Unlocking the Potential: Harnessing ChatGPT's Power in Powershell's API Interaction
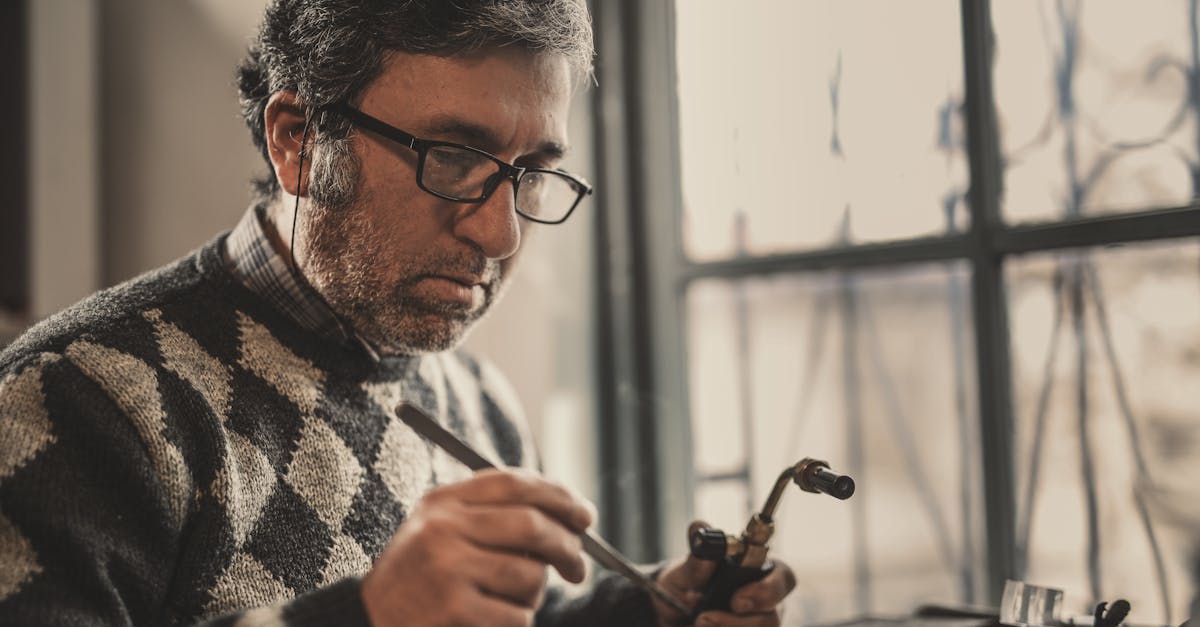
Powershell is a powerful scripting language that allows users to automate tasks and manage system configurations. One of its great features is the ability to interact with REST APIs, which enables users to access, submit, and manipulate data from various web services. With the help of ChatGPT-4, we can explore how to use Powershell commands to interact with REST APIs effectively.
Why use Powershell for API interaction?
Powershell provides a comprehensive set of tools and functions to interact with REST APIs. It has built-in cmdlets (command-lets) specifically designed for API communication. These cmdlets simplify the process of making API calls and handling the data returned by the APIs. Moreover, PowerShell's flexibility and scripting capabilities make it an ideal choice for automating API interactions and integrating them into your workflows.
Accessing API Data
The first step in interacting with REST APIs using Powershell is to establish a connection and retrieve the desired data. Powershell makes it easy to send HTTP requests and handle the responses.
$url = "https://api.example.com/data/endpoint"
$response = Invoke-RestMethod -Uri $url -Method Get
$data = $response | ConvertTo-Json
In the above example, we use the Invoke-RestMethod cmdlet to send a GET request to the specified API endpoint. The response is stored in the $response variable, which we can then manipulate further as needed. The ConvertTo-Json cmdlet is used to convert the response data into JSON format, which is commonly used for data exchange.
Submitting Data to APIs
Powershell also allows us to submit data to REST APIs using various HTTP methods such as POST, PUT, or PATCH. This is useful when we need to create or update records in the API's database.
$url = "https://api.example.com/data/endpoint"
$data = @{
"name" = "John Doe"
"email" = "johndoe@example.com"
"phone" = "123-456-7890"
}
$json = $data | ConvertTo-Json
$response = Invoke-RestMethod -Uri $url -Method Post -Body $json -ContentType "application/json"
In this example, we prepare the data to be submitted in a PowerShell hash table format. The ConvertTo-Json cmdlet is used again to convert the data into JSON format. The Invoke-RestMethod cmdlet is then used to send a POST request to the API endpoint with the JSON data in the request body. The response is stored in the $response variable for further processing.
Manipulating API Data
Once we have retrieved data from an API or received a response after submitting data, we can manipulate it according to our requirements.
For example, we can filter and extract specific values from the API response:
$filteredData = $response | Where-Object { $_.age -gt 18 }
In this example, we filter the API response data to only include records where the "age" field is greater than 18. The filtered data is stored in the $filteredData variable and can be further processed or displayed as needed.
Conclusion
With the help of ChatGPT-4, we have explored how to use Powershell commands to interact with REST APIs effectively. Powershell's built-in cmdlets and scripting capabilities provide a convenient way to access, submit, and manipulate data from various web services. By leveraging the power of Powershell and REST APIs, you can automate tasks, integrate systems, and streamline your workflows.
Comments:
Thank you all for taking the time to read my article on harnessing ChatGPT's power in Powershell's API interaction. I hope you found it informative and useful. I'm here to answer any questions you may have, so feel free to ask!
Great article, Mourad! I've been using Powershell for a while, and integrating ChatGPT in API interaction sounds promising. Can you elaborate on the potential use cases where this combination can be really helpful?
Thank you, Amanda! There are several exciting use cases where ChatGPT and Powershell can work together. For example, you can automate chat-based interactions with APIs, create intelligent chatbots, support natural language queries for your applications, and even generate code snippets based on conversational prompts. The possibilities are endless!
I'm a developer, and I've been exploring various ways to enhance user interactions in my applications. This integration seems interesting. How easy is it to set up ChatGPT with Powershell's API?
Hi Alex! Integrating ChatGPT with Powershell's API is relatively straightforward. OpenAI provides a user-friendly API that you can call using Powershell scripting. You'll need to register for an API key and familiarize yourself with the documentation, but once set up, you can leverage the power of ChatGPT within your Powershell scripts with ease.
I've been using Python to interact with APIs, but recently started exploring Powershell as well. What advantages does Powershell offer for API interaction compared to other scripting languages?
That's a great question, David! Powershell has several advantages over other scripting languages when it comes to API interaction. It is a native Windows shell and scripting language, which means it seamlessly integrates with Windows tools and processes, making it perfect for automation tasks in the Windows ecosystem. Powershell also has robust support for. NET and COM objects, giving you powerful ways to interact with APIs and other system resources.
Interesting article, Mourad! As a sysadmin, I'm always looking for ways to automate tasks. Can you provide an example of how ChatGPT can be used for API interaction in a sysadmin context?
Absolutely, Sarah! As a sysadmin, you can leverage ChatGPT in Powershell to automate tasks like troubleshooting common issues, generating reports, and even performing system configurations based on chat-based instructions. For example, you can build a chatbot that assists users with common support queries and provides automated solutions or recommendations based on the API responses.
This article got me interested in exploring more about ChatGPT and its integration possibilities. Is there a comprehensive tutorial or guide you would recommend to get started?
Glad to hear that, George! OpenAI provides extensive documentation and guides on using ChatGPT with various programming languages, including Powershell. I recommend checking out OpenAI's official documentation and their sample code repositories on GitHub to get started. They have some great examples that will help you understand the integration process and get you up and running quickly.
I'm curious about the limitations of using ChatGPT in Powershell's API interaction. Are there any potential challenges or drawbacks to be aware of?
Good question, Emily! While ChatGPT is a powerful tool, there are a few limitations to be aware of. It can sometimes generate incorrect or nonsensical responses, so it's important to validate and filter the output. Additionally, long conversations may receive incomplete replies due to token limitations. It's crucial to manage the conversation context and handle these limitations to ensure the best user experience. OpenAI provides guidelines and optimizations to address these challenges.
Thanks for sharing this informative article. I'm interested in exploring ChatGPT's capabilities further. Can you provide some insights into the model's training data?
You're welcome, Michael! ChatGPT is trained using a combination of supervised fine-tuning and Reinforcement Learning from Human Feedback (RLHF). The initial model is trained using human AI trainers who provide conversations and responses. It is also fine-tuned using comparison data, with multiple model responses ranked for quality. The training process is designed to align the model with human feedback and make it useful and safe for various applications.
This article has opened up new possibilities for me. I'm excited to dive deeper into ChatGPT and its integration with Powershell. Thank you, Mourad!
Can you provide some performance insights when using ChatGPT in Powershell's API interaction? Are there any latency concerns?
Certainly, Tom! When making API calls to ChatGPT, you can expect some latency depending on factors like network connection and the complexity of the conversation. The time taken for the API call and response can vary, so it's important to consider this while implementing it in time-sensitive scenarios. However, OpenAI provides recommendations to mitigate latency and optimize the performance of your ChatGPT interactions.
Interesting read! How can one manage the cost aspect while using ChatGPT in Powershell's API interaction? Are there any pricing considerations or best practices to follow?
Hi Olivia! Cost management is an essential aspect when using ChatGPT's API. OpenAI provides detailed pricing information on their website, which includes factors like the number of tokens used in requests and the type of API call made. To manage costs effectively, you can control the number of tokens used by truncating or omitting some parts of the conversation history when making an API call. OpenAI also offers a guide on cost optimization that you may find useful.
I'm impressed by the potential capabilities of ChatGPT in Powershell's API interaction. Are there any security measures to consider when using this integration?
Absolutely, Sophia! Security is a crucial aspect of any integration. When using ChatGPT's API in Powershell, ensure you handle any user input with appropriate sanitization and validation to prevent any security vulnerabilities. It's also crucial to follow best practices for secure data storage, especially if your scripts deal with sensitive information. By adopting robust security measures, you can confidently harness the power of ChatGPT and Powershell together.
This article has piqued my interest in exploring ChatGPT with Powershell. Do you have any recommended resources to learn more about Powershell scripting?
Glad to hear that, Emma! Powershell is a versatile scripting language, and there are plenty of great resources available to learn and enhance your skills. I recommend checking out the official Microsoft documentation for Powershell, where you'll find tutorials, guides, and code examples. Additionally, websites like Stack Overflow have active communities that can help answer specific questions and provide valuable insights.
I'm amazed by the possibilities that ChatGPT and Powershell's integration offers. Can you share any real-world examples where this combination has been successfully implemented?
Certainly, Samuel! Several organizations have successfully integrated ChatGPT with Powershell to enhance their workflows. For instance, companies have developed intelligent chatbots that handle customer queries and automate support processes. Some have used this integration for PowerShell-based automation in system administration and DevOps tasks, where interactive and dynamic interactions are required. The combination of ChatGPT and Powershell opens up a wide range of possibilities for various domains.
This article provides a great introduction to ChatGPT and its applications in Powershell. What are some potential future advancements or developments we can expect in this field?
Great question, Liam! As AI and natural language processing continue to advance, we can expect even more sophisticated interactions and capabilities when using ChatGPT in Powershell. Improved models may provide more accurate responses and better context understanding. OpenAI is actively working on research and development in this field, and we can anticipate exciting advancements that will further enhance the integration between ChatGPT and Powershell.
Thanks for sharing your insights, Mourad! This article has been really helpful. I'm excited to explore ChatGPT and its integration with Powershell.
You're welcome, Daniel! I'm glad you found the article helpful. Feel free to reach out if you have any questions or need any assistance while exploring ChatGPT and Powershell's integration. Happy exploring!
This article has sparked my curiosity about ChatGPT's potential in Powershell. How accessible is ChatGPT for someone new to AI and natural language processing?
Hi Isabella! ChatGPT can indeed be accessible for someone new to AI and natural language processing. While prior experience can be helpful, OpenAI has made efforts to make ChatGPT easy to use and integrate with various programming languages, including Powershell. By following their documentation and guides, even those new to the field can effectively leverage the power of ChatGPT within their Powershell scripts.
I'm interested in understanding how ChatGPT performs with different types of APIs and data formats. Are there any limitations or specific considerations to keep in mind?
Great question, Ryan! ChatGPT is versatile when it comes to working with different APIs and data formats. However, it's essential to format the API requests and responses properly to ensure compatibility with ChatGPT. Depending on the specific data formats and APIs you are working with, you may need to handle data parsing and serialization appropriately. OpenAI's documentation and sample code can provide valuable guidance for working with different APIs and data types.
I appreciate the insights shared in this article, Mourad! ChatGPT's integration with Powershell seems very promising. Could you provide some tips on effectively managing conversations and maintaining context during API interactions?
Absolutely, Grace! Managing conversations and maintaining context is crucial for effective API interactions with ChatGPT. OpenAI recommends including important context from prior messages in the conversation history. It helps to keep the conversation history concise yet relevant, ensuring that the model understands the context accurately. By effectively managing the conversation whilst providing relevant prompts, you can have more meaningful interactions with ChatGPT in your Powershell scripts.
As a developer, I'm always concerned about the resource utilization and scalability of integrated tools. Can you shed some light on the resource requirements when using ChatGPT in Powershell's API integration?
Certainly, Lily! When using ChatGPT in Powershell's API integration, you need to consider the resource utilization aspects. The API call requires an active internet connection, and you need to manage network latencies and response timings. Additionally, since you'll be leveraging the OpenAI API, ensure you have the necessary authentication and authorization mechanisms in place. Consider scalability by monitoring resource utilization and choosing appropriate OpenAI API plans based on your integration requirements.
This article has provided valuable insights, Mourad. I'm curious about the ongoing advancements in Natural Language Processing. How often is ChatGPT updated or fine-tuned?
Thanks, Ellie! OpenAI regularly updates and improves their models, including ChatGPT. They take user feedback and iteratively deploy model updates to address limitations and improve performance. So, you can expect continuous advancements and enhancements in the future. OpenAI's collaborative approach encourages user feedback to make the models even more beneficial for various applications, including Powershell integration.
As a PowerShell enthusiast, I'm thrilled to see the integration possibilities of ChatGPT. Are there any recommended practices to ensure secure user interactions during API calls?
Absolutely, Hayden! Security is crucial when interacting with APIs, especially in chat-based interactions with users. It's essential to validate and sanitize user inputs to prevent common vulnerabilities like injection attacks. You should also consider rate limiting to prevent abuse or excessive API calls. Additionally, OpenAI provides guidelines on safety and usage to help ensure secure and responsible interactions while using ChatGPT in your Powershell API integration.
I'm intrigued by the possibilities of integrating ChatGPT with Powershell for automation. Can you provide any insights into how the combination can benefit DevOps processes?
Certainly, Lucas! The combination of ChatGPT and Powershell opens up exciting opportunities in DevOps processes. You can automate tasks like provisioning cloud resources, managing deployments, and interacting with CI/CD pipelines using chat-based instructions. By integrating ChatGPT with Powershell's API interaction, you can streamline and optimize your DevOps workflows while having interactive and dynamic conversations within your scripts.
This article has been really insightful, Mourad. Can you share any tips on optimizing the performance of ChatGPT in Powershell's API interaction?
Absolutely, Sophie! To optimize the performance of ChatGPT in Powershell's API interaction, one key tip is to truncate or omit unnecessary parts of the conversation history to reduce the total tokens used in the API call. This can help manage the costs as well. Additionally, you can experiment with different values for 'max_tokens' parameter while making API calls to strike the right balance between response length and context. OpenAI's documentation provides more in-depth guidelines to optimize performance.
I'm curious about the supported types of APIs that work well with ChatGPT in Powershell. Could you shed some light on the compatibility aspect?
Certainly, Ashley! ChatGPT can be used with a wide range of APIs and services in Powershell. It can interact with RESTful APIs, SOAP-based web services, cloud provider APIs (like Azure or AWS), and even custom PowerShell Cmdlets that wrap specific API functionality. As long as you structure the API calls properly and handle the responses, you can leverage ChatGPT in conjunction with almost any API that Powershell can interact with.
This article has sparked my interest in exploring ChatGPT's integration with Powershell. Can this combination be used to develop voice-activated automation or chatbots?
Absolutely, Emma! The combination of ChatGPT and Powershell can indeed be used to develop voice-activated automation or chatbots. By integrating ChatGPT with voice recognition APIs and leveraging Powershell's audio processing capabilities, you can build voice-activated applications that respond to user input and provide intelligent conversational interactions. This opens up exciting possibilities for voice-controlled automation and chatbot development using the powers of ChatGPT and Powershell together.