Enhancing Binary Tree Construction: Leveraging ChatGPT for Advancements in Data Structures
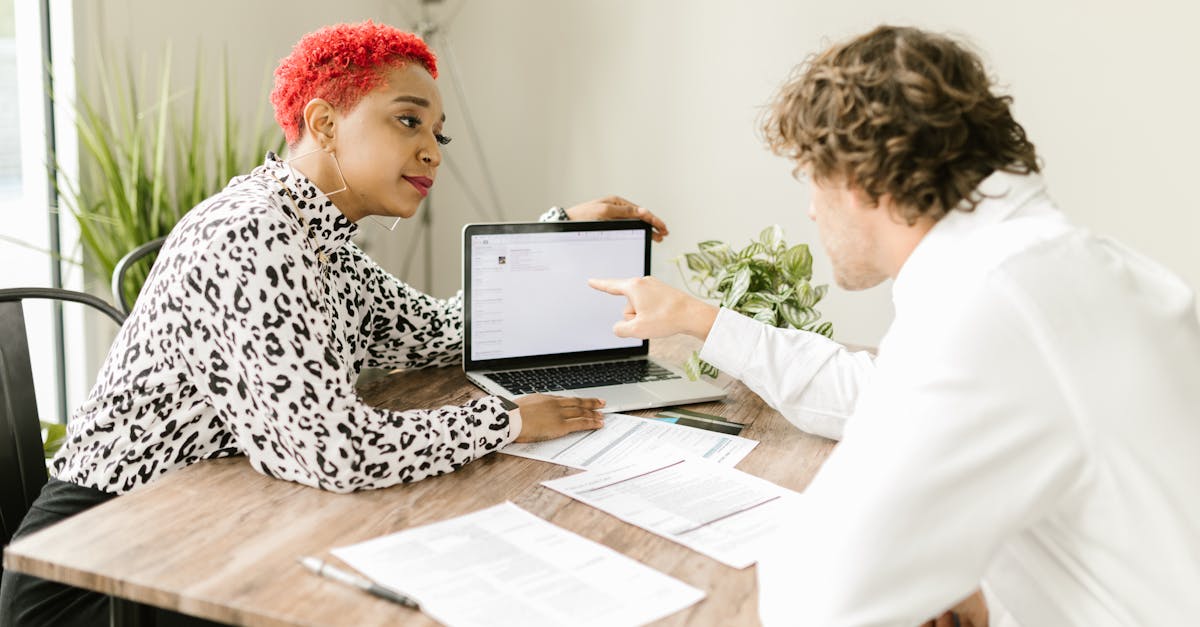
In the realm of computer science and data structures, a binary tree is a crucial concept utilized for organizing and representing hierarchical relationships between elements. Binary trees are extensively used for a wide range of applications, including the construction of intelligent conversational agents like ChatGPT-4, which can exhibit knowledge of constructing various types of binary trees and explain related concepts.
Understanding Binary Trees
A binary tree is a tree data structure with nodes having at most two children: left child and right child. Each node can contain a piece of data, also known as a "payload," and two pointers to its left and right children.
To construct a binary tree, one can start by defining a Node class. Here's a simple implementation in Python:
class Node:
def __init__(self, data):
self.data = data
self.left = None
self.right = None
Binary Tree Construction
Binary trees can be constructed in several ways, depending on the desired arrangement of nodes. Some common methods include:
- Recursive Construction: In this approach, a binary tree is built recursively by assigning the left and right children to each node.
- Level Order Construction: This method constructs a binary tree level by level, starting from the root node.
- Preorder Construction: Preorder traversal of a binary tree can be used to construct the tree based on the sequence of nodes visited.
Once a binary tree is constructed, various operations like inserting new nodes, deleting existing nodes, searching for a specific node, and traversing the tree can be performed.
Using ChatGPT-4 for Binary Tree Construction
ChatGPT-4, an advanced conversational agent, possesses the capability to demonstrate the construction of different types of binary trees and explain the underlying concepts. By interacting with ChatGPT-4, users can inquire about binary tree construction methods, understand the steps involved, and gain a comprehensive understanding of this foundational data structure.
With its vast knowledge base and conversational abilities, ChatGPT-4 can guide programmers, computer science enthusiasts, and learners in constructing binary trees effectively and efficiently.
Comments:
Thank you all for your interest in my article on enhancing binary tree construction! I appreciate your comments and look forward to an insightful discussion.
Great article, Andrew! I found your explanation of leveraging ChatGPT for advancements in data structures fascinating. It truly showcases the potential of combining AI with traditional computer science concepts.
@Victoria, I agree! The integration of AI into data structures opens up new possibilities for improved efficiencies. It's amazing how AI continues to transform various areas of computing.
@Lisa, indeed! AI's transformative capabilities extend far beyond its initial applications. The collaboration of AI and traditional computing concepts holds tremendous promise.
@Victoria, I couldn't agree more! The integration of AI technologies into data structures not only enhances efficiency but also enables the development of smarter algorithms.
@Victoria, AI has come a long way, and its integration into data structures showcases the remarkable potential of these technologies. It's exciting to witness the transformation taking place.
@Victoria, the possibilities unleashed by combining AI and traditional computer science concepts are staggering. It's exciting to envision the impact on various industries.
@Michelle, precisely! It's mind-boggling to think about the impact this integration could have on diverse sectors like healthcare, finance, transportation, and more.
@Victoria, absolutely! The collaboration between AI and traditional computing concepts has immense potential beyond what we can currently imagine. It's exciting to witness the impact.
@Lisa, indeed! The integration of AI and traditional computer science concepts has the power to revolutionize the data structures field and enable more efficient solutions.
@Victoria, I couldn't agree more! AI has the potential to revolutionize not just data structures but also other aspects of computer science. Exciting times ahead!
@Thomas, couldn't agree more. The advancements brought by AI in various domains hold immense potential for future developments. It's an exciting time to be in the field of computer science!
@Thomas, no doubt! The continuous advancements in AI and machine learning are reshaping the world as we know it. It's intriguing to witness the impact of these technologies.
@Victoria, the combination of AI and traditional computer science concepts is indeed powerful. It brings innovative solutions and the potential to revolutionize how we approach data structures.
Andrew, I have to say, your article brought a fresh perspective. The idea of utilizing AI-powered language models for improving data structures is innovative. I'm curious about the performance implications, though.
@Michael, I'm glad you found it interesting! Performance implications are indeed crucial. While AI can assist in constructing more optimal trees, we must consider the computational overhead introduced by AI techniques.
Andrew, I appreciate the effort you've put into this research! It's an exciting intersection of AI and data structures. I wonder, though, if there are any limitations in leveraging AI models for constructing binary trees?
@Michelle, thank you for your kind words! Indeed, there are challenges. Training AI models for optimal tree construction requires extensive training data and careful fine-tuning to balance performance improvements and computational overhead.
@Andrew, I see. Achieving the right balance between performance enhancements and computational complexity is a challenge indeed. The quality and quantity of training data are paramount. Thank you for the clarification!
@Michelle, I share your curiosity about limitations. It's important to explore potential constraints and challenges associated with AI-empowered binary tree construction. In certain scenarios, traditional methods may still hold advantages.
@Emily, I completely agree with your statement. Andrew's article encourages innovation and reminds us to explore unconventional strategies. It's through these efforts that we can pave the way for future advancements.
@Daniel, I share your excitement about the potential practical implications of AI-integrated data structures. It's a promising path for enhancing the performance and efficiency of various algorithms.
@Emily, I'm glad you found the article excellent! Andrew's research indeed emphasizes the importance of exploring new approaches to drive innovation in the field of data structures.
@Michelle, you're absolutely right. Traditional methods, particularly for smaller datasets or specific applications, may continue to provide advantages. AI techniques should be seen as a tool in the toolbox, not a replacement.
@Michelle, you're absolutely right. Traditional methods, particularly for smaller datasets or specific applications, may continue to provide advantages. AI techniques should be seen as a tool in the toolbox, not a repl
Great article, Andrew! AI-driven advancements in data structures have the potential to reshape how we optimize algorithms and handle large-scale data processing. Looking forward to seeing more research in this area.
@Andrew, you're welcome! It's important to consider the context and make informed decisions regarding AI integration. Proper evaluation and assessment are key to understanding when and where AI techniques provide the most value.
@Andrew, your article sheds light on the potential of AI in data structures. However, do you think there could be concerns about the black-box nature of AI models and their interpretability in critical applications?
@Andrew, absolutely! AI techniques should complement traditional methods, and understanding the right balance between the two is vital. It requires careful considerations in each use case.
@Andrew, absolutely! AI techniques should complement traditional methods, and understanding the right balance between the two is vital. It requires careful considerations in each use case.
@Andrew, you've covered an interesting topic! The combination of ChatGPT with data structures opens up new avenues for exploration. I'm curious if you foresee any specific applications of this integration.
@Jonathan, thank you! The potential applications of this integration are vast. One specific area could be optimizing decision-making algorithms in complex systems, such as finance or healthcare.
@Jonathan, interpretability is a valid concern. Ensuring transparency and interpretability of AI models, especially in critical applications, remains an active area of research to address potential risks.
@Andrew, optimizing decision-making algorithms in finance and healthcare would be incredibly beneficial. The combination of AI and data structures could revolutionize these domains.
@Michelle, AI models' reliance on large amounts of quality data can be seen as a limitation. In scenarios where data is scarce or high-quality labeling is difficult, traditional methods may outperform AI-empowered techniques.
@Andrew, I appreciate your response! Balancing performance improvements and computational overhead is indeed crucial. It's an exciting direction for future research.
@Michael, I share your curiosity about performance implications. While AI may help optimize tree construction, we need to consider trade-offs in terms of computational complexity and potential challenges in training AI models effectively.
@Robert, I concur. While AI brings valuable improvements, we should also consider factors like data availability, model interpretability, and potential biases. These aspects need careful consideration.
@Robert, you raised a valid point. Training AI models effectively requires meticulous attention to prevent biases and ensure transparency. It's crucial for AI-integrated data structures to be reliable and unbiased.
@Robert, I completely agree. Ensuring fairness and avoiding biases in AI-integrated systems is critical. We should prioritize ethics and transparency in such developments.
@Robert, bias and interpretability concerns should be addressed upfront when adopting AI-integrated data structures. It's important to ensure fairness and avoid reinforcing or amplifying existing biases.
Excellent article, Andrew! Your work showcases the importance of exploring unconventional methods and thinking outside the box when it comes to improving existing concepts like binary tree construction.
@Emily, I fully agree. Andrew's article emphasizes the importance of exploring unconventional approaches to enhance existing concepts. It stimulates innovation and pushes boundaries.
@David, I believe embracing unconventional approaches will lead to significant progress in the field. By thinking outside the box, we can unlock new opportunities and push the boundaries of what is possible.
@Jessica, absolutely! Embracing unconventional approaches often leads to the most significant breakthroughs. It's essential to encourage creative thinking and innovation.
@David, absolutely! By thinking beyond the established norms, we can challenge existing limitations and drive meaningful progress.
@David, embracing unconventional methods is crucial to foster innovation. It's inspiring to see how AI can contribute to enhancing traditional concepts and drive us forward.
Hi Andrew! This topic always fascinated me, and your article provides valuable insights into the future of data structures with AI integration. It'll be interesting to see the practical implications in real-world applications.
@Samuel, absolutely! This integration of AI and data structures has immense potential. We might witness groundbreaking advances in fields like artificial intelligence, robotics, and more.
Thank you all for your valuable contributions to the discussion! I appreciate your engagement and insights. Let's continue this dialogue to further explore the potential of AI in advancing data structures.