Transforming Data Migration with ChatGPT: Leveraging LINQ Technology for Streamlined Results
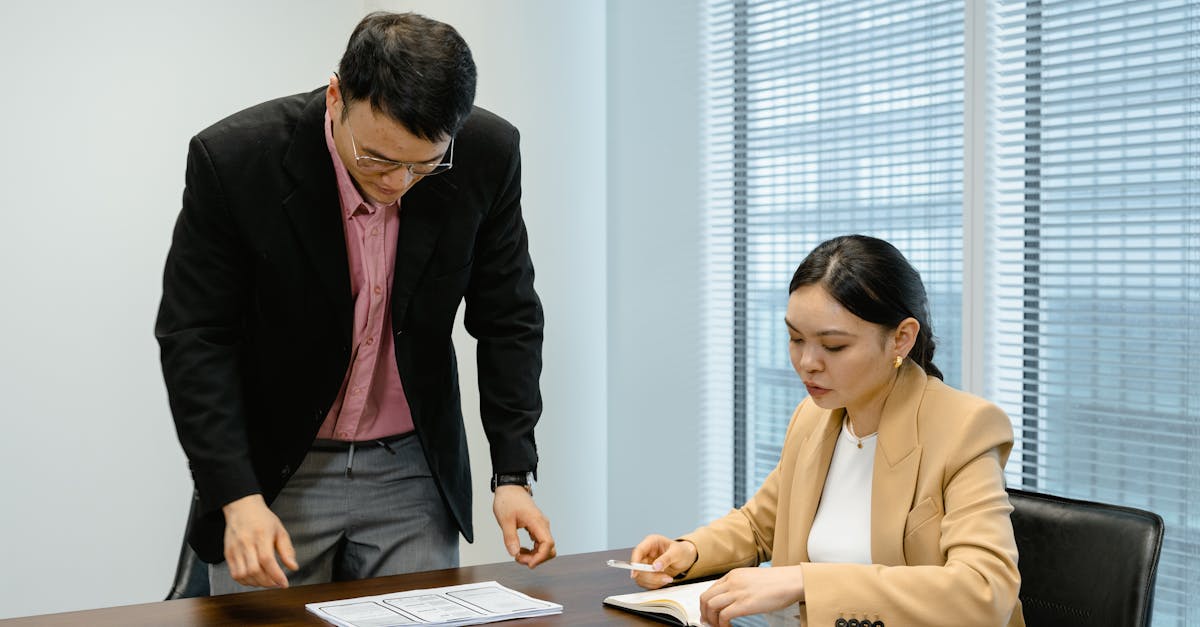
When it comes to data migration, the process of transferring data from one system to another, efficiency and accuracy are crucial. Migrating large amounts of data can be a daunting task, but with the right tools and technologies, it can be simplified and automated. One such tool is LINQ (Language Integrated Query).
What is LINQ?
LINQ is a powerful technology introduced in .NET Framework that provides a simplified and consistent way to query data across different data sources. It allows developers to perform queries on various data types such as databases, XML documents, and collections, using a SQL-like syntax.
How does LINQ help in data migration?
When migrating data, it is often necessary to transform and manipulate the data before transferring it to the target system. LINQ provides a flexible and intuitive way to perform these transformations using its query operators. These operators allow developers to filter, sort, group, and project data, among other operations.
For example, let's say we have a database table containing customer data with inconsistent date formats. We want to migrate this data to another system that expects dates in a specific format. Using LINQ, we can easily write a query that converts the dates to the desired format:
// Assuming customers is a collection of customer objects
var transformedData = from customer in customers
select new Customer
{
Name = customer.Name,
DateOfBirth = customer.DateOfBirth.ToString("yyyy-MM-dd")
};
In this example, LINQ allows us to iterate over the collection of customer objects, select the desired properties, and perform any necessary transformations on the fly.
Benefits of using LINQ for data migration:
1. Efficiency: LINQ provides optimized query execution, allowing for efficient processing of large datasets. It leverages the benefits of database indexes and other optimizations to ensure speedy data retrieval and manipulation.
2. Flexibility: LINQ supports various data sources including databases, XML documents, and in-memory collections. This flexibility allows developers to migrate data from different systems using a consistent approach.
3. Code readability: LINQ uses a declarative syntax that resembles standard SQL queries. This makes the code more readable and easier to understand, reducing the chances of errors and improving maintainability.
4. Integration with other technologies: LINQ is seamlessly integrated with other .NET technologies, such as Entity Framework and LINQ to XML. This integration enables developers to take advantage of additional features and tools when performing data migration tasks.
Conclusion
LINQ is a powerful technology that can greatly simplify and enhance the process of data migration. Its flexible query operators, efficient execution, and integration with other technologies make it an excellent choice for handling data migration tasks. By leveraging LINQ, developers can transform and manipulate data seamlessly, ensuring successful and accurate data migration.
Comments:
Great article! I found it very informative.
Thank you, Mary! Glad you found it helpful.
You're welcome, Mary! I'm happy to help.
Francois, your insights would be valuable here.
Robert, ChatGPT has shown excellent performance in natural language understanding tasks.
Absolutely, Francois. Would love to hear your thoughts.
Amanda, compared to other technologies, ChatGPT has achieved notable advancements in generating coherent and context-aware responses.
That's great to know, Francois! Thanks for the insights.
Francois, has ChatGPT been widely adopted in the industry for data migration purposes?
Amanda, ChatGPT has gained significant adoption in the industry, especially due to its ability to streamline complex data migration tasks. Many organizations have successfully leveraged it to accelerate their migration processes.
Yes, thank you, Francois. This discussion has been very helpful.
You're most welcome, Robert and Amanda! I'm glad I could provide valuable insights.
Francois, how does ChatGPT handle privacy and security concerns during data migration?
Mary, ChatGPT prioritizes privacy and security by offering options to anonymize or encrypt sensitive data during the migration process, minimizing risks.
That's reassuring. Thank you, Francois.
Francois, does ChatGPT offer any support for data migration tracking and monitoring?
Mary, ChatGPT provides features to track and monitor the progress of data migration tasks, allowing users to identify bottlenecks and ensure smooth operation.
Thank you, Francois! Your responses were very informative.
Absolutely, Francois. Monitoring is crucial.
That's great, Francois! ChatGPT covers all the necessary aspects.
Thank you, Francois! Your participation made a difference.
The LINQ technology sounds promising. Can't wait to try it out.
David, let me know how it goes when you try it!
This is exactly what I needed. Thank you for sharing!
Emily, I'm in the same boat. Hoping this will make things easier.
Impressive insights on data migration.
Michael, any specific insights that stood out to you?
I've been struggling with data migration lately. This could be a game-changer.
Sarah, definitely worth giving it a shot if you're facing challenges with data migration.
Agreed, Sarah.
Sure, will do!
Let's hope it's the solution we've been looking for.
The idea of leveraging LINQ for streamlined results caught my attention.
I'm hoping it will save us a lot of time during the migration process.
Are there any specific use cases where ChatGPT has excelled?
I'm curious to know how ChatGPT compares to other similar technologies.
Is the LINQ technology compatible with all database systems?
Mark, LINQ is primarily designed for Microsoft. NET Framework and works well with SQL Server, Oracle, MySQL, and other popular database systems.
Thank you for the clarification, Francois.
I'm interested in understanding the implementation details behind this.
Jennifer, LINQ enables developers to write queries using C# or Visual Basic directly in their code, minimizing the need for separate SQL syntax.
You're welcome, Jennifer! Let me know if you'd like more technical details.
I appreciate the offer, Francois. I'll reach out if I need more information.
Francois, we appreciate your expertise and prompt responses.
Thank you, Francois! Your input has been invaluable to all of us.
Has anyone tried ChatGPT with large-scale data migrations?
I haven't yet, Sarah. But I'm eager to explore its capabilities in that context.
Sarah, keep us updated if you decide to go for it.
I definitely will, James.
James, I'll be sure to share my experience with it.
Looking forward to hearing about it, David.
How does ChatGPT handle complex migration scenarios?
Robert, ChatGPT's ability to understand context helps it handle complex migration scenarios by generating more accurate and relevant recommendations.
That sounds promising. Thank you, Francois!
Francois, the ease of use and scalability of LINQ technologies are quite impressive.
Indeed, Olivia. LINQ technology simplifies database queries and adapts well to various project sizes.
Francois, does ChatGPT offer any data validation features during the migration process?
Olivia, ChatGPT can assist in data validation by suggesting error checks and validation rules based on the input data, enhancing the overall correctness of the migration.
Thank you, Francois! Your explanations were very clear.
Thank you, Francois! We've gained valuable knowledge from this conversation.
Absolutely, Olivia and Michael.
Olivia, I found the comparison with traditional methods particularly enlightening.
Glad it resonated with you, Michael.
Francois, how does ChatGPT handle data migration challenges involving different data formats?
Robert, ChatGPT handles data migration challenges by leveraging LINQ to transform, combine, or adapt data from different formats, ensuring seamless migration.
That's impressive! Thanks, Francois.
Thank you, Francois, for your insights!
That's fantastic! It would help in ensuring data integrity.
Indeed, Olivia. Ensuring data integrity is crucial during migration.
Are there any limitations or potential challenges when using LINQ technology for data migration?
Mark, while LINQ offers numerous benefits, one potential challenge can be performance issues with extremely large datasets. It's important to optimize queries and leverage LINQ providers to mitigate this.
Got it. Thanks for the heads up, Francois.
Francois, any recommendations for getting started with ChatGPT for data migration projects?
Emily, I recommend starting with small-scale projects to familiarize yourself with the technology. Then gradually expand to larger datasets while optimizing performance and exploring customization options.
That makes sense. Thanks for the advice, Francois.
Much appreciated, Francois. You've answered all our questions.
Much obliged, Francois! Your expertise and insights were top-notch.
Thanks, Francois! I appreciate your insights.
Good to know about the performance considerations. Thanks, Francois!
Thanks, Francois! This article and discussion have been insightful.
Francois, you've been an excellent resource. Thank you for your time.
That's fantastic! It would greatly help in maintaining control over the migration process.
That's great to hear! It seems ChatGPT is becoming the go-to solution for data migration.
Indeed, Robert. Its efficiency and accuracy make it a compelling choice.
Thank you all for the enlightening conversation!
Thank you, Robert! It's been a pleasure discussing this with all of you.
Much obliged, Francois! Your insights were invaluable.
Francois, your guidance throughout this discussion has been exceptional.
Indeed! It was great discussing this topic with everyone.
I'm happy to hear that, Amanda. Feel free to reach out if you have any further questions.
Thank you, Francois! It was a productive discussion.
Thank you, Francois! Your expertise and patience are highly appreciated.
Thank you all for your engagement and valuable comments! If you have any more questions or need further clarification, feel free to ask.
Francois, thank you for your time and expertise.
Thank you, Francois! We'll be sure to explore ChatGPT further.
Thank you, Francois! Your insights have been valuable.
Francois, this discussion has been enlightening. Thank you.
Francois, your expertise and guidance are greatly appreciated.
Thank you, Francois! It was a pleasure engaging with you.
Francois, your knowledge and willingness to help are commendable.
Thank you, Francois! We're grateful for your valuable contributions.
Francois, thank you for sharing your expertise and insights.
Much appreciated, Francois! This discussion has been enriching.
Francois, your contributions have been invaluable. Thank you.
Francois, your explanations and guidance have been exceptional. Thank you.
Thank you, Francois! We're grateful for your contributions to this discussion.