Supercharging Data Querying with ChatGPT in ADO.NET Technology
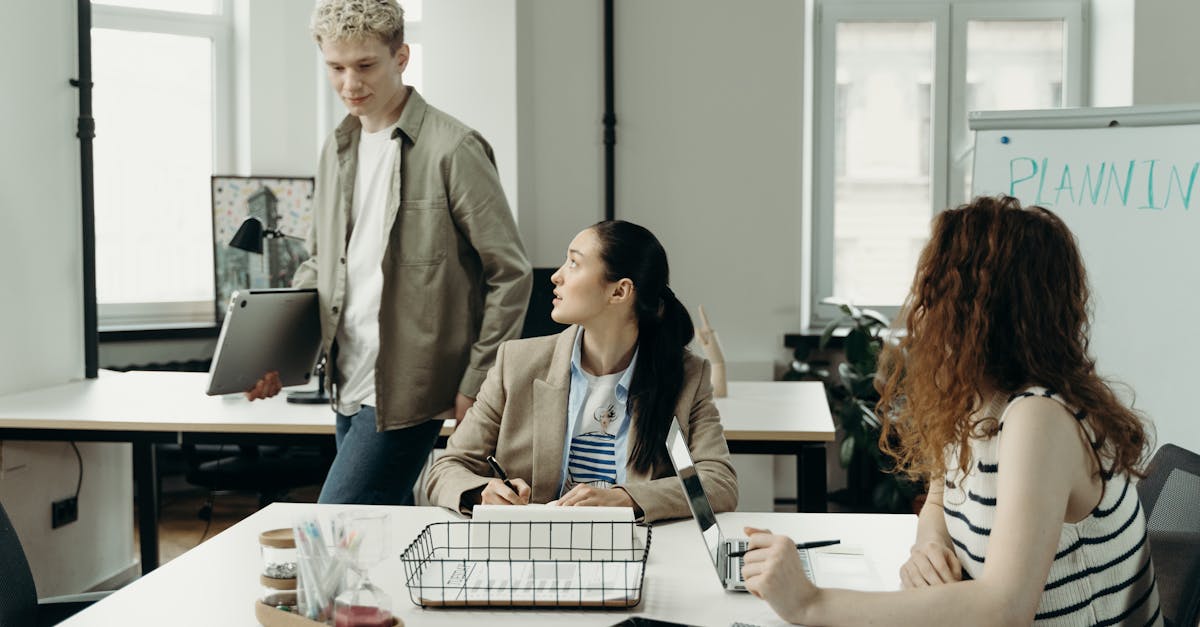
Technology: ADO.NET
Area: Data Querying
Usage: ChatGPT-4 can provide assistance with querying data using ADO.NET technologies.
When it comes to retrieving and manipulating data from databases, ADO.NET is a widely used technology. It is a component of the Microsoft .NET framework specifically designed for data access, providing a set of libraries and functionalities that simplify the process of querying data from various data sources, including SQL databases, XML documents, and more.
ADO.NET follows a disconnected architecture, which means that it creates a separate connection to the database for each operation and then closes it once the operation is complete. This approach offers several advantages in terms of performance and scalability.
One of the core features of ADO.NET is the ability to execute SQL queries against a database. The framework provides a set of classes and methods that allow developers to build, execute, and retrieve data from SQL queries efficiently.
For developers leveraging ChatGPT-4, ADO.NET proves to be a valuable tool when it comes to querying data. Whether it's retrieving a list of users, filtering data based on specific criteria, or performing complex aggregate functions, ADO.NET offers the flexibility and efficiency needed to work with data efficiently.
Here's an example of how ChatGPT-4 can assist with querying data using ADO.NET:
using System;
using System.Data;
using System.Data.SqlClient;
public class DataQuery
{
public void QueryData()
{
string connectionString = "Your-Connection-String";
string query = "SELECT * FROM Customers WHERE Country = 'USA'";
using (SqlConnection connection = new SqlConnection(connectionString))
{
SqlCommand command = new SqlCommand(query, connection);
connection.Open();
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
Console.WriteLine(reader["CustomerName"]);
}
reader.Close();
}
}
}
In the above example, the ChatGPT-4 application connects to a SQL database using a connection string and executes a simple SQL query to retrieve all customers from the United States. The retrieved data is then processed and displayed as per the application's requirements.
ADO.NET provides various other functionalities and features that assist in handling data querying efficiently, such as parameterized queries, transaction support, and data manipulation capabilities.
Overall, ADO.NET proves to be a powerful technology for querying data, and ChatGPT-4 can make the most of it to provide efficient and accurate assistance with data querying tasks.
In conclusion, ADO.NET is a technology widely used for querying data from various data sources. Its flexible and efficient nature allows developers to retrieve and manipulate data with ease. Leveraging ADO.NET, ChatGPT-4 can provide valuable assistance in querying data, making it a reliable choice for developers working in this area.
Comments:
Thank you all for your interest in my article on Supercharging Data Querying with ChatGPT in ADO.NET Technology. I'm excited to hear your thoughts and answer any questions you may have.
Great article, Troy! ChatGPT seems like a powerful tool for enhancing data querying. Can you elaborate on how it helps in ADO.NET?
Thanks, Lisa! ChatGPT can assist in ADO.NET by providing a conversational interface for querying data. It allows users to submit queries in natural language and receive accurate results without the need to write complex SQL statements.
I'm curious about the performance of ChatGPT in ADO.NET. Does it introduce any latency in data retrieval?
Good question, David. While ChatGPT introduces some overhead due to natural language processing, it is designed to provide fast responses by leveraging efficient algorithms. In most cases, the latency impact is negligible.
The ability to query data using natural language sounds amazing, but are there any limitations or potential issues with ChatGPT in ADO.NET?
Indeed, Emily, there are a few limitations. ChatGPT relies heavily on the data it was trained on, so if the input query is outside its training data distribution or contains ambiguous requests, it may struggle to provide accurate results. It's important to provide clear and specific queries to maximize its effectiveness.
I'm concerned about security when using ChatGPT for data querying. How does it handle sensitive information?
Valid concern, Michael. ChatGPT works by executing queries against the underlying ADO.NET data sources, so it doesn't directly handle sensitive information. However, it's crucial to ensure that the underlying data sources have appropriate security measures in place.
Is there any customization available in ChatGPT for ADO.NET? Can it be trained on specific domains or adapt to different data schemas?
Yes, Sophia! ChatGPT can be fine-tuned on specific domains and data schemas. By training it on relevant datasets, you can improve its performance and customization to your specific requirements.
What are the hardware and software requirements for running ChatGPT in ADO.NET?
To run ChatGPT in ADO.NET, you'll need hardware capable of running. NET applications and sufficient memory to accommodate the model. Regarding software, the required dependencies include ADO.NET, GPT-3 SDK, and compatible frameworks.
Can ChatGPT assist with complex JOIN queries involving multiple tables in ADO.NET?
Absolutely, Jennifer! ChatGPT can handle complex JOIN queries by understanding the underlying relationships between tables. It can help you formulate and execute JOIN queries seamlessly.
How does ChatGPT handle data types and conversions in ADO.NET?
Great question, Robert. ChatGPT has built-in intelligence to handle data types and conversions in ADO.NET. It can assist in automatic type inference and perform the necessary conversions to ensure accurate data retrieval and manipulation.
Is ChatGPT limited to querying databases, or can it also interact with other types of data sources in ADO.NET?
ChatGPT can interact with a variety of data sources in ADO.NET, not just databases. It can also query file systems, web services, and other relevant data sources, making it a versatile tool for data retrieval and analysis.
Are there any licensing requirements or costs associated with using ChatGPT in ADO.NET?
ChatGPT operates under OpenAI's usage-based pricing model. You'll need valid API credentials to access its functionality. The exact costs depend on factors like usage volume and instance types.
Are there any plans to integrate ChatGPT directly into ADO.NET as a native feature in the future?
While I can't speak for ADO.NET's development roadmap, it's certainly a possibility. As ChatGPT gains more popularity and usage, native integration could be explored to provide even tighter integration with ADO.NET's capabilities.
How does ChatGPT handle large datasets in ADO.NET? Are there any scalability concerns?
When dealing with large datasets, ChatGPT's performance depends on the underlying ADO.NET data provider. As long as the data provider can efficiently handle large data volumes, ChatGPT should seamlessly integrate and scale accordingly.
Is there any potential for ChatGPT to suggest optimizations or alternative query approaches in ADO.NET?
Absolutely, Michael! ChatGPT can analyze queries and suggest optimizations or alternative approaches based on its understanding of the underlying data. It can help identify bottlenecks and propose efficient query formulations.
Can ChatGPT assist with data visualization in ADO.NET?
While ChatGPT's primary focus is on data querying, it can certainly provide insights and suggestions for visualizing data in ADO.NET. It can recommend relevant charts, graphs, or other visualization techniques to enhance data understanding.
Does ChatGPT support multi-threaded querying in ADO.NET?
Yes, Robert! ChatGPT supports multi-threaded querying in ADO.NET. It is designed to handle concurrent queries efficiently, ensuring optimal performance in a multi-user environment.
Are there any tutorials or resources available for getting started with ChatGPT in ADO.NET?
Certainly, Jennifer! The OpenAI documentation provides detailed guides and tutorials for integrating ChatGPT in various scenarios, including ADO.NET. Additionally, the ADO.NET community forums can be a valuable resource for support and sharing experiences.
Can ChatGPT handle real-time data streams in ADO.NET?
ChatGPT is primarily geared towards querying structured data. While it may not handle real-time data streams directly, it can still assist in retrieving and analyzing historical data from your real-time data sources in ADO.NET.
How does ChatGPT handle data source authentication and authorization in ADO.NET?
Good question, Lisa. ChatGPT leverages the authentication and authorization mechanisms available in ADO.NET. It can use the same credentials and security measures you employ for accessing your data sources, ensuring proper data protection.
Is ChatGPT compatible with older versions of ADO.NET or does it require specific versions?
ChatGPT should work with most versions of ADO.NET, but it's always recommended to use the latest stable versions to leverage any performance improvements or bug fixes that may have been introduced.
How does ChatGPT handle errors or unexpected inputs in ADO.NET?
If ChatGPT encounters an error or unexpected input, it can handle such situations gracefully by providing error messages or suggestions on how to refine the query. This helps users troubleshoot and overcome any issues they may encounter.
What are the benefits of using ChatGPT for data querying, compared to traditional SQL querying in ADO.NET?
ChatGPT introduces a more conversational and intuitive approach to data querying compared to traditional SQL. It allows users to express their queries in natural language, reducing the need for SQL expertise and enabling a wider range of users to interact with the data.
Can ChatGPT assist with data preparation tasks in ADO.NET, such as data cleansing or transformation?
While ChatGPT's primary focus is on data querying, it can offer insights and recommendations for data preparation tasks like data cleansing and transformation. It can suggest approaches or tools to improve data quality before querying.
Is there any feature in ChatGPT to prevent or detect SQL injection attacks in ADO.NET applications?
ChatGPT itself doesn't provide SQL injection prevention or detection mechanisms. However, it's crucial to implement best practices in your ADO.NET application and properly sanitize user inputs to mitigate the risk of SQL injection attacks.
Does ChatGPT maintain any query history or access logs in ADO.NET?
ChatGPT doesn't maintain query history or access logs within itself. However, if logging is enabled in your ADO.NET application, you can capture the queries and access logs through the existing logging mechanisms.
Can ChatGPT assist with optimizing database indexes in ADO.NET?
While ChatGPT may not directly optimize database indexes, it can provide recommendations and insights on query performance. By analyzing queries and their execution plans, it can suggest possible index optimizations to enhance performance.
How does ChatGPT handle concurrent queries from multiple users in ADO.NET?
ChatGPT is designed to handle concurrent queries in ADO.NET by leveraging multi-threading and efficient query execution methods. It ensures fair and efficient access to the underlying data sources, even in multi-user scenarios.
Are there any known performance bottlenecks when using ChatGPT in ADO.NET?
While ChatGPT strives for optimal performance, certain factors like the size of the conversation, complexity of the query, or underlying data source limitations can introduce performance bottlenecks. It's recommended to benchmark and optimize your application based on your specific requirements.
Can ChatGPT assist with automated report generation in ADO.NET?
Certainly, Susan! ChatGPT can provide insights and suggestions for automated report generation in ADO.NET. It can help you define queries, select appropriate data, and structure the report to enhance your reporting capabilities.
How does ChatGPT handle non-English queries in ADO.NET?
ChatGPT's ability to handle non-English queries in ADO.NET depends on its language support. While the model is predominantly trained on English, it can provide reasonable results for some non-English queries as long as they fall within the supported languages.
Can ChatGPT assist in query optimization by suggesting ways to minimize I/O operations in ADO.NET?
Absolutely, John! ChatGPT can analyze queries and suggest optimizations to minimize I/O operations in ADO.NET. By carefully evaluating the data requirements and query formulation, it can help reduce unnecessary data transfers and improve performance.
Does ChatGPT require any specialized hardware acceleration for optimal performance in ADO.NET?
ChatGPT's performance in ADO.NET largely depends on the underlying hardware capabilities. While specialized hardware acceleration can enhance performance, it is not mandatory. Modern CPUs and sufficient memory are generally sufficient to achieve satisfactory performance.
Can ChatGPT handle temporal or time-series queries in ADO.NET?
Absolutely, Emily! ChatGPT can assist in temporal or time-series queries in ADO.NET. It can help you retrieve and analyze data based on specific time ranges, perform date-based calculations, or identify patterns and trends within a time-series dataset.
Are there any performance benchmarks available for ChatGPT in ADO.NET to compare with traditional querying approaches?
While specific benchmarks comparing ChatGPT with traditional querying approaches in ADO.NET may not be readily available, it's recommended to conduct your own performance evaluations based on your specific use cases and requirements.
Is ChatGPT compatible with cloud-based data storage solutions in ADO.NET?
ChatGPT can be used with cloud-based data storage solutions in ADO.NET, as long as the data provider supports the necessary protocols and authentication mechanisms required for accessing the respective cloud services.
What kind of optimization techniques does ChatGPT employ for faster data retrieval in ADO.NET?
ChatGPT employs various optimization techniques in ADO.NET, such as query caching, result set pagination, and smart query execution planning. These techniques aim to reduce latency, optimize resource utilization, and improve overall performance.
Can ChatGPT assist with data modeling or schema design in ADO.NET?
While ChatGPT's primary focus is on querying, it can provide insights and recommendations for data modeling or schema design in ADO.NET. It can help you structure your data and define appropriate relationships to optimize querying and analysis.
Are there any known limitations around ChatGPT's query complexity or result set size in ADO.NET?
ChatGPT can handle various levels of query complexity in ADO.NET, but extremely complex or resource-intensive queries may pose challenges. Similarly, result set size can impact performance, so it's advised to optimize queries and limit result sizes to improve responsiveness.
Can ChatGPT assist in selecting appropriate data models or database designs in ADO.NET?
ChatGPT can provide recommendations and insights for selecting appropriate data models or database designs in ADO.NET. It can analyze requirements, understand relationships, and offer suggestions to help you make informed decisions.
Does ChatGPT support batch querying or handling multiple queries in a single request in ADO.NET?
While ChatGPT primarily operates on individual queries, you can certainly design your ADO.NET application to support batch querying or handle multiple queries in a single request. This allows you to optimize communication overhead and enhance overall efficiency.
Are there any security implications or vulnerabilities when using ChatGPT for data querying in ADO.NET?
When using ChatGPT for data querying in ADO.NET, it's essential to follow best security practices. Ensure proper authentication, authorization, and input validation to prevent any security implications or vulnerabilities. Additionally, regular updates and monitoring can help maintain a secure environment.
Can ChatGPT assist with automatically detecting and handling missing or incomplete data in ADO.NET?
While ChatGPT may not specifically handle missing or incomplete data detection and handling, it can provide insights and recommendations on data quality and integrity checks to help identify and address such issues in ADO.NET.
How does ChatGPT handle multi-language queries in ADO.NET?
ChatGPT supports multi-language queries in ADO.NET through its trained capabilities. However, the accuracy and performance for non-English queries may vary based on the available training data and language-specific nuances.
Are there any known performance trade-offs when using ChatGPT in ADO.NET applications?
While ChatGPT aims for optimal performance, there can be trade-offs depending on the complexity of queries and underlying data sources. It's important to conduct performance testing and optimizations specific to your use case to strike the right balance.
Can ChatGPT assist in query result analysis or provide insights on retrieved data in ADO.NET?
Absolutely, Emily! ChatGPT can help analyze query results and provide insights on retrieved data in ADO.NET. It can offer statistical summaries, identify patterns or anomalies, and suggest suitable visualization techniques to aid in data understanding.
Is there an upper limit on the number of concurrent queries that ChatGPT can handle in ADO.NET?
The upper limit on the number of concurrent queries that ChatGPT can handle in ADO.NET depends on various factors, including hardware resources, query complexity, and underlying data source limitations. It's recommended to benchmark and optimize based on your specific requirements.
Are there any existing applications or companies that have successfully used ChatGPT for data querying in ADO.NET?
While specific applications or companies using ChatGPT for data querying in ADO.NET may not be publicly available, the technology has shown promise in various domains. It's rapidly evolving, and more organizations are adopting it to enhance their data querying capabilities.
How does ChatGPT handle data privacy or compliance requirements in ADO.NET?
To handle data privacy and compliance requirements in ADO.NET, it's essential to adhere to established data protection practices at the data source level. ChatGPT itself doesn't introduce additional privacy concerns, but ensuring appropriate security mechanisms is crucial.
Can ChatGPT assist in optimizing query performance by suggesting appropriate indexes in ADO.NET?
ChatGPT can offer insights and recommendations to optimize query performance in ADO.NET, including suggestions for appropriate indexes. By understanding the query patterns and underlying data relationships, it can propose index optimizations to improve query execution.
What are the deployment options available for ChatGPT in ADO.NET?
ChatGPT can be deployed in ADO.NET applications by integrating with the OpenAI API and the necessary. NET frameworks. It can be hosted on-premises, in the cloud, or in a hybrid environment, depending on your requirements.
Does ChatGPT handle real-time collaboration features in ADO.NET? For example, multiple users working simultaneously on a shared query.
While ChatGPT doesn't inherently provide real-time collaboration features, you can incorporate such functionality in your ADO.NET application to enable collaborative query editing and execution. It depends on your specific application design and requirements.
Are there any known limitations in terms of query complexity or data volume when using ChatGPT in ADO.NET?
ChatGPT can handle a wide range of query complexities and data volumes in ADO.NET. However, extremely complex queries or large data volumes may impact performance and responsiveness. It's recommended to optimize queries, utilize pagination techniques, and leverage appropriate data processing mechanisms.
Can ChatGPT assist with data classification or clustering tasks in ADO.NET?
While ChatGPT's primary focus is on data querying, it can offer limited insights for data classification or clustering tasks in ADO.NET. It can suggest algorithms, review data attributes, and provide general guidance for those tasks.
Is ChatGPT compatible with all ADO.NET data providers, or are there any specific ones it works best with?
ChatGPT should work reasonably well with most ADO.NET data providers, as long as they comply with the ADO.NET standards. However, it's always recommended to test compatibility and performance with your specific data provider and configuration.
How does the cost of using ChatGPT in ADO.NET compare to traditional querying approaches?
The cost of using ChatGPT in ADO.NET depends on factors such as usage volume, API rates, and instance types. While it may introduce some additional costs compared to traditional querying approaches, it's important to evaluate the overall benefits and the value it brings to your organization.
Can ChatGPT assist with query optimization for distributed databases or federated query scenarios in ADO.NET?
ChatGPT can help optimize queries for distributed databases or federated query scenarios in ADO.NET. By understanding the data sources, query requirements, and available optimizations, it can assist in formulating efficient and effective distributed querying strategies.