Using ChatGPT for Routing and Navigation in Angular: Streamlining User Experiences
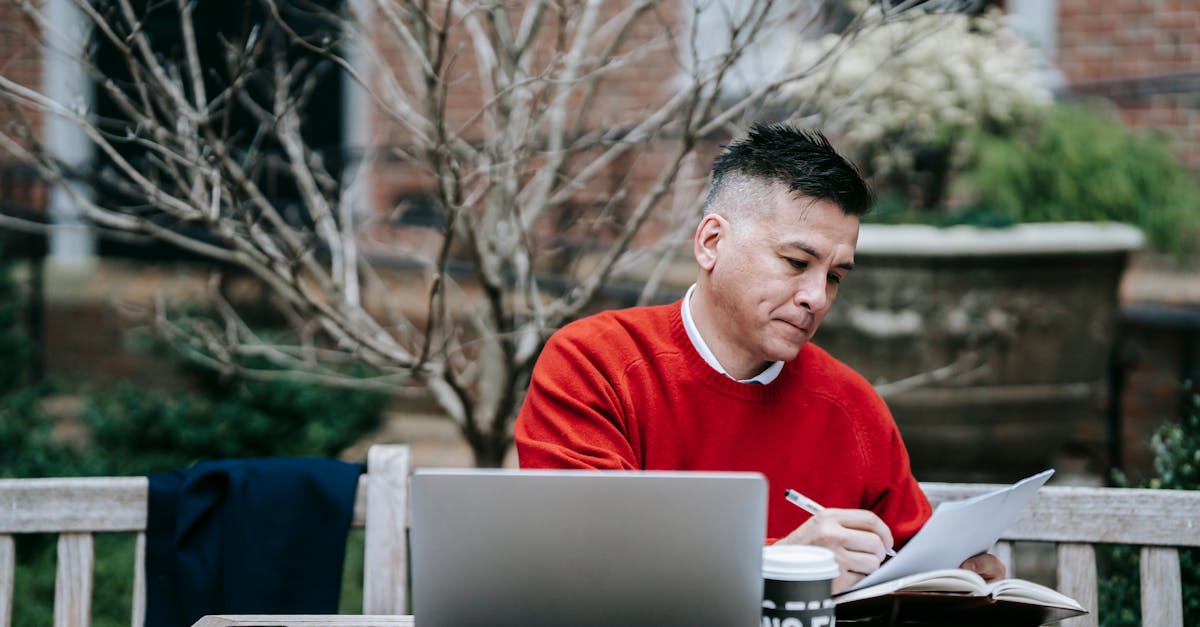
Angular is a widely-used JavaScript framework for building web applications. One of its key features is the ability to set up routing and navigation within an application, allowing users to navigate through different views seamlessly. In this article, we will explore how to implement routing and navigation in Angular applications using the latest version, Angular 12.
Why Routing and Navigation?
Routing and navigation in Angular applications provide a way to divide your application into different views or pages. This allows users to move from one view to another without the need for a full page reload. With routing, you can create a single-page application (SPA) that gives users a more responsive and seamless experience.
Getting Started with Routing in Angular
In Angular, routing is configured using the Angular Router module. To get started, you need to import the necessary modules and define your routes. You can create a file called app-routing.module.ts
to handle all the routing configurations.
In this file, you need to import the required Angular modules:
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
Next, you can define your routes using the Routes
interface:
const routes: Routes = [
{ path: '', redirectTo: '/home', pathMatch: 'full' },
{ path: 'home', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: 'contact', component: ContactComponent }
];
In the above example, we define three routes: /home
, /about
, and /contact
. When a user navigates to /home
, the HomeComponent
will be displayed, and so on.
The redirectTo
property is used to specify the default route if no specific route is provided. In this case, it redirects to the /home
route when the application starts.
To enable routing in your application, you need to import the RouterModule
and use the forRoot()
method to configure the routes:
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Finally, you need to add the <router-outlet>
directive in the app.component.html
file. This directive acts as a placeholder where the components associated with the routes will be rendered:
<router-outlet></router-outlet>
Navigating between Routes
Once you have set up your routes, you can navigate between them using built-in Angular directives or programmatically using the Router
service.
To navigate to a specific route using a hyperlink, you can use the routerLink
directive:
<a >Home</a>
Alternatively, you can also navigate programmatically using the Router
service. To navigate to a specific route, you can call the navigate()
method:
import { Router } from '@angular/router';
@Component({
// Component configuration
})
export class HomeComponent {
constructor(private router: Router) { }
navigateToAbout() {
this.router.navigate(['/about']);
}
}
In the above example, the navigateToAbout()
method navigates to the /about
route when called.
Conclusion
Routing and navigation are essential aspects of modern web applications. With Angular, you can easily set up routing and navigation to provide a seamless user experience. By defining routes, importing necessary modules, and utilizing Angular directives, you can navigate through different views efficiently.
In this article, we explored how to set up routing and navigation in Angular applications. Remember to import the necessary modules, define your routes, and use Angular directives to navigate between views. With these steps, you can create a fully functional Angular application with routing and navigation support.
Comments:
Thank you all for reading my article! I'm excited to hear your thoughts on using ChatGPT for routing and navigation in Angular.
Great article, Diego! I never thought of using ChatGPT for routing and navigation. It could really streamline the user experience. Have you tried implementing it yourself?
Thank you, Anna! Yes, I've been experimenting with ChatGPT for this purpose and it's been quite promising. It can understand user intents and provide contextual suggestions for a smoother navigation flow.
This is interesting! How does ChatGPT handle complex routing scenarios with multiple user inputs?
Hi Michael! ChatGPT can handle complex routing scenarios by maintaining conversation context and understanding user intents across multiple inputs. It can help guide users through a series of steps or retrieve relevant information to provide accurate routing.
I can see how this could simplify user interactions. However, what about personalization? How does ChatGPT adapt to different user preferences?
Great question, Sarah! ChatGPT can be personalized to adapt to different user preferences by leveraging user profiles or preferences stored in a database. It can then tailor the routing and navigation suggestions based on individual needs.
This could be really valuable in reducing user frustrations during navigation. Are there any limitations you've encountered when using ChatGPT for this purpose?
Hi Mark! While ChatGPT is powerful, one limitation is that it heavily relies on the quality of training data and might not handle rare or contextually ambiguous situations as well. It's important to provide clear instructions to avoid confusion.
Diego, have you considered potential security concerns when using ChatGPT for routing and navigation?
Hi Emily! Yes, security is an important consideration. It's crucial to implement robust input validation and sanitization measures to prevent potential exploits or malicious inputs that could affect the routing or compromise user data.
Interesting concept, Diego! Do you have any implementation recommendations for integrating ChatGPT into an Angular project?
Thanks, Samuel! For integration, you can use the OpenAI API to interact with ChatGPT. You can make API calls from an Angular service to handle user inputs and retrieve the routing suggestions. It's important to handle API rate limits and ensure a seamless user experience.
I love the idea of using ChatGPT for routing and navigation. It could make complex processes feel much more intuitive. What are the potential use cases you envision for this?
Hi Hannah! Indeed, it can make complex processes more intuitive. Potential use cases include guiding users through onboarding flows, helping users find specific content or resources, and providing personalized navigation assistance in complex applications.
This sounds fascinating! Could ChatGPT also handle voice-based navigation in Angular applications?
Good question, Jonathan! While ChatGPT itself is designed for text-based conversations, you can integrate it with a speech recognition system to handle voice-based inputs for navigation in Angular applications. The core routing logic would remain the same.
Diego, do you think using ChatGPT for routing and navigation could result in over-reliance on AI, potentially hindering users' navigation skills?
That's a valid concern, Emma. While ChatGPT can streamline navigation, it's important to strike a balance. Providing users with both AI assistance and allowing them to explore and learn the interface organically can help prevent over-reliance and foster user autonomy.
I'm curious, Diego, how would you handle cases where ChatGPT fails to understand the user's intent?
Hi Olivia! When ChatGPT fails to understand the user's intent, a fallback approach could be employed, such as providing a list of common navigation options or suggesting rephrasing the request. This ensures the user can still access the desired routing even if ChatGPT doesn't comprehend the initial input.
Diego, what are your thoughts on using ChatGPT for real-time navigation in dynamic applications?
That's an interesting idea, Lucas! While ChatGPT can handle real-time interactions, it might not be suitable for dynamic applications that require instant updates or data synchronization. For routing, it's best suited for applications with a relatively stable hierarchy or predefined navigation flows.
Diego, I'm amazed by the potential of ChatGPT for routing and navigation! Are there any specific libraries or frameworks you recommend pairing with Angular for this?
Thank you, Sophia! Angular itself provides a solid foundation for implementing routing and navigation. If you'd like to enrich the ChatGPT experience, you can consider integrating additional libraries like ngx-bootstrap or Angular Material to create informative and visually appealing UI components.
Diego, have you encountered any performance issues when using ChatGPT for routing and navigation in Angular?
Hi Jacob! ChatGPT's performance can be affected by factors like network latency and API usage. It's important to optimize the integration by minimizing unnecessary API calls, caching responses where appropriate, and handling error scenarios gracefully to ensure smooth routing and navigation experiences.
This approach seems quite innovative! Diego, did you face any ethical considerations while implementing ChatGPT for routing?
Ethical considerations are crucial, Chloe. It's important to handle user data responsibly, ensure privacy, and prevent biases in the conversation model. Regularly reviewing and refining the training data can help address potential biases and ensure a fair and inclusive user experience.
Diego, what are some fallback strategies you can suggest for cases when ChatGPT encounters an unknown user query?
Hi Daniel! A possible fallback strategy is to provide general navigation instructions, such as a list of commonly accessed pages or a search functionality. Additionally, having a well-designed error handling system in place can guide users when ChatGPT cannot provide a satisfactory response.
What about using ChatGPT for multi-language support in Angular routing and navigation?
Good point, Victoria! While ChatGPT primarily operates with a single language model, you can consider using translation libraries or services like Google Translate to handle multi-language support in input processing and routing responses, enabling internationalization in your applications.
Diego, do you think using ChatGPT for routing and navigation could also benefit visually impaired users?
Absolutely, Max! ChatGPT's text-based interactions can help visually impaired users navigate through applications more efficiently by providing clear instructions and accurate routing suggestions. Pairing ChatGPT with screen readers or other assistive technologies can enhance accessibility for visually impaired users.
Diego, what are the key factors to consider when deciding whether to use ChatGPT or traditional routing logic in Angular applications?
Great question, Sophie! When deciding between ChatGPT and traditional routing logic, consider factors like application complexity, desired user experience, training data availability, and the need for personalized recommendations. For relatively simple and straightforward navigation, traditional routing logic might suffice, while ChatGPT adds value in more complex scenarios or when personalization is crucial.
Diego, have you explored the use of ChatGPT in combination with other AI-powered tools for enhanced routing and navigation experiences?
Hi Owen! Absolutely, combining ChatGPT with other AI-powered tools like natural language processing (NLP) models or recommendation systems can create even more powerful routing and navigation experiences. Leveraging multiple AI technologies allows for sophisticated understanding, context-aware suggestions, and enhanced personalization.
Thank you all for your insightful comments and questions! I appreciate your engagement with the topic. If you have any more thoughts or queries, feel free to share them.