Using ChatGPT for Module Development in Angular.js: Enhancing Development Efficiency and User Experience
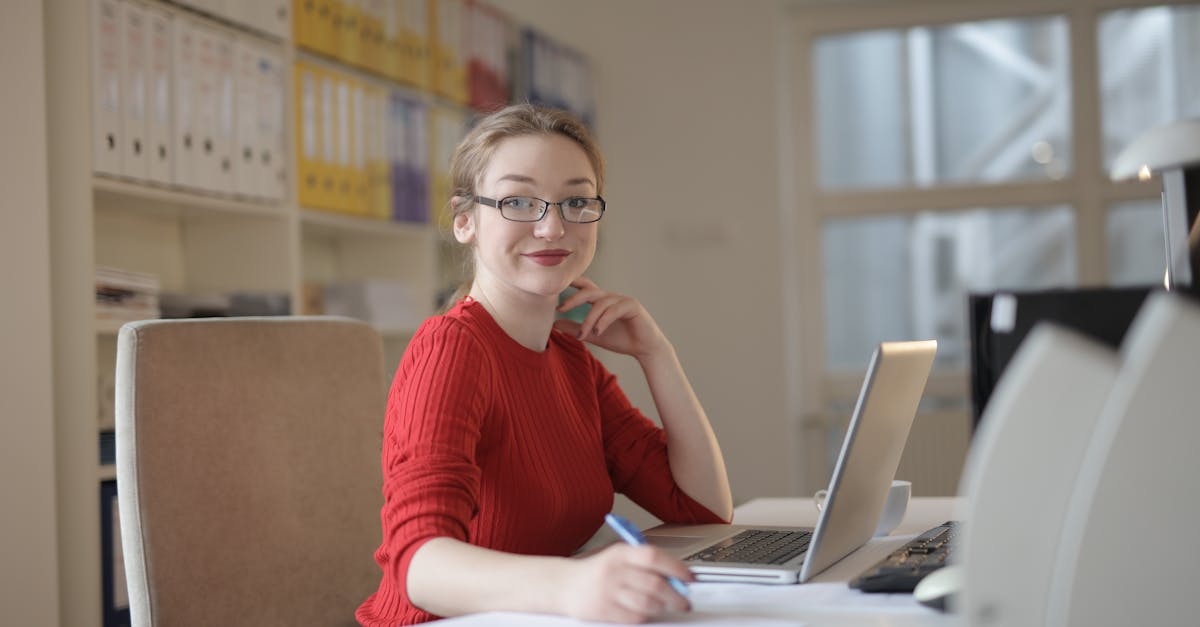
AngularJS is a popular JavaScript framework used for building dynamic web applications. One of its key features is the ability to create reusable modules, which allows developers to organize their code and promote code reusability throughout the project. In this article, we will explore the technology of AngularJS and its usage in the area of module development.
What is AngularJS?
AngularJS is an open-source JavaScript framework developed by Google. It provides a structured approach to building web applications by extending the functionality of HTML. AngularJS follows the Model-View-Controller (MVC) architectural pattern, which separates the presentation logic from the business logic and data.
Module Development in AngularJS
In AngularJS, a module is a container for the different components of an application, such as controllers, services, directives, and filters. It encapsulates related functionality into a single unit, making it easier to manage and reuse across different parts of the application. By creating modules, developers can create self-contained and modular components.
Creating a Module
To create a module in AngularJS, you can use the angular.module
method. The method takes two arguments - the name of the module and an array of dependencies (if any). Here's an example:
var myModule = angular.module('myApp', []);
In this example, a module named 'myApp' is created with no dependencies. You can specify other modules as dependencies by passing their names as strings in the array.
Adding Components to a Module
Once a module is created, you can add components such as controllers, services, directives, and filters to it. This can be done using the module's controller
, service
, directive
, and filter
methods respectively. Here's an example of adding a controller to a module:
myModule.controller('myController', function($scope) {
// Controller logic goes here
});
In this example, a controller named 'myController' is added to the 'myApp' module. The function passed to the controller
method is the controller's constructor function, which contains the logic for the controller.
Importing Modules
AngularJS allows you to import existing modules into your application. This can be done by specifying the imported module as a dependency when creating a new module. Imported modules can provide additional functionality and services that can be used in your application. Here's an example:
var myApp = angular.module('myApp', ['ngRoute']);
In this example, the 'ngRoute' module is imported into the 'myApp' module, which provides routing functionality for the application.
Conclusion
Reusable module development is a powerful feature of AngularJS that helps in building scalable and maintainable web applications. By creating modular components and importing existing modules, developers can organize their code and promote code reusability. This article provided a brief introduction to AngularJS and its usage in the area of module development. We hope this guide helps developers in creating reusable modules and enhances their web application development experience.
Comments:
Thank you all for taking the time to read my article on using ChatGPT for module development in Angular.js! I hope you found it informative and helpful. Please feel free to share your thoughts and ask any questions you may have.
Great article, Rowena! I've been using Angular.js for a while now, and incorporating ChatGPT for module development seems like a game-changer. Can you share any specific use cases where you found it particularly effective?
Thank you, Maria! One use case where I found ChatGPT to be highly effective was in creating chat-based help modules within Angular.js apps. This allowed users to interact with the app itself using natural language queries and receive relevant assistance. It significantly improved the user experience and reduced the learning curve for new users.
Interesting concept, Rowena! However, I'm curious about the potential limitations of using ChatGPT for module development. Have you encountered any challenges or drawbacks while implementing it?
Good question, Alexis! While ChatGPT can enhance development efficiency and user experience, it does come with a few challenges. One limitation is that it heavily relies on the quality and comprehensiveness of the training data. In some cases, it might generate inaccurate or irrelevant responses. Ensuring a robust training dataset and implementing proper validation mechanisms can help mitigate this issue.
Hi Rowena! Thanks for sharing this informative article. I'm new to Angular.js, so I was wondering if you have any recommendations for getting started with using ChatGPT for module development in Angular.js projects?
Hi Samantha! I'm glad you found the article helpful. To get started with using ChatGPT for module development in Angular.js projects, I recommend familiarizing yourself with the Angular.js framework first. Once you have a good understanding, you can explore incorporating ChatGPT by using its API or building a custom integration. Additionally, referring to the OpenAI documentation and examples can provide valuable insights and guidance.
Rowena, excellent article! I've been considering integrating ChatGPT into my Angular.js projects, but I'm concerned about the impact on performance. Have you noticed any significant performance differences while using ChatGPT for module development?
Thank you, Daniel! Performance can be a consideration when using ChatGPT, especially if you have a large number of users or complex modules. One approach to mitigate this is by using caching mechanisms and optimizing API calls. Additionally, properly structuring and organizing your codebase can improve the overall performance of your Angular.js projects.
Fantastic article, Rowena! I'm curious, are there any security considerations to keep in mind when implementing ChatGPT for module development in Angular.js?
Thank you, Michael! Security is indeed an important aspect when using any third-party services like ChatGPT. It's crucial to follow best practices such as securing API keys, implementing proper user authorization and authentication, and protecting against potential vulnerabilities like cross-site scripting (XSS) attacks. OpenAI also provides guidelines and recommendations on securely handling ChatGPT requests and responses.
Hi Rowena, thanks for sharing your insights! I'm curious about the potential impact of using ChatGPT on the development workflow. Did you find any changes in the way you approached module development in Angular.js after incorporating ChatGPT?
Hi Emily! Incorporating ChatGPT does introduce some changes to the development workflow. It's essential to carefully design and build the conversation flows within the modules. Additionally, iterating and testing the chat interactions along with the traditional Angular.js development process can take some extra effort. However, the benefits in terms of enhanced user experience and development efficiency outweigh these additional considerations.
Rowena, great article! I would love to see some examples or code snippets showcasing the integration of ChatGPT into an Angular.js project. Do you have any resources you can recommend?
Thank you, Sarah! Absolutely, there are various resources available to help you with the integration. OpenAI's documentation provides code examples and guidelines for using ChatGPT. Additionally, you can check out community forums, GitHub repositories, and blog posts where developers often share their experiences and code snippets related to Angular.js and ChatGPT integration.
Rowena, I enjoyed your article! However, I'm wondering if using ChatGPT for module development in Angular.js could potentially lead to over-reliance on the chat-based interface and neglecting other aspects of the user interface. What are your thoughts?
Thank you, Nathan! It's a valid concern. While chat-based interfaces can offer a dynamic and user-friendly experience, it's crucial to strike a balance and ensure they complement the other aspects of the user interface. ChatGPT should be considered as an augmentation to the existing UI elements, such as buttons, forms, and visual cues. Properly integrating and maintaining a cohesive UI/UX design is essential.
Rowena, thank you for sharing your expertise! I'm curious about the learning curve involved in using ChatGPT for module development. Did you find it challenging to adapt to this new approach, or was it relatively smooth?
Hi Jennifer! Adapting to using ChatGPT for module development does involve a learning curve. It requires understanding how to design conversational flows, training the model with relevant data, and fine-tuning the responses. However, with practice and experimentation, it becomes smoother. Also, OpenAI's documentation and resources provide valuable guidance for getting started and overcoming any initial hurdles.
Great article, Rowena! I'm curious, have you observed any noticeable improvements in development efficiency and user satisfaction after integrating ChatGPT into Angular.js projects?
Thank you, David! Yes, integrating ChatGPT has resulted in noticeable improvements in both development efficiency and user satisfaction. By providing users with an intuitive chat-based interface for module interactions, the development cycle can be accelerated, and the overall user experience becomes more user-friendly. It simplifies complex processes, reduces the need for extensive documentation, and empowers users to get real-time assistance within the app itself.
Rowena, excellent article! I'm curious, does using ChatGPT for module development in Angular.js require any specific hardware or software dependencies?
Thank you, Jason! Using ChatGPT for module development in Angular.js typically doesn't have any strict hardware or software dependencies. It can be integrated into web applications that run on standard web-based technologies. However, it's important to ensure that your hosting environment has the necessary system requirements, such as the required versions of web servers, frameworks, and libraries, to support the Angular.js and ChatGPT integration.
Great read, Rowena! How does the usage of ChatGPT impact the accessibility of Angular.js modules? Are there any considerations to make these modules accessible to users with disabilities?
Thank you, Lisa! Ensuring the accessibility of Angular.js modules when using ChatGPT is indeed important. It's crucial to follow accessibility best practices, such as providing alternative means of interaction, like keyboard support, for users who may not be able to use the chat-based interface effectively. A proper balance should be struck between the chat interaction and other accessibility features, making sure all users, including those with disabilities, can seamlessly use the modules.
Rowena, superb article! Is it possible to use ChatGPT with Angular.js for multi-language support? If so, are there any considerations to keep in mind while implementing it?
Thank you, Eric! Yes, ChatGPT can be used with Angular.js to achieve multi-language support. When implementing it, it's important to handle the translation of user inputs and responses properly. This can involve leveraging existing translation libraries or services to ensure accurate and contextually appropriate translations. Additionally, providing a seamless language-switching experience within the chat interface can enhance the overall user experience and accessibility across different languages.
Rowena, thanks for sharing your insights! I'm curious, what are your recommended approaches or best practices for testing and validating the chat interactions within Angular.js modules?
Hi Matthew! Testing and validating chat interactions within Angular.js modules is essential to ensure smooth user experiences. One approach is to create test cases covering various scenarios and edge cases, specifically focusing on chat-based interactions. This can involve simulating inputs and verifying the expected responses. Additionally, user feedback and user acceptance testing play a crucial role in refining the chat interactions and making improvements based on real-world usage.
Great article, Rowena! I'm curious, have you encountered any privacy concerns or data security issues when using ChatGPT for module development in Angular.js? If yes, how did you address them?
Thank you, Oliver! Privacy and data security are indeed important considerations when using ChatGPT. To address these concerns, it's crucial to ensure that no sensitive or personally identifiable information is shared within the chat interactions. Proper data anonymization and sanitization techniques should be implemented to avoid any potential data breaches or privacy violations. Also, following best practices like using secure connections (HTTPS) and encrypting sensitive data can further enhance the security of the application.
Rowena, your article is spot on! I'm curious, can ChatGPT be used for real-time chat applications within Angular.js, or is it more suitable for static module interactions?
Thank you, Justin! ChatGPT can be used for real-time chat applications within Angular.js. By integrating ChatGPT's conversational abilities, you can develop interactive chat modules that allow users to have dynamic and real-time conversations with the application. So, whether it's for static module interactions or engaging real-time chats, ChatGPT can be a suitable choice.
Hi Rowena, thanks for writing this informative article! I'm curious, how does ChatGPT handle complex or specific queries that may require a deep understanding of the application and its modules?
Hi Sophia! ChatGPT's ability to handle complex or specific queries essentially relies on the training data it has seen. While it does have the potential to understand various application-specific queries, it's important to train the model with a diverse and representative dataset that captures the necessary domain knowledge. Incorporating feedback loops, user interactions, and continuous training can also improve its ability to handle complex and domain-specific queries effectively.
Rowena, excellent article! I'm curious, how does the performance of ChatGPT in Angular.js compare to other chatbot frameworks or tools available in the market?
Thank you, Emily! Comparing the performance of ChatGPT in Angular.js with other chatbot frameworks or tools can depend on various factors, including the specific requirements of your project and the training data used. ChatGPT, with its language generation capabilities, can provide a more fluent and human-like conversation experience. However, it's always recommended to evaluate and benchmark different frameworks or tools based on your project's needs and constraints to make an informed decision.
Rowena, great insights in your article! I'm curious, how can the generated responses by ChatGPT in Angular.js be moderated or filtered to ensure they meet the desired quality and maintain a positive user experience?
Thank you, Richard! To moderate or filter the generated responses by ChatGPT in Angular.js, you can implement a moderation layer that screens the responses before they are displayed to the users. OpenAI provides a moderation guide that outlines techniques, including content filtering and maintaining a list of disallowed or sensitive topics, to ensure the responses meet the desired quality, align with your application's guidelines, and maintain a positive user experience.
Hi Rowena, great article! I'm curious, can ChatGPT be used for voice-based interactions in Angular.js modules, or is it primarily designed for text-based conversations?
Hi Kimberly! While ChatGPT is primarily designed for text-based conversations, it can be used for voice-based interactions within Angular.js modules by leveraging speech-to-text and text-to-speech conversion techniques. You can integrate speech recognition libraries or services for capturing voice input from users and converting it into text format compatible with ChatGPT. On the response side, you can convert the generated text to voice using text-to-speech libraries or services for voice-based feedback.
Rowena, thanks for sharing your knowledge! I'm curious, how does ChatGPT handle multilingual applications in Angular.js that require language-specific responses based on user preferences or inputs?
Thank you, Christopher! ChatGPT can handle multilingual applications in Angular.js by incorporating language-specific responses based on user preferences or inputs. By training the model with data in different languages and implementing language detection mechanisms, you can generate responses in the appropriate language. Additionally, maintaining language-specific conversational flows and handling translations effectively can enable a seamless multilingual experience within the chat modules.
Rowena, great article! I'm curious, are there any specific browser compatibility or performance considerations to keep in mind while using ChatGPT in Angular.js projects?
Thank you, Daniel! While using ChatGPT in Angular.js projects, it's essential to consider browser compatibility and performance. ChatGPT leverages JavaScript APIs and libraries, so ensuring compatibility across different browsers and versions is crucial. Additionally, optimizing the size and loading time of the model can contribute to improved performance. Regularly monitoring and addressing any browser-specific issues or performance bottlenecks can help provide a seamless experience across different browser environments.
Rowena, thanks for sharing your expertise! I'm curious, can ChatGPT be trained with custom domain-specific data to make it more adept at understanding and responding to module-specific queries?
Thank you, Victoria! Yes, ChatGPT can be trained with custom domain-specific data to improve its understanding and response capabilities regarding module-specific queries. By fine-tuning the model using domain-specific data, you can adapt it to your application's context and provide more accurate and relevant responses. OpenAI provides guidelines and resources on training custom models, enabling you to further enhance ChatGPT's domain-specific effectiveness.
Rowena, excellent article! How well does ChatGPT handle intents or user commands that require triggering specific actions within Angular.js modules, such as submitting a form or executing a function?
Thank you, Julia! ChatGPT can handle intents or user commands that require triggering specific actions within Angular.js modules. By mapping user inputs to corresponding module actions, you can define the necessary conversational flows to enable form submissions, function executions, or any other module-specific actions. Deepening the model's understanding of user intents through appropriate training data and incorporating contextual information when generating responses can help achieve effective command execution.
Hi Rowena, thanks for sharing your knowledge! I'm curious, can ChatGPT be used in combination with other natural language processing (NLP) tools or libraries within Angular.js projects to augment its capabilities?
Hi Erica! Absolutely, ChatGPT can be used in combination with other NLP tools or libraries within Angular.js projects to enhance its capabilities. You can leverage NLP libraries for tasks like intent recognition, sentiment analysis, or named entity recognition, and utilize ChatGPT for generating fluent and context-aware responses. Integrating multiple NLP tools in a modular and synergistic manner can empower your applications with a broad spectrum of conversational capabilities.