Revolutionizing Unit Testing in C++: Harnessing the Power of ChatGPT
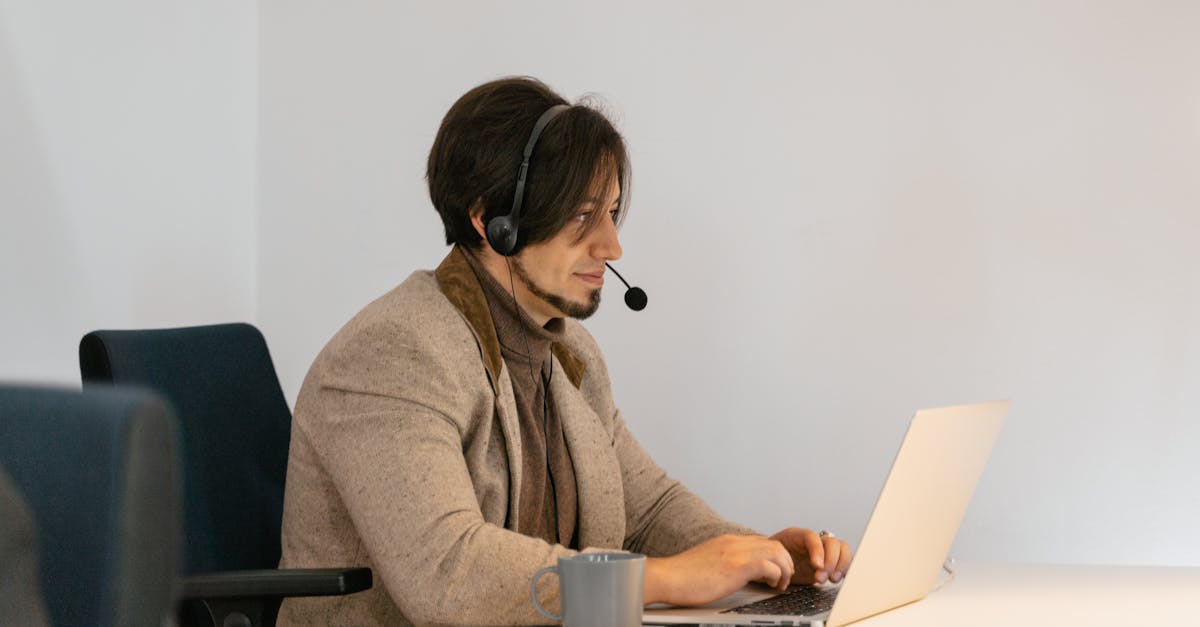
C++ is a powerful programming language commonly used for developing high-performance applications, including systems software, game engines, and libraries. Along with its robustness, C++ provides developers with the ability to write unit tests to ensure the correctness of their programs.
What is Unit Testing?
Unit testing is a software testing methodology where individual units of source code are tested to determine whether they are functioning as expected. In C++, a unit typically refers to a function, class, or component.
Why is Unit Testing Important?
Unit testing is crucial for several reasons:
- Verification: Unit tests verify the correctness of the code by checking its behavior against known input, output, and functionality.
- Identification of Bugs: Unit tests can help identify bugs and issues early in the development process, making it easier to fix them.
- Refactoring: Unit tests provide confidence in refactoring or modifying existing code, as they ensure that the intended functionality remains intact.
- Regression Testing: Unit tests act as a safety net, preventing the introduction of new bugs when making changes to the codebase.
How to Write Unit Tests in C++
C++ provides several frameworks and libraries that facilitate writing unit tests.
- Google Test: Google Test is a popular C++ testing framework that provides a rich set of assertions, fixtures, and utilities for writing and running tests. It follows the xUnit architecture.
- Catch2: Catch2 is another widely used C++ testing framework known for its simplicity and expressiveness. It supports various styles of test specification.
- Boost.Test: Boost.Test is part of the Boost C++ Libraries and offers a comprehensive set of testing capabilities. It integrates seamlessly with other Boost libraries.
Considerations for Writing Unit Tests
When writing unit tests for C++ programs, consider the following scenarios and edge cases:
- Boundary Conditions: Test the behavior of the code at the boundaries of expected input values.
- Null or Invalid Input: Test how the code handles null or invalid input parameters.
- Corner Cases: Test for unusual or extreme input values that might trigger unexpected behavior.
- Exception Handling: Test how the code handles exceptions and whether appropriate exception handling mechanisms are in place.
- Performance: If relevant, write tests to verify the performance characteristics of the code under different loads and scenarios.
By considering a wide range of scenarios and edge cases, you can increase the confidence in the correctness and robustness of your C++ programs.
Conclusion
Unit testing is an essential practice in software development, and C++ provides developers with a variety of frameworks and libraries to facilitate this process. By writing unit tests, developers can verify the correctness of their code, identify bugs early, and ensure the integrity of their systems. Considering different scenarios and edge cases enhances the effectiveness of unit tests and improves the overall quality of the software produced with the C++ language.
Comments:
Thank you for reading my article on Revolutionizing Unit Testing in C++. I hope you found it informative and helpful!
Great article, Amanda! Unit testing is such an important part of software development, and it's interesting to see how ChatGPT can be used to enhance it.
Thank you, Mike! Indeed, ChatGPT opens up new possibilities for improving unit testing in C++. It can help automate test generation and provide interactive feedback.
I never thought about using AI in unit testing before. This article makes me want to explore it further. Thanks, Amanda!
You're welcome, Sarah! AI can be a powerful tool in various areas of software development, and unit testing is no exception. I'm glad you found it intriguing!
The idea of generating test cases using ChatGPT is fascinating. I wonder how it compares to other testing frameworks out there.
Good point, Tom! While ChatGPT can assist in generating test cases, it's important to consider factors like code coverage and the specific testing needs of your project. It can be a valuable addition to existing frameworks.
I've been using C++ for years, but I've struggled with writing comprehensive tests. This article has given me some ideas to improve my approach. Thanks!
You're welcome, Rachel! Unit testing can be challenging, but with the right techniques and tools, the process becomes much smoother. I'm glad I could provide you with some helpful insights.
ChatGPT seems like a promising technology, but I wonder about potential limitations when dealing with complex C++ codebases. Any thoughts, Amanda?
That's a valid concern, David. While ChatGPT can handle complexity to some extent, it may face challenges in highly intricate codebases. It's essential to understand its limitations and combine it with other testing practices for comprehensive coverage.
I appreciate how this article emphasizes the importance of proper unit testing. It's often overlooked but plays a crucial role in software quality.
Absolutely, Emily! Unit testing is essential for maintaining the stability and reliability of code. By investing time in writing proper tests, developers can detect issues early and ensure higher software quality.
The concept of using ChatGPT for unit testing is exciting, but I'm concerned about potential false positives or false negatives. Thoughts?
Valid point, Daniel. Like any AI-based approach, false positives and negatives are possible. It's crucial to validate the generated tests and use other testing techniques to minimize such occurrences.
I'm interested in trying out ChatGPT for my C++ projects. Are there any specific libraries or tools you recommend, Amanda?
Certainly, Sophia! For integrating ChatGPT into your C++ projects, you can explore libraries like OpenAI's GPT-3 library or other AI frameworks compatible with C++. These can provide an interface to leverage ChatGPT's capabilities in your unit testing process.
I appreciate how this article demonstrates practical examples of using ChatGPT. It helps visualize the potential benefits.
Thank you, Michael! Concrete examples can enhance understanding and inspire developers to explore innovative approaches. I'm glad you found them helpful.
I can see ChatGPT being useful for generating edge cases in unit testing. It can help cover scenarios that developers might overlook.
Exactly, Linda! Edge cases are often overlooked, but they are crucial for ensuring robustness. ChatGPT can assist in generating such scenarios and greatly improve test coverage.
This article has given me a fresh perspective on unit testing. The combination of AI and testing is fascinating!
I'm thrilled to hear that, Chris! The integration of AI into unit testing can indeed revolutionize the way we approach software quality. It opens up new possibilities and enhances testing effectiveness.
I wonder if ChatGPT can be used for testing legacy C++ codebases or if it's more suitable for new projects.
Good question, Julia! ChatGPT can be used for both legacy codebases and new projects. However, it may require more effort to adapt and integrate with existing code in legacy systems.
As a software testing enthusiast, I find this article inspiring. The potential for AI in testing is vast!
I'm glad to hear that, Robert! AI has immense potential in software testing, and advancements in technologies like ChatGPT continue to unlock new possibilities. Exciting times ahead!
I'm curious about the training process for ChatGPT when it comes to C++. How does it handle the language's intricacies?
Great question, Alexandra! ChatGPT is trained on a large corpus of text from the internet, including C++ resources. While it can handle many intricacies of the language, it's essential to validate the generated tests to ensure correctness within your project's specific context.
I can see tremendous potential in ChatGPT for reducing manual effort in unit testing. It could free up developers to focus on other critical tasks.
Absolutely, Jonathan! Automating certain aspects of unit testing with ChatGPT can save developers time and effort. It allows them to allocate more energy towards designing robust software and addressing complex challenges.
This article has sparked my interest in AI-driven testing. Are there any recommended resources to learn more?
Certainly, Grace! You can explore resources like AI-driven testing books, online tutorials, and research papers. OpenAI's documentation and forums are also valuable sources to gain insights into leveraging ChatGPT for testing. It's an exciting field to dive into!
I'm cautiously optimistic about AI-assisted testing. While it has great potential, it should be used as a tool alongside other testing practices.
Well said, Daniel! AI-assisted testing is a powerful approach, but it should supplement, not replace, other testing practices. The combination of human expertise, traditional testing methods, and AI tools can lead to more effective testing processes.
I have reservations about relying too heavily on AI for testing. Human intuition and creativity might be difficult to replicate.
Valid concern, Olivia! Human intuition and creativity play a crucial role in testing. While AI can automate certain aspects, the human element is irreplaceable. AI tools should be used to augment human skills, helping us tackle complex scenarios more effectively.
I'm excited to see how ChatGPT progresses in the future. Its potential in software testing is just the tip of the iceberg!
Absolutely, Lucas! ChatGPT's capabilities continue to evolve, and it's exciting to think about the possibilities. As it advances, we'll likely see even more innovative applications across various domains, including software testing.
Do you have any recommendations for integrating ChatGPT into existing testing frameworks, Amanda?
Certainly, Emily! Depending on your testing framework, you can leverage ChatGPT's capabilities by building a custom integration or utilizing existing AI libraries compatible with your chosen framework. The key is to establish a seamless connection between ChatGPT and your testing environment.
This article has sparked a lot of discussion. It's exciting to see the intersection of AI and unit testing getting attention!
I'm thrilled about the lively discussion too, Michelle! The fusion of AI and unit testing is an emerging area with immense potential. It's gaining attention because it offers new possibilities to improve software quality and development processes.
Thank you for sharing your insights, Amanda. This article has piqued my interest in exploring AI techniques for my testing projects!
You're very welcome, Peter! I'm delighted to hear that the article has sparked your interest in AI techniques for testing. There's a wealth of potential waiting to be explored, and I hope you find success in your projects!
I'm excited about the future of AI in testing, but I also worry about job displacement in the testing industry.
That's a valid concern, Samantha. While AI can automate certain tasks, it's unlikely to replace human testers entirely. AI tools will likely augment the testing process, freeing up testers to focus on more complex analysis and critical decision-making.
Great article, Amanda! It's fascinating to see the application of AI in a domain I'm passionate about.
Thank you, Mark! AI's application in software testing is an exciting space, and I'm thrilled to see it spark interest in fellow enthusiasts like you. It's a testament to the ever-evolving nature of our industry!
I appreciate how this article highlights the potential benefits and considerations when using ChatGPT for unit testing. It's important to have a balanced perspective.
Absolutely, Natalie! Adopting any new technology or approach requires weighing both the benefits and considerations. It ensures a balanced understanding and allows for informed decision-making. I'm glad you found the article comprehensive in that regard!