Maximizing Efficiency: Utilizing ChatGPT in Eager Loading with Entity Framework
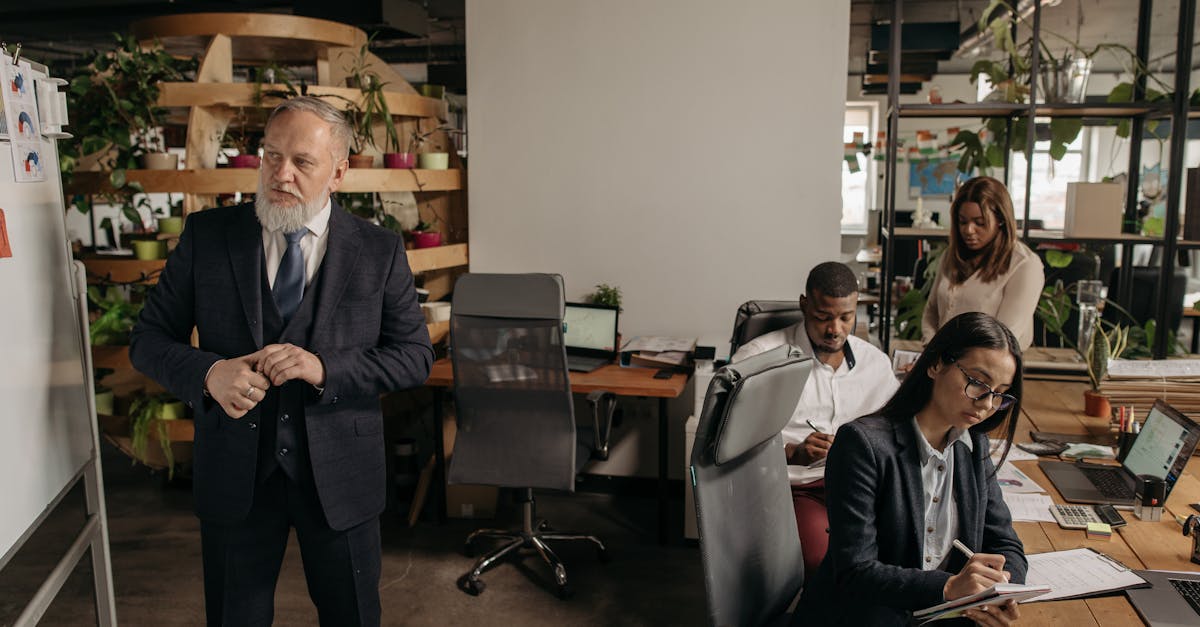
Entity Framework is a powerful Object-Relational Mapping (ORM) framework that enables developers to work with databases using object-oriented techniques. One of the key features of Entity Framework is Eager Loading, which allows developers to load related entities in a single query, reducing the number of round trips to the database and improving the overall performance of an application.
Eager Loading is particularly beneficial in scenarios where we need to access multiple related entities. Without Eager Loading, Entity Framework would retrieve the data for each related entity separately, resulting in additional round trips to the database.
ChatGPT-4, the latest version of OpenAI's language model, can provide helpful instructions on implementing Eager Loading with Entity Framework.
Step 1: Define Relationships in the Data Model
The first step in implementing Eager Loading is to define the relationships between the entities in the data model. Entity Framework uses these relationships to determine how to generate the appropriate SQL queries to retrieve the related entities.
For example, consider a simple data model consisting of two entities: Author
and Book
. An Author
can have multiple Book
entities associated with it. To define this relationship in Entity Framework, you would create a foreign key property in the Book
entity that references the Author
entity.
Step 2: Query the Data with Include
Once the relationships are defined, you can use the Include
method to eager load related entities in a query. The Include
method tells Entity Framework to fetch the specified navigation property along with the primary entity.
Using our example data model, if we want to retrieve a list of authors along with their books, we can construct a query like this:
var authorsWithBooks = context.Authors
.Include(a => a.Books)
.ToList();
This query will retrieve all authors from the database and also load their associated books in a single round trip to the database, thanks to Eager Loading.
Step 3: Access the Loaded Data
Once the data is loaded into memory, you can access the related entities directly from the primary entity. In our example, you can loop through the authorsWithBooks
collection and access the associated books for each author.
foreach (var author in authorsWithBooks)
{
Console.WriteLine($"Author: {author.Name}");
foreach (var book in author.Books)
{
Console.WriteLine($"Book: {book.Title}");
}
}
By utilizing Eager Loading, you can access the related entities without triggering additional queries to the database, resulting in improved performance and reduced latency.
ChatGPT-4 can provide comprehensive guidance on implementing Eager Loading with Entity Framework for your specific application requirements. It can assist you with advanced scenarios, such as filtering, sorting, and pagination, while still leveraging the benefits of Eager Loading.
Conclusion
Eager Loading is a powerful feature of Entity Framework that allows developers to reduce round trips to the database by fetching related entities in a single query. By implementing Eager Loading, you can improve the performance and responsiveness of your application.
With the assistance of ChatGPT-4, you can obtain helpful instructions tailored to your specific use case on how to effectively implement Eager Loading with Entity Framework. Leverage this technology to optimize your application's performance and reduce the time taken to retrieve related entities from the database.
Comments:
Great article! I've been looking for ways to improve efficiency with Entity Framework. ChatGPT seems like a promising tool.
I totally agree, Sophia! Incorporating ChatGPT in eager loading sounds like a smart approach. Cantrina Dent, what do you think about this technique?
Thank you both for your comments! I appreciate your interest. Eager loading with Entity Framework can indeed benefit from utilizing ChatGPT. It provides faster data retrieval and reduces unnecessary round trips to the database. Plus, the intelligent responses from ChatGPT improve the overall user experience.
I've tried eager loading before, and it significantly improved the performance. Adding ChatGPT to the mix sounds intriguing. Cantrina, have you encountered any specific challenges with this approach?
Karen, integrating ChatGPT into eager loading can introduce challenges in maintaining a balance between performance and accuracy. It requires training the model properly and monitoring its responses to ensure reliable results.
Thanks for sharing your insights, Cantrina. It sounds like a delicate balancing act, indeed.
I wonder how well ChatGPT handles complex relational data with eager loading. Cantrina, any thoughts on this?
Ethan, ChatGPT's performance with complex relational data largely depends on the training data it has been exposed to. With proper training, it can handle complex relationships effectively, ensuring accurate responses.
I suppose the benefits could outweigh the complexity, especially if the system is designed for scalability. Cantrina, any recommended resources for implementing ChatGPT with eager loading?
Benjamin, to successfully integrate ChatGPT with eager loading, you can refer to the OpenAI documentation, which provides implementation details, best practices, and guidelines for training the model specifically for your use case.
Thank you, Cantrina! I'll make sure to check it out.
I'm concerned about the inherent bias and ethical considerations of employing ChatGPT for data retrieval. Cantrina, did you face any ethical challenges while implementing this approach?
Olivia, ethical considerations are indeed crucial when implementing AI models like ChatGPT. It's essential to review and mitigate biases during training and monitor model responses to ensure fairness and avoid potential issues.
Thank you for addressing my concern, Cantrina. It's reassuring to know that steps are taken to ensure ethical usage.
Indeed, Cantrina. Thank you for your expertise and for addressing our questions.
Thank you, Cantrina. Your responses were informative.
I have some concerns though. Won't using ChatGPT add an extra layer of complexity to the system?
That's a valid point, Liam. The integration may require some additional setup and maintenance, but the potential benefits outweigh the extra complexity.
That's good to know. Thanks for clarifying!
How does the performance of eager loading with ChatGPT compare to other optimization techniques?
William, eager loading with ChatGPT can offer superior performance compared to other optimization techniques. It minimizes the number of database queries and leverages the intelligent responses from ChatGPT to enhance the user experience.
That's impressive, Cantrina! I'm excited to explore this further.
Is there a risk of overreliance on ChatGPT? It might cause issues if the model becomes unavailable.
James, you bring up a valid concern. It's crucial to have contingency plans and fallback mechanisms in place to handle situations when the model is unavailable. Proper error handling ensures the system maintains functionality even in those scenarios.
Thanks for the response, Cantrina. I'll keep that in mind while implementing it.
As a developer, I'm always concerned about performance bottlenecks. Does eager loading with ChatGPT help address these bottlenecks effectively?
Isabella, eager loading with ChatGPT can significantly reduce performance bottlenecks by reducing the number of database round trips and enhancing user interactions with intelligent responses.
That's great to hear! Thanks, Cantrina.
Thank you, Cantrina! Your input has been immensely valuable.
What are the limitations of this approach? Are there any scenarios where eager loading with ChatGPT might not be suitable?
Oliver, while eager loading with ChatGPT offers significant benefits, it may not be suitable for real-time systems with stringent response time requirements. Additionally, if the data volume is excessively large, it might impact performance.
Thanks for the clarification, Cantrina. I'll keep those factors in mind.
ChatGPT relies on pre-trained models. Cantrina, how frequently should the models be updated to maintain optimal performance?
Emily, updating the models periodically is recommended to ensure optimal performance. The frequency depends on factors like data changes, user feedback, and advancements in the underlying models.
Got it. Thank you for the insight, Cantrina.
Is there a significant resource overhead associated with ChatGPT in eager loading?
Michael, integrating ChatGPT in eager loading may require additional computational resources for running the models. It's essential to ensure the infrastructure is appropriately provisioned to handle the increased resource requirements.
That's something to consider. Thanks for the heads up, Cantrina.
We appreciate your time and expertise, Cantrina. Thank you!
Do you have any examples or case studies showcasing the effectiveness of eager loading with ChatGPT?
Sophie, you can find case studies and examples of the effectiveness of eager loading with ChatGPT on the OpenAI website. They provide insights into how varying applications have achieved improved efficiency using this approach.
Thank you, Cantrina. I'll check out the case studies for more information.
Cantrina, thank you for being so accessible and responsive to our questions.
What kind of impact does ChatGPT integration have on system scalability?
Daniel, integrating ChatGPT in eager loading doesn't significantly impact system scalability. With the right infrastructure and optimization techniques, the system can still handle growing user bases and increasing data volumes effectively.
Thank you for your guidance and expertise, Cantrina.
I'm curious about the training aspect. How much training data is typically required for a reliable ChatGPT model?
Naomi, the amount of training data required for a reliable ChatGPT model can vary based on the complexity of your use case. It's recommended to have a diverse dataset of a few thousand examples, but further fine-tuning can be done to improve performance.
That gives me a better idea. Thank you, Cantrina!
Cantrina, how do you address privacy concerns when ChatGPT interacts with user data via eager loading?
Sophia, privacy concerns are carefully addressed by ensuring proper access control measures, data encryption, and anonymization when ChatGPT interacts with user data. It's essential to align with privacy regulations and follow best practices to protect user information.
Thank you for clarifying that, Cantrina. It's crucial to prioritize data privacy.
Thank you, Cantrina! This discussion has been enlightening. I appreciate your active involvement.
In scenarios where data changes frequently, how does ChatGPT handle dynamic content retrieval through eager loading?
Oliver, ChatGPT can handle dynamic content retrieval through eager loading by incorporating mechanisms like caching or invalidation strategies. The system can be designed to detect changes and update the model accordingly to maintain accuracy.
That makes sense. Thank you, Cantrina.
Cantrina, thank you for sharing your expertise and insights.
Thank you all for actively participating in this discussion! Your questions and insights are valuable. If you have any more queries or thoughts, please feel free to share.
You're all very welcome! It has been a pleasure discussing this topic with such an engaged community. Feel free to reach out anytime.