Leveraging ChatGPT for Automated Testing in LINQ Technology: Enhancing Efficiency and Accuracy
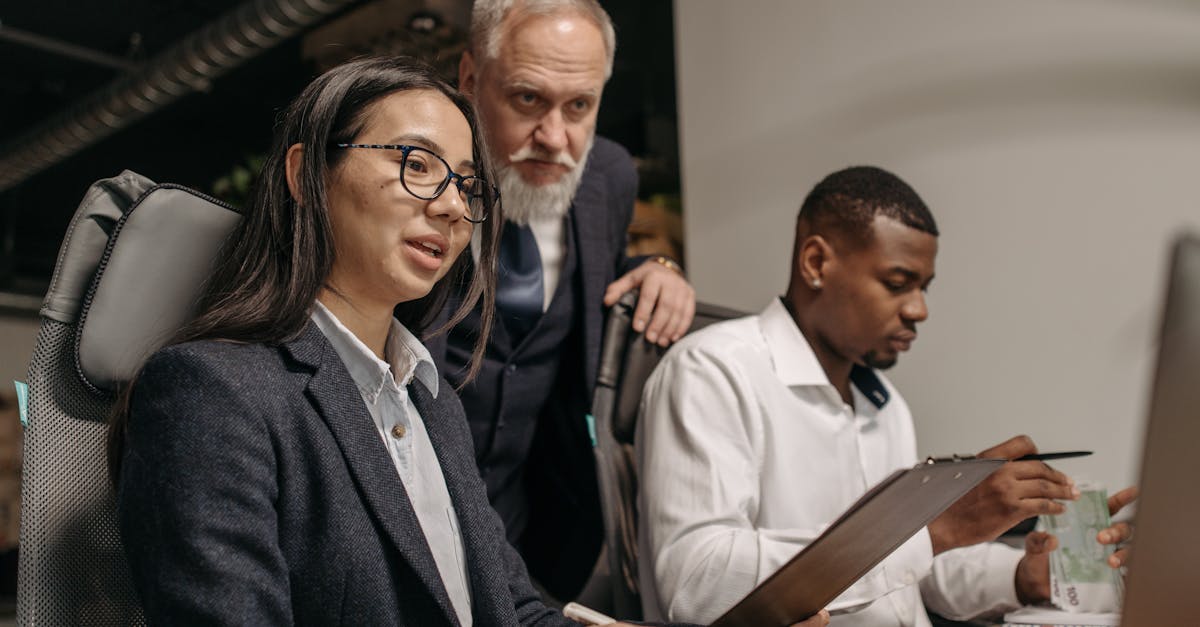
Technology: LINQ
Area: Automated Testing
Usage: It generates tests for LINQ queries and expressions to ensure they're working correctly.
Introduction
LINQ (Language Integrated Query) is a powerful technology that allows developers to query and manipulate data in a unified and expressive way. While LINQ is primarily used for data querying in various domains, it can also be employed for automated testing purposes.
Automated Testing with LINQ
Automated testing is an essential aspect of modern software development. It helps ensure the reliability and correctness of software applications. LINQ can be utilized to generate tests for LINQ queries and expressions, guaranteeing their accuracy and efficiency.
LINQ provides a rich set of querying capabilities, such as filtering, sorting, grouping, and aggregating data. By constructing test cases with LINQ, developers can simulate different scenarios and validate the behavior of LINQ queries and expressions. This approach simplifies the creation of test data, reduces manual effort, and improves test coverage.
Benefits of Using LINQ for Automated Testing
There are several advantages of leveraging LINQ for automated testing purposes:
- Expressive Test Cases: LINQ's declarative syntax allows developers to express test cases in a concise and readable manner. This makes it easier to understand the intended behavior of the tested LINQ queries and expressions.
- Data Generation: LINQ's querying capabilities can be used to generate test data. Developers can easily define and manipulate collections of objects with customized properties, enabling them to create diverse and relevant test scenarios.
- Data Validation: LINQ facilitates efficient data validation. Developers can compare the results of LINQ queries and expressions against expected outcomes, ensuring that the logic and calculations are correct.
- Efficiency: By automating the testing process with LINQ, developers can save time and effort, reducing manual testing tasks. LINQ's optimizations and query execution strategies also contribute to improved performance.
Example Usage
Let's consider an example where a LINQ query is employed to retrieve a list of active users from a database. In our automated testing scenario, we can verify the correctness of the query by generating test data with different user states (active, inactive, pending, etc.) and comparing the actual results to the expected list of active users.
IQueryable<User> activeUsersQuery = from user in dbContext.Users
where user.IsActive
select user;
List<User> expectedActiveUsers = new List<User>()
{
new User() { IsActive = true, Name = "John" },
new User() { IsActive = true, Name = "Jane" }
};
Assert.AreEqual(expectedActiveUsers, activeUsersQuery.ToList());
In the above example, LINQ enables us to construct the test case with ease and validate the query results against the expected list of active users.
Conclusion
LINQ is a versatile technology that extends beyond traditional data querying. By leveraging LINQ for automated testing, developers can improve the reliability and efficiency of their software applications. The expressiveness and querying capabilities of LINQ enable the generation of concise and effective test cases for LINQ queries and expressions, ensuring their accuracy and correctness.
Comments:
This article is very informative! I never considered using ChatGPT for automated testing in LINQ technology before. It seems like it could greatly enhance efficiency and accuracy.
I agree, Mary! Leveraging ChatGPT for automated testing in LINQ technology has the potential to revolutionize how we approach testing. The combination of AI and LINQ can be a game-changer.
Absolutely, Robert! Combining AI capabilities with LINQ technology opens up immense possibilities. I'm excited to explore this further.
Amy, I'm glad you share the excitement! It seems like ChatGPT can bring a new level of efficiency and innovation to the field of testing.
Robert, exactly! Embracing these advancements allows us to stay at the forefront of software testing.
Amy, staying updated with cutting-edge technologies like ChatGPT ensures that software testing keeps up with the ever-evolving software landscape.
Julia, you're absolutely right! Continuous learning and exploration of new tools ensure we stay ahead in the fast-paced field of software testing.
I'm not familiar with LINQ technology, but this article has piqued my interest. Can someone explain how ChatGPT can be harnessed for automated testing in this context?
Sure, Ann! LINQ stands for Language Integrated Query and it is a component of. NET Framework that provides a consistent way to query data from different sources. ChatGPT, being a language model, can be used to generate test cases automatically based on given specifications, making the testing process more efficient.
I have some concerns regarding the accuracy of ChatGPT. While it sounds promising, how do we ensure that the generated test cases are reliable?
Valid concern, Emily. One approach is to have a validation phase where human testers review and verify the automated test cases generated by ChatGPT. This way, any inaccuracies or false positives can be caught before implementation.
That seems like a sensible approach, Mark. Having human testers involved in the validation phase should help maintain the quality and accuracy of the test cases.
Mark, I completely agree. Fine-tuning the model and continuous iterations can greatly improve the accuracy and reliability of the automated testing process.
I've been using ChatGPT in other projects, and in my experience, it has been quite accurate. It's important to fine-tune the model for your specific domain and have the necessary checks in place to handle edge cases.
Interesting article! I wonder if there are any limitations to using ChatGPT for automated testing. Are there any scenarios where it might not be as effective?
Great question, Alex! While ChatGPT can be powerful, it's not suitable for testing scenarios that require visual verification or complex user interactions. In those cases, it's still necessary to have human testers involved.
Thanks for the clarification, Julia! It's good to know the limitations of ChatGPT to better assess its applicability in different testing scenarios.
You're welcome, Alex! It's always important to assess the strengths and limitations of any tool or technology to make informed decisions.
Thank you all for your comments and questions! It's great to see the interest in leveraging ChatGPT for automated testing in LINQ technology. I'll try my best to address your concerns and provide further insights.
I appreciate your willingness to address our queries, Francois. Looking forward to learning more from your insights.
I have been using ChatGPT in my testing workflows and it has been fantastic! The time saved in test case generation is remarkable. Highly recommend giving it a try.
Daniel, could you provide some examples of how you've utilized ChatGPT in your testing workflows? I'm curious to hear about your experiences.
Sure, Mary! One example is using ChatGPT to automatically generate API test cases based on the expected input/output combinations. It significantly reduced the manual effort required to create test cases.
That sounds impressive, Daniel! It's amazing to see how AI can streamline testing workflows.
Mary, I couldn't agree more. AI has the potential to transform the way we approach testing and improve overall efficiency.
Daniel, I completely agree. The potential impact of AI on testing efficiency is remarkable, and ChatGPT seems to be one of the frontrunners.
Mary, another way I've used ChatGPT is for generating test environments and datasets automatically. It saves a lot of time in prepping the necessary infrastructure.
I'm curious about the computational requirements for leveraging ChatGPT in automated testing. Does it require substantial computing power?
Good question, Michael! While ChatGPT is resource-intensive during inference, it can be efficiently managed by utilizing cloud computing platforms or powerful hardware configurations.
Emily, absolutely! The collaboration between AI-generated test cases and human testers creates a more robust testing process.
Well said, Mark! It's the combination of AI and human expertise that optimizes the overall effectiveness of testing.
Thank you for explaining, Paul! You've rightly highlighted one of the key benefits of leveraging ChatGPT for automated testing in LINQ technology.
Francois, thank you for sharing this enlightening article. It's a fresh perspective on leveraging ChatGPT for automated testing.
Robert, being open to embracing new horizons like ChatGPT paints a bright future for the testing community.
Robert, staying at the forefront of technology also lets us adapt to the changing landscape of the software industry.
Amy, embracing innovation is what keeps the field of testing exciting and relevant. ChatGPT can certainly be a valuable addition.
Sarah, continuous learning and adaptation are essential as AI technologies like ChatGPT evolve to become better testing assistants.
Amy, continuous refinement and adaptation are key to leveraging AI effectively in software testing.
Julia, I couldn't agree more. AI technologies like ChatGPT are still evolving, hence the need to continuously learn and refine their applications in testing.
Sarah, precisely! Combining AI's automation capabilities with human judgment ensures that potential blind spots and edge cases are covered.
Mark, well said! Continuous improvement and learning are essential in harnessing the full potential of AI for testing purposes.
Sarah, well said! The dynamic nature of AI technologies calls for an adaptable and continuously improving testing approach.
Amy, keeping up with emerging trends and adopting innovative solutions ensures our testing strategies remain effective and efficient.
You're welcome, Robert! I'm thrilled that the article has sparked interest and provided valuable insights into leveraging ChatGPT in automated testing.
Francois, your article has truly opened our minds to the possibilities of ChatGPT for automated testing. It's an exciting time for the testing community.
Robert, being ahead of the curve and embracing innovative solutions like ChatGPT puts us in a better position to tackle future testing challenges.
Amy, striking the right balance between AI automation and human intervention is key to achieving the optimum testing process.
Amy, indeed! Embracing emerging trends and innovative solutions enables us to continuously evolve and improve our testing practices.
Amy, staying updated and incorporating technological advancements like ChatGPT keeps us ahead in the ever-evolving software testing landscape.
Amy, staying updated with emerging technologies helps us deliver quality testing services to our clients, and ChatGPT seems to be an important addition to our arsenal.
Anna, you're absolutely right! Being at the forefront of technology enables us to offer cutting-edge solutions and value to our clients.
Sarah, continuous improvement and learning are crucial elements in adapting to the evolving landscape of AI-powered testing.
You're welcome, Robert! I'm glad you found the article enlightening. It's an exciting time for individuals and organizations interested in innovative testing approaches.
Robert, keeping up with the latest trends and embracing innovative solutions helps us stay ahead in the testing landscape.
Amy, I couldn't agree more. The testing landscape is dynamic, and staying updated with emerging technologies ensures we remain relevant.
Amy, absolutely! Continuous learning and adaptation are essential when incorporating AI technologies into any testing workflow.
Mark, indeed! The collaboration between AI and human testers acts as a checks-and-balances system, ensuring thorough and accurate testing.
Thank you all for the engaging discussion! It's been great to hear your thoughts and experiences. If you have any further questions, please feel free to ask.
Thank you, Francois! Your article has sparked my interest in exploring ChatGPT for automated testing in LINQ technology.
You're welcome, Ann! I'm thrilled to hear that the article has inspired you to explore ChatGPT further. It's a fascinating technology with great potential.
Francois, thank you for your willingness to address our questions and provide insights. It's greatly appreciated.
Absolutely, Ann! Francois has provided us with valuable insights into the possibilities of leveraging ChatGPT for enhanced testing.
Francois, thank you for sharing your knowledge and engaging with us. This discussion has been enlightening.
Thank you, Francois! This has been an engaging discussion, and your expertise has shed light on the potential of ChatGPT in automated testing.
Francois, thank you for your time and valuable insights! This discussion has undoubtedly broadened our understanding of ChatGPT in automated testing.
You're welcome, Ann! I'm honored to have been part of this educational conversation. As always, feel free to reach out if you have any further questions.
Francois, thank you once again for sharing your expertise on ChatGPT. This discussion has further fueled my curiosity about AI in the testing domain.
Mary, I'm glad my insights have been helpful! ChatGPT offers so much potential in reducing manual efforts and improving testing efficiency.
Thank you all for your active participation in this discussion! It's been insightful hearing your perspectives and experiences with ChatGPT and automated testing.
Francois, thank you for shedding light on this fascinating aspect of automated testing! Your insights have been immensely helpful to the community.
Thank you, Alex! I'm glad to have contributed to the community's understanding of leveraging ChatGPT in automated testing. Feel free to inquire further if needed.
Thank you, Francois! This discussion has been informative and has really highlighted the potential of ChatGPT for automated testing.
Mark, absolutely! Human testers provide the essential context and intuition that AI models like ChatGPT still lack.
Emily, having the flexibility to manage the computational requirements is crucial for utilizing ChatGPT effectively in automated testing.
Thank you all once again for your active participation and valuable insights! It has been a pleasure discussing ChatGPT for automated testing with such an engaged community.