Streamlining Project Management with ChatGPT for Backbone.js Technology
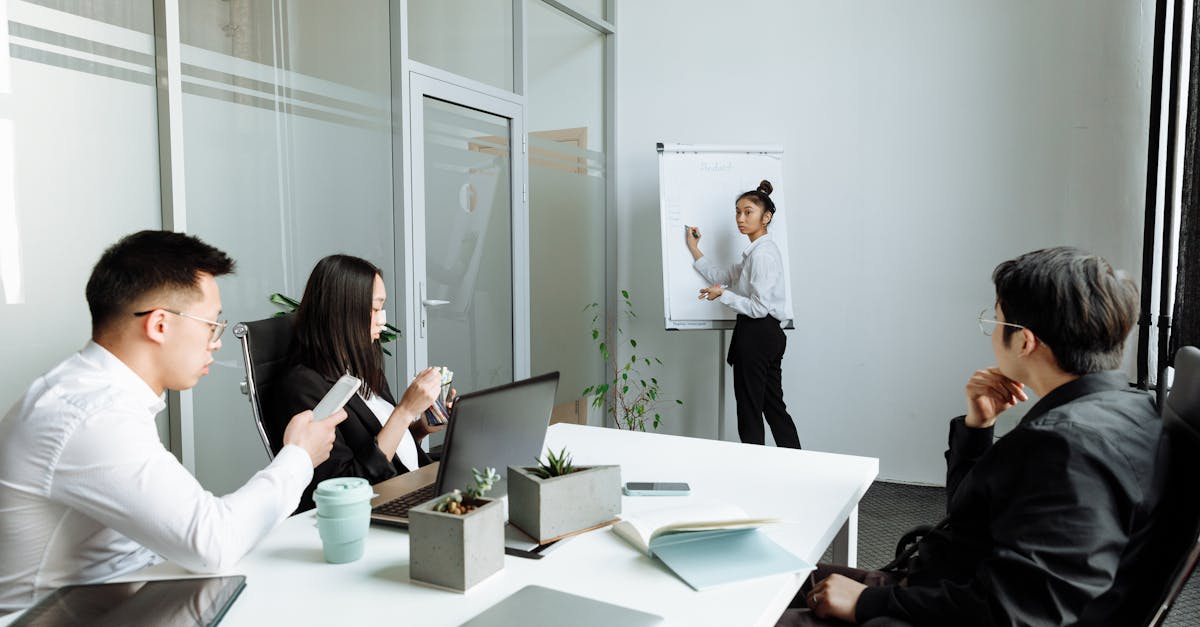
Introduction
Backbone.js is a lightweight JavaScript library used for structuring and organizing web applications. It provides an easy way to handle data in your applications along with creating reusable models and views. Thanks to its minimal setup and flexibility, Backbone.js has gained popularity among developers in the realm of project management.
Role of Backbone.js in Project Management
Backbone.js simplifies the process of building and managing complex frontend applications. Its key features, such as event-driven architecture and modular components, make it an ideal framework for project management applications. Project managers can utilize Backbone.js for:
- Real-time collaboration: Backbone.js allows project managers to build chat systems, enabling team members to communicate seamlessly. The event-driven architecture ensures instant updates without reloading the page, facilitating effective collaboration.
- Data binding: Backbone.js helps in automatically updating the user interface when data changes. This feature proves useful when managing project information, enabling project managers to keep track of updates made by team members in real-time.
- Flexible task management: Backbone.js lets project managers create efficient task management systems. They can categorize and organize tasks, assign them to team members, and track progress with ease. With reusable models and views, project managers can build custom workflows tailored to their specific project requirements.
Introducing ChatGPT-4 for Backbone.js Project Management
ChatGPT-4, powered by advanced natural language processing algorithms, can assist project managers in managing Backbone.js projects effectively. Its capabilities include:
- Automated task updates: ChatGPT-4 can integrate with Backbone.js applications to provide automated task updates based on user input. Project managers can interact with ChatGPT-4 via a chat interface and receive real-time updates on task progress or other project-related queries.
- Smart task assignment: With its deep learning capabilities, ChatGPT-4 can analyze project requirements and team member skills to suggest the most suitable candidates for task assignments. This feature saves time for project managers, ensuring efficient team allocation and task delegation.
- Intelligent project insights: By analyzing project data and user interactions, ChatGPT-4 can provide valuable insights to project managers. It can generate reports, offer suggestions for process improvements, and identify potential bottlenecks, empowering project managers to make informed decisions.
Implementation Example
Here's a code snippet demonstrating how Backbone.js and ChatGPT-4 can work together in a project management context:
// Backbone.js model for tasks var TaskModel = Backbone.Model.extend({ defaults: { title: '', assignee: '', status: 'pending' } }); // Backbone.js view for a task item var TaskView = Backbone.View.extend({ tagName: 'li', template: _.template($('#task-template').html()), render: function() { this.$el.html(this.template(this.model.toJSON())); return this; } }); // Backbone.js collection for tasks var TasksCollection = Backbone.Collection.extend({ model: TaskModel, url: '/api/tasks' }); // Initializing ChatGPT-4 interface var chatGPT4 = new ChatInterface({ el: $('#chat-interface'), models: ['/models/backbone-js-project-management/model.bin'], datasets: ['/datasets/backbone-js-project-management/dataset.txt'] }); // Event listener for task updates chatGPT4.on('taskUpdate', function(data) { // Perform necessary actions for task updates }); // Event listener for task assignment chatGPT4.on('taskAssignment', function(data) { // Perform necessary actions for task assignment }); // Event listener for project insights chatGPT4.on('projectInsights', function(data) { // Perform necessary actions for project insights });
Conclusion
Backbone.js offers project managers a robust framework for efficiently managing their project tasks and collaborating with team members. When combined with the powerful capabilities of ChatGPT-4, project managers can elevate their productivity and decision-making process. By leveraging ChatGPT-4's task updates, task assignment, and project insights, project managers can streamline their project management workflow and achieve successful project outcomes.
Comments:
Thank you for reading my article! I'm excited to hear your thoughts on using ChatGPT for Backbone.js.
Great article, Dan! I'm particularly intrigued by the idea of using ChatGPT for project management. It could really streamline communication and collaboration.
I've never heard of ChatGPT before, but it sounds promising. Can you provide more details about its effectiveness in project management?
Sure, Emily! ChatGPT is a powerful language model developed by OpenAI. It can understand natural language and generate human-like responses. By integrating it into project management tools, teams can have interactive conversations with the system, making it easier to track progress, assign tasks, and discuss project-related matters.
Emily, I've used ChatGPT in a couple of projects, and it has been quite effective in managing tasks and discussing project requirements. It's definitely worth exploring.
Thanks, Adam! It's helpful to hear from someone who has hands-on experience with ChatGPT. I'll definitely consider giving it a try.
I completely agree, Adam! Communication bottlenecks can be a hindrance in project management, and ChatGPT has the potential to bridge those gaps.
Exactly, Emma! Conversational interfaces provide a more intuitive way for users to interact with project management tools.
That's fascinating, Sarah! It would definitely make the project management app more user-centric and engaging.
I can see the benefits of using ChatGPT in project management. It would reduce the need for lengthy meetings and emails, making communication more efficient.
Exactly, Sarah! It could also help automate repetitive tasks and provide instant assistance to team members.
I'm curious about the integration of ChatGPT with Backbone.js. How does that work, Dan?
Great question, Emma! Backbone.js is a popular JavaScript framework for building web applications. By combining ChatGPT with Backbone.js, you can create interactive chat-based interfaces within your project management app. This allows users to interact with ChatGPT seamlessly.
Dan, looks like ChatGPT has great potential to transform project management. Thanks for sharing your insights with us.
Absolutely, Emma! ChatGPT has the potential to transform how teams collaborate and communicate within project management tools.
Emma, integrating ChatGPT with Backbone.js brings a conversational element to your project management app. Team members can type commands or questions, and ChatGPT will respond accordingly. It adds a layer of interactivity and natural language interaction.
I agree, Sarah! Having a conversational interface can enhance user experience and improve engagement.
Seems like a cool integration, Dan! How is the performance of ChatGPT for real-time conversations?
Thanks, John! The performance of ChatGPT for real-time conversations is quite impressive. It provides low-latency responses, making it suitable for interactive use-cases like project management. However, it's important to note that the model should be fine-tuned and curated to ensure it aligns with the desired behavior.
Thanks for the clarification, Dan! It's good to know that low-latency responses are achievable with ChatGPT.
John, I've used ChatGPT to manage multiple projects simultaneously, and it has been reliable. Its real-time performance was crucial for effective collaboration.
Definitely, Dan! Low-latency responses are crucial for maintaining effective real-time conversations.
Agreed, John! ChatGPT's reliability and real-time performance have made it my go-to choice for managing project communications.
Absolutely, Alice! Automation can free up project managers' time, allowing them to focus on more critical aspects of the project.
Sarah, conversational interfaces can also help onboard new team members more smoothly, making the project management tool easier to adopt.
Sounds great, Sarah! I'm excited to explore the interactivity of a ChatGPT-powered project management app.
Sarah, you're absolutely right. Combining functionality with an intuitive interface is a win-win for project managers and team members.
That's true, Sarah! Experimentation is key in finding the optimal setup for using ChatGPT effectively.
John, based on my experience, ChatGPT performs quite well for real-time conversations. You can expect quick and coherent responses, especially when you fine-tune it to your specific use-case.
Thanks, Alex! I'm excited to try ChatGPT for managing real-time conversations in my projects.
Absolutely, John! ChatGPT provides a lot of potential for enhancing project management processes.
You're welcome, Michael! I completely agree, the advancements in AI models like ChatGPT open up exciting possibilities for project management.
Dan, integrating ChatGPT with Backbone.js seems like a great way to enhance collaboration and make project management more dynamic.
Thanks for the tip, Michael! I'll explore different prompt designs and fine-tuning techniques to optimize the usage of ChatGPT.
Emily, feel free to ask if you have any more questions or need guidance on using ChatGPT effectively. Happy to help!
Thanks, Alex! I'll definitely consider fine-tuning ChatGPT to achieve the best results for my project management needs.
I can see the potential, but I'm concerned about the reliability and accuracy of ChatGPT. Can it handle complex conversations and understand context properly?
Valid concern, Michael! While ChatGPT has shown remarkable improvements, it can sometimes generate inaccurate or nonsensical responses. However, it can handle complex conversations and understand context to some extent. Ongoing research and developments aim to enhance its reliability and accuracy.
I appreciate your response, Dan. It's good to see improvements in machine learning models. Exciting times for project management!
Dan, can ChatGPT also handle multiple natural languages, or is it limited to a specific language?
Jake, ChatGPT has been trained on a large corpus of English text, so it primarily understands and generates text in English. However, you can adapt it to other languages by training it on data specific to those languages.
Thanks for the clarification, Dan! I'll keep that in mind if I decide to experiment with ChatGPT in the future.
ChatGPT with Backbone.js sounds like a game-changer. It would make project management more interactive and user-friendly.
Integrating ChatGPT with Backbone.js seems like a straightforward process. I'll definitely explore this combination.
Emily, make sure to experiment with different prompt designs and fine-tuning techniques to get the best results when using ChatGPT. It improves the system's effectiveness.
Emily, ChatGPT can understand commands like 'Create task ABC' or 'Assign task ABC to John'. It allows for interactive task management.
Automation is key in improving productivity. ChatGPT could help reduce the burden on project managers by automating routine tasks.
I can foresee many benefits in using ChatGPT in project management, especially in increasing efficiency and reducing communication overhead.
Automation is indeed crucial for enhancing productivity and reducing manual effort. Exciting possibilities lie ahead with ChatGPT!
John, real-time conversations facilitated by ChatGPT can significantly improve collaboration and decision-making in projects.