Boosting Performance Optimization in C++ Language: Unleashing the Power of ChatGPT
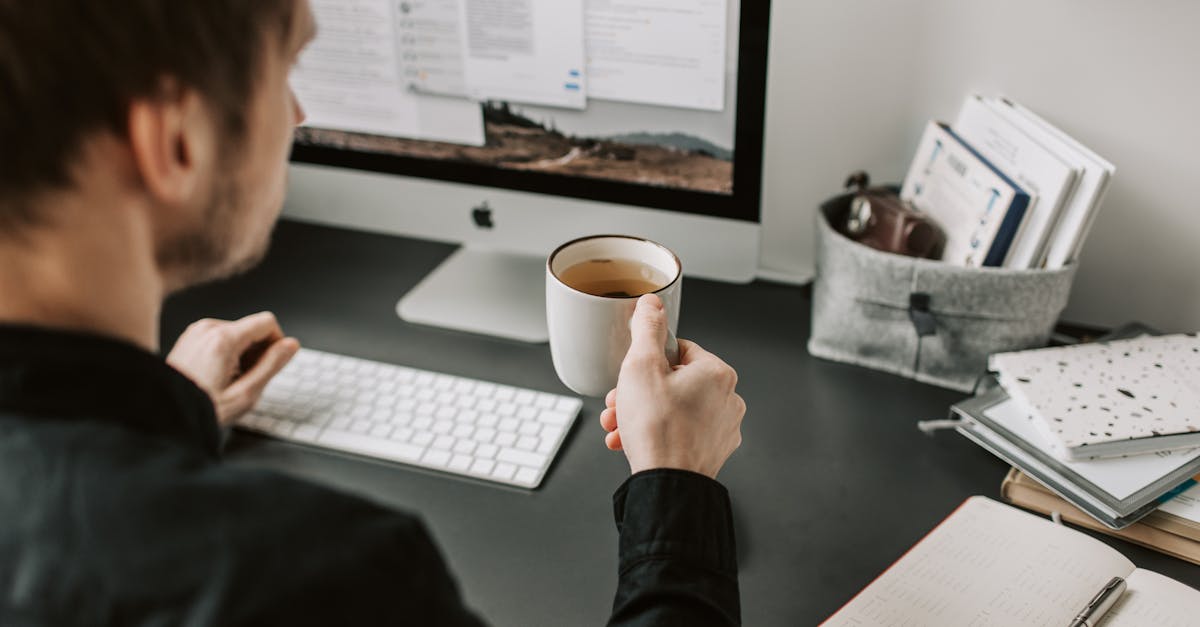
Introduction
In the world of programming, performance optimization is crucial for achieving fast and efficient code execution. The C++ programming language, known for its power and speed, provides various techniques and best practices to optimize the performance of your code.
Understanding Performance Optimization
Performance optimization involves making changes to a program to improve its execution speed, memory usage, and overall efficiency. It involves analyzing and identifying performance bottlenecks, finding ways to reduce CPU cycles, minimizing memory allocations, and optimizing algorithms and data structures. These optimizations can lead to significant improvements in both small-scale and large-scale C++ projects.
Techniques for Performance Optimization in C++
1. Algorithmic Optimization: Optimizing the algorithms used in your code can lead to substantial improvements in performance. This includes choosing the most efficient algorithm for a given task, reducing unnecessary computations, and leveraging existing libraries and data structures.
2. Memory Optimization: Efficient memory usage is crucial for performance optimization. Techniques like object pooling, memory reclamation, and minimizing memory allocations can help reduce memory fragmentation and improve cache utilization, resulting in faster execution times.
3. Compiler Optimization: Modern C++ compilers offer numerous optimization features like inline expansion, loop unrolling, and constant propagation. Understanding these optimization settings and enabling them appropriately can often lead to significant performance improvements without manual coding changes.
4. Multithreading and Parallelism: Leveraging multiple threads and parallelism can help improve performance by distributing tasks across multiple CPU cores. Techniques like thread pooling, parallel algorithms, and lock-free programming can enhance performance in computationally intensive applications.
5. Profiling and Benchmarking: Profiling tools provide insights into the performance of your code by identifying hotspots, bottlenecks, and areas where performance can be improved. Benchmarking helps measure the impact of optimizations, ensuring that changes indeed result in better performance.
Best Practices for C++ Performance Optimization
1. Avoid premature optimization: It is essential to profile and benchmark your code before starting the optimization process. Identify the critical sections that consume significant execution time and focus on optimizing them.
2. Use the right data structures and algorithms: Selecting the appropriate data structures and algorithms based on the problem domain can significantly impact performance. Understand the strengths and weaknesses of different data structures and algorithms before making choices.
3. Minimize memory allocations and deallocations: Dynamic memory allocation and deallocation can be expensive operations. Avoid unnecessary memory allocations and reuse memory whenever possible.
4. Optimize loops and conditionals: Reducing the number of loop iterations, eliminating unnecessary branches, and optimizing conditionals can improve performance. Unrolling loops, loop fusion, and loop blocking are techniques that can be applied to enhance performance.
5. Use const and constexpr: Marking variables and functions as const or constexpr can enable compiler optimizations like constant folding, which can eliminate unnecessary computations.
Conclusion
Performance optimization is a critical aspect of software development, especially when working with computationally intensive tasks or large-scale applications. By applying the right techniques and following best practices, you can significantly improve the performance of your C++ code.
Remember to profile and benchmark your code, choose efficient algorithms and data structures, minimize memory allocations, leverage compiler optimizations, and make use of multithreading and parallelism when appropriate. With these strategies, you can unlock the full potential of C++ and deliver high-performance applications.
Comments:
Thank you all for your interest in my article! I'm excited to discuss the topic of boosting performance optimization in C++. Let's start the conversation.
Great article, Amanda! The tips you shared for optimizing C++ performance are really helpful. Especially the part about reducing unnecessary copying. Do you have any additional insights on that?
Agreed, Daniel! I found that section particularly interesting as well. Amanda, could you please elaborate on how to minimize unnecessary copying in C++?
Thanks, Daniel and Karen! Minimizing unnecessary copying in C++ can greatly improve performance. One way to achieve this is by using references instead of making unnecessary copies of objects. Additionally, move semantics and using smart pointers can help reduce copying overhead. It's crucial to understand object lifetimes and choose the appropriate techniques to avoid unnecessary copies.
I found the section on multithreading optimizations quite enlightening. Amanda, could you provide some examples of when and how to use multithreading to improve performance in C++?
Sure, Jonathan! Multithreading can be a powerful tool for performance optimization in C++. For example, if you have independent tasks that can be executed concurrently, you can leverage multiple threads to complete them faster. Be careful with synchronization and data access, though, as it can lead to race conditions and other issues. Properly dividing the workload and protecting shared data is crucial.
Thank you, Amanda! That makes sense. I'll keep those considerations in mind when working with multithreading in C++.
I really enjoyed the section on profiling and benchmarking. Amanda, do you have any recommendations for profiling tools to use in C++ development?
Certainly, Sara! Some popular profiling tools for C++ development are Valgrind, Gprof, and Intel VTune. These tools provide insights into your program's performance characteristics, memory usage, and bottlenecks. It's crucial to profile your code to identify areas of improvement and optimize accordingly.
Thank you, Amanda! I'll definitely explore those profiling tools to improve the performance of my C++ programs.
Excellent article, Amanda! You provided some valuable insights. I'm curious to know if you have any tips on optimizing memory usage in C++.
Certainly, Emily! Optimizing memory usage is essential for efficient C++ programs. Some tips include using data structures that match the problem requirements, avoiding unnecessary data duplication, and freeing unused memory when appropriate. Smart pointers, containers with reserved capacities, and custom memory allocators can also aid in memory optimization.
Amanda, thanks for the explanation regarding multithreading optimizations. It's crucial to handle shared data properly to prevent unexpected behaviors.
Thank you, Amanda! These tips will definitely help me optimize the memory usage of my C++ programs.
Amanda, thanks for sharing your expertise! I'd love to hear your thoughts on code optimization techniques specific to embedded systems development in C++.
You're welcome, Michael! Optimizing code for embedded systems requires additional considerations due to their resource-constrained nature. Some techniques include reducing memory allocations, utilizing fixed-size containers, minimizing dynamic memory usage, and avoiding virtual function calls whenever possible. It's important to understand the hardware architecture and constraints of the embedded system you're targeting.
Great article, Amanda! I have a question about leveraging inline functions for performance optimization in C++. When should we consider using inline functions, and are there any caveats?
Thanks, Carlos! Inline functions can provide performance benefits in C++ by reducing the function call overhead. However, the decision to use them should be made judiciously. Inline functions work best for small, frequently-used functions, and their actual inlining is ultimately up to the compiler. It's important to profile and measure the impact when considering inline functions, as inappropriate use can lead to code bloat and decreased performance.
Amanda, your article was a great read! I'm wondering if you have any suggestions for reducing the compilation time in C++ projects.
Thank you, Jennifer! Compilation time can become a bottleneck, especially in large C++ projects. Some techniques to reduce compilation time include precompiled headers, forward declarations instead of including unnecessary headers, and modularizing project structure. Additionally, using distributed or parallel build systems can speed up compilation on multi-core machines. It's important to strike a balance between compilation time and maintainability when applying these techniques.
Amanda, I found your article extremely helpful! I'm curious to know if there are any specific C++ language features or constructs to avoid for performance reasons.
I'm glad you found it helpful, Robert! When it comes to C++ performance, some language features or constructs can have performance implications. For example, excessive use of virtual functions, unnecessary deep inheritance hierarchies, excessive dynamic memory allocations, and excessive string concatenations can negatively impact performance. It's important to be mindful of these constructs and use them judiciously, considering their performance trade-offs.
Amanda, your article provided great insights! Could you shed some light on techniques for efficient exception handling in C++?
Thank you, Eric! Efficient exception handling is crucial for maintaining performance in C++. Some techniques include using exceptions only for exceptional situations, avoiding unnecessary try-catch blocks, catching exceptions by const reference, and using RAII (Resource Acquisition Is Initialization) to manage resources. Limiting the scope of exception handling and avoiding exceptions in performance-critical parts of the code can also improve performance.
Thank you, Amanda! These techniques will certainly help me handle exceptions more efficiently in my C++ projects.
Great article, Amanda! I'm curious to know if you have any tips for optimizing C++ code for specific hardware architectures, like SIMD instructions or GPU acceleration.
Thanks, Adam! Optimizing C++ code for specific hardware architectures can provide significant performance gains. Techniques like leveraging SIMD instructions for parallel processing and utilizing GPU acceleration through libraries like CUDA can greatly enhance performance. However, such optimizations require understanding the target hardware, its capabilities, and appropriate libraries or frameworks. It's important to profile and measure the impact of these optimizations to ensure they deliver the expected improvements.
I appreciate your article, Amanda! Are there any common pitfalls or mistakes that developers should watch out for in C++ performance optimization?
Thank you, Sarah! There are a few common pitfalls to avoid when optimizing C++ performance. Some include premature optimization without profiling, optimizing non-bottlenecks, not considering the Big-O complexity of algorithms, excessive use of inefficient data structures, and not keeping up with modern C++ language features. It's important to profile code, identify bottlenecks, and prioritize optimizations based on empirical data.
Amanda, your article was a great resource! Do you have any suggestions for optimizing I/O operations in C++?
I'm glad you found it helpful, David! Optimizing I/O operations in C++ can improve performance. Some tips include minimizing the number of I/O operations, using buffered I/O, reducing disk seeks, avoiding unnecessary conversions, and utilizing asynchronous I/O when applicable. It's important to profile and measure the impact of these optimizations to ensure they align with your specific I/O requirements and improve performance accordingly.
Amanda, thanks for sharing your expertise! Your tips on optimizing C++ performance will certainly come in handy for my projects. Looking forward to your future articles!
You're welcome, James! I'm glad you found the tips helpful. I'll definitely continue sharing insights through future articles. Feel free to reach out if you have any more questions. Happy coding!
Amanda, your article was informative and well-written. I enjoyed every bit of it. Thank you for sharing your knowledge!
Thank you, Linda! I'm glad you enjoyed the article and found it informative. Sharing knowledge and helping fellow developers is always a pleasure. Feel free to revisit the article anytime you need a refresher on C++ performance optimization techniques.
Amanda, your insights into C++ performance optimization are outstanding. Thank you for sharing your expertise in such a clear manner!
You're welcome, Daniel! I appreciate your kind words. Making complex topics accessible and clear is one of my goals when sharing knowledge. I'm glad you found the insights valuable. Let me know if you have any further questions or if there's anything specific you'd like to learn more about.
Amanda, your article has provided me with a solid foundation for optimizing performance in C++. Thank you for the comprehensive insights!
You're welcome, Karen! I'm thrilled that the article helped you establish a strong foundation for C++ performance optimization. Remember, practice and hands-on experience will further enhance your skills in this area. Don't hesitate to ask if you need any further guidance or clarification on any of the discussed topics.
Amanda, as always, your articles are incredibly insightful! I appreciate the level of detail you provided on C++ performance optimization. Looking forward to your next piece!
Thank you, Jason! I'm delighted that you found the level of detail helpful. I strive to provide valuable insights to fellow developers. Stay tuned for my next article, and feel free to reach out if you have any specific topics you'd like me to cover.
Amanda, your article was a great refresher on C++ performance optimization techniques. Your explanations were clear and concise. Thank you!
You're welcome, Melissa! I'm glad you found the article to be a valuable refresher. Clear and concise explanations are essential when delving into performance optimization topics. If you have any specific questions or need further clarification, please don't hesitate to ask. Happy coding!
Amanda, your expertise shines through in this article. Thank you for sharing your knowledge and guiding us through C++ performance optimization!
Thank you, Emily! Sharing knowledge and guiding fellow developers through programming topics is a passion of mine. I'm thrilled that my expertise shines through in the article. Feel free to ask any questions or request further guidance whenever you need it. Happy optimizing!
Amanda, your article provided excellent insights into optimizing C++ performance. I look forward to implementing these techniques in my projects.
Thank you, James! I'm glad you found the insights excellent. Implementing these techniques will certainly enhance the performance of your C++ projects. If you encounter any challenges or have questions while applying the optimizations, don't hesitate to reach out. I'm here to help. Good luck with your projects!
Amanda, your article was a valuable resource on C++ performance optimization. The tips you shared are actionable and well-explained. Thank you!
You're welcome, Sophia! Providing actionable and well-explained tips is essential to assist fellow developers in optimizing C++ performance. If you ever need further clarification or have specific questions, feel free to ask. Thank you for your kind words and happy optimizing!
Amanda, your article was a fantastic dive into C++ performance optimization. Your expertise shines brightly, and I can't wait to implement these techniques!
Thank you, Oliver! It's always rewarding to see fellow developers excited to implement performance optimization techniques in their projects. If you need any guidance or have questions while applying these techniques, feel free to ask. I'm here to support your optimization journey. Best of luck!