Improving Speech Recognition in Django with ChatGPT
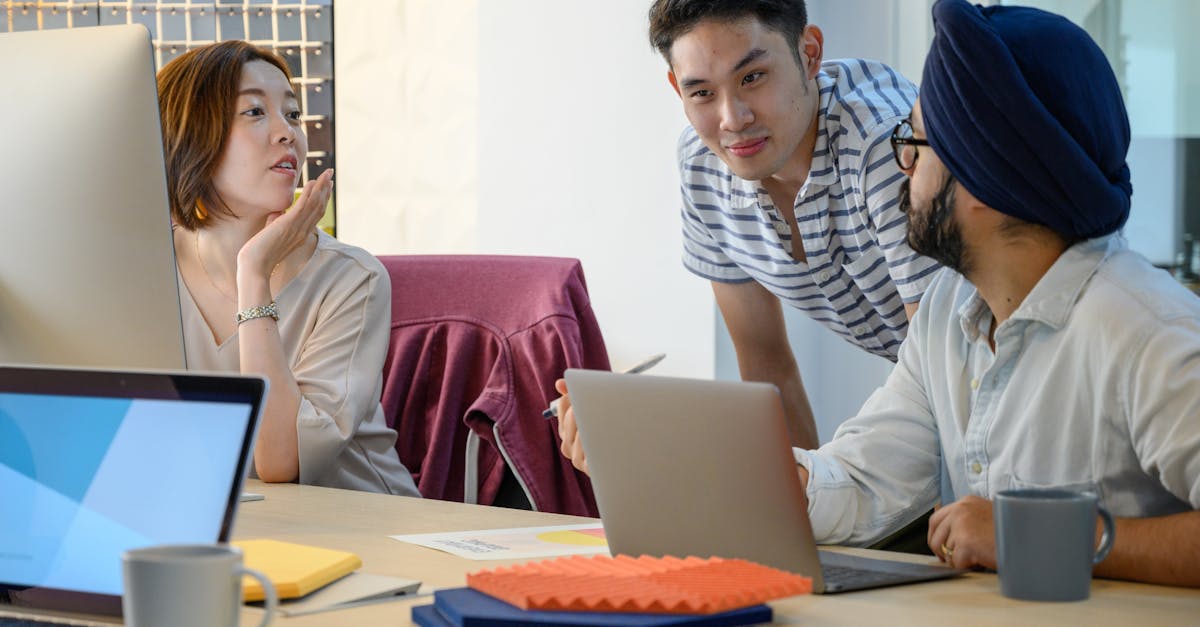
Django is a powerful web framework that provides developers with the tools they need to build robust and dynamic web applications. With the advent of speech recognition technology, it is now possible to further enhance web applications by enabling voice-based input and interactions. This article explores how you can integrate speech recognition capabilities into your Django applications using ChatGPT-4.
Speech Recognition Technology
Speech recognition technology, also known as automatic speech recognition (ASR), is a technology that converts spoken language into written text. It has made significant advancements in recent years, thanks to machine learning and natural language processing techniques. Speech recognition enables users to interact with devices or applications using their voice, providing a convenient and hands-free mode of communication.
Speech Recognition in Django
Integrating speech recognition into Django applications can enhance user experiences and provide an alternative input method for users. One way to achieve this is by leveraging the power of ChatGPT-4, a state-of-the-art language model developed by OpenAI.
To enable speech recognition in Django, follow these steps:
Step 1: Set Up Django Project
If you haven't already, create a new Django project or navigate to your existing project directory.
django-admin startproject myproject
Change to the project directory:
cd myproject
Step 2: Install Required Dependencies
Install the necessary dependencies for speech recognition using ChatGPT-4. This includes the OpenAI Python library and any other packages required for audio processing.
pip install openai # Install the OpenAI Python library
pip install <other dependencies>
Step 3: Create a Django App
Create a new Django app within your project. This will be the module where you implement the speech recognition functionality.
python manage.py startapp speech_recognition
Step 4: Implement Speech Recognition
In the Django app's views.py file, create a new view function that handles the speech recognition functionality. You can use the ChatGPT-4 library to perform the actual speech-to-text conversion.
from django.http import JsonResponse
import openai
def speech_recognition(request):
# Handle audio input from the request
audio_data = request.FILES.get('audio')
# Perform speech recognition using ChatGPT-4
transcribed_text = openai.chat.complete(
,
messages=[
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": audio_data} # Pass the audio data as user input
]
)
# Extract transcribed text from ChatGPT-4 output
transcribed_text = transcribed_text['choices'][0]['message']['content']
# Return the transcribed text as a JSON response
return JsonResponse({'transcribed_text': transcribed_text})
In this example, the audio data is received as a file upload. You can modify the implementation based on your specific requirements and use case.
Step 5: Configure URLs
Map a URL pattern to the speech recognition view function in your project's urls.py file:
from django.urls import path
from speech_recognition.views import speech_recognition
urlpatterns = [
# Other URL patterns
path('speech-recognition/', speech_recognition, name='speech_recognition'),
]
Step 6: Update Templates
In your Django templates, add a form or any other user interface component for capturing audio input. Use JavaScript to handle the audio recording and request the speech recognition endpoint.
<form >
<input capture>
<input >
</form>
Conclusion
Integrating speech recognition capabilities into your Django applications opens up new possibilities for user interactions and accessibility. By leveraging ChatGPT-4 or other speech recognition libraries, you can easily incorporate voice-based input into your web applications. Remember to handle audio processing and implement appropriate security measures to protect user privacy and ensure the best user experience.
With speech recognition, Django applications can become more inclusive and interactive, providing a seamless experience for users who prefer voice-based interactions. Experiment with speech recognition in your Django projects and unlock the full potential of voice-based input in your web applications.
Comments:
Great article, Billy! I've been struggling with speech recognition in Django. Looking forward to reading your insights.
Thank you, Maria! I'm glad you found the article helpful. Let me know if you have any specific questions.
Billy, when do you plan to cover more advanced topics related to speech recognition in Django?
Hi Maria! I'm currently working on a follow-up article that dives deeper into advanced techniques. Stay tuned!
That's great to hear, Billy! Looking forward to your advanced speech recognition article.
I never thought about using ChatGPT for improving speech recognition in Django. This sounds intriguing.
Interesting read, Billy! I wonder if this approach would work well for languages other than English.
Hi Sara! While the article focuses on English, the concepts can be applied to other languages as well. However, you might need to consider language-specific nuances.
Thanks for the clarification, Billy!
Billy, I appreciate the detailed examples you've provided in the article. They make it easier to understand and follow along.
I'm excited to give ChatGPT a try with Django speech recognition. Hopefully, it will improve accuracy.
Billy, do you have any recommendations for handling background noise in speech recognition?
Hi Matt! To handle background noise, you can preprocess the audio by applying noise reduction techniques before passing it to ChatGPT for transcription.
Thank you, Billy! I'll explore noise reduction techniques to improve speech recognition in Django.
Billy, have you compared the accuracy of ChatGPT with other speech recognition models?
Hi Robert! ChatGPT has proven to be quite accurate in my experiments. However, I recommend testing it on your specific use cases to validate its performance.
It's great to hear, Billy! ChatGPT has really revolutionized the way I handle speech recognition in my applications.
Thanks for the response, Billy! I'll give ChatGPT a try in my speech recognition project.
Thank you, Billy! I'll run some tests with ChatGPT to evaluate its performance in my use case.
Billy, do you have any recommendations for noise reduction techniques to use with speech recognition in Django?
Hi Matt! You can explore techniques like spectral subtraction, wavelet denoising, or deep learning-based denoising models to reduce noise before passing it to the speech recognition system.
Thank you, Billy! I'll dig into those techniques and experiment with improving speech recognition accuracy.
Great article! I have already implemented ChatGPT with Django and it's been a game-changer.
Billy, how does ChatGPT compare to other speech recognition libraries in terms of accuracy?
I'm a beginner in Django. Will I be able to follow your article and implement this approach?
Hi Emma! Yes, the article is beginner-friendly. I've tried to explain the concepts step-by-step. Give it a try!
Thank you, Billy! I'll definitely give it a shot. Your article has sparked my interest in Django.
Fantastic article, Billy! Looking forward to implementing this in my Django project.
Thanks, Billy, for this informative article. I've been meaning to integrate speech recognition into my Django project, and this seems like the way to go.
Billy, what are the main challenges you faced while implementing ChatGPT in Django?
Hi Laura! One of the main challenges was handling long audio files efficiently. Chunking them into smaller segments and processing them in parallel helped overcome this limitation.
I've been looking for a way to improve speech recognition in Django. Billy, thanks for sharing your knowledge!
Billy, your article couldn't have come at a better time. I was just about to start working on speech recognition in Django. Thanks!
Billy, which version of Django is best suited for implementing this approach?
Hi Nathan! This approach works well with Django 3.x and Django 4.x versions.
Fantastic insights, Billy! I'm excited to try implementing ChatGPT in Django for speech recognition.
Billy, could you recommend any specific use cases where ChatGPT with Django speech recognition shines?
Certainly, Sophie! ChatGPT combined with Django speech recognition can be applied in various domains, such as transcription services, voice assistants, and audio processing pipelines.
Billy, your article provides a clear roadmap for enhancing speech recognition in Django projects. Thanks for sharing your expertise!
Impressive work, Billy! I've been looking for ways to improve speech recognition accuracy, and your approach seems promising.
Billy, how does ChatGPT handle accents and dialects in speech recognition?
Hi Lucas! ChatGPT can handle different accents and dialects reasonably well, but it may benefit from fine-tuning or additional data specifically for certain accents or dialects.
Great article, Billy! I'm excited to implement ChatGPT in my Django project and see the improvements in speech recognition.
Billy, do you recommend any specific microphone models or configurations for better input quality in speech recognition?
Hi Liam! While it's not mandatory, using high-quality microphones and ensuring proper noise cancellation can significantly improve speech recognition accuracy.
Billy, how does ChatGPT handle real-time speech recognition?
Hi Charlotte! ChatGPT can handle real-time speech recognition, but it often depends on the specific implementation and the latency requirements of your application.
Billy, could you recommend any alternative speech recognition libraries or models in case ChatGPT doesn't meet specific requirements?
Hi James! If ChatGPT doesn't meet your specific requirements, you can explore other options like Mozilla's DeepSpeech, Google's Speech-to-Text API, or Nvidia's OpenSeq2Seq.
Thanks, Billy! Your article has given me some great ideas for improving speech recognition in my Django applications.