Unleashing the Power of ChatGPT: Revolutionizing the Angular JS Technology
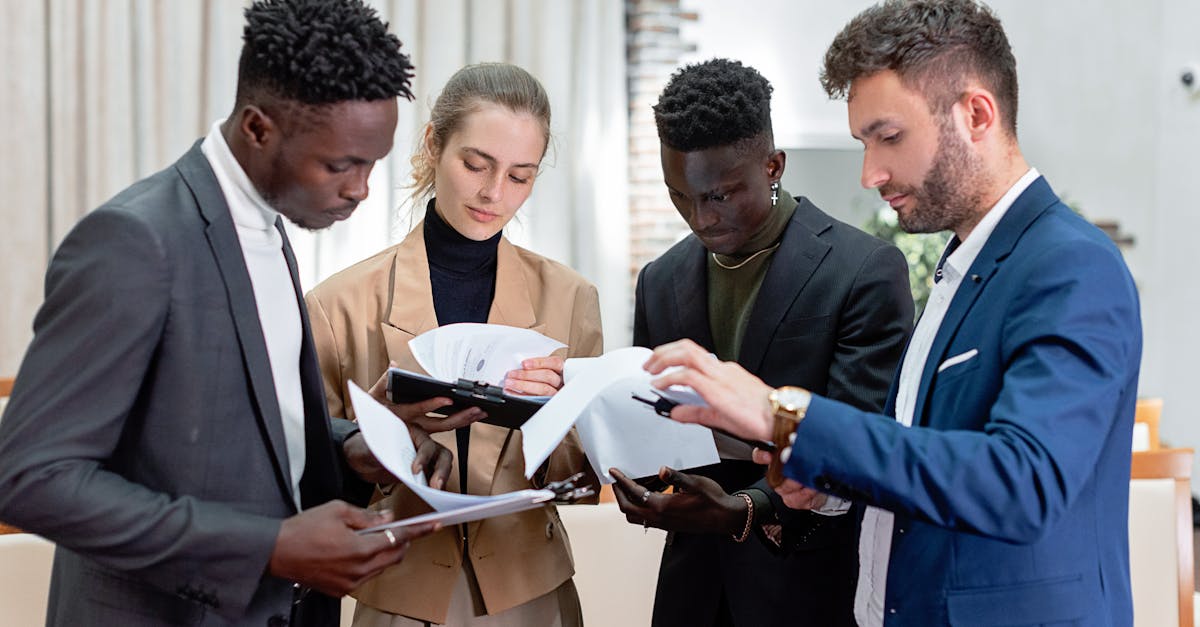
AngularJS is a powerful JavaScript framework that provides a comprehensive set of tools for building dynamic web applications. One of its key features is its ability to enable real-time data binding. This allows changes in the model to be automatically reflected in the view without requiring any manual updates.
Understanding Real-Time Data Binding
Real-time data binding is a fundamental concept in AngularJS that enables developers to create interactive and responsive web applications. It eliminates the need for manual updates by automatically synchronizing the model and the view.
When a variable in the model changes, AngularJS updates the corresponding element in the view, and vice versa. This bidirectional data flow ensures that any changes made to the model are immediately reflected in the user interface.
Implementing Real-Time Data Binding with AngularJS
The implementation of real-time data binding in AngularJS is straightforward. First, you need to include the AngularJS library in your HTML file:
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.9/angular.min.js"></script>
Next, you can define your application module and controller:
<script>
var app = angular.module('ChatApp', []);
app.controller('ChatController', function($scope) {
// Define your model and functions here
});
</script>
Inside your controller, you can define your model and functions that handle the real-time data binding. For example, you can create a chat messaging system where messages are dynamically updated in real-time:
<div ng- ng->
<div ng->
{{ message.text }}
</div>
<input ng->
<button ng->Send</button>
</div>
In the above code snippet, the ng-repeat
directive is used to iterate over each message in the messages
array and display its text. The ng-model
directive binds the input field to the newMessage
variable in the controller, allowing users to enter new messages. The ng-click
directive is used to trigger the sendMessage
function when the send button is clicked.
ChatGPT: Demonstrating Real-Time Data Binding
With the concept of real-time data binding in AngularJS, you can create interactive experiences, such as chat applications. One example of such an application is ChatGPT.
ChatGPT is a chatbot powered by OpenAI's GPT-3 language model. It can be integrated with AngularJS to demonstrate real-time data binding dynamically via an interactive chat interface. As users type messages, the chatbot generates responses in real-time, creating a seamless and interactive conversation.
By utilizing AngularJS's real-time data binding capabilities, ChatGPT can display messages and responses without the need for manual updates. The chat interface automatically updates as new messages or responses are received, providing users with a dynamic and engaging experience.
Conclusion
Real-time data binding is a powerful feature provided by AngularJS, enabling developers to build highly interactive and responsive web applications. By utilizing this capability, developers can create seamless and dynamic user experiences, as demonstrated by ChatGPT.
Whether you are building a chat application, a live data dashboard, or any other real-time application, AngularJS's real-time data binding can greatly simplify the process and enhance the user experience.
Comments:
Thank you all for visiting my blog! I'm excited to bring you this article on unleashing the power of ChatGPT and its potential impact on Angular JS technology.
Great article, Melissa! I've always been a fan of Angular JS, and this new integration with ChatGPT sounds promising. Can't wait to see how it revolutionizes the technology.
I'm also excited about this potential integration. Angular JS is already powerful, and with the addition of ChatGPT, it can open up even more possibilities for developers.
Is there any documentation available on how to utilize ChatGPT with Angular JS? I'd love to explore it further.
Robert Foster and Emily Watson, thank you for your positive feedback! I also believe this integration has immense potential. George Adams, currently there is no official documentation available, but I plan to cover that in my next blog post to provide more insights into utilizing ChatGPT with Angular JS.
Wow, I can imagine the possibilities! ChatGPT could greatly enhance the user experience of Angular JS applications.
I'm a beginner in Angular JS, so this integration sounds a bit intimidating to me. Will it be easy for newcomers to adopt?
Megan Simmons, indeed! The combination of ChatGPT and Angular JS can significantly improve user experience. Jacob Davis, while there might be a learning curve for newcomers, the integration will provide new opportunities and improve productivity for developers of all levels. I'll make sure to cover that aspect in future posts as well.
This could be a game-changer! Angular JS is a popular framework, and adding the power of ChatGPT opens up a whole new world of possibilities.
Daniel Ramirez, I completely agree! This integration has the potential to revolutionize how we develop Angular JS applications and enhance their capabilities. The future looks exciting for developers! If you have any questions or specific topics you'd like me to cover, feel free to let me know.
I'm curious to know if there are any limitations or challenges when using ChatGPT with Angular JS. Any insight on that, Melissa?
Sophie Turner, that's a great question. While ChatGPT brings many benefits, there might be challenges related to training data, context handling, and real-time interactions. It's important to be aware of these challenges and explore potential solutions. In my upcoming posts, I'll dive deeper into these aspects of using ChatGPT with Angular JS.
Looking forward to your next blog post, Melissa. This integration sounds promising, and I can't wait to learn more about it!
Oliver Lee, thank you for your support! I'm glad you find this integration promising. Stay tuned for more detailed insights in my next blog post!
Will this integration have any impact on Angular JS performance? I'm concerned about introducing potential bottlenecks.
Liam Collins, that's a valid concern. While there might be some performance impact, the gains in functionality and user experience can outweigh it. It's crucial to strike a balance between performance optimization and utilizing the power of ChatGPT. I'll address this aspect in more detail in future posts to assist developers in making informed decisions.
This is exciting! Just when I thought Angular JS couldn't get any better, ChatGPT comes along. Can't wait to experiment with it.
Grace Thompson, I'm thrilled that you find this integration exciting! The combination of Angular JS and ChatGPT indeed opens up a new realm of possibilities. Feel free to share your experiments and discoveries as you explore it further!
Melissa, this is such an innovative idea! Kudos to you for exploring the potential of ChatGPT in the Angular JS landscape.
Samuel Scott, thank you for your kind words! I'm passionate about exploring the potential of new technologies and sharing insights with the developer community. It's an exciting journey, and I'm glad to have you all along!
As a developer, I'm always on the lookout for ways to improve user experience. Can't wait to check out this integration. Thanks for sharing, Melissa!
Emma Clark, you're welcome! I'm glad you're excited about improving user experience. This integration has the potential to take it to new heights. Feel free to share your thoughts and experiences once you check it out!
This sounds like a fascinating combination! I'm looking forward to seeing the practical applications of ChatGPT in the Angular JS ecosystem.
Sarah Peterson, I share your excitement! The possibilities of practical applications are indeed fascinating. As developers experiment and explore this integration, we'll uncover new use cases and ways to leverage ChatGPT in the Angular JS ecosystem.
Will ChatGPT make it easier to build conversational interfaces with Angular JS? It could be a game-changer for chatbots and virtual assistants.
Alice Roberts, absolutely! Building conversational interfaces with Angular JS can be greatly facilitated with ChatGPT. The natural language processing capabilities of ChatGPT can revolutionize chatbots and virtual assistants by making them more intelligent and context-aware. The potential is indeed game-changing!
I have concerns about potential security vulnerabilities with ChatGPT. How can we ensure secure integration with Angular JS applications?
David Morgan, security is an essential consideration indeed. As with any integration, it's crucial to follow best practices for secure implementation. I'll address security aspects and provide guidelines for ensuring a secure integration with Angular JS in an upcoming blog post. Stay tuned!
I'm impressed by your insights, Melissa! The potential benefits of combining Angular JS and ChatGPT seem immense. Looking forward to more informative posts from you.
Ryan Cooper, thank you for your kind words! I'm glad you find the potential benefits immense. I'm committed to providing more informative posts to assist developers in leveraging the power of this integration. If you have any specific topics or questions in mind, feel free to share.
This is exciting news! I can't wait to explore the possibilities of ChatGPT in Angular JS development.
Lucy Anderson, I'm glad you're excited about the possibilities! As you explore ChatGPT in Angular JS development, feel free to share your experiences and insights. Together, we can push the boundaries of what's possible in this field.
Will this integration require additional resources or dependencies for Angular JS projects? I'm concerned about the impact on project setup and maintenance.
John Collins, that's a valid concern. While there might be additional resources or dependencies to consider, proper setup and maintenance practices can help mitigate any potential issues. In future posts, I'll cover these aspects to ensure a smooth integration without adversely affecting project setup and maintenance.
As a developer, I'm always looking for ways to enhance user interactions. This integration seems like a step in the right direction. Can't wait to give it a try!
Sophia Mitchell, I'm glad you see the potential in enhancing user interactions! Giving it a try is definitely the best way to explore its possibilities firsthand. Feel free to share your feedback and experiences once you dive into it!
How does ChatGPT handle language variations and different user inputs in Angular JS applications? I'm curious about its adaptability.
Thomas White, great question! ChatGPT has the ability to learn from vast amounts of data, making it adaptable to various language variations and user inputs. However, it's important to provide clear instructions and context to ensure accurate responses. I'll delve deeper into this topic in upcoming posts to help developers harness the adaptability of ChatGPT in Angular JS applications.
This article caught my attention! Combining the power of Angular JS with ChatGPT can definitely bring Angular JS applications to the next level.
Ethan Reed, I'm thrilled that the article caught your attention! Indeed, the combination of Angular JS and ChatGPT has the potential to elevate applications to new heights. Stay tuned for more insights and practical guidance on utilizing this powerful integration!
Will there be any performance trade-offs when using ChatGPT with Angular JS? I'm concerned about the impact on application responsiveness.
Dylan Carter, that's a valid concern. While there might be some performance considerations, proper implementation techniques can help mitigate any impact on application responsiveness. In future posts, I'll share optimization strategies to ensure an optimal balance between the power of ChatGPT and application performance.
You did an amazing job explaining the potential of ChatGPT with Angular JS, Melissa! Looking forward to your next article.
Julia Turner, thank you for your kind words! I'm glad you found the explanations helpful. Your support and encouragement mean a lot. Stay tuned for more valuable content in my upcoming article!
I appreciate your effort in highlighting the benefits of this integration, Melissa. Angular JS developers can definitely benefit from embracing the power of ChatGPT.
Maxwell Hill, thank you for your appreciation! I'm glad you recognize the benefits this integration brings. Embracing the power of ChatGPT can indeed unlock new possibilities and enhance development workflows for Angular JS developers. If you have any specific areas you'd like me to cover in future articles, feel free to let me know!
I'm excited to learn about the practical implementation of ChatGPT in Angular JS projects. It seems like it can add a layer of intelligent interaction.
Victoria Turner, I share your excitement! Practical implementation examples can demonstrate the true potential of ChatGPT in adding intelligent interaction to Angular JS projects. I'll include such examples in my future articles. If you have any specific use cases in mind, please let me know!
Melissa, your article is informative and well-written! I'm looking forward to exploring the integration potential in my Angular JS projects.
Angelina Green, thank you for your kind words! I'm glad you found the article informative. I wish you great success in exploring the integration potential in your Angular JS projects. If you encounter any challenges or have interesting experiences, feel free to share them!
I'm impressed by how ChatGPT enhances the interactivity of Angular JS applications. Great insights, Melissa!
Steven Campbell, thank you for your kind words! I'm glad you recognize the enhanced interactivity that ChatGPT brings to Angular JS applications. The possibilities are vast, and I'll continue to provide valuable insights to help developers make the most of it!
This article has opened my eyes to a new realm of possibilities. I'm excited to experiment with ChatGPT in my Angular JS projects!
Laura Mitchell, I'm thrilled to hear that the article has sparked your curiosity! Experimenting with ChatGPT in your Angular JS projects can indeed unlock a new realm of possibilities. Feel free to share your experiments and discoveries along the way!
Do you foresee any challenges in incorporating ChatGPT into existing Angular JS projects that heavily rely on data bindings and complex logic?
Daniel Walker, incorporating ChatGPT into existing Angular JS projects with heavy reliance on data bindings and complex logic can indeed present challenges. It's essential to carefully consider the integration and ensure compatibility. In upcoming articles, I'll address this aspect and provide guidance on effectively incorporating ChatGPT into such projects without disrupting existing functionality.
The combination of ChatGPT and Angular JS has the potential to create truly intelligent and intuitive applications. Looking forward to exploring it further!
Nathan Roberts, I'm glad you share the excitement! Indeed, the combination of ChatGPT and Angular JS can pave the way for intelligent and intuitive applications. As you explore this combination further, feel free to share your findings and discoveries!
Melissa, this integration sounds fascinating! I can't wait to see what developers can achieve by combining Angular JS with ChatGPT.
Isabella Clark, I'm glad you're fascinated by this integration! The possibilities are indeed exciting. Developers can achieve remarkable results by harnessing the combined power of Angular JS and ChatGPT. Stay tuned for insights and examples that demonstrate the potential!
I'm a long-time Angular JS user, and this integration has caught my attention. Looking forward to seeing it in action!
David Adams, it's great to hear from a long-time Angular JS user! I'm thrilled that this integration has caught your attention. Seeing it in action will showcase the power and possibilities it brings to Angular JS projects. Feel free to share your experiences once you start implementing it!
As a frontend developer, I'm always looking for ways to enhance user interactions. Angular JS with ChatGPT seems like a perfect combination!
Valeria Nelson, I'm glad you find the combination of Angular JS with ChatGPT intriguing! Enhancing user interactions is indeed a primary goal, and this integration brings us closer to achieving that. If you have any specific ideas or areas you'd like me to explore, feel free to share!
With ChatGPT, Angular JS projects can provide more engaging and personalized experiences. Can't wait to integrate it!
Alex Turner, you're absolutely right! ChatGPT enables Angular JS projects to deliver engaging and personalized experiences to users. Integration opens up a world of possibilities. Once you integrate it, feel free to share your success stories and insights!
I'm excited to see developers explore the limits of this integration. It can truly change the way users interact with Angular JS applications.
Jessica Watson, I'm glad you share the excitement! Developers pushing the limits of this integration will undoubtedly reshape user interactions with Angular JS applications. The journey of exploration and discovery is just beginning. If you have any specific aspects or applications you'd like me to cover, please let me know!
Can ChatGPT be trained on specific domain-specific knowledge to enhance Angular JS applications tailored to certain industries?
Leo Davis, yes, ChatGPT can be trained on specific domain-specific knowledge to enhance Angular JS applications tailored to different industries and use cases. Custom training can enable applications to provide more accurate and context-aware responses within specific domains. In future posts, I'll delve into the process and benefits of domain-specific training to assist developers in harnessing its potential.
This is a fascinating read, Melissa! I'm eager to experiment with ChatGPT and Angular JS in my projects.
Ava Roberts, I'm thrilled that you find the article fascinating! Experimenting with ChatGPT and Angular JS in your projects will provide valuable insights and unlock innovative possibilities. Feel free to share your experimentation journey and any interesting outcomes!
Melissa, this is an insightful article! I'm confident that leveraging ChatGPT will take Angular JS applications to new heights.
Jack Wright, thank you for your kind words! I'm glad you found the article insightful. Indeed, leveraging ChatGPT has the potential to elevate Angular JS applications to new heights. Stay tuned for more insights and practical guidance!
Will the integration with ChatGPT introduce any compatibility issues with existing Angular JS libraries or plugins?
Eleanor Young, compatibility issues can arise when integrating ChatGPT with existing Angular JS libraries or plugins. It's important to carefully consider the dependencies and ensure compatibility. In future articles, I'll address this aspect and provide guidance on smooth integration without compatibility headaches.
I'm impressed by the potential of ChatGPT to enhance user interactions in Angular JS applications. Looking forward to practical implementation examples.
Samantha Hill, thank you for your comment! I'm glad you're impressed by the potential of ChatGPT. Practical implementation examples can shed light on the possibilities and inspire developers to explore similar interactions in their Angular JS applications. Stay tuned for upcoming articles with practical implementation guidance!
As a developer, I'm excited about this integration! ChatGPT can revolutionize how we build and interact with Angular JS applications.
Ruby Wright, I'm thrilled to hear your excitement! The integration of ChatGPT truly opens up new possibilities and revolutionizes the development and interaction landscape of Angular JS applications. Your enthusiasm further fuels my passion to provide valuable insights and resources on this topic. Thank you!
Do you foresee any challenges with the scalability of Angular JS applications utilizing ChatGPT in real-time interactions?
Nora Evans, scalability can be a challenge when incorporating ChatGPT for real-time interactions in Angular JS applications. It's vital to consider resource management and efficient interaction handling. In future posts, I'll dive into scalability considerations and provide guidelines for seamless integration even in resource-intensive scenarios.
I'm excited about the potential for more natural language interactions in Angular JS applications using ChatGPT. Can't wait to explore it!
Sophie Lewis, I'm glad you share the excitement for more natural language interactions! ChatGPT can unlock a whole new level of communication with Angular JS applications. When you start exploring it, feel free to share any interesting findings or use cases you come across!
ChatGPT seems like a powerful tool to bring conversational AI and natural language understanding to Angular JS. Looking forward to incorporating it into my projects.
William Turner, indeed! ChatGPT is a powerful tool that brings conversational AI and natural language understanding to Angular JS. Incorporating it into your projects will unlock new opportunities. If you encounter any interesting challenges or have implementation questions, feel free to reach out!
This article is fascinating, Melissa! I'm genuinely excited to utilize ChatGPT in my Angular JS projects.
Michael Cox, thank you for your kind words! I'm thrilled that you find the article fascinating. Utilizing ChatGPT in your Angular JS projects can have a significant impact. Feel free to share your experiences and any innovative use cases you discover!
As an Angular JS enthusiast, I'm thrilled about the potential of ChatGPT. It adds a new dimension to development!
Zoe Turner, I'm glad to hear your enthusiasm for the potential of ChatGPT! It indeed adds a new dimension to Angular JS development. Embracing it can lead to innovative solutions and enhanced user experiences. If you have any particular aspects or ideas you'd like me to explore, feel free to share!
Melissa, this integration has piqued my curiosity. I'm excited to see the possibilities unfold for Angular JS developers.
Olivia Mitchell, I'm thrilled that the integration has piqued your curiosity! The possibilities indeed hold great potential for Angular JS developers. I'll be unveiling more insights and examples to help you explore these possibilities. Thank you for your support!
Melissa, this article is an eye-opener! I'm excited to dive deeper into the world of ChatGPT in Angular JS.
Jayden Smith, I'm glad the article served as an eye-opener! Diving deeper into the world of ChatGPT in Angular JS will reveal fascinating possibilities. Feel free to share your experiences and discoveries as you navigate this exciting territory!
The combination of Angular JS and ChatGPT opens up so many doors in terms of user interactions. Your article is enlightening, Melissa!
Brooklyn Turner, I'm thrilled that you find the article enlightening! Indeed, the combination of Angular JS and ChatGPT opens up numerous doors, leading to innovative and interactive user experiences. If you have any specific use cases or scenarios in mind, feel free to share!
This integration is an exciting prospect for Angular JS developers. Your article provides valuable insights, Melissa!
Adam Stewart, I appreciate your comment! I'm glad you found the article valuable. The integration of ChatGPT in Angular JS is indeed an exciting prospect. Stay tuned for more valuable insights to help you make the most of this opportunity!
This integration opens up endless possibilities for Angular JS applications. I'm excited to explore the potential in my projects!
Mia Walker, I'm thrilled to hear your excitement! The integration of ChatGPT does indeed open up endless possibilities. Exploring the potential in your projects can lead to remarkable user experiences. Feel free to share your progress and any interesting outcomes along the way!
Thank you all for the wonderful comments! I'm glad you found the article interesting.
Great article, Melissa! ChatGPT seems like a promising technology for revolutionizing Angular JS.
I agree, Tom! The potential of ChatGPT to enhance Angular JS is exciting.
I think ChatGPT can greatly improve user interactions in Angular JS applications.
The possibilities seem endless! Can't wait to see how ChatGPT transforms Angular JS.
I'm not sure I fully understand the benefits of ChatGPT in the context of Angular JS. Can someone explain it to me?
Sure, David! ChatGPT is a language model developed by OpenAI that can generate human-like responses. By integrating it into Angular JS, we can create more interactive and dynamic user experiences.
Ah, I see. So it can help in creating chatbots or virtual assistants within Angular JS applications, right?
Exactly, David! ChatGPT can power chatbots or virtual assistants, enabling more natural and engaging conversations.
This article opened my eyes to the potential of ChatGPT in Angular JS development.
I'm curious to know if ChatGPT can handle multi-language support in Angular JS applications.
Good question, Samuel! ChatGPT has been trained on a wide range of languages, so it can indeed handle multi-language support.
I wonder if ChatGPT could be used for real-time chat applications in Angular JS.
Absolutely, Robert! ChatGPT can be integrated with real-time chat applications to provide intelligent responses on the fly.
What are the challenges in integrating ChatGPT into Angular JS?
Good question, Laura! One challenge is handling user inputs and ensuring ChatGPT understands the context. Proper training and validation mechanisms are required.
I see. So, it's important to fine-tune the language model to make it more context-aware.
Yes, Laura. Fine-tuning and continuous improvement are necessary to make ChatGPT contextually reliable.
What are some real-world examples where ChatGPT has been successfully integrated with Angular JS?
Good question, Jason! ChatGPT has been used to create virtual shopping assistants, customer support bots, and automated response systems in various industries.
Can ChatGPT handle complex user queries in Angular JS applications?
Yes, Sophia! ChatGPT has shown remarkable ability to handle complex queries and generate coherent responses.
I'm concerned about data privacy when using ChatGPT in Angular JS. How is user data handled?
Valid concern, John! OpenAI takes user privacy seriously. While ChatGPT interacts with user inputs, they don't retain any user data after the conversation ends.
What are the limitations of using ChatGPT in Angular JS applications?
Great question, Ella! ChatGPT may generate plausible-sounding but incorrect responses, so careful validation and monitoring are essential.
Do you have any tips for developers who want to integrate ChatGPT into their Angular JS projects?
Certainly, Oliver! Start with small experiments, validate outputs, and leverage community resources for best practices.
Can ChatGPT be used in mobile app development with Angular JS?
Absolutely, Emma! ChatGPT can be utilized in Angular JS mobile app development to create intelligent conversational interfaces.
I'm excited about the potential of ChatGPT in Angular JS projects. Any upcoming improvements we can look forward to?
Definitely, Aaron! OpenAI is continuously working on refining ChatGPT, addressing limitations, and expanding its compatibility with different frameworks.
How does ChatGPT handle user authentication and security in Angular JS applications?
Great question, Grace! User authentication and security measures need to be implemented separately in Angular JS applications while integrating ChatGPT.
I'm amazed by the advancements in natural language processing. ChatGPT seems like a game-changer for Angular JS.
Indeed, Daniel! Natural language processing technologies like ChatGPT have immense potential to shape the future of Angular JS and beyond.
Does integrating ChatGPT require advanced programming skills in Angular JS?
Not necessarily, Sophie! Though some programming knowledge is helpful, OpenAI provides user-friendly tools and documentation to facilitate integration.
Will ChatGPT revolutionize the way we interact with web applications developed using Angular JS?
Absolutely, Lucas! ChatGPT has the potential to revolutionize user interactions, making them more conversational, interactive, and personalized.
How does ChatGPT handle dialogue context in Angular JS conversations?
Good question, Alexandra! ChatGPT utilizes the context of the conversation history to generate relevant and coherent responses.
What are the potential ethical concerns of employing ChatGPT in Angular JS applications?
Ethical considerations are important, Joshua. Developers must ensure the responsible and unbiased use of ChatGPT to avoid amplifying harmful biases or generating inappropriate content.
I'd love to see some examples of how ChatGPT has improved existing Angular JS projects.
Certainly, Abigail! I can share some case studies and success stories where ChatGPT has enhanced user experiences in Angular JS projects. Please feel free to reach out.
What are some potential use cases for ChatGPT in Angular JS app development?
Great question, Chris! Some potential use cases include virtual assistants, chatbots for customer support, interactive tutorials, and intelligent content recommendations within Angular JS applications.
I wonder how ChatGPT can handle slang or informal language in user inputs within Angular JS?
Good point, Sophia! ChatGPT has been trained on a diverse corpus, including informal language, so it can understand and respond appropriately to slang or informal inputs.