Using ChatGPT for Code Organization in Microsoft Visual Studio C++
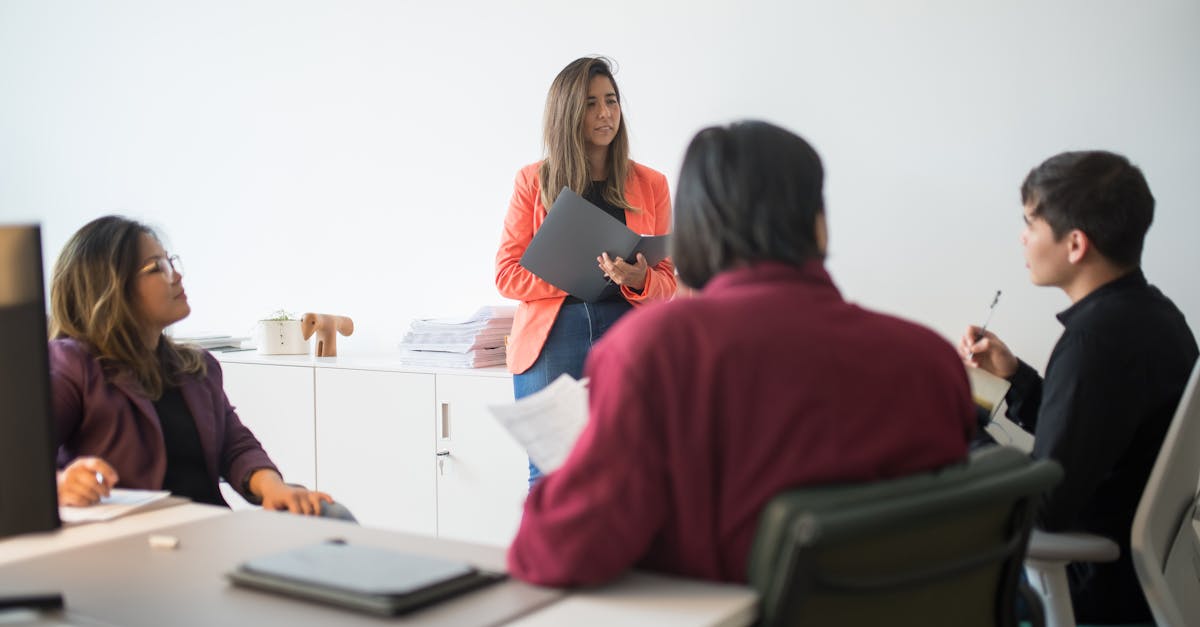
Organizing code is a crucial aspect of software development. It not only ensures readability and maintainability but also enhances collaboration and reduces complexities in the long run. In this article, we will explore various techniques and best practices for organizing your codebase in Microsoft Visual Studio C++.
1. File Structure
Creating a logical and consistent file structure is the first step towards well-organized code. Divide your codebase into meaningful folders based on functionality or modules. For example, you can have separate folders for headers, source files, libraries, tests, and documentation. This modular approach will make it easier to locate and manage specific components of your project.
2. Naming Conventions
Follow a consistent and intuitive naming convention for your files, classes, functions, and variables. Use descriptive names that accurately convey the purpose or functionality. Avoid generic or ambiguous names that can cause confusion. Consistency in naming conventions across the codebase ensures clarity and improves readability.
3. Modularization
Break down your code into smaller, reusable modules. Each module should have a single responsibility and encapsulate related functionality. This approach promotes code reusability and makes it easier to update or maintain specific components without affecting the entire codebase. Use namespaces to group related modules together.
4. Class Organization
When organizing classes, consider the Single Responsibility Principle (SRP). Each class should have a well-defined purpose and encapsulate a single functionality. Group related classes together, such as base classes, derived classes, or classes implementing a specific interface. Utilize inheritance and composition wisely to establish relationships between classes.
5. Function and Method Order
Arrange your functions and methods in a logical order within a class or file. Consider grouping related functions or methods together, such as constructors, destructors, getters, setters, and utility functions. This arrangement improves code readability and makes it easier to locate and understand the functionality of each function.
6. Code Documentation
Document your code to provide clarity and context to other developers. Use comments to explain the purpose and functionality of classes, functions, and critical code segments. Ideally, follow a consistent commenting convention throughout the codebase. Consider using tools like Doxygen to generate API documentation automatically.
7. Version Control Integration
Integrate your codebase with a version control system, such as Git, to manage changes and track the history of your code. Proper version control allows you to create branches, merge changes, and roll back to previous versions if necessary. Maintain a clear and well-defined branching strategy to ensure code stability.
8. Build System Configuration
Define a robust build system configuration to compile, build, and package your codebase. Utilize build tools like CMake, MSBuild, or Visual Studio's built-in tools. Configure build scripts and makefiles to automate the build process and specify dependencies. This ensures consistency and ease of deployment across different environments.
9. Error Handling and Logging
Implement a consistent error handling mechanism to handle exceptions, errors, and unexpected scenarios. Use appropriate error codes or exceptions based on the context. Incorporate logging mechanisms to track and log important events, errors, and debugging information. This helps in troubleshooting and diagnosing issues efficiently.
10. Testing and Debugging
Include unit tests, integration tests, and debugging capabilities within your codebase. Write test cases to validate the functionality of individual components and ensure proper integration between modules. Leverage debugging tools provided by Visual Studio C++ to identify and fix issues efficiently.
By following these code organization practices in Microsoft Visual Studio C++, you can significantly improve the readability, maintainability, and collaboration within your codebase. Utilize the power of a well-organized codebase to streamline your development process and build robust software systems.
Comments:
Thank you all for taking the time to read my article on using ChatGPT for code organization in Visual Studio C++. I hope you found it informative and helpful. If you have any questions or comments, feel free to post them here!
Great article, Chris! I've been using Visual Studio C++ for a while, and this approach seems like a very innovative way to streamline code organization. I'll definitely give ChatGPT a try!
Hey Alice, have you tried using ChatGPT with Visual Studio C++ yet? I'm curious to know how well it integrates with the IDE.
Hi Daniel! Not yet, but I plan to give it a shot soon. I'll be sure to share my experience once I've tried it out. From what I've read, it seems like a promising tool for code organization.
Thanks, Alice! I'll keep an eye out for your experience with it. Exciting times for C++ developers!
Thanks for sharing your insights, Chris! I've always struggled with code organization, and this technique looks promising. Excited to delve deeper into ChatGPT for helping me with my C++ projects!
Bob, I'm in a similar boat. Code organization has always been a challenge for me, too. Let's give ChatGPT a try and share our experiences here!
Charlie, I'm up for that! Let's share our insights once we've explored ChatGPT in Visual Studio C++. Collaboration can make the learning process smoother.
Absolutely, Bob! Collaboration is key in this field. Looking forward to exchanging insights with you.
Charlie, definitely! Feel free to drop me a message whenever you're ready to discuss our findings. Collaboration can lead to great breakthroughs!
Bob, I've been testing ChatGPT with C++ code snippets, and so far, it has been quite impressive. I'd be happy to share my findings and experiment with you!
Bob, sounds good! I'll definitely reach out to you once I have some results to discuss. Collaboration makes the learning process more thorough and insightful.
Charlie and Bob, count me in too! Let's learn and explore together. Collaboration always leads to better outcomes.
Charlie and Eve, I'm thrilled to have you on board for our collaboration. Let's make the most out of this opportunity and help each other elevate our C++ projects!
Bob, sounds like a plan! Let's test ChatGPT's abilities and discuss how it responds to various code organization scenarios in C++. Looking forward to exchanging insights.
This article couldn't have come at a better time! I've been searching for ways to improve code organization, and the combination of Visual Studio C++ and ChatGPT sounds fascinating. Thanks, Chris!
Eve, I agree! This article is a gem. I've been struggling with maintaining large C++ projects, and ChatGPT seems like a potential game-changer. Can't wait to give it a go!
Sure, Frank! Collaborating and sharing experiences will help us leverage the true potential of ChatGPT in our C++ projects. Let's stay connected!
George, absolutely! Sharing insights and experiences is invaluable. Together, we can navigate through complex C++ projects with more efficiency and ease.
Frank, completely agree with you. Collaboration is crucial, especially when exploring new tools like ChatGPT. Looking forward to sharing our progress!
George, definitely! Collaboration fuels progress, and I'm excited to witness the collective growth we can achieve by leveraging ChatGPT for our C++ projects.
Frank, absolutely! Together, we can navigate through complexities and reach new heights in coding proficiency with the assistance of ChatGPT. Exciting times ahead!
Charlie, I'm eager to share our findings and insights. Let's dive in and experiment with ChatGPT's capabilities in C++ code organization!
Bob, I'm thrilled to embark on this journey with you. Let's discover the possibilities of code organization using ChatGPT and share our discoveries!
Eve, this seems like a powerful tool, especially when dealing with complex C++ projects. Let's give it a shot and compare notes!
It's great to see such positive feedback! I believe ChatGPT can make a significant difference in code organization and developer productivity. Looking forward to hearing more about your experiences!
Chris, do you have any specific tips on using ChatGPT effectively for code organization in Visual Studio C++? Many of us would appreciate some practical advice.
Alice, certainly! One tip is to provide clear and concise prompts to ChatGPT, outlining your code organization requirements. Additionally, regular interactions with the model help fine-tune its understanding and response accuracy. Experimentation is key!
Chris, thanks for the advice! I'll make sure to provide clear prompts and engage in regular interactions with ChatGPT to get the best out of it.
Thanks, Chris! I'll keep those tips in mind while using ChatGPT. Looking forward to seeing how it improves my code organization workflow.
Chris, any advice on the size of code snippets to feed ChatGPT? Should we aim for smaller portions or can we provide larger segments without affecting its performance?
Alice, I've heard mixed reviews about ChatGPT's performance with C++ projects. I'd be interested to know your experience, especially with larger codebases.
Eva, I haven't yet tested ChatGPT with large codebases, but I'll certainly give it a try. Let's hope it lives up to the expectations!
Alice, I completely agree! This approach can be a game-changer for code organization. Can't wait to see how it works with Visual Studio C++.
Carol, indeed! If it lives up to the expectations, it'll revolutionize how we manage our C++ projects. Exciting times ahead!
You're welcome, Alice! Experimenting with code snippet sizes is a good idea. Initially, you can start with smaller portions and then gradually increase the size to find the balance that works best for your specific needs. It's a learning process!
Chris, are there any limitations or potential challenges that we should be aware of when using ChatGPT for code organization? It would be great to know and prepare accordingly.
Eva, one challenge can be ensuring that the prompts provided to ChatGPT are precise and cover the specifics of your code organization preferences. Also, fine-tuning the model by training it on your particular codebase can help overcome any limitations. Remember, a reliable backup strategy is essential too.
Chris, that's a helpful tip. I'll be cautious while providing prompts and train the model on my codebase to get more accurate results. Thanks!
Chris, another question: When using ChatGPT, should we strictly adhere to its suggestions, or should we exercise our discretion and evaluate its output before implementing it into our codebase?
Daniel, while ChatGPT can provide helpful suggestions, exercising your discretion and evaluating its output is crucial. It's always a good practice to review and verify the generated code snippets before integrating them into your codebase.
Thanks for the advice, Chris! It's important to maintain control and ensure the quality of the generated code. I'll make sure to carefully review any suggestions provided by ChatGPT.
Daniel, I'd recommend starting with smaller code snippets to gauge ChatGPT's suggestions initially. Once you gain confidence in its accuracy, you can work with larger segments. It's a trial-and-error process!
Alice, that sounds like a sensible approach. Starting small and gradually incorporating larger portions will help in understanding the capabilities and limitations of ChatGPT effectively.
Chris, I agree! Applying critical thinking while evaluating ChatGPT's suggestions is crucial to maintain code quality. It shouldn't replace our expertise but rather serve as a valuable aid.
Chris, starting with smaller snippets and then expanding the scope based on accuracy makes sense. It allows us to mitigate any potential risks while exploring ChatGPT's capabilities.
Chris, I appreciate your emphasis on reviewing and verifying the suggestions from ChatGPT. We can get the best of both worlds by leveraging the model's insights and our own expertise as developers.
Eva, you summarized it perfectly! ChatGPT's insights combined with developers' expertise and critical evaluation can result in effective code organization and heightened productivity.
Thanks, Chris! I'll experiment with code snippet sizes and find the optimal balance. Looking forward to exploring a more efficient code organization approach!
Thank you all for the engaging discussion so far! I'm glad to see the enthusiasm for using ChatGPT to enhance code organization. Keep the conversations going, and let's continue sharing our experiences!