Revolutionizing Redux Architecture: Unleashing the Power of ChatGPT for Expert Advice
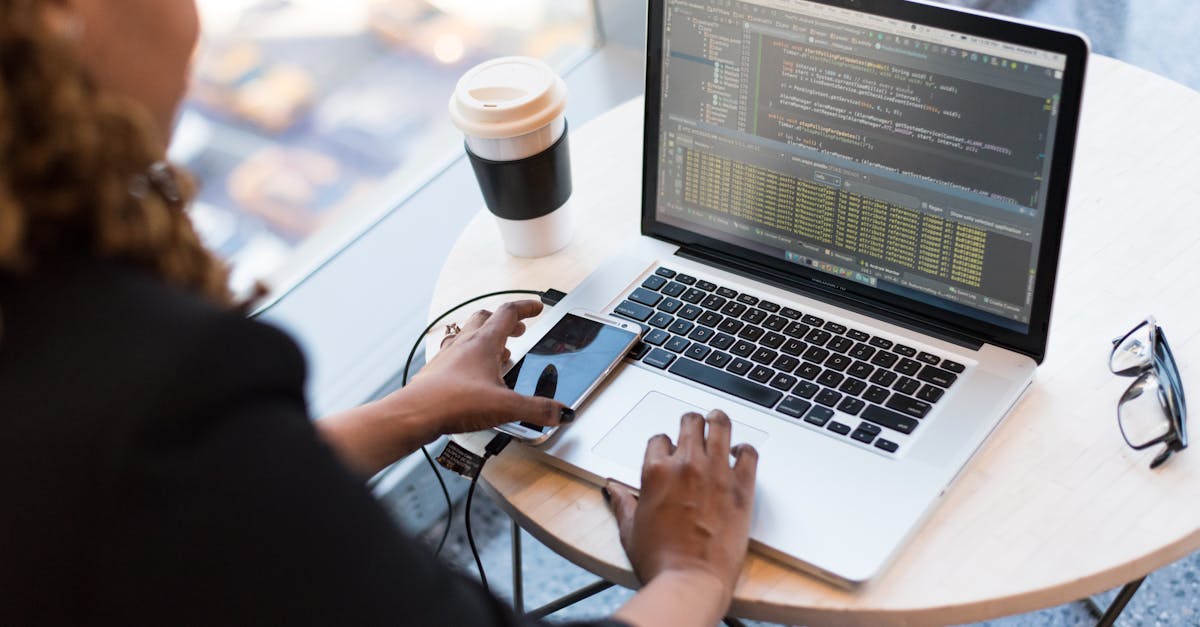
In the world of web development, Redux has become a popular technology for managing state in JavaScript applications. Redux provides a predictable state container that can be used with any JavaScript framework or library. It promotes a one-way data flow and helps in managing complex application states. In this article, we will explore how Redux can be used to make architecture and design decisions.
Redux Architecture
The Redux architecture consists of three main components: the store, actions, and reducers. The store is a JavaScript object that holds the application state. Actions are plain JavaScript objects that represent an intention to change the state. Reducers are pure functions that transform the current state and an action into a new state.
Store
The store is the single source of truth for your application's state. It holds the complete state tree of your application and provides methods to update the state. The state can only be modified by dispatching actions to the store.
Actions
Actions are payloads of information that send data from your application to the Redux store. They are plain JavaScript objects and must have a property called 'type' that describes the type of action being performed. Actions can also carry additional data, called a payload, that provides the necessary information to update the state in the reducers.
Reducers
Reducers are functions that specify how the application's state changes in response to actions. They take the current state and an action as arguments and return a new state based on the action's type. Reducers are pure functions, meaning they should not modify the existing state, but instead return a new state object.
Design Decisions
When designing a Redux architecture, there are several key decisions that need to be made:
- State Shape: Defining the shape of the state tree is important to ensure data is stored in a structured manner. It helps in writing efficient selectors and accessing state properties easily.
- Reducers: Deciding on the composition of reducers is crucial for managing state updates. Breaking down the state and associated actions into smaller independent reducers can lead to a more maintainable codebase.
- Actions: Choosing when and how to dispatch actions is essential. Actions should be dispatched when a state change is needed in response to user interactions, asynchronous requests, or other events.
- Middlewares: Middleware functions can be used to add custom logic around the dispatch process. They enable advanced features like async actions, logging, and routing.
Benefits of Redux Architecture
Using Redux in your application architecture can provide several benefits:
- Predictability: Redux enforces a strict pattern for state management, which leads to predictable application behavior. It helps in debugging and understanding how the state changes over time.
- Scalability: Redux architecture makes it easier to scale your application by separating concerns and ensuring a single source of truth for state management.
- Testability: The predictable nature of Redux makes it easier to write unit tests for your reducers and actions. Testing becomes more straightforward as the state changes are isolated and well-defined.
- Community Support: Redux has a large and active community, which provides a wealth of resources, tutorials, and libraries. Learning and adopting Redux is made easier due to the availability of community-driven support.
Conclusion
Redux is a powerful state management tool that can significantly improve your application architecture and design decisions. Its well-defined patterns and separation of concerns make it easier to manage complex states and promote maintainability. By adopting Redux, you can create more predictable, scalable, and testable JavaScript applications.
Comments:
Thank you all for your comments on my article! I'm excited to discuss further.
Great article, Rene! I've been using Redux for a while now, and I'm curious to see how ChatGPT can enhance the architecture.
I agree, Michael. Incorporating AI into Redux could open up new possibilities. Looking forward to exploring it further.
Absolutely, Emily. It could introduce smarter decision-making and automated troubleshooting within the Redux architecture.
That's exactly what I'm hoping for, Michael. It could greatly improve efficiency and reduce development time.
Michael, could you share some examples of how ChatGPT can assist with state management in Redux?
Sure, Lisa! ChatGPT can analyze the state and provide suggestions for restructuring or optimizing the Redux store based on patterns it learns.
That sounds incredibly helpful, Michael. It would be like having an AI-powered Redux consultant.
Agreed, Lisa. It's crucial to thoroughly evaluate the security risks and implement proper safeguards.
I'm new to Redux, but I'm intrigued by your article. Looking forward to learning more about ChatGPT integration.
Interesting concept, Rene. I wonder what impact using ChatGPT might have on performance and scalability.
I've been struggling with managing complex state in Redux. Excited to see if ChatGPT can provide some expert advice in that area.
I'm skeptical about introducing AI to Redux. It seems like overkill and could make the codebase more complex.
I'm in the same boat, David. However, if implemented thoughtfully, it may provide valuable insights and aid in debugging.
That's a valid concern, Sarah. It's crucial to strike a balance between AI assistance and maintaining code simplicity.
Exactly, David. The key is to use ChatGPT as a helpful tool rather than relying on it excessively.
I'm excited about this implementation, Rene. It could elevate Redux to a whole new level and improve development workflows.
Rene, have you encountered any limitations or challenges when integrating ChatGPT with Redux?
Chris, one challenge I faced was handling the longer response times of ChatGPT, which required optimizing the UI to prevent delays.
Do you think the integration of ChatGPT will require significant changes to existing Redux projects?
Eric, based on my experience with AI integration, there might be some adjustments needed, but it depends on the complexity of the project.
Thanks for the insight, Sophia. I'm excited to experiment with ChatGPT integration in my Redux project.
Absolutely, Eric. Start with smaller components or modules and assess the impact before scaling up the integration.
Eric, it's always exciting to explore new possibilities. Start small, test, and gather feedback from your team to ensure a smooth integration.
Sounds like a plan, Sophia. Thanks for the advice!
You're welcome, Eric. Best of luck with your Redux and ChatGPT endeavors!
Additionally, training the AI model and ensuring it understands the context of Redux actions required some effort.
Rene, have you observed any performance impacts when using ChatGPT in Redux applications with high user concurrency?
Very insightful feedback, Rene. Thanks for sharing your experience.
Rene, have you explored using ChatGPT for form validations or generating validation rules based on user input?
Great question, Emily. While ChatGPT can provide suggestions for validations, it's essential to ensure user inputs are validated on the server-side as well.
Exactly, Rene. AI assistance can be valuable, but we must always enforce rigorous data validation and security measures.
Thank you for addressing the performance concerns, Rene. It's important to consider scalability and response times when using ChatGPT in production.
Rene, did you encounter any difficulties in aligning the generated ChatGPT responses with the existing Redux actions and reducers?
That's a good point, Rene. Combining server-side validation with AI-generated suggestions can provide a robust and user-friendly solution.
Agreed, Emily. It's all about finding the right balance and leveraging the strengths of both AI and traditional development practices.
Rene, can you share any best practices when it comes to training and fine-tuning ChatGPT models for Redux assistance?
David, aligning ChatGPT responses with Redux actions required careful mapping and training examples for the AI model.
David, it did pose some challenges initially, but I found that clearly defining intent and supporting actions helped improve alignment.
Thank you for addressing my question, Rene. It's good to know that performance and scalability can be managed effectively.
Adam, you're welcome! It's crucial to evaluate the performance impact in your specific use case, especially under high user concurrency.
Indeed, Rene. Understanding the performance implications and properly managing scalability is crucial for successful adoption.
Thanks, Rene. Training the model with actual Redux interactions seems like a valuable approach for increased accuracy.
Appreciate the insight, Rene. Clearly defining intent and actions should help with aligning ChatGPT-generated responses.
I'm concerned about the potential security implications of integrating an AI model like ChatGPT with Redux.
Can ChatGPT handle multi-step form validations within Redux? That's been a pain point for me in the past.
Absolutely, Emily. Security should be a top priority in any application, especially when integrating external AI models.
Precisely, Jason. We must consider authentication, access control, and data protection when dealing with AI-powered enhancements.
To ensure accuracy, continuously updating and retraining the model with real-world Redux interactions proved beneficial.
Thanks for the helpful discussions, everyone. This has been enlightening. Looking forward to implementing ChatGPT in my projects!