Using ChatGPT for Seamless Integration with ExtJS APIs
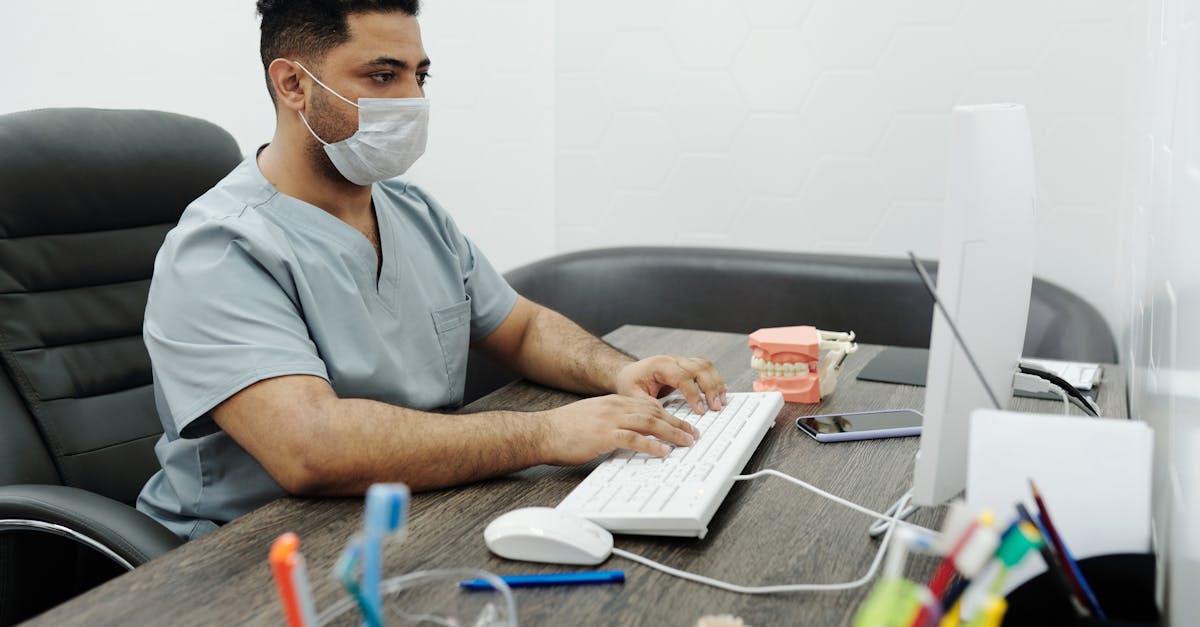
Introduction
ExtJS, a popular JavaScript framework for building web applications, provides developers with a robust set of tools and components. One of the key features of ExtJS is its ability to integrate with external APIs, allowing developers to access and manipulate data from various sources. In this article, we will explore how to make API requests and handle responses using ExtJS.
API Requests
Integrating APIs with ExtJS begins with making requests to external services. ExtJS provides the Ext.data.Connection class, which allows developers to send HTTP requests to API endpoints. To make a request, developers can use the Ext.Ajax.request() method, which provides a simple and intuitive API for sending both GET and POST requests.
Here is an example of making a GET request to an API endpoint:
Ext.Ajax.request({
url: 'https://api.example.com/data',
method: 'GET',
success: function(response) {
// Handle successful response
var data = Ext.decode(response.responseText);
console.log(data);
},
failure: function(response) {
// Handle failed response
console.error(response.statusText);
}
});
In the above example, we specify the API endpoint URL, the HTTP method (GET), and provide success and failure callbacks to handle the response. The success callback is executed when the request is successful, and the failure callback is executed in case of any errors.
API Responses
Once the API request is made, ExtJS provides several methods and properties to handle the response data. The response object passed to the success and failure callbacks contains various properties, including the responseText, responseXML, and status.
The responseText property contains the response data as a string, which can be parsed using Ext.decode() to obtain a JavaScript object. The responseXML property is useful when the API returns XML instead of JSON.
Here is an example of handling a successful API response:
success: function(response) {
var data = Ext.decode(response.responseText);
// Process the response data
console.log(data);
}
In the above example, we parse the response data using Ext.decode() and then process it as needed. The processed data can be used to update the ExtJS application's UI or perform other actions.
Conclusion
Integrating external APIs with ExtJS provides developers with the ability to access and manipulate data from various sources. By using the Ext.data.Connection class and the Ext.Ajax.request() method, developers can easily make API requests and handle the responses in their ExtJS applications. This capability opens up endless possibilities for creating dynamic and data-driven applications.
Comments:
Thank you all for reading my blog article on using ChatGPT with ExtJS APIs! I hope you found it informative.
Great article, Dale! I've been using ExtJS for a while now, and integrating it with ChatGPT seems like a fantastic idea. Can't wait to try it out.
Hi Maria! Thank you for your kind words. I'd love to hear how your experience goes once you give it a try.
I agree, Maria. Dale's article is indeed enlightening. I think this integration will benefit all ExtJS developers.
As a developer, I'm always looking for ways to enhance user experience. This integration sounds promising. Thanks for sharing, Dale!
You're welcome, James! I believe using ChatGPT alongside ExtJS can indeed take user experience to a whole new level.
I appreciate the detailed explanation of how to use ChatGPT with ExtJS APIs, Dale. It makes the integration process much clearer.
Hi Emily! I'm glad the explanation was helpful. If you have any questions or need further assistance, feel free to ask.
I agree, Dale. A clear roadmap helps developers navigate new integrations with confidence. Thank you for providing one in your article.
This is fascinating, Dale. The combination of artificial intelligence with ExtJS opens up exciting possibilities for web development.
Indeed, Michael! AI integration can add a whole new dimension to the capabilities of ExtJS.
I'm somewhat new to ExtJS, but this article has sparked my curiosity. I'm eager to explore how ChatGPT can enhance my projects.
That's great to hear, Sophia! If you have any questions along the way, don't hesitate to ask for guidance.
Absolutely, Dale. Interactive and engaging user experiences are crucial nowadays. Thank you for sharing your insights!
I've worked extensively with both ChatGPT and ExtJS, but this combination never occurred to me. Thanks for the insightful article, Dale!
You're welcome, Robert! Sometimes the most powerful solutions emerge when we combine existing technologies.
I love how ChatGPT can enhance user interactions. Integrating it with ExtJS APIs seems like a smart move to create more engaging web applications.
Hi Linda! I totally agree. With ChatGPT and ExtJS together, developers can create highly interactive and engaging user experiences.
Incorporating AI into web development is becoming more prevalent, and this article shows an interesting way of utilizing it with ExtJS.
Exactly, Daniel! AI integration is becoming increasingly common, and it's exciting to explore its potential within frameworks like ExtJS.
This is a great read, Dale! The examples you provide really illustrate how ChatGPT can complement ExtJS APIs.
Thank you, Sophie! I find that practical examples often help readers grasp the concepts more effectively.
Thank you, Dale! I'll make sure to reach out if I have any questions during my integration process.
Dale, your article has inspired me to experiment with ChatGPT and ExtJS. I can't wait to see what innovative solutions I can come up with.
That's fantastic, David! I'm thrilled to hear that the article has sparked your creativity. Good luck with your experimentation!
I've been hesitant to incorporate AI into my projects, but this article presents a compelling case for using ChatGPT with ExtJS.
Hi Karen! It's natural to be hesitant about new technologies. If you decide to give it a try, I'm here to support you along the way.
Thank you, Dale! I appreciate your offer of support as I consider incorporating ChatGPT into my projects.
You're welcome, Karen! If you decide to incorporate ChatGPT, I'm here to provide the assistance you need.
As someone new to ExtJS, I appreciate how you explain both ChatGPT and ExtJS APIs in a way that's easy to understand, Dale.
Thank you, Sarah! I aim to make complex concepts approachable for all readers, regardless of their level of experience.
This is a brilliant concept, Dale. The combination of ChatGPT and ExtJS can definitely revolutionize the web development landscape.
I appreciate your enthusiasm, Oliver! Indeed, the possibilities that arise from this combination are truly exciting.
I've dabbled with ChatGPT before, but I'm eager to explore its integration with ExtJS. The article has given me a solid starting point.
That's great, Melissa! It's always exciting to delve into new possibilities. Don't hesitate to reach out if you need any guidance.
I've never heard of ExtJS before, but your article has piqued my interest. Looking forward to exploring it further with ChatGPT.
Hi Ryan! ExtJS is a powerful framework, and combining it with ChatGPT can lead to incredible innovations. Feel free to ask questions as you explore.
The possibilities seem endless when integrating AI into web development. Thanks for sharing this fascinating approach, Dale!
You're welcome, Emma! AI integration does open up a world of possibilities, and it's exciting to see those possibilities materialize.
Great article, Dale. I'll definitely be giving ChatGPT and ExtJS integration a try in my next project. Thanks for the inspiration!
That's wonderful to hear, Julian! I'm glad you found inspiration in the article. Best of luck with your upcoming project.
ChatGPT has shown immense potential in various applications. Integrating it with ExtJS APIs sounds like a winning combination.
Hi Grace! I couldn't agree more. The combination of ChatGPT and ExtJS presents an incredible opportunity for developers.
Great point, Grace. The combination of ChatGPT and ExtJS can revolutionize web development, making it more dynamic and user-oriented.
I'm glad you find the article enlightening too, Robert! It's exciting to see how this integration can benefit all ExtJS developers.
Indeed, Maria! Dale's article has convinced me to give this integration a try, and I'm excited to see the benefits firsthand.
That's fantastic, Robert! I hope you have a great experience integrating ChatGPT with ExtJS in your projects.
Thank you, Maria! I'm excited to dive into it and explore the potential for innovative solutions.
Your article is well-written and highly informative, Dale. I appreciate how you demonstrate the benefits of integrating ChatGPT with ExtJS.
Thank you for your kind words, Marcus! It's important to highlight the benefits that this integration brings to the development process.
I've been using ExtJS for a while now, but I hadn't considered integrating it with ChatGPT until I read your article, Dale. Thanks for the suggestion!
You're welcome, Olivia! It's never too late to explore new possibilities, and I'm glad the article prompted you to consider this integration.
Thank you for sharing this informative article, Dale. The use of AI in web development is becoming increasingly important, and your insights are valuable.
Hi Jack! Indeed, AI is transforming web development, and it's essential to stay updated with the latest trends. I'm glad you found the insights valuable.
Integrating AI into ExtJS APIs is an exciting prospect. Your article presents a clear roadmap for developers, Dale. Well done!
Thank you, Eva! Providing a clear roadmap is essential to help developers embrace this integration with confidence.
ChatGPT's ability to understand user context can greatly enhance the user experience when integrated with ExtJS APIs. Fantastic article, Dale!
Hi Nathan! You're absolutely right. Contextual understanding takes user experience to a whole new level. I appreciate your kind words.
You're welcome, Dale! The potential of ChatGPT's contextual understanding can't be underestimated. It really enhances user interactions.
Absolutely, Nathan! Contextual understanding adds a human-like touch to the user experience, enhancing their interactions.
I'm eager to explore this integration, Dale. The article highlights the potential benefits of using ChatGPT with ExtJS APIs in a concise manner.
That's wonderful, Victoria! Concise explanations can be highly effective in conveying the value of new integrations. Enjoy your exploration!
This integration can definitely elevate web applications to new heights. Thanks for sharing your knowledge in this well-written article, Dale.
It's amazing how AI can replicate human understanding to such a degree. The possibilities are truly fascinating.
Absolutely, Nathan! The advancements in AI are reshaping the digital landscape and opening up limitless possibilities.
Indeed, Dale! It's an exciting time to be a developer, with AI playing a transformative role in various domains.
Absolutely, Nathan! Exciting times lie ahead, and it's up to us to embrace the opportunities and push the boundaries.
Thank you all once again for your valuable comments and engagement in this discussion. If you have any further questions or thoughts, feel free to share!
Thank you, everyone!