Revolutionizing C++ Library Development with ChatGPT
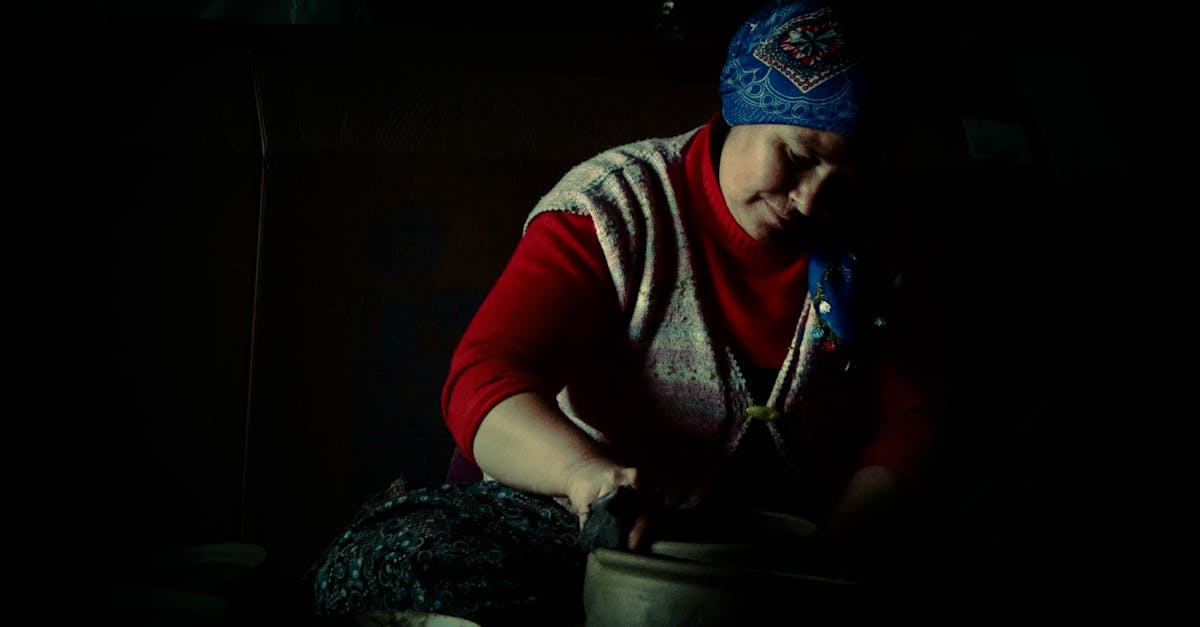
Introduction
C++ is a powerful programming language that offers several features to assist developers in various areas. One such area is the development of libraries. Libraries in C++ are collections of precompiled code that can be reused by developers to simplify the development process and enhance code reusability.
The Significance of Libraries in C++
When developing applications, developers often encounter common tasks or functionalities that can be abstracted and encapsulated into standalone libraries. These libraries can provide ready-made solutions, saving developers time and effort. Moreover, libraries enable code reusability across multiple projects, reducing redundancy and promoting efficient software development.
Functionalities of Libraries in C++
C++ libraries can offer a wide range of functionalities, catering to various development requirements. Some common functionalities provided by libraries include:
- Data structures and algorithms: Libraries can provide implementations of commonly used data structures and algorithms, such as linked lists, binary trees, sorting algorithms, etc. These ready-made solutions save developers from reinventing the wheel and allow them to focus on higher-level tasks.
- Networking: Libraries can handle network-related tasks, such as socket programming, server-client communications, and data serialization. These functionalities are especially helpful when developing networking applications.
- User interface: Libraries can provide graphical user interface (GUI) components that developers can use to build interactive applications efficiently. GUI libraries often offer pre-designed widgets, event handling mechanisms, and other UI-related functionalities.
- File handling: Libraries can simplify file operations, such as reading from and writing to files, parsing various file formats, and handling file system-related tasks.
- Mathematics and scientific computing: Libraries can include mathematical functions, linear algebra routines, numerical optimization methods, and other tools required for scientific computing applications.
Examples of Libraries in C++
Some popular libraries in C++ include:
- STL (Standard Template Library): Provides a collection of generic algorithms and data structures, including containers (like vectors, lists, and maps), algorithms (like sorting and searching), and iterators.
- Boost: Offers a wide range of utilities and libraries, covering areas such as smart pointers, multithreading, regular expressions, cryptography, and more.
- OpenCV (Open Source Computer Vision Library): Concentrated on computer vision and machine learning, it provides many functions and algorithms to handle tasks related to computer vision, image processing, and object detection.
- OpenSSL: A robust library for secure communication and cryptography, enabling developers to implement secure network protocols and encryption algorithms.
- Qt: A cross-platform GUI framework that provides a large set of user-friendly components and tools to develop graphical applications.
Developing Libraries in C++
When developing libraries in C++, certain practices should be followed to ensure their effectiveness:
- Simplicity: Libraries should provide simple and intuitive interfaces that are easy to understand and use. Clear documentation is essential for developers to effectively integrate and utilize the library.
- Modularity: Libraries should be designed in a modular fashion, allowing developers to use only the necessary components and avoid unnecessary dependencies.
- Compatibility: Libraries should be compatible with different C++ compilers and platforms, enabling developers to use them across a wide range of environments.
- Efficiency: Libraries should be optimized for performance and memory usage, ensuring they do not introduce significant overhead to the application using them.
- Error handling: Libraries should handle errors gracefully and provide appropriate mechanisms for error reporting and recovery.
- Testing: Libraries should be thoroughly tested to ensure their correctness, robustness, and compatibility with different scenarios.
- Versioning: Libraries should follow versioning schemes to allow developers to track changes and updates, ensuring backward compatibility whenever possible.
Conclusion
Developing libraries in C++ can be a powerful technique to enhance code reusability and simplify the development process. By encapsulating common functionalities into libraries, developers can focus on higher-level tasks and create more efficient and maintainable applications.
Comments:
Thank you all for reading my article on Revolutionizing C++ Library Development with ChatGPT! I'm excited to hear your thoughts and answer any questions you may have.
This is a fascinating concept! As a C++ developer, I'm always interested in exploring new ways to improve library development. ChatGPT seems like a powerful tool. How does it enhance the development process in specific ways?
I agree, Michael! ChatGPT sounds really promising. Amanda, could you provide some examples of how ChatGPT has been utilized in C++ library development? I'd love to see some practical use cases.
Absolutely, Michael and Samantha! ChatGPT can enhance C++ library development in several ways. It can assist with generating code snippets, providing documentation, answering questions, suggesting improvements, and even aiding in identifying bugs. It's like having an intelligent assistant for the development process!
This is truly mind-blowing! It seems like ChatGPT could save a lot of time and effort in library development. Is it readily available for developers to use?
Great question, Jeff! ChatGPT is not publicly available yet, but OpenAI has plans to make it accessible. Currently, developers can join the waitlist to get early access and start experimenting with it.
As a beginner in C++, I find the idea of using ChatGPT in library development intriguing. Will it be beginner-friendly, or is it more suitable for experienced developers?
That's a great question, Rachel! While ChatGPT can definitely be helpful to experienced developers by providing advanced suggestions and insights, it is designed to be beginner-friendly as well. It can assist with providing explanations, offering code examples, and guiding users through the development process.
I'm curious about the performance of ChatGPT in a resource-intensive language like C++. Can it handle large codebases efficiently?
Good question, Greg! While ChatGPT is indeed powerful, it's important to note that its performance may vary depending on the complexity and size of the codebase. It works best for smaller or medium-sized projects where it can provide valuable assistance.
I can see how ChatGPT would be valuable for generating code snippets. Are there any limitations or challenges developers should be aware of when using ChatGPT for this purpose?
Absolutely, Oliver! While ChatGPT can generate code snippets, it's important to carefully review and validate the generated code as it may not always be perfect. It's recommended to consider ChatGPT's suggestions as aids rather than directly copying the generated code without understanding its implications.
Is there any pricing information available for ChatGPT? Will it be free to use?
Good question, Lisa! OpenAI offers both free and paid plans for using ChatGPT. The details regarding pricing and plans can be found on OpenAI's website.
As a beginner, I often struggle with understanding complex C++ concepts. Would ChatGPT be able to provide explanations and simplify things for me?
Absolutely, Daniel! ChatGPT can be a valuable resource for beginners. It can help explain complex concepts, provide simplified explanations, and offer code examples that can aid in your understanding of C++ development. Think of it as having a knowledgeable mentor by your side!
I'm concerned about potential security risks. How does OpenAI ensure the trustworthiness of the code snippets generated by ChatGPT?
That's an important concern, Ethan! OpenAI is actively working on ensuring the trustworthiness of ChatGPT's outputs. They have implemented measures like safety mitigations and fine-tuning techniques to minimize the risk of code snippets introducing vulnerabilities. It's an ongoing focus for them to prioritize the security aspect.
It's great to hear that OpenAI is taking security seriously. Will developers have control over the generated code, allowing them to review and modify it as needed?
Absolutely, Caroline! Developers will have full control over the generated code and can review, modify, and adapt it based on their specific requirements. ChatGPT's suggestions are meant to assist and empower developers, not replace their expertise and judgment.
Are there any limitations on the number of API calls that a developer can make while using ChatGPT?
Yes, Brian. OpenAI does have rate limits in place to ensure fair usage of the ChatGPT API. The specifics regarding rate limits and other technical details can be found in the developer documentation on OpenAI's website.
I'm excited about the potential of using ChatGPT as a learning tool for C++. Can it provide practice exercises or quizzes to help reinforce concepts?
That's a great idea, Sophia! While ChatGPT currently does not provide practice exercises or quizzes directly, it can certainly assist in guiding you through existing resources, recommend educational materials, and answer questions as you learn and practice C++ development.
I'm curious to know if there are any plans to expand ChatGPT's capabilities to other programming languages apart from C++ in the future.
Absolutely, Hannah! OpenAI has plans to expand ChatGPT's capabilities to cover a broader range of programming languages based on user feedback and demand. So, we can look forward to seeing its application in other programming languages in the future.
That's great to hear! I'm primarily a Python developer, and I'd love to see ChatGPT's capabilities extended to Python as well.
Python is definitely a popular language, Emily, so it's highly likely that ChatGPT's capabilities will be extended to Python in the future. Keep an eye out for updates from OpenAI!
In addition to programming language support, are there any plans to integrate ChatGPT with popular IDEs or code editors?
That's an interesting idea, Tom! While I don't have specific details, integrating ChatGPT with popular IDEs or code editors could certainly be a valuable enhancement. It's always exciting to think about how developer tools can be improved using AI technologies.
I can imagine how beneficial it would be to have ChatGPT integrated into the development environment. It could provide real-time suggestions and even automatically fix simple coding mistakes!
Absolutely, Henry! Integrating ChatGPT with IDEs or code editors could enable interactive and productive development experiences. From providing suggestions to automated fixes, the possibilities are exciting. It could greatly enhance developers' productivity and learning process.
Can multiple developers collaborate using ChatGPT in real-time to work on a library?
Currently, ChatGPT is designed for individual use and does not support real-time collaboration directly. However, developers can use ChatGPT independently and share their findings or suggestions with their team members to facilitate collaboration and improve library development collectively.
Are there any restrictions on the type of questions or assistance developers can ask from ChatGPT? Can it provide non-C++ related help?
Good question, Victoria! While ChatGPT is primarily focused on assisting with C++ library development, it can also provide general programming guidance and answer non-C++ related questions to some extent. However, its expertise and accuracy remain strongest within the domain of C++ development.
Is there a limit to the number of waitlist participants? When can we expect broader availability of ChatGPT?
OpenAI has not specified a specific limit for the waitlist participants, David. As for broader availability, OpenAI is actively working towards it. They are actively iterating on the model and plan to refine and expand its offerings based on user feedback. Keep an eye on OpenAI's website for updates!
It would be amazing if future versions of ChatGPT could support real-time collaboration! Working together on a library using such an advanced tool would be a developer's dream!
Indeed, Grace! Real-time collaboration features in the context of ChatGPT could be a game-changer, enabling developers to work seamlessly together on libraries. While it's not currently available, your feedback is valuable, and I'm sure OpenAI will take it into consideration for future iterations.
Considering the broad capabilities of ChatGPT, is there any concern that developers might become overly reliant on its assistance, potentially impacting their own growth and expertise?
That's a valid concern, Lucas. While ChatGPT is a powerful tool, it's important for developers to strike a balance and not solely rely on its assistance. It's designed as a helpful companion, offering guidance and suggestions, but developers should continue to actively learn, practice, and grow their own expertise in C++ development.
What precautions are in place to ensure that ChatGPT's suggestions align with best practices and industry standards in C++ development?
That's an important consideration, Martin. OpenAI is actively training ChatGPT to align the assistance offered with best practices and industry standards in C++ development. They are continuously working on improving ChatGPT's training process to ensure it stays up-to-date with the latest practices and produces more accurate and reliable suggestions.
Could ChatGPT potentially be used for educational purposes in teaching C++ programming?
Absolutely, Samuel! ChatGPT can be a valuable educational resource in teaching C++ programming. It can provide explanations, code examples, and guidance that can enhance the learning experience. I can envision it being utilized in tutorials, online courses, or even as virtual teaching assistants.
Could the integration of ChatGPT with IDEs potentially raise concerns about privacy and data security?
Privacy and data security are indeed important considerations, Sarah. OpenAI understands these concerns and strives to ensure the privacy and security of users' data. Any integration with IDEs would need to prioritize user privacy and provide clear transparency regarding data usage and access.
As an educator, I'm excited about the prospects of using ChatGPT in the classroom. Are there any plans for educational licenses or special programs for educational institutions?
That's great to hear, Robert! While I don't have specific information on educational licenses or programs, OpenAI has shown interest in academic partnerships and collaborations. It's worth reaching out to OpenAI to explore potential opportunities for leveraging ChatGPT in educational contexts.