Unlocking the Potential of ChatGPT in Concurrent Programming with C++ Language
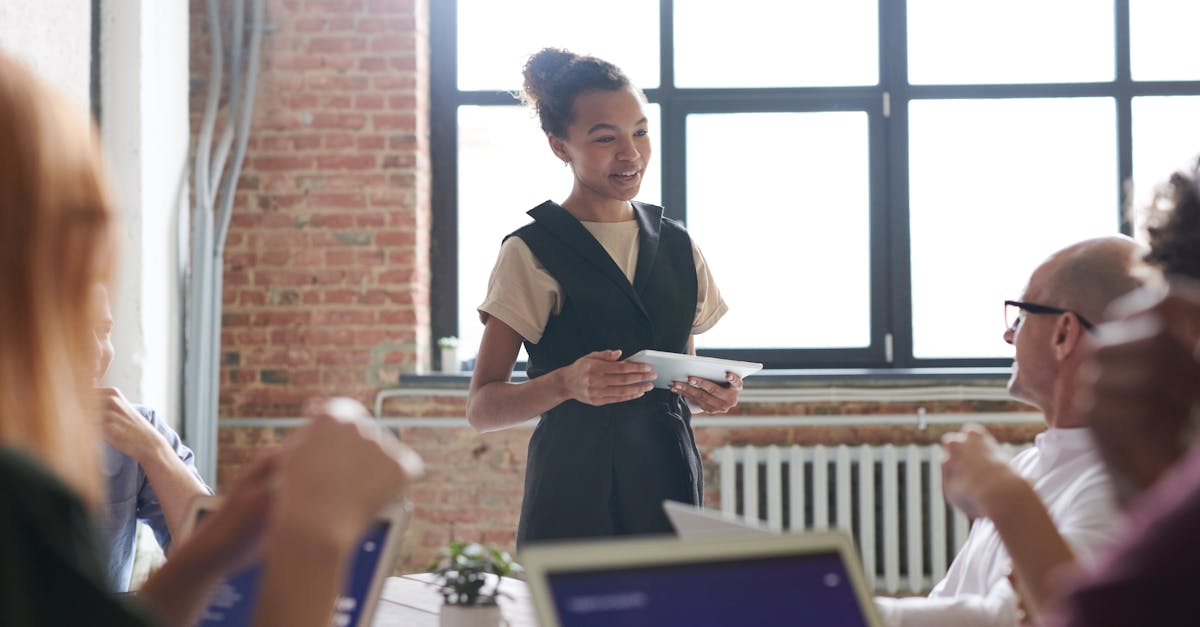
Introduction
Concurrent programming plays a significant role in developing robust and efficient software systems. It involves executing multiple tasks or threads simultaneously, allowing for improved performance and better resource utilization. C++ is a powerful programming language that offers excellent support for concurrent programming, making it an ideal choice for developing multi-threaded applications.
Concurrency in C++
Concurrency in C++ is primarily achieved through the use of threads. A thread is an independent sequence of instructions that can run concurrently with other threads. C++ provides built-in support for creating, managing, and synchronizing threads using the Standard Library's threading facilities.
The C++ threading facilities allow developers to create multiple threads within a program and coordinate their execution. Threads can be used to perform tasks in parallel, enabling faster execution and improved responsiveness. Additionally, threads can communicate with each other through shared data or message passing, enabling coordination and synchronization between concurrent tasks.
Benefits of Concurrent Programming in C++
Using concurrent programming in C++ offers several benefits:
- Improved Performance: Concurrent programming allows for parallel execution of tasks, leading to significant performance gains. C++ provides low-level control over thread creation and management, enabling developers to fine-tune performance optimizations.
- Better Resource Utilization: Concurrent programs can make efficient use of available system resources by distributing work among multiple threads. This leads to enhanced resource utilization and reduced resource contention.
- Responsiveness: Concurrent programming makes it possible to perform time-consuming tasks in the background while keeping the application responsive to user interactions. This can greatly improve the user experience.
- Fault Isolation: By encapsulating concurrent tasks within separate threads, developers can isolate potential issues, making it easier to debug and maintain the codebase.
Concurrency Challenges and Management
While concurrent programming offers many benefits, it also introduces certain challenges that developers must address. Some common challenges include:
- Race Conditions: Race conditions occur when multiple threads access shared data simultaneously, leading to unpredictable and incorrect behavior. Proper synchronization techniques such as mutexes or locks must be employed to prevent race conditions.
- Deadlocks: Deadlocks can occur when threads are waiting for resources that are held by other threads, resulting in a situation where all threads are blocked and unable to proceed. Careful design and usage of synchronization primitives can help avoid deadlock situations.
- Thread Safety: Ensuring thread safety requires careful consideration of shared data and their access patterns. Proper synchronization mechanisms and techniques must be employed to guarantee consistent and correct results.
Conclusion
C++ concurrent programming provides developers with powerful tools for writing and managing multi-threaded applications. By leveraging the language's threading facilities, developers can create efficient, responsive, and scalable software systems. However, it is crucial to understand the challenges associated with concurrent programming and utilize appropriate synchronization techniques to ensure correct, reliable, and performant applications.
With its robust support for concurrent programming, C++ remains a top choice for developers seeking to build high-performance and scalable applications that can effectively utilize modern multicore systems.
Comments:
Great article! I'm really excited about the potential of ChatGPT in concurrent programming with C++. It has the potential to revolutionize the way we develop software.
I have to agree, Kate. ChatGPT's capabilities combined with the power of the C++ language can open up endless possibilities for concurrent programming. This is definitely an area to watch!
I'm not entirely convinced. While ChatGPT is impressive, I think the complexity involved in concurrent programming might still pose challenges. What are your thoughts?
I understand your concerns, Sarah. Concurrent programming can be complex, but with the help of ChatGPT, developers can get assistance in handling the intricacies. It won't eliminate the challenges completely, but it can certainly make it easier.
I can see the potential, but I wonder if it might also introduce new challenges. How do you ensure that the chatbot understands the specific concurrency requirements of a given system?
That's a valid point, Adam. It's important to train and fine-tune the ChatGPT model using domain-specific data to ensure it understands the concurrency requirements. It will require careful validation and testing.
The possibilities are exciting, but I wonder about the scalability. Could ChatGPT handle large-scale concurrent programming projects without performance issues? What are your thoughts?
Excellent question, Linda. Scalability is indeed a concern. While ChatGPT has its limitations, it can assist developers in understanding and designing concurrent programming solutions. Performance optimization will still require manual effort and expertise.
I'm curious to know if anyone has already tried implementing ChatGPT in C++ concurrent programming projects. Are there any success stories or real-world case studies?
As far as I know, there haven't been many case studies published yet. However, some developers have started experimenting with ChatGPT in C++ concurrent programming, and initial results look promising. It would be great to see more practical examples and experiences shared.
I think the potential of ChatGPT in concurrent programming is fascinating. It can provide helpful suggestions and insights, acting as an intelligent assistant during the development process. It's like having an experienced mentor right by your side!
While ChatGPT can be a useful tool, I believe it shouldn't replace the developer's expertise and critical thinking. It's important to approach it as a helpful assistant rather than a substitute for human intelligence.
I'm excited to see how ChatGPT evolves in concurrent programming. It has the potential to enhance collaboration and knowledge-sharing among developers, making the development process more efficient and effective.
In terms of the practical implementation, how do you envision integrating ChatGPT into existing C++ development workflows?
That's an important question, Sarah. Integration might involve creating dedicated plugins or extensions for popular IDEs, allowing developers to interact with ChatGPT seamlessly within their familiar development environment.
Another approach could be integrating ChatGPT into collaborative coding platforms, enabling developers to ask questions and get suggestions from the chatbot while working on shared codebases.
The collaborative potential is indeed exciting. ChatGPT's ability to assist multiple developers simultaneously could lead to more efficient and streamlined collaboration, especially in large-scale projects.
To fully unlock the potential of ChatGPT in concurrent programming, it will be crucial to provide developers with an intuitive interface and ensure it understands crucial concepts of concurrent programming languages like C++. Otherwise, the assistance might be limited.
I'm really intrigued to see how ChatGPT's responses would differ based on the specific concurrent programming paradigms like multithreading and multiprocessing. It would be interesting to explore its strengths and limitations in different contexts.
That's a great point, David. Different concurrent programming paradigms require different approaches and considerations. It would be valuable to see ChatGPT adapt to these variations and provide appropriate guidance.
Indeed, Sarah. The ability of ChatGPT to grasp the nuances of specific concurrent programming paradigms would make it a more valuable resource for developers regardless of the paradigm they are working with.
I can see how ChatGPT can help both experienced and novice developers in understanding and navigating the challenges of concurrent programming. It can be a valuable learning tool as well.
While ChatGPT can be immensely helpful, developers should be cautious not to overly rely on it. It's crucial to maintain critical thinking and validate the suggested solutions as per specific project requirements.
Thank you all for the valuable comments and insights! It's great to see the enthusiasm and thoughtful discussions around the potential of ChatGPT in concurrent programming with C++. Keep the conversation going!
This article presents an exciting concept! The combination of ChatGPT and C++ in concurrent programming can reduce development time and enhance code quality. I can't wait to explore its practical applications!
Thank you all for reading my article on unlocking the potential of ChatGPT in concurrent programming with C++! I'm excited to discuss this topic with you.
Great article, Amanda! I've been using C++ for concurrent programming for a while, and I'm curious to learn how ChatGPT can be applied in this context.
Thanks, Steve! ChatGPT can help in concurrent programming by assisting with debugging, code completion, and generating parallelization suggestions, among other things. It can act as a helpful assistant to programmers.
Interesting topic indeed! I'm still exploring concurrent programming with C++, so I'm looking forward to gaining insights from both the article and the discussion.
That sounds like a powerful tool! By leveraging the capabilities of ChatGPT, it could potentially enhance productivity and reduce coding errors in concurrent programming.
I'm skeptical about the effectiveness of ChatGPT in concurrent programming. How can it understand the intricacies and challenges of parallel execution?
Good point, Michael. While ChatGPT may not possess domain-specific knowledge out of the box, it can be trained on relevant data and obtain a reasonable understanding of concurrent programming concepts, allowing it to provide useful suggestions and insights.
I see. So, training ChatGPT on a large dataset of concurrent programming examples could enable it to better grasp the nuances and intricacies.
But how do we ensure that ChatGPT generates correct and efficient parallelization suggestions?
Valid concern, Michael. It's important to review and validate any suggestions generated by ChatGPT. Ultimately, human expertise should guide the final decisions to ensure correctness and efficiency.
I'm curious how ChatGPT can handle the complexities of multi-threading and synchronization in concurrent programming.
That's a great point, Linda. While ChatGPT may not have practical experience with multi-threading, it can learn from vast amounts of information and provide insights regarding best practices, common pitfalls, and synchronization techniques.
Ah, I see. So, it can serve as a knowledge base for concurrent programming, helping programmers make informed decisions.
Can ChatGPT assist in optimizing the performance of concurrent programs?
Indeed, Oliver! ChatGPT can provide suggestions for optimizing parallel execution, such as identifying potential bottlenecks, recommending data structures, or proposing loop parallelization techniques.
That's impressive! It seems like ChatGPT can be a valuable aid in improving the efficiency of concurrent programs.
Are there any limitations or challenges when applying ChatGPT to concurrent programming in C++?
Great question, Sophia. ChatGPT's responses may not always be accurate or ideal, especially with complex or novel situations. It might also lack real-time knowledge of evolving programming practices. Human judgment is crucial in weighing the suggestions provided by ChatGPT.
So, it's important to treat ChatGPT's suggestions as helpful insights rather than strict instructions.
Exactly, Sophia! Treating the suggestions as a starting point and applying critical thinking is essential to ensure the correctness and appropriateness of the generated parallel programming solutions.
Are there efforts to develop specialized models or fine-tune ChatGPT specifically for concurrent programming tasks?
Absolutely, Benjamin! Researchers are actively working on adapting and fine-tuning ChatGPT models for specific domains and tasks, including concurrent programming. This specialization can lead to even more accurate and targeted suggestions.
That's great news! Looking forward to seeing the advancements in this area.
ChatGPT seems interesting, but to what extent can it actually speed up the development process in concurrent programming?
Good question, Sarah. While ChatGPT can speed up tasks like code completion and providing suggestions, its impact on the overall development process might vary depending on the programmer's familiarity with concurrent programming concepts and the complexity of the task at hand.
I see. So, it can be more beneficial for less experienced programmers, but experienced programmers might still find value in its assistance.
How does ChatGPT handle performance concerns while providing immediate assistance?
Good point, Robert. ChatGPT can provide immediate assistance by leveraging pre-trained models and caching results for commonly encountered scenarios. The system can prioritize quick responses, but it's important to note that its immediate suggestions might not always be the most optimized ones.
Understood. So, it's necessary to validate and refine ChatGPT's initial suggestions to improve performance further.
How can privacy concerns be addressed when utilizing ChatGPT for concurrent programming?
Privacy is crucial, Lucy. To address concerns, organizations can run ChatGPT locally on their own infrastructure or use privacy-focused models that minimize data exposure. Anonymizing code examples during training is another approach to protect proprietary information.
That's reassuring. It's essential to ensure the security and confidentiality of sensitive code while utilizing the capabilities of ChatGPT.
Can ChatGPT provide explanations for its suggestions in concurrent programming?
Absolutely, Hannah! ChatGPT can provide explanations for its suggestions, detailing the reasoning behind them. This transparency helps programmers understand the suggestions better and gain insights into concurrent programming techniques.
That's wonderful. Interactive explanations can contribute to knowledge transfer and help programmers improve their concurrent programming skills.
How accessible is ChatGPT for programmers who are visually impaired or prefer command-line interfaces?
Accessibility is important, Emily. ChatGPT can be used via command-line interfaces and integrated with screen readers or other accessibility tools to support visually impaired programmers. Its versatility allows for various interaction methods.
That's great to hear. Inclusivity and accessibility are important aspects when incorporating AI tools into programming workflows.
I'm eager to try out ChatGPT in my concurrent programming projects! Where can I find more resources and examples?
Glad to hear that, Steve! OpenAI's platform provides resources and documentation on using ChatGPT. You can explore their guides, sample code, and community forums to find more information and connect with other developers. Best of luck with your projects!
ChatGPT sounds promising for concurrent programming. Are there any real-world success stories or case studies?
Indeed, Julia! While ChatGPT is still a relatively new tool, there are case studies and success stories emerging in various domains. OpenAI offers resources highlighting different use cases and experiences that demonstrate the potential value of ChatGPT in real-world scenarios.
I'll definitely check them out. Real-world examples can provide great insights into the practical applications of ChatGPT in concurrent programming.
Are there any known limitations or biases to be aware of when using ChatGPT for concurrent programming?
Great question, Ethan. ChatGPT can sometimes produce responses that are plausible-sounding but incorrect or biased. It's important to exercise caution and critically evaluate the suggestions. OpenAI actively encourages feedback from users to tackle these limitations and biases.
I appreciate the transparency. User feedback plays a crucial role in refining and improving AI systems.
Can ChatGPT assist in learning parallel programming concepts for those new to concurrency?
Absolutely, Nathan! ChatGPT can provide explanations, offer insights into parallel programming concepts, and even help with code samples. It can be a valuable learning tool for those new to concurrency, guiding them in understanding and applying parallel programming principles.
That's fantastic. Combining learning capabilities with the assistance of ChatGPT can greatly benefit aspiring concurrent programmers.
Thank you all for the engaging discussion and your insightful questions! I hope this article and our conversation have shed light on the potential of ChatGPT in concurrent programming with C++. Keep exploring and leveraging AI tools to enhance your programming journey!