary across different languages and frameworks. It generally performs better in more mainstream coding environments that have richer training data available. However, OpenAI is actively working on improving support for niche or lesser-known languages and frameworks, aiming to make ChatGPT adaptable to a wider range of coding environments.
Thank you all for your engaging questions and thoughts. I appreciate your participation in this discussion about harnessing the power of ChatGPT in coding languages technology. It was wonderful interacting with you all!